I wrote Lua require
function based on the include
function found in /system/lib/head.lua:791
.
This function loads Lua modules similar to standard require found in Lua:
- it loads the file
- executes the file
- returns the module returned by included file
- caches the module, so next call to require will return already loaded module
How to use:
- put the source code of
require
function in yourmain.lua
:
function require(name) if _modules == nil then _modules={} end local already_imported = _modules[name] if already_imported ~= nil then return already_imported end local filename = fullpath(name..'.lua') local src = fetch(filename) if (type(src) ~= "string") then notify("could not include "..filename) stop() return end -- https://www.lua.org/manual/5.4/manual.html#pdf-load -- chunk name (for error reporting), mode ("t" for text only -- no binary chunk loading), _ENV upvalue -- @ is a special character that tells debugger the string is a filename local func,err = load(src, "@"..filename, "t", _ENV) -- syntax error while loading if (not func) then send_message(3, {event="report_error", content = "*syntax error"}) send_message(3, {event="report_error", content = tostr(err)}) stop() return end local module = func() _modules [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=143480#p) |
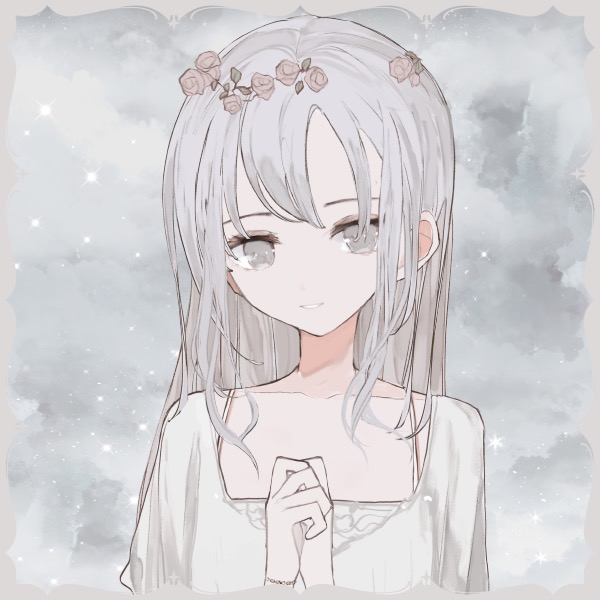
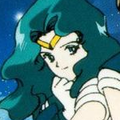

I wanted to share a few projects that I've been working on to prove out that making low-vision / blind accessible games is possible in PICO-8!
To get to the meat of it, here are the two games that my friend and I were able to put together that we built in prep for, and as a submission to the Games for Blind Gamers game jam:
Tiger & Dragon - https://jrjurman.itch.io/tiger-dragon
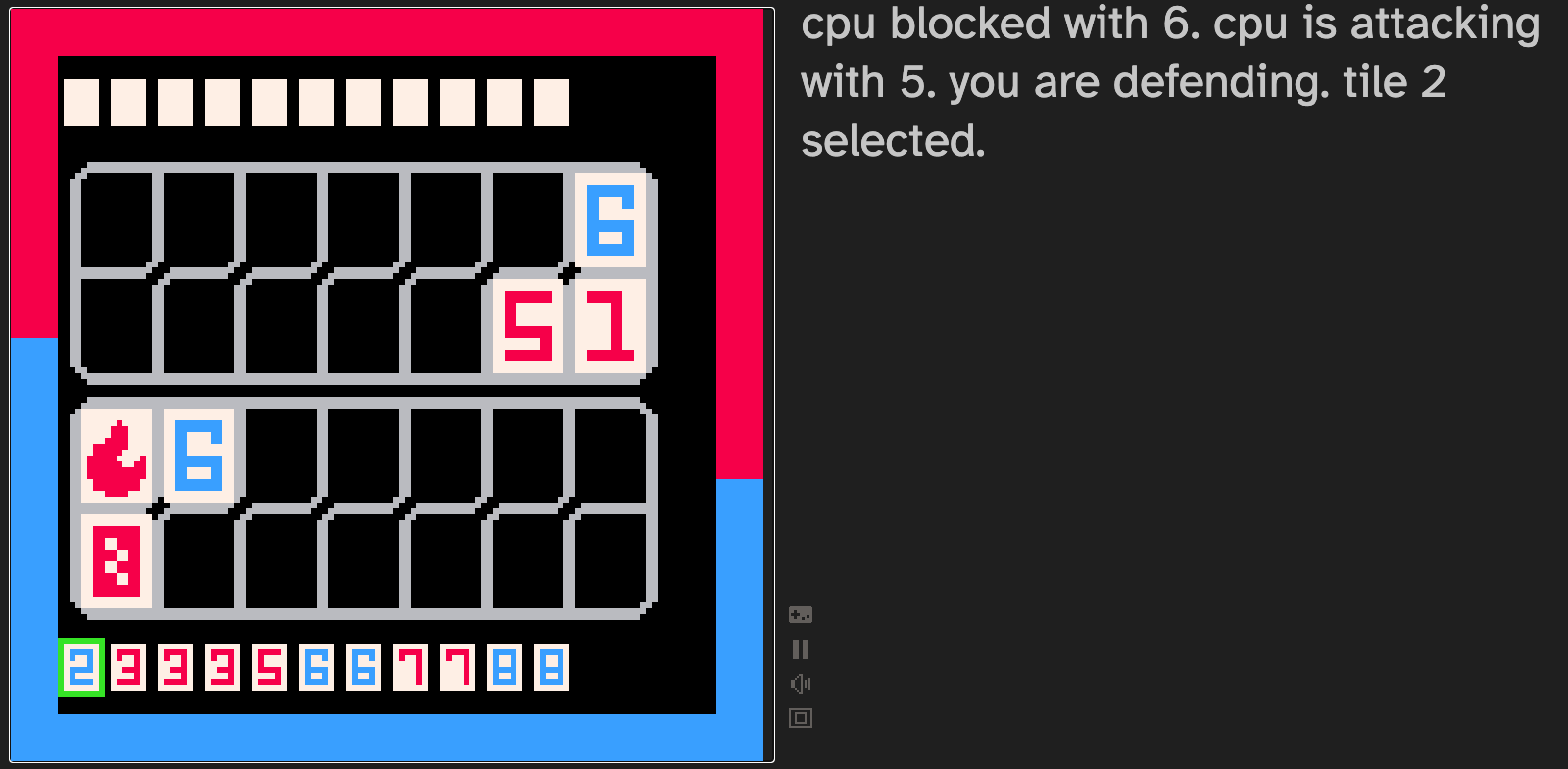
Lunch Gambit - https://jrjurman.itch.io/lunch-gambit
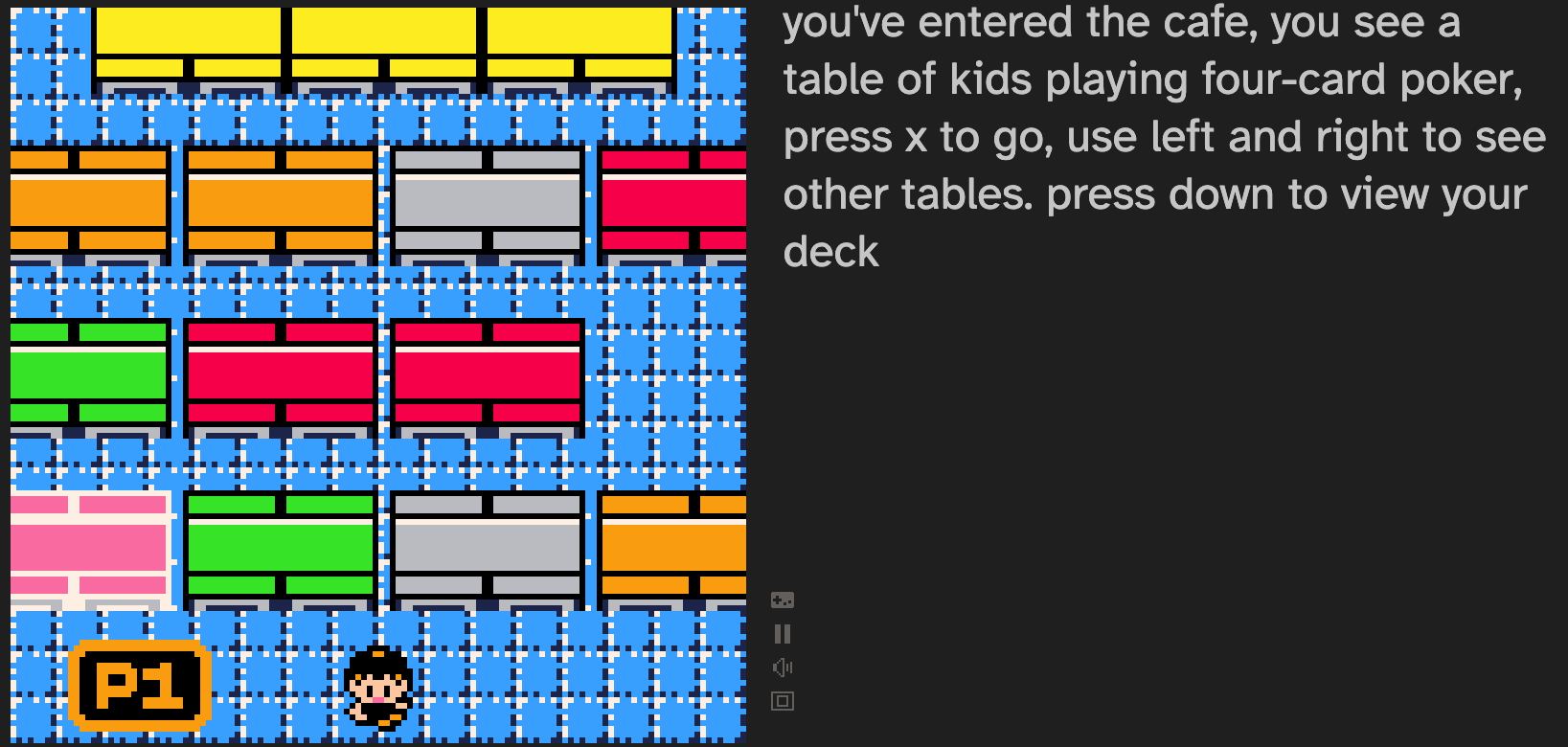
The games require a screen reader if you want to hear the text being read aloud, but the long and short of it is that the text on the right will be read out to screen readers. For people who might have trouble reading the text or need especially large fonts, the right side panel respects the font-size of the page, and allows players to zoom in if that would help readability.
The way that we were able to accomplish this is by having a special HTML template that reads the GPIO output from PICO-8 - pico-a11y-template
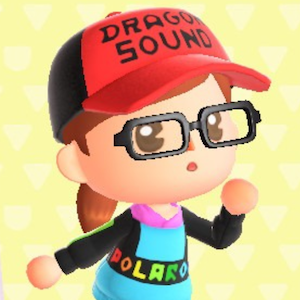
A limited graphic program for Picotron (stripped-down recreation of Desmos)
Planned features
- ❌ Make the different functions editable within the program
- ✅ Optimize rendering of axis and increments
- ❌ Improved UI (zoom slider, collapsible sidebar)
- ❌ Have the coordinates of a point appear when cursor hovers above a line
- ❌ Preferences menu to change theme, increments etc.
Version 0.2
- Added infinite axis and increments
- Equations are now -∞ < x < ∞, upgraded from -100 < x < 100
- Equation resolution now scales with zoom (higher resolution zoomed in, lower resolution zoomed out)
PREVIOUS UPDATES
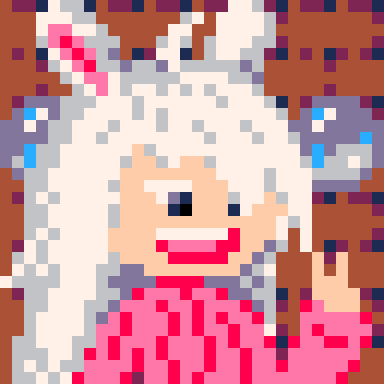
I made a handful of commandline utilities for picotron that you might find useful.
https://github.com/Rayquaza01/picotron-utilities
So far, Picotron Utilities has:
cat - print files
touch - create new files
tree - print tree view of a directory
wget - download a file using fetch()
grep - search in a file or recursively search through all files in a folder
frange - print a file or range w/ line numbers
pwd - print the current working directory
echo - print given arguments
fd - search for a file name
stat - print file status and metadata
Installing as a Yotta Package
You can install the utilities included in this cart with yotta util install #picotron_utilities

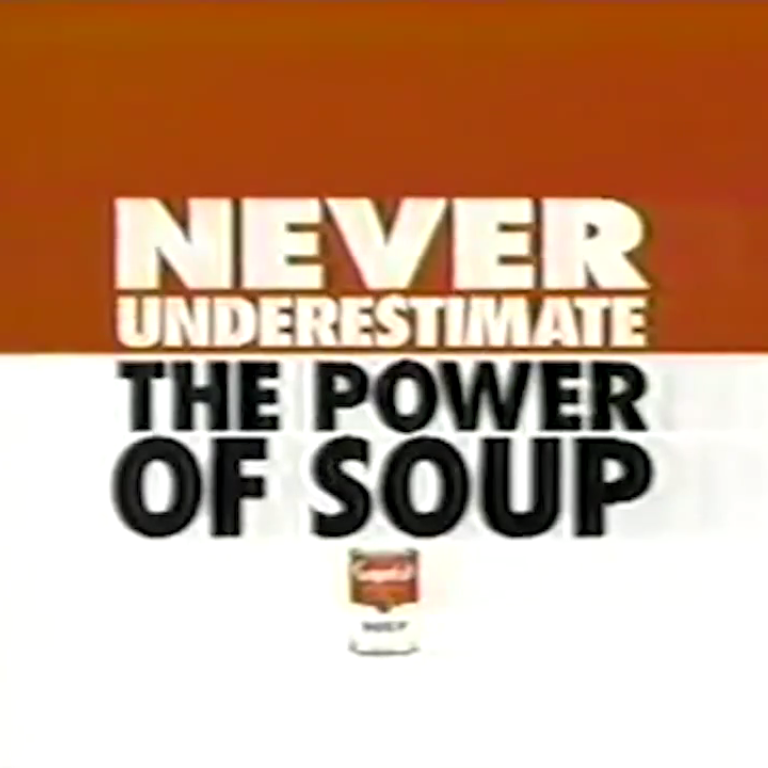
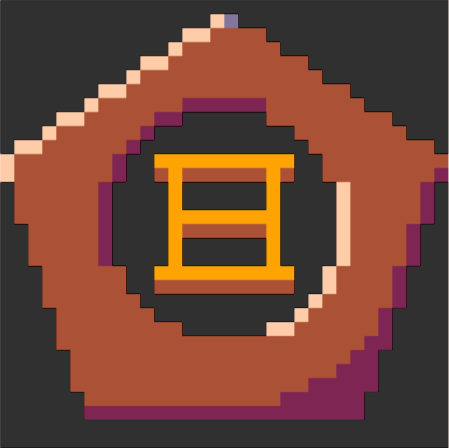
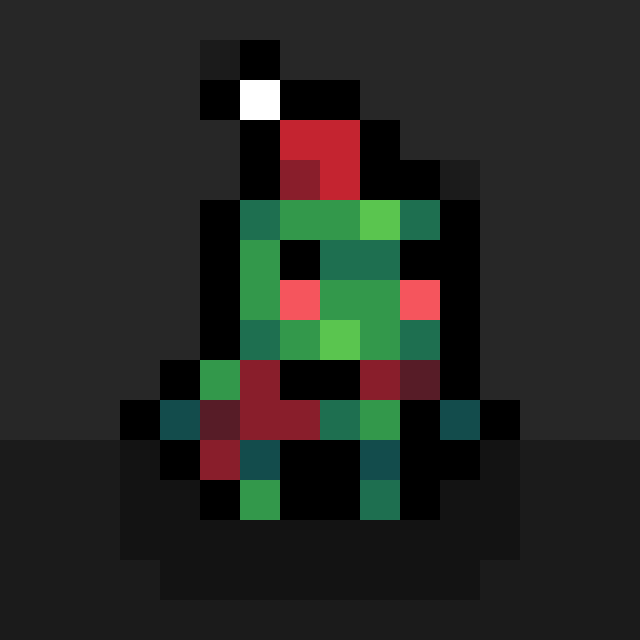
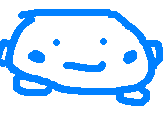
makes locs on desktop
usage: loc path (name)
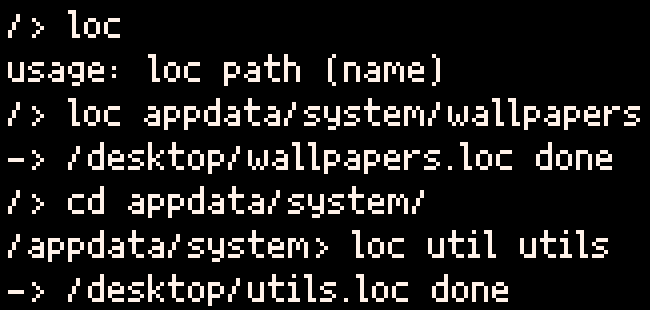
put code in appdata/system/util/loc.lua
you can change file name to whatever you like, like ln.lua to use as ln
function ext(str) for i=1,#str do if (str:sub(i,i)==".") return i-1 end return #str end local orig=env().argv[1] if (not orig) print("usage: "..env().argv[0].." path (name)") exit(1) if (orig:sub(#orig)=="/") orig=orig:sub(1,#orig-1) if (orig:sub(1,1)!="/") orig=fetch("/ram/system/pwd.pod").."/"..orig if (not fstat(orig)) print("file does not exist ("..orig..")") exit(1) local name=env().argv[2] and #env().argv[2]!=0 and env().argv[2] or orig:basename() name=name:sub(1,ext(name)) local targ="/desktop/"..name..".loc" if (fstat(targ)) print("loc already exists ("..targ..")") exit(1) [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=143431#p) |
While poking around in Picotron, I found myself wanting to view the contents of a file without opening it in the editor (via the edit command), so I wrote a little cat function. In order to implement it I needed to grab the current working directory so I went ahead and implemented a pwd command as well. Both of these are very simple and really just wrap existing Picotron functions for ease of use in the command line.
-=cat=-
local argv = env().argv local foldr = env().path if #argv < 1 or #argv > 1 then print("usage: cat filename") else local f = foldr.."/"..argv[1] print(fetch(f)) end |
-=pwd=-
local foldr = env().path print(foldr) |
To implement these, just save each in it's own project in the /appdata/system/util folder, using the name of each command as the filename- so copy the code for cat into 'main.lua' and cd into /appdata/system/util and type save cat
. Same goes for pwd. Once you do this, you can run them straight from the Picotron command line.
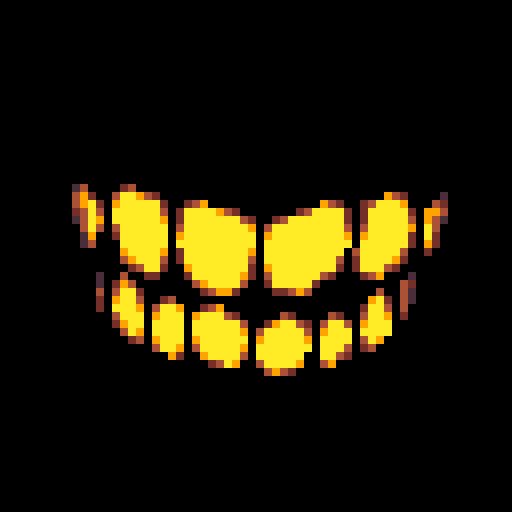
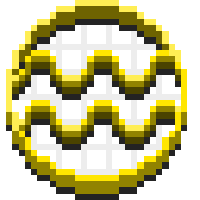
Software Standards
Hello, I'm here to propose some software standards. This post isn't supposed to be all about me, I want the community to come together to make sure we're not stepping on each others toes whenever we make software, games, ect. Currently it's sorta the wild west, so the only thing I can suggest (which I've done with SLATE) is that we put application installs and preferences inside of their own folder within the /appdata/
folder.
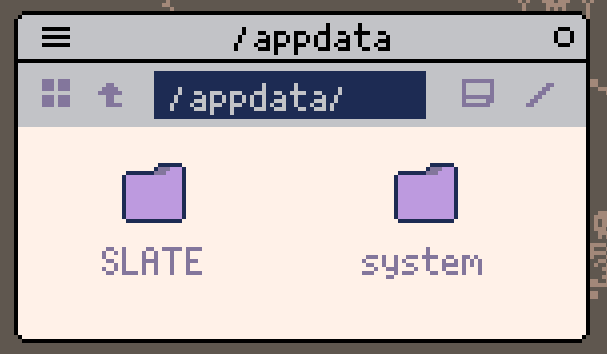
I don't want to make standards nobody is going to use, so please comment on this post to talk about any standards for anything that could be useful to everyone.
Personally a new standard I've been thinking of is creating a central libraries folder that software can prompt downloads for libraries it might need, so that no one has to reinvent the wheel every time they make an application (although it is so early I don't think this needs to be a focus yet).

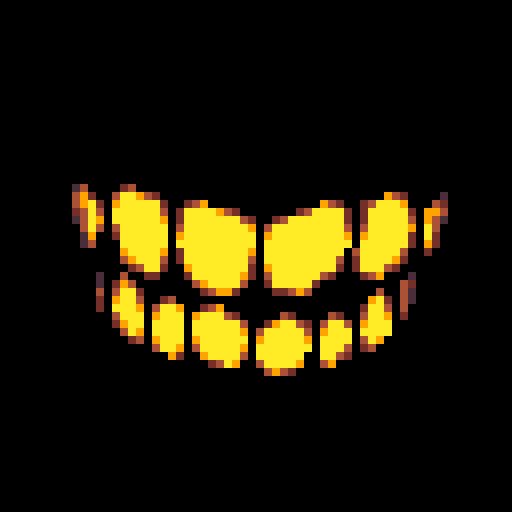


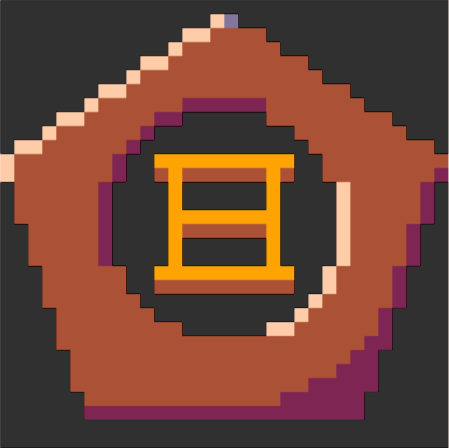
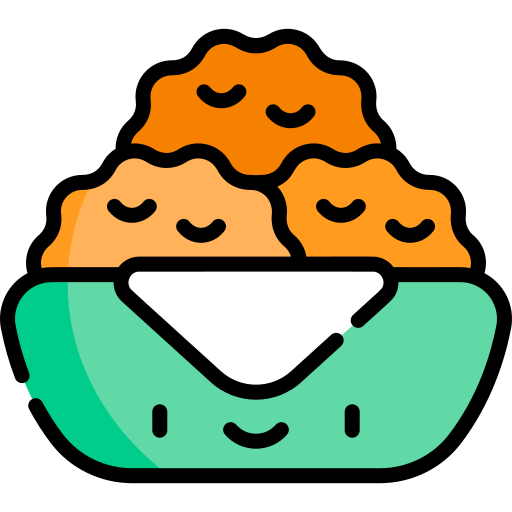
Some Discord servers have been quite busy lately and we've been discovering (less trivial) things here and there than the officially documented things and I thought it was good to have them available in the BBS instead of losing them in a Discord server's chat log. I'll try to update this post as more discoveries are uncovered.
Don't hesitate to write more discoveries in the thread.
Notice
At the moment I'm writing this post, we're running 0.1.0b, stuff might change in the future, nothing is set in stone for now.
POD is data, data is POD
Most of the file one will find on their systems are just POD files with an extension. The extension allows the OS to determine which app to launch but the internal data seems to work the same for all formats: they're PODs.
podtree (your_file)
will pop a tool window containing a tree interface with the guts of your file. So you can determine how those file are formatted.
For instance the default drive.loc
file, once opened, will show that it has only one property: location. So creating a table looking like that my_table = {location="/path/to/a/program.p64"}
and then saving it with store(my_shortcut.loc, my_table)
will efectively crete a new shortcut.
.jpg)

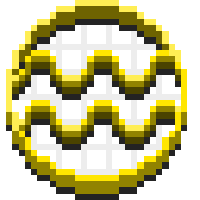
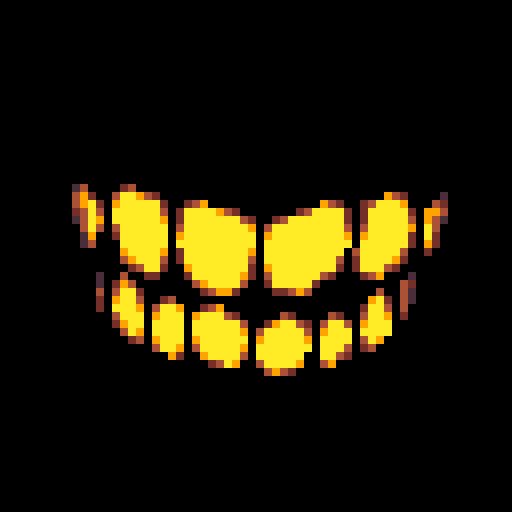
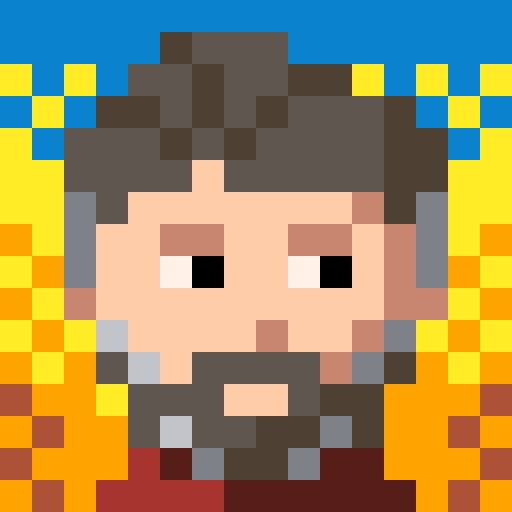
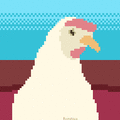


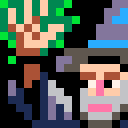
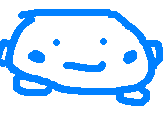
so the new picotron is finally out, and the browser version is no where to be seen! even though pico-8 and voxatron had browser versions! but I think that the reason lexaloffle cant make the browser version is just because they can't figure out what to do with the workstation, and I don't blame them! it would be hard to make it a seamless transition between the full version and the browser version, and the only way I could ever think of is using a default background and limiting use to just the cartridge. I hope lexaloffle will make the browser version one day to open the field to wider audiences!
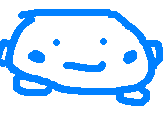
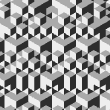
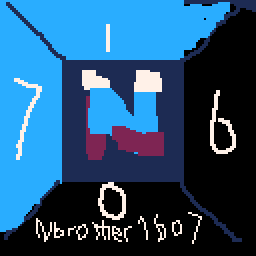
Overview
PICO DAW is a digital audio workstation for creating PICO-8 tunes. If you've ever been frustrated by the piano style layout keyboard layout of the music editor or just wished you could draw your music, PICO DAW is for you. It's a mouse-based alternative for tune creation.
THIS CART IS A WORK IN PROGRESS AND MINIMALLY TESTED. Consider it alpha. Save early and often! Please report any bugs you find.
Quick Start
- Open the PICO-8 console and enter
load #daw
. - Save the cart under a new name.
- Optional: Add any desired custom instruments in SFX slots 0 through 7.
- Edit music patterns for length, looping, channels, and SFX assignments.
- Edit SFX for length, speed, and filters.
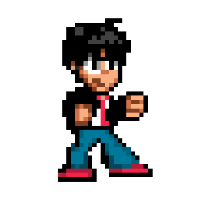
Hi! I have been trying to run code from multiple lua files for organization's sake. Since the "require" keyword from lua still seems to not work, I've been trying to figure out other ways. I've been scouring what I could find about picotron and the only way I could figure to import code from another lua file was this, based on what I could understand of the proggy code:
this lists out the dependencies and runs the text in those files as functions as far as I understand it:
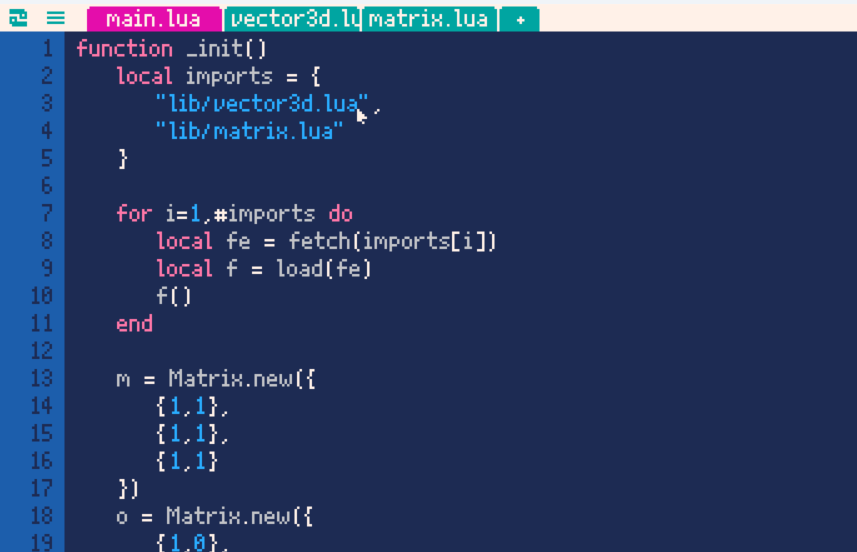
here's the matrix lua file:
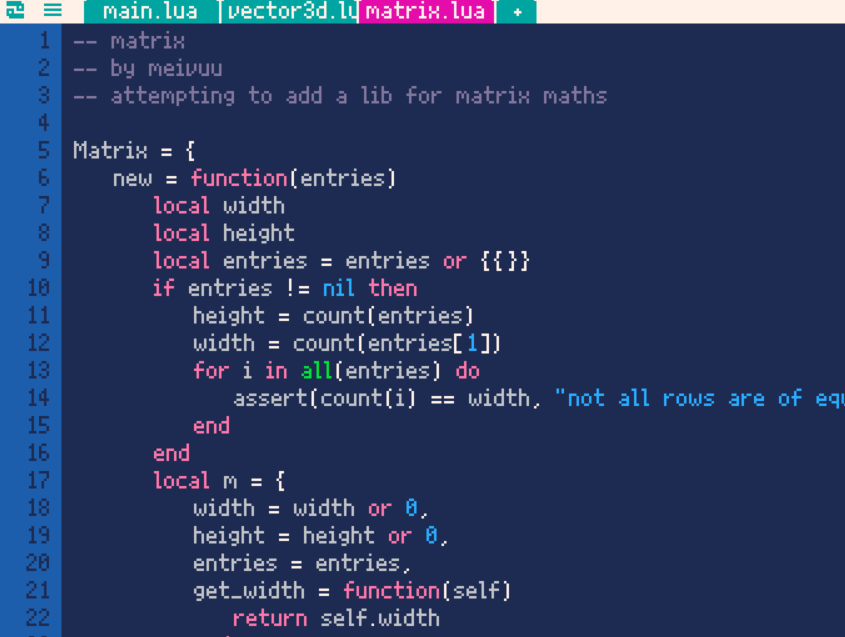
and here's the folders in the loaded cart:
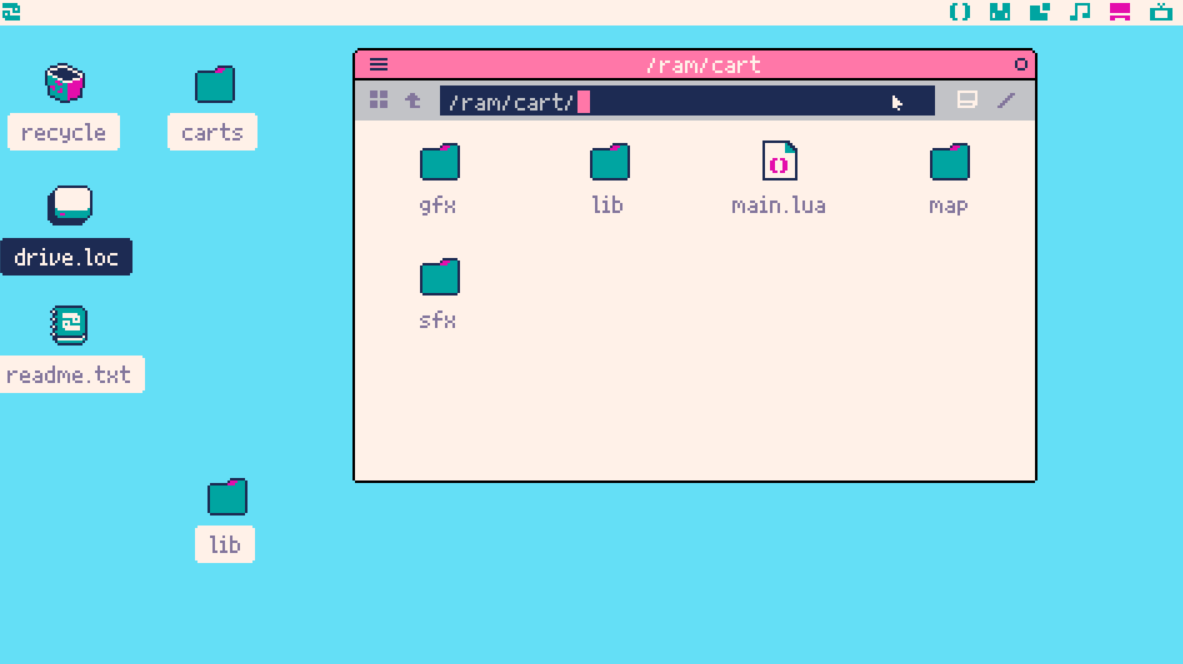
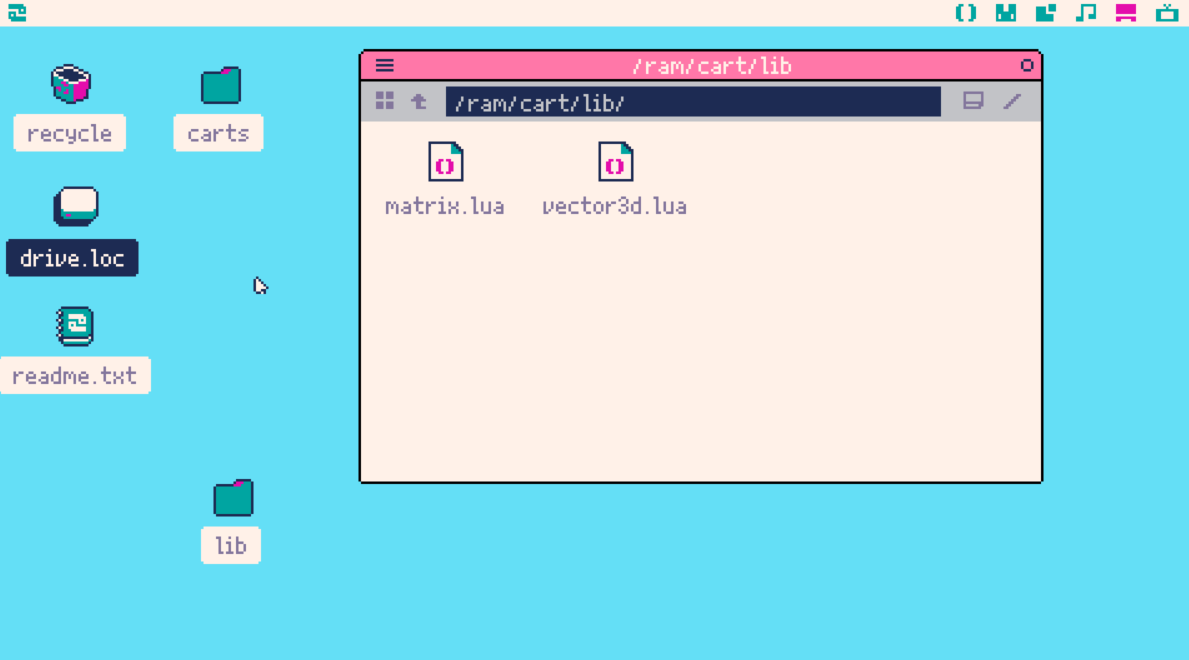
if anyone else has figured out how to do this in a better way, please let me know because I'm probably doin this in a very silly way. but otherwise, I hope this is helpful!!!
(sorry if this is in the wrong category, picotron isn't showing up for me in the category option for posting, unless i click the post button directly from the cartridges page. edit: I figured out how to post in the right category, I will do that properly next time!)

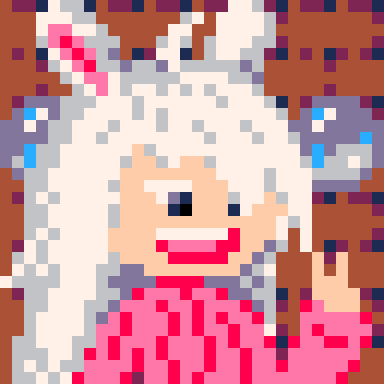


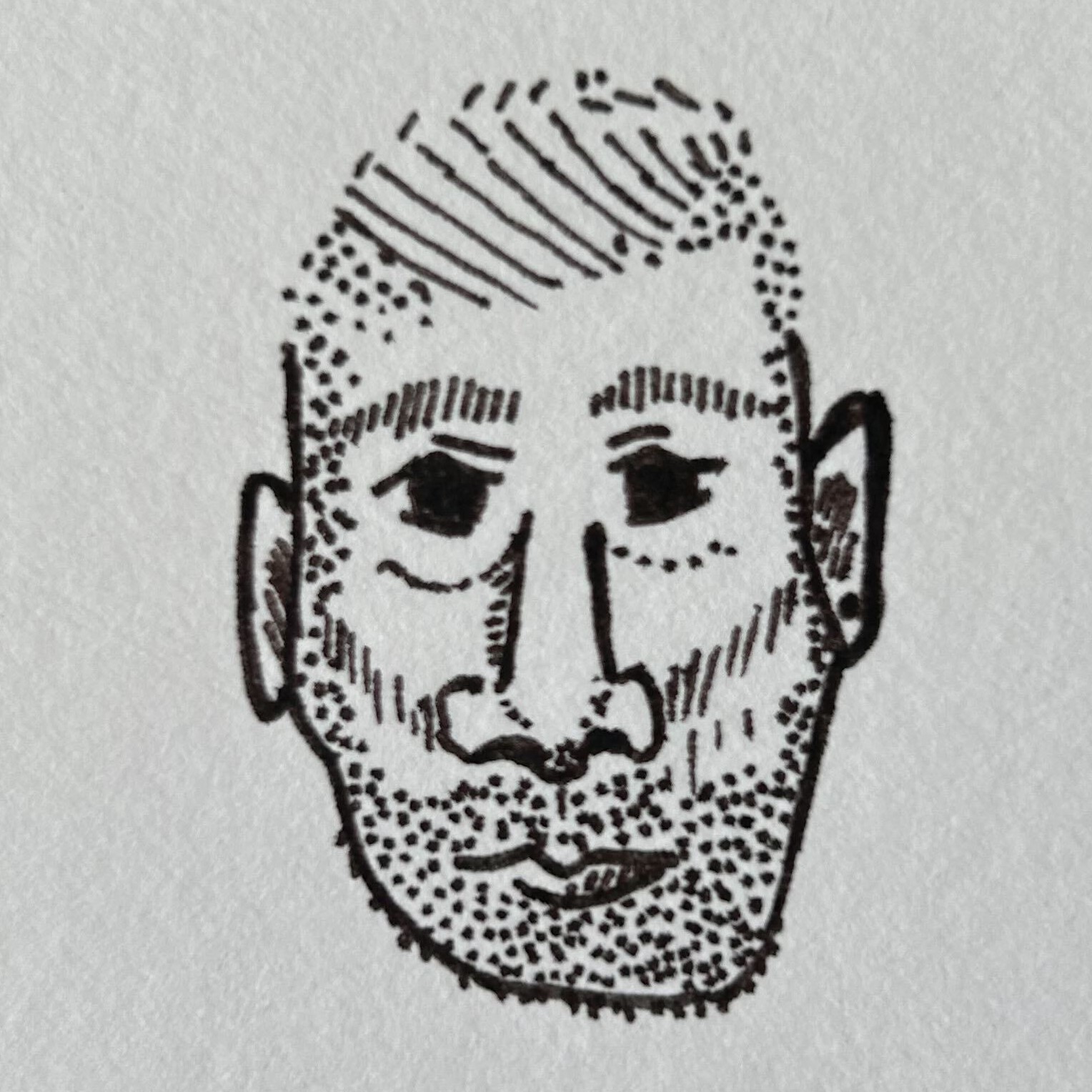
A small program for displaying running processes, capable of killing them from the application menu or with a shortcut.
I mainly made this simple project to figure out the picotron gui library for use in other programs I'm working on.
UPDATE 0.3:
- The window now remembers its size and position when closed
UPDATE 0.4:
- The app now only refreshes data every second instead of every frame, making rapidly changing numbers readable
UPDATE 0.5:
- Window settings are now stored in a different file, among other internal changes
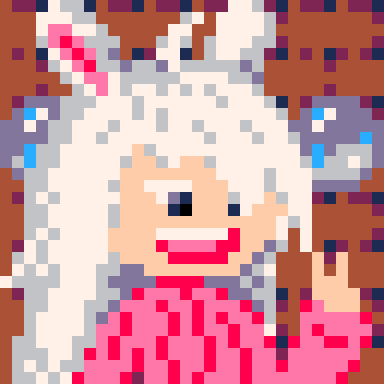

Wordle for Picotron
This is a basic wordle clone. A green letter is in the correct position, a yellow letter is in the wrong position, and a gray letter is wrong.
This uses an invisible text editor to let you type the letters. Press enter to submit your guess.
If you enter a guess that is less than 5 letters, it will clear your text input. There is also no check to see if your guess is a real word, so you can enter random letters if you want. The word list I used is the official wordle list, which I got from here.
Hope you enjoy it!
Changelog
[2024-03-17]
- Gray boxes on the line you're typing always display
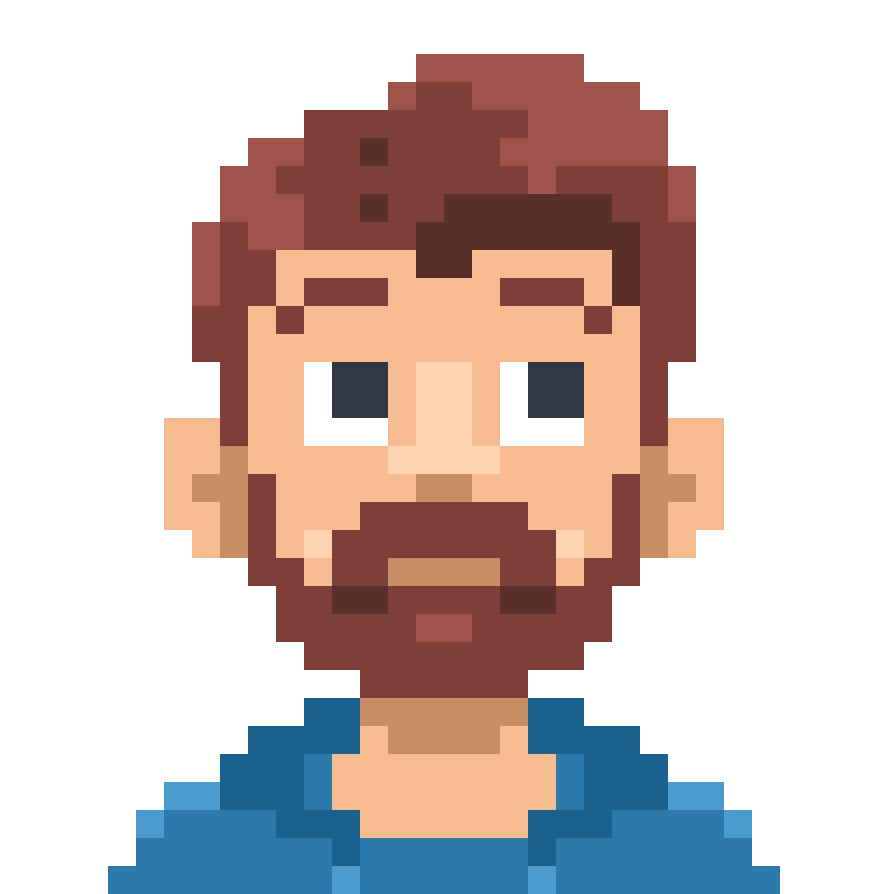
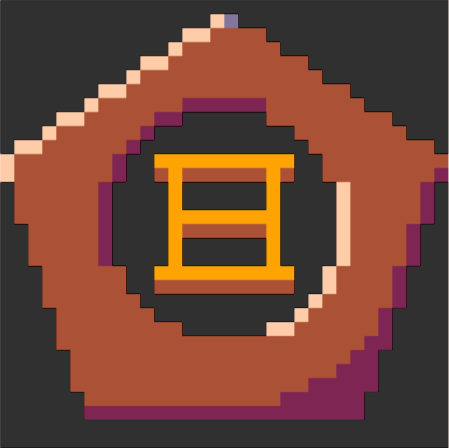
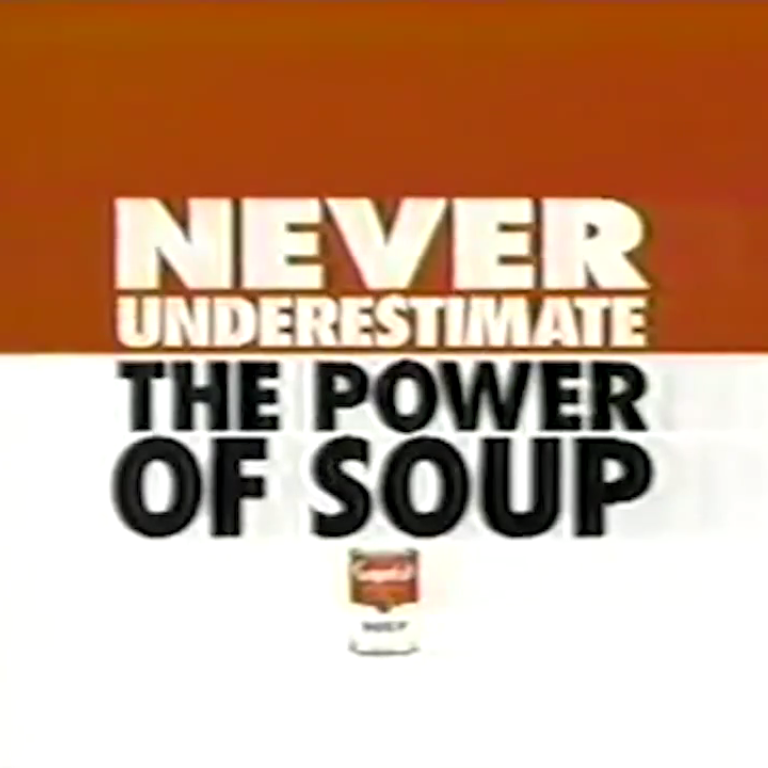

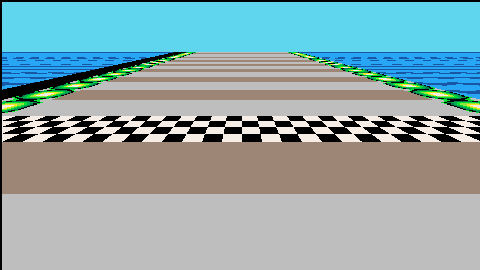
A simple mode7-like effect, as seen in multiple SNES titles like Super Mario Kart and F-Zero.
This is archieved with the tline3d
function, called once per line on the screen. Change the debug
variable in the source code to true to see what the tline3d
function indexes on the real tilemap.
The effect uses around 40% of the CPU

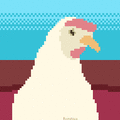
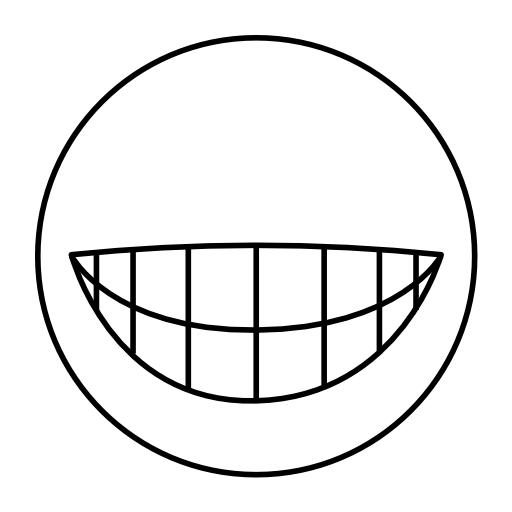
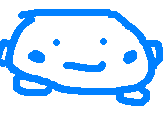
