I extracted all the public names of functions and tables that are visible to Picotron, it might be useful to explore everything that is available
printh doesn't seems to work like in Pico 8 so I couldn't easily make it into a text format, so here is just a screenshot of all the globals functions (in white), tables (in dark blue) and others (in red):
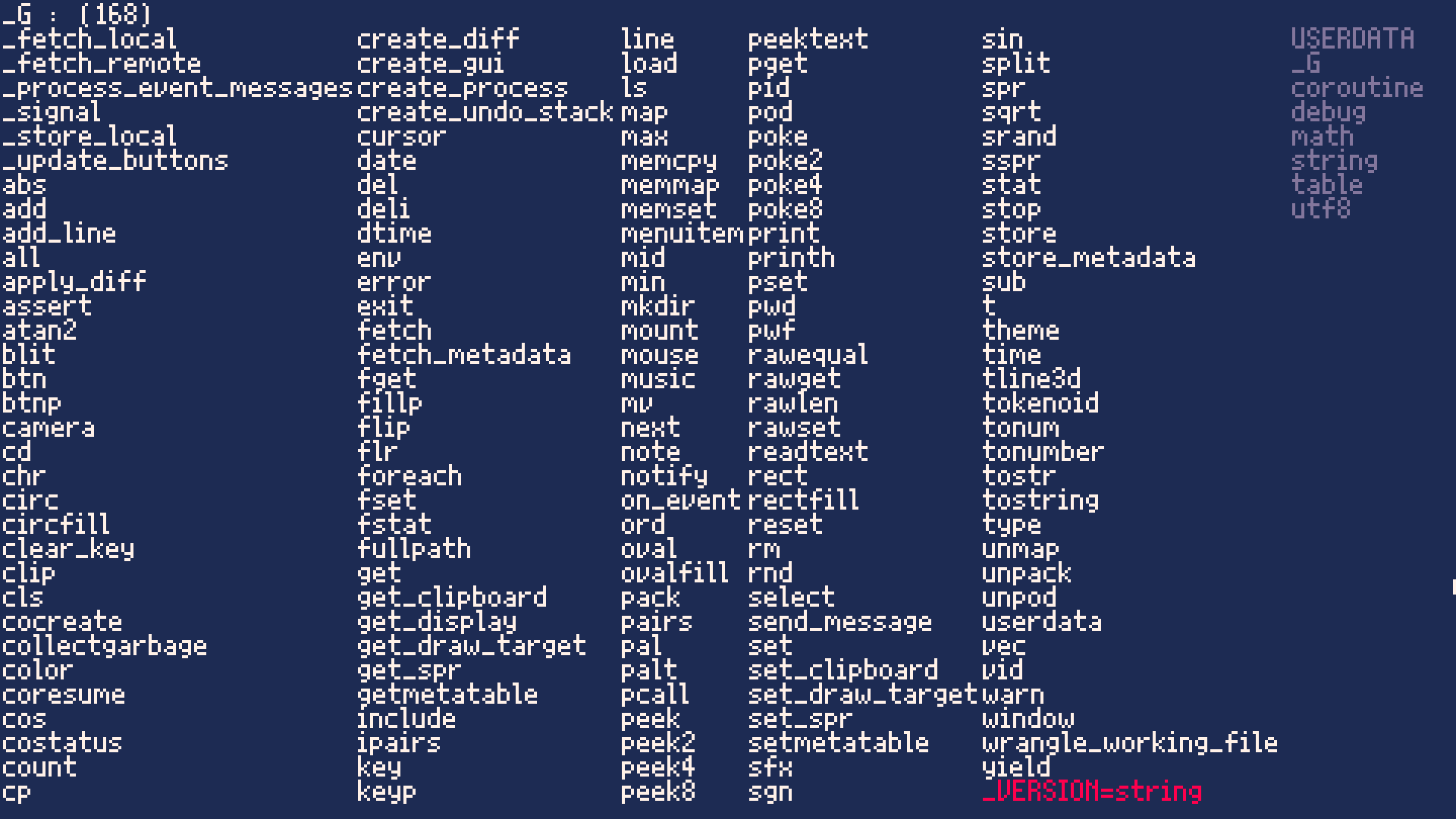
And I also got the functions inside all the tables:
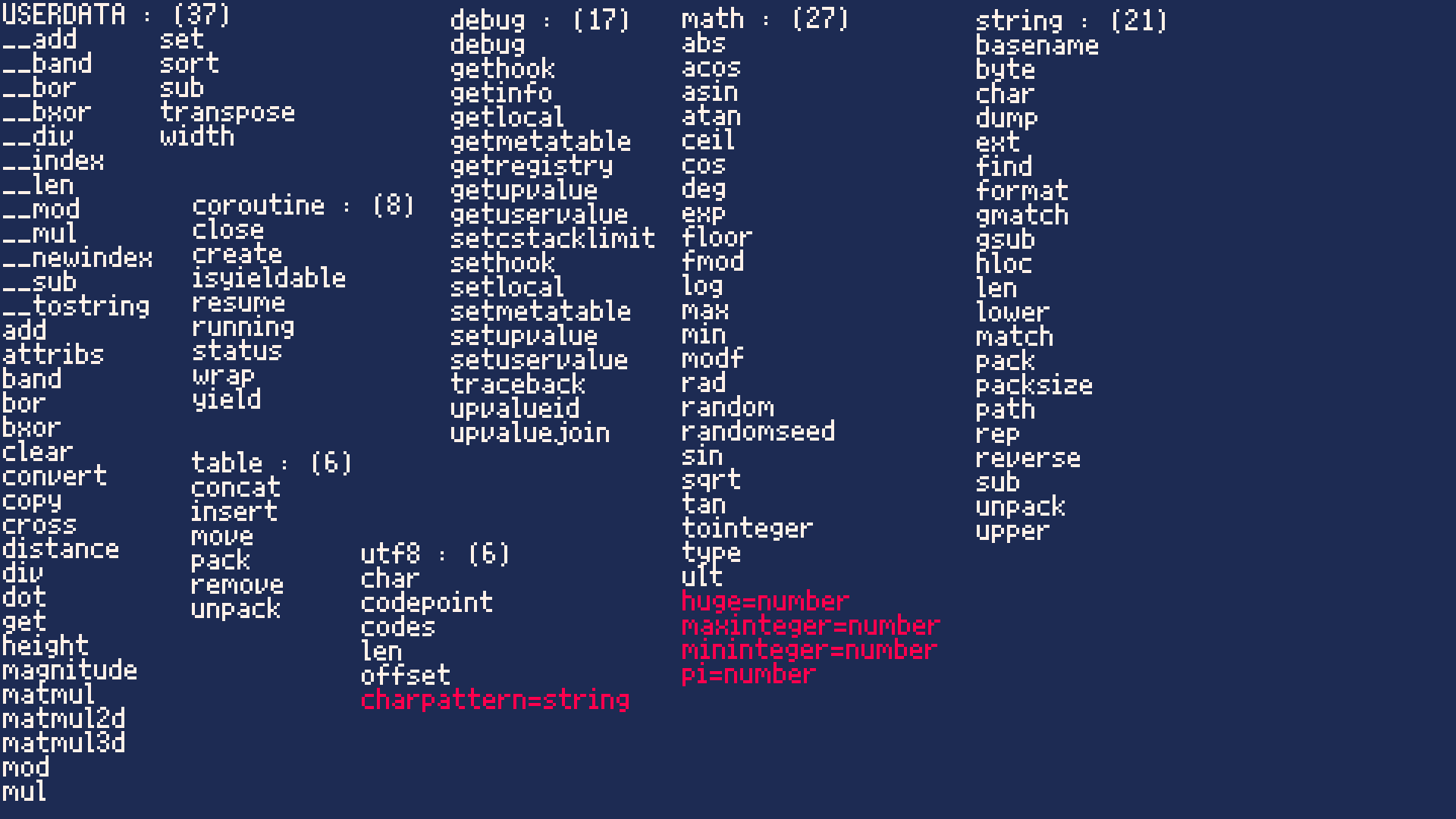
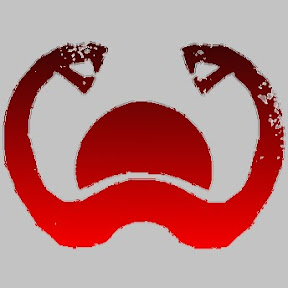
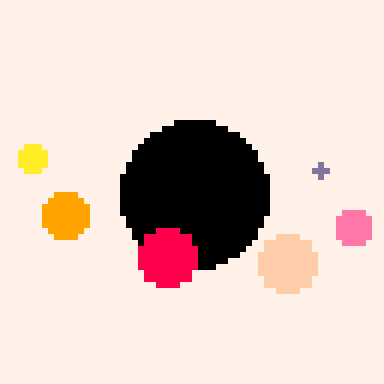


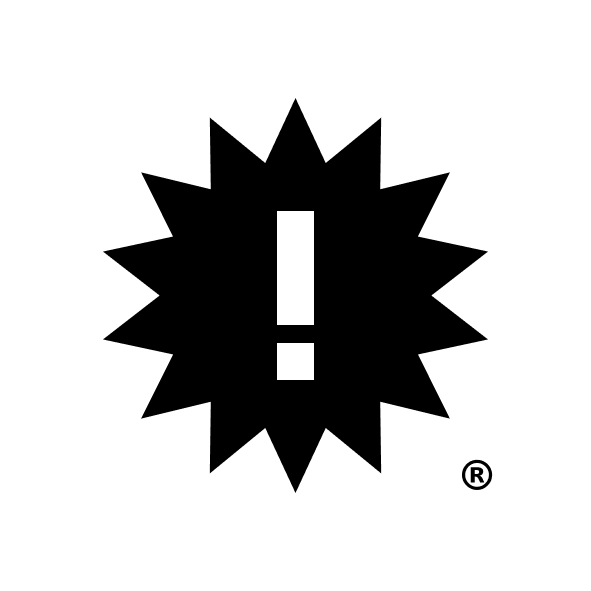
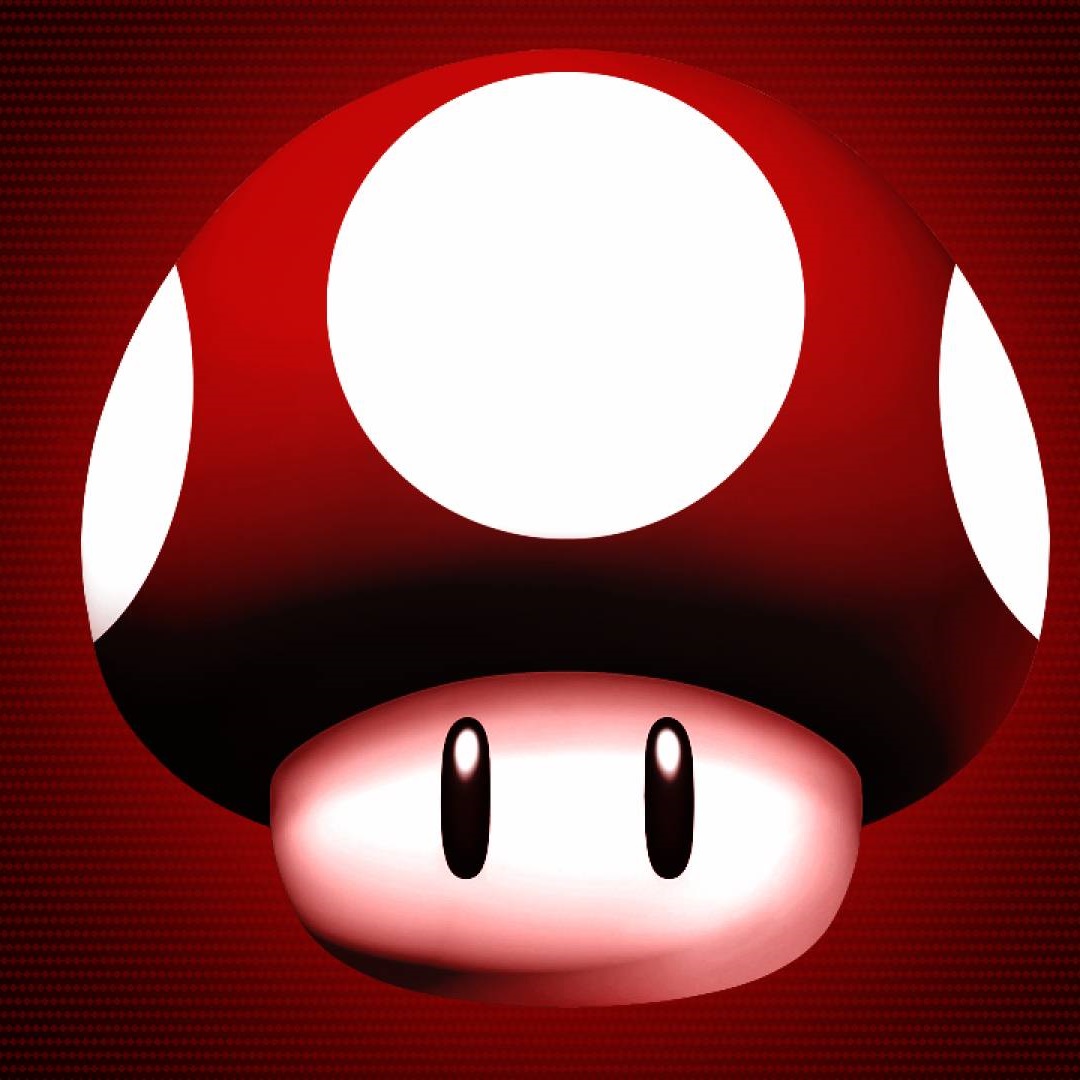
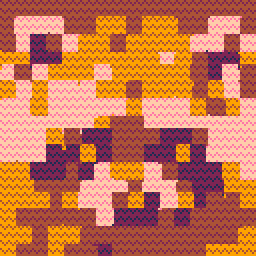

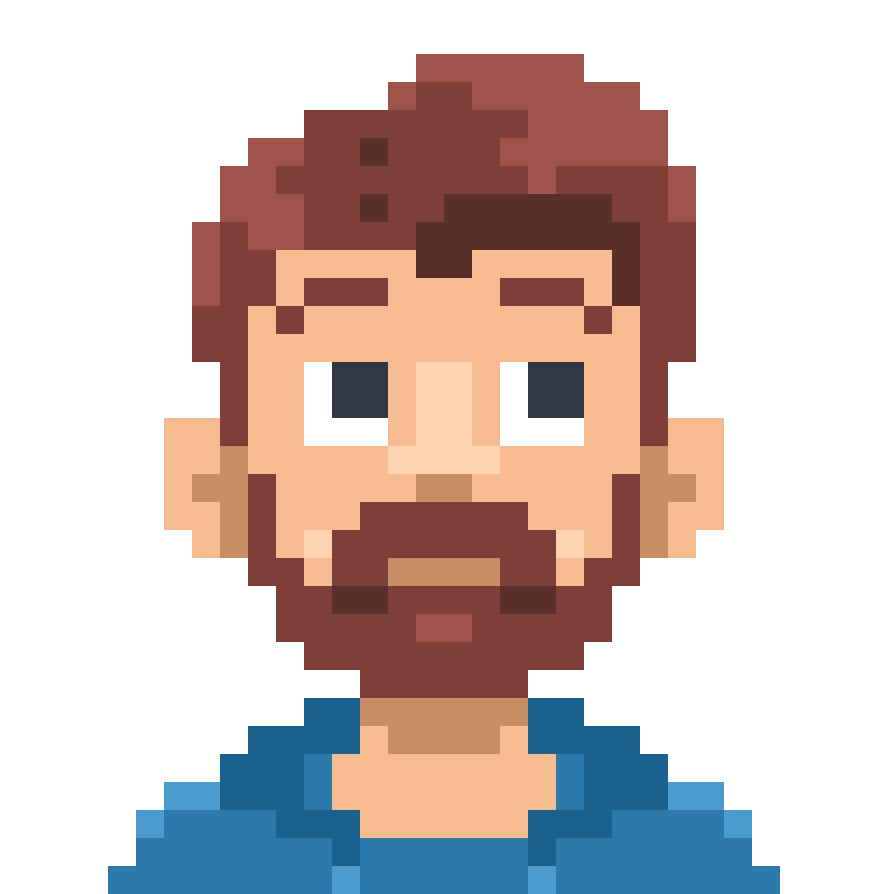
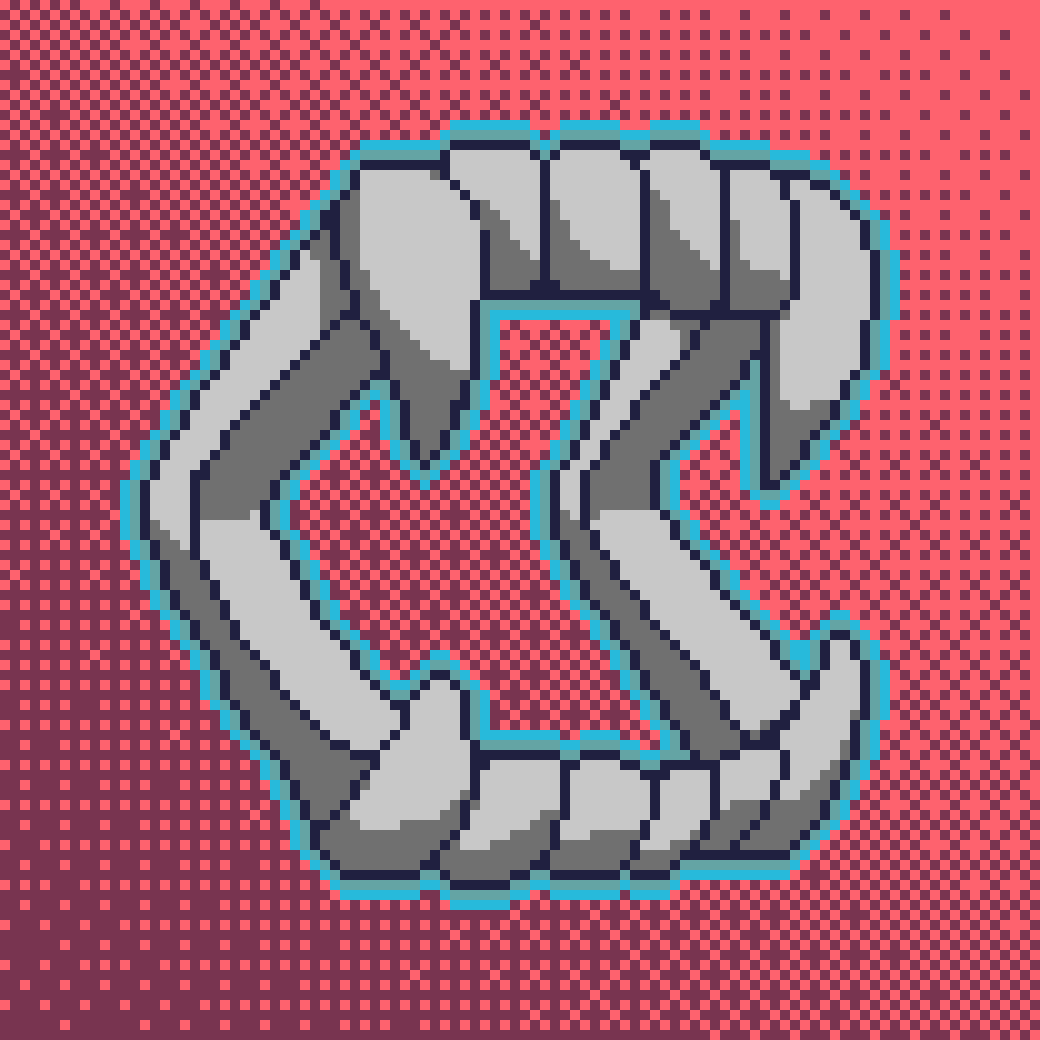
Why isn't there an english speaking Picotron telegram group chat already?
Feel free to join and chat about Picotron related stuff. This console is going to be huge!
https://t.me/picotron_community
I did it to not spam the forum with repetitive issues
@zep tell me if this isn't something you like
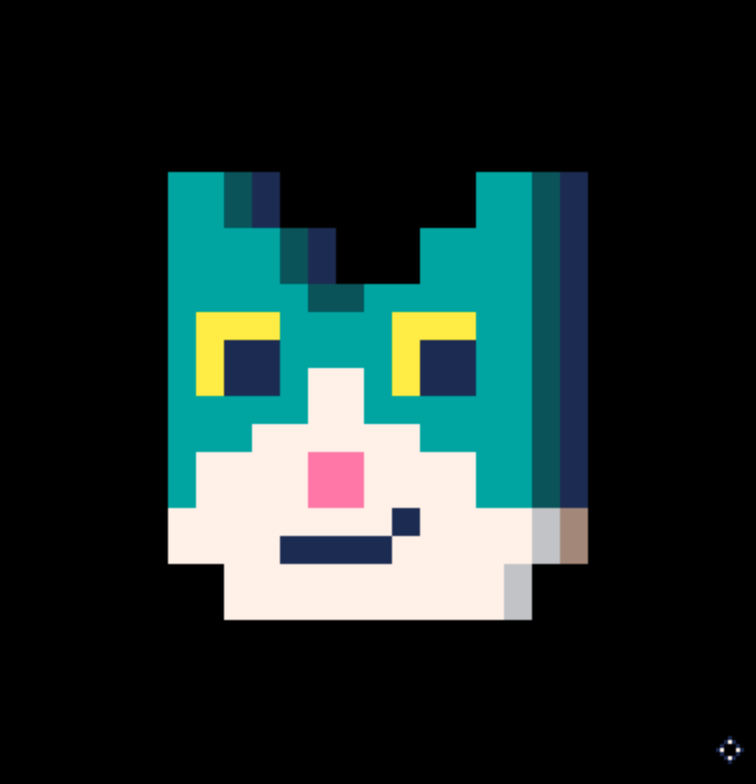
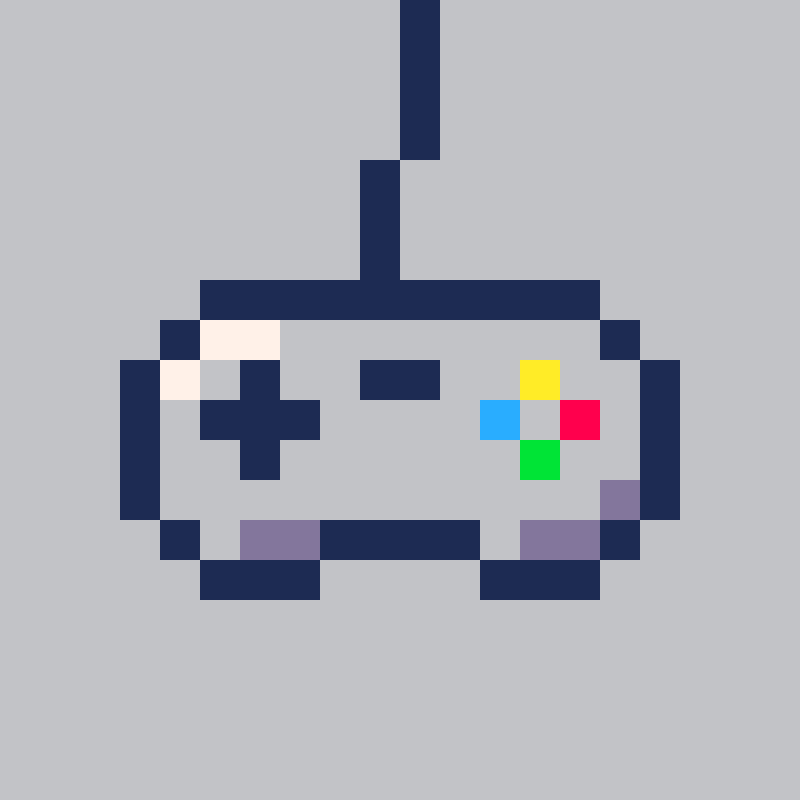
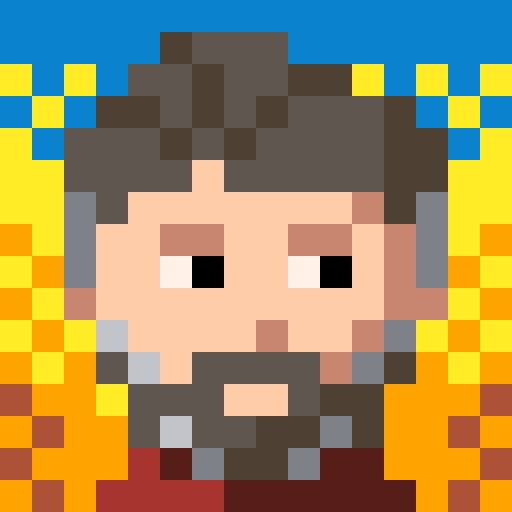

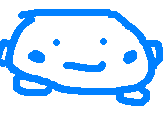
I'm writing a simple toy program to get familiar with the API.
I have a string, "Hello World!", that I am intending to print centred horizontally and vertically, adapting to window dimensions on the fly.
In the top line in the screenshot I am printing it in one go, in the bottom line I am printing it letter by letter to enable a "rainbow" effect.
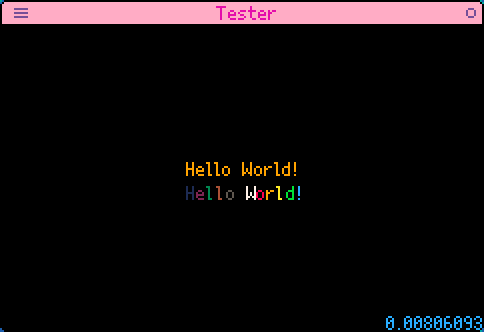
I noticed the two strings are spaced differently, and I realised Picotron in fact uses a variable-width font. I was allotting 5 pixels for each character in my printing routine, but as it turns out the lowercase "l" is one pixel narrower, while the uppercase "W" is one pixel wider.
How do I deal with the variable width of characters for printing and layout work? Is there an API function to get the exact dimensions of a character?
My code:
[hidden]
---[[ Comment/uncomment to run fullscreen/windowed window{ width = 240, height = 120+32, title = "Tester" } --]] text="Hello World!" function _draw() local width = get_display():width() local height = get_display():height() local align = width/2-((string.len(text)-1)*5/2) -- Assuming char width 5 cls() --Print all text in one go print(text, align, height/2-8, 9) --Print text letter by letter for i = 1,string.len(text) do print(string.sub(text,i,i),align+5*(i-1),height/2+4,i) -- Assuming char width 5 end --Print CPU usage print(stat(1), width-48, height-7) end function _update() end |
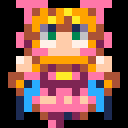
just a port of a tweetcart of mine to the new system :)
move this into /system/screensavers/
and it'll show up as an option in your settings
If you run it on its own as a cart, you'll want to run reset() vid(0)
in the console afterwards to get things back to normal
edit: if you want it to be permanently available, you need to put it in /appdata/system/screensavers
(otherwise, you need to re-add it every time you start picotron). create the folder by copying the system folder: cp /system/screensavers /appdata/system/screensavers
A port of my recent pico-8 minesweeper to Picotron.
I want it to fully adjust to the system theme, for now it just uses it for the mine counter :)
UPDATE 0.2:
- No longer crashes on continue
- The time is displayed correctly
UPDATE 0.3:
- Beginner, intermediate and expert difficulty selection from the menu (but windows don't resize automatically yet)
- Lots of windows at once
- Game doesn't crash on victory
- Added missing sound effects
UPDATE 0.4:
- Changed how the program creates new windows, now new instances use the correct about menu
UPDATE 0.5:
- Windows now open in a cascade from the previous window (but difficulties beyond beginner don't yet open with the correct size)

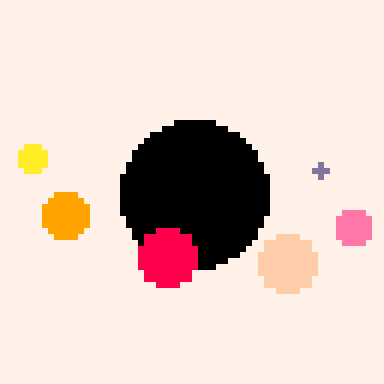

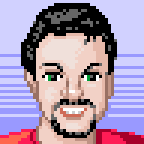

.jpg)
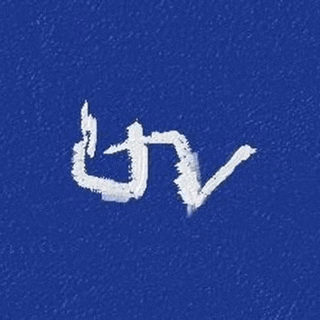
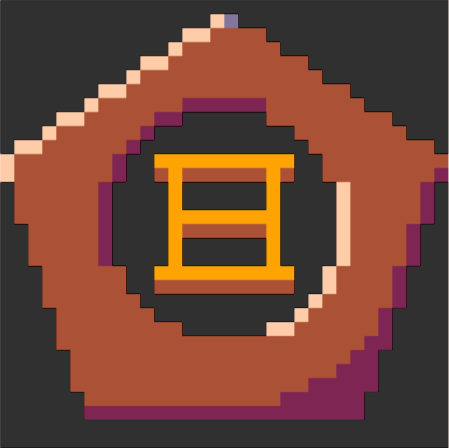
Hope this is the right place to post this. First time programmer, and I've been having a ton of fun trying to make a game in PICO-8.
I've been following Lazy Devs excellent Roguelike tutorial series on YouTube, but I've been running into a consistent issue.
For my game, I decided to make my player sprite 16x16. However, Lazy Devs' tutorial (and most of the ones I've seen online) assume an 8x8 character sprite. This is specifically giving me grief when it comes to collision. Right now, my player bounces off the walls on the top of the screen, and ignores collision entirely when moving left into them.
From what I can gather, the collision on a 16x16 sprite is calculated from the top left tile. Ideally, I'd love collision to be calculated from the bottom two tiles on the sprite. What's the best way to adjust/offset my collision to account for a larger sprite?
I've attached my cartridge to this post. If someone could point me in the right direction, that would be much appreciated.
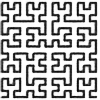
hi there
edit: i've updated this a bit and put this into an installer cartridge (here) which can also be installed via yotta, a package and dependency manager.
i've already wanted to throw out what i'm working on and start a cart from scratch a few times and am accustomed to being able to use PICO-8's reset
to do so. this doesn't seem to work well here, and i don't like just closing and reopening picotron. we have save
and load
but not reset
or new
. so... i made new
.
you can place this code in /appdata/system/util/new.lua
:
-- remove current cart and replace with template cart source_template = "/appdata/new/template.p64" default_filename = "/untitled.p64" if(fstat("/appdata/new") != "folder") mkdir("/appdata/new") -- (most of this copied from /system/util/load.lua, thx zep) -- remove currently loaded cartridge [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=143060#p) |
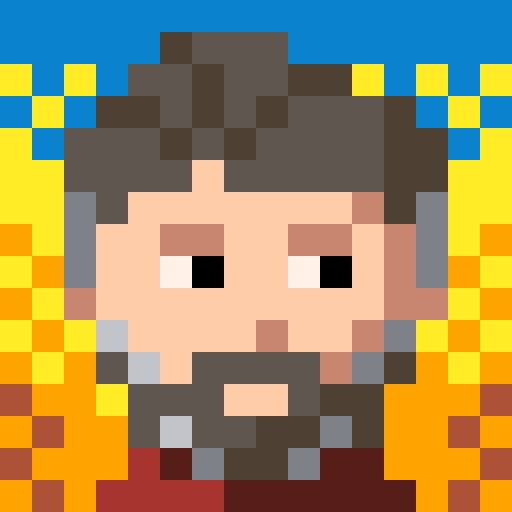
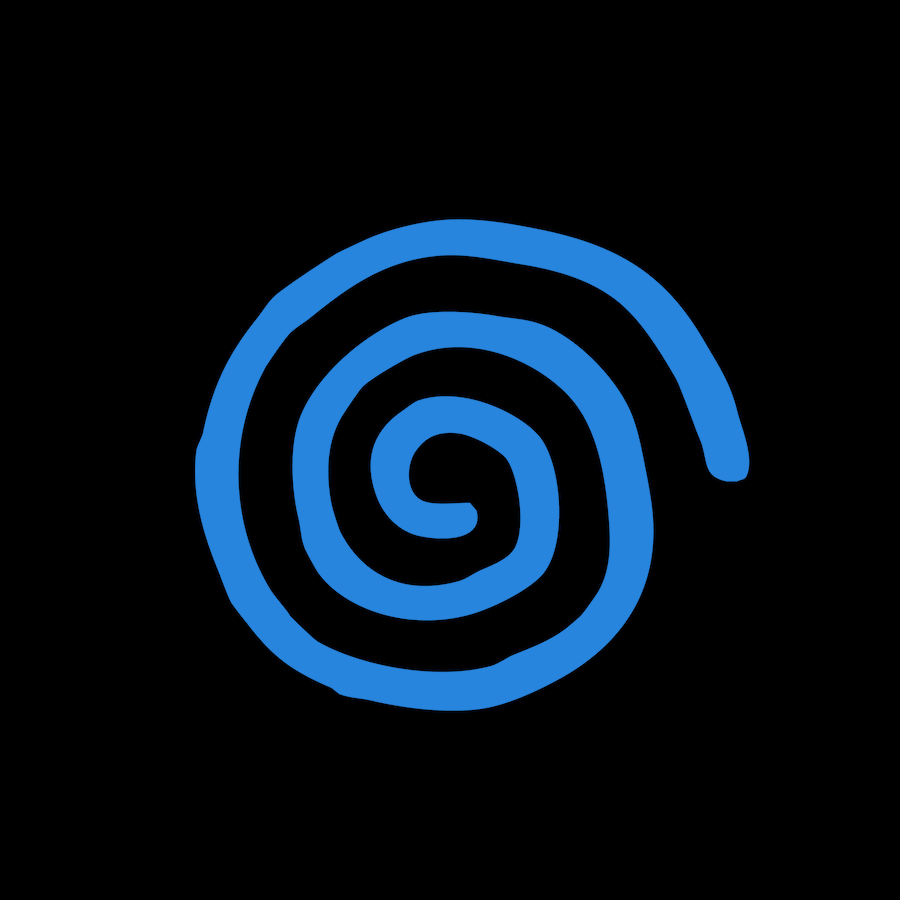
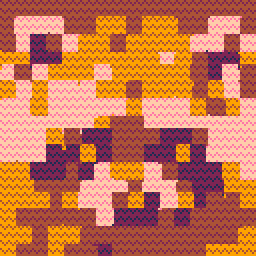
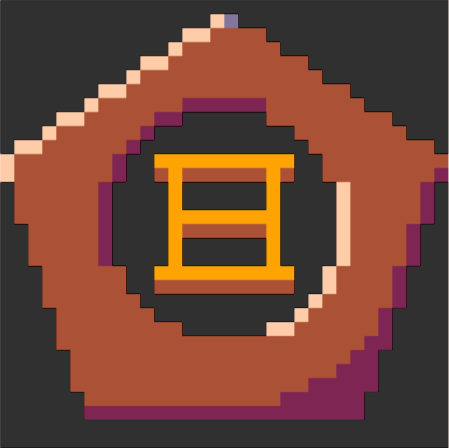
A quick gamepad tester I wrote for picotron to test the gamepad api. The tester also supports changing the player number to use multiple gamepads.
I labeled the buttons according to their corresponding keyboard inputs, and layed out the buttons according to how they mapped on my xbox controller.
The two buttons in the middle are buttons 6 and 7, which the readme lists as reserved, but I think represent start and select buttons.
Button 6 seems to map to the start and home buttons, as well as the enter key.
Button 7 doesn't seem to map to anything yet.
As of writing there seems to be a bug in picotron where higher player numbers don't work correctly.
player 0 works properly, but odd numbered players, such as player 1, will have their inputs split across two controllers.
I've also confirmed that as of writing picotron only fully supports up to 4 controllers. If you connect a 5th controller, half of their inputs will register on player 7, and player numbers higher than 7 aren't supported so only half the controller will work.
Update:
Now highlights the player tab when that player's controller has active inputs, making it easier to find what player your controller is mapped to.
Update 2:
Now reports analogue values for the sticks
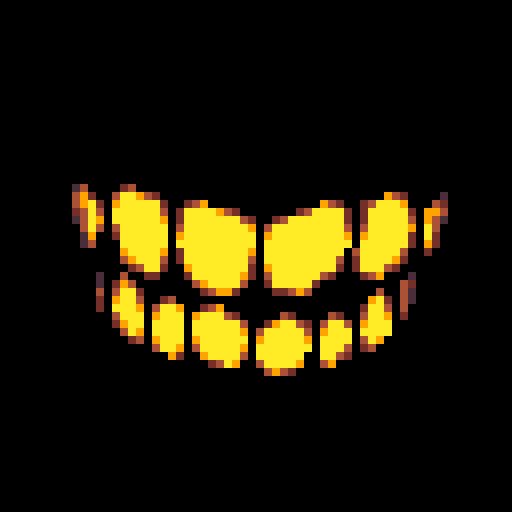
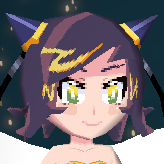
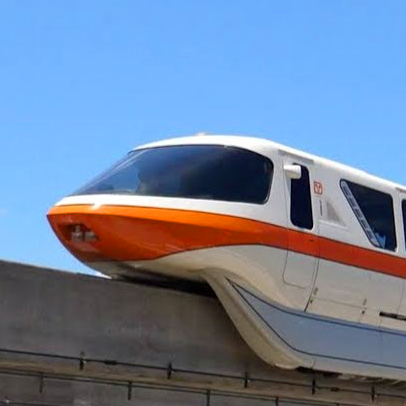
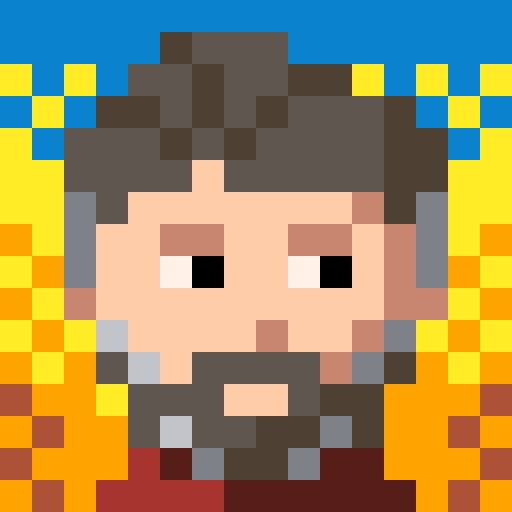

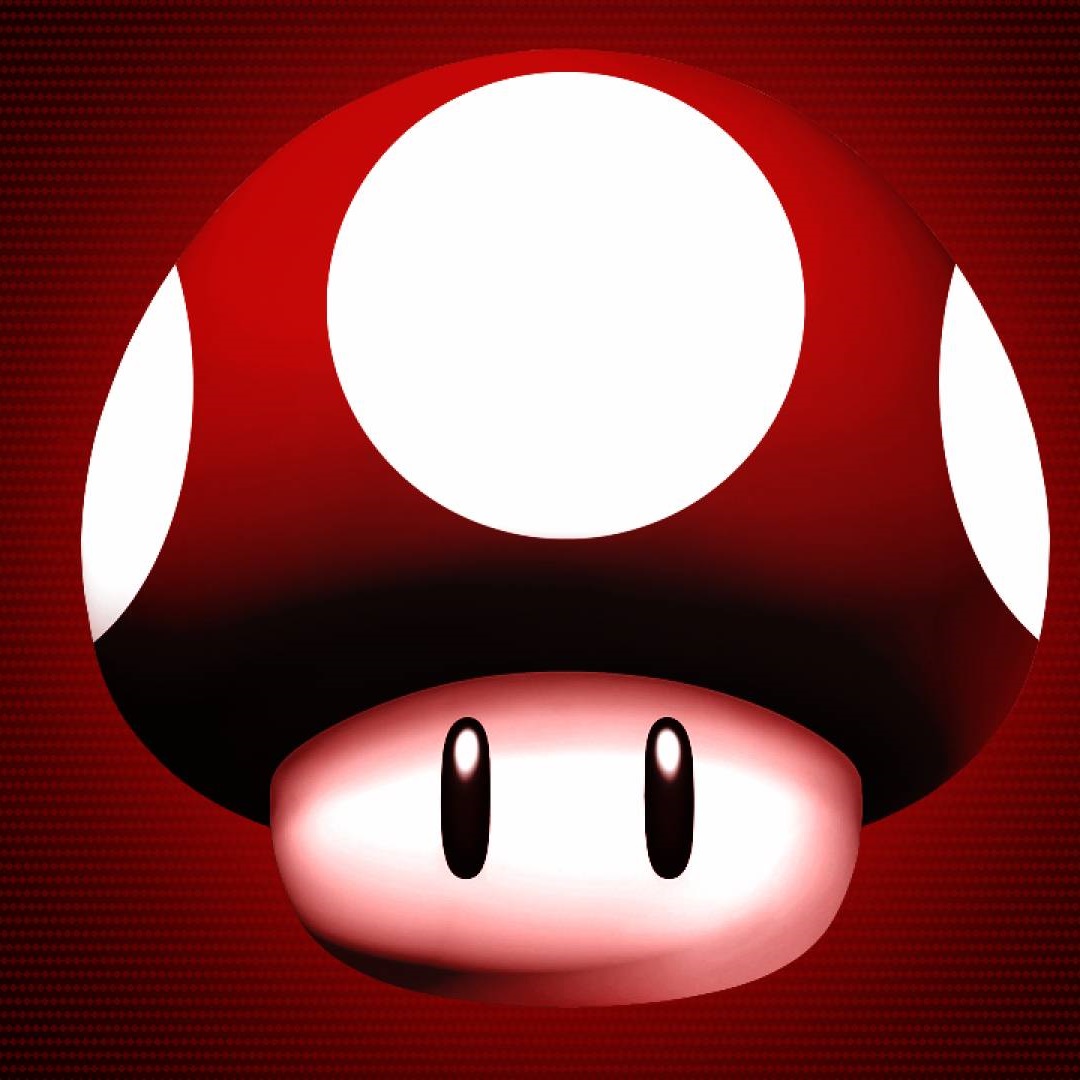
A Pico-8 port of astrosmash I made back in 2021.
For those interested, here's the source code: https://github.com/TheDuude420/AstroSmash-2021-Source-Code
I've been thinking about ways to execute fast pixel effects on Picotron, and so I'm looking for ways to perform the effect entirely in userdata-land, and display the results as sprites. I've tried a few approaches, none of which seems to be quite ideal.
-
Do math on
f64
userdata, then render that to the screen. This gets me fast math operations, but of course the rendering result is completely garbled. I suppose some pack-ints-into-floats type nonsense could be attempted? But with just basic arithmetic ops this seems like a bad time. -
Do math on
f64
userdata, then cast that tou8
in a loop. This is a lot of time spend calling getters/setters in a loop, and may not be any better thanpset
, not sure. - Do math on integer userdata, then cast to
u8
using theuserdata:convert()
method and display that. This would work fine if I could figure out how to get fast fixed-point going. Unfortunately, div and mod are slow, and shifts don't seem to work.
I think my preferred resolution would be to allow convert()
to go from f64
to u8
, but anything that allows any kind of math with fractional quantities, either floating-point or fixed-point, would be great. Drawing f64
userdata with spr
with implicit casting would be great too. Just trying to avoid explicit loops to get to u8
....


EDIT: The issue has been resolved! You can find your Lexaloffle key at the top of the Humble purchase page!
=================
Congrats on the release, @zep! Just purchased Picotron. I've been watching its development with an excited glimmer in my eyes over the years! It really looks like the perfect mix of specs (really excited about the wavetable sound in particular!) and I'm excited to start exploring my creativity with it.
I've been looking at how to add the product to my Lexaloffle account following the instructions on this page https://www.lexaloffle.com/account.php?page=activate, but have not had any luck with it. Is it correct that the Humble purchases page for Picotron simply does not have the 'Lexaloffle Account Activation' button yet?


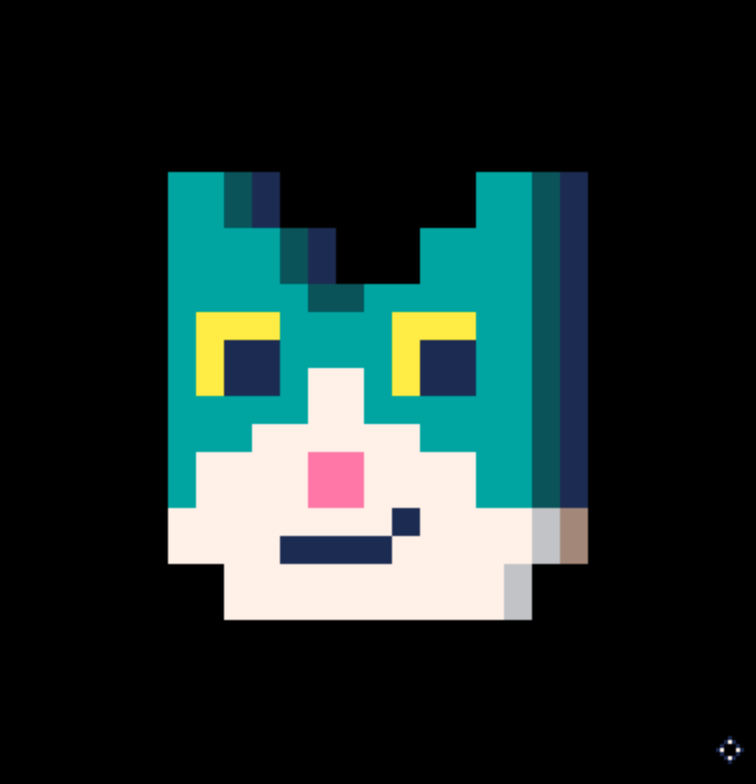
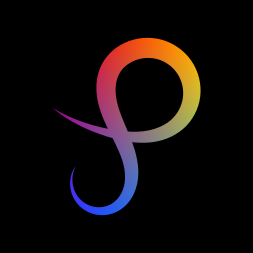

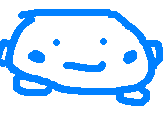



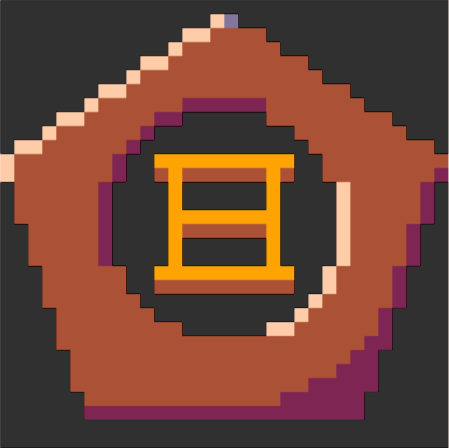
I'm trying to make a classic synth pluck sound: a couple of detuned saw oscillators, plus a resonant lowpass filter that quickly opens up when a note starts, then closes back down. Unfortunately, I can't quite figure it out. I have an ADSR envelope modulating the filter cutoff, but since the envelope has a positive polarity and higher lowpass knob values = lower frequencies, the effect is exactly the opposite of what I want: the filter immediately closes down on note onset, then more slowly opens back up.
Is there any way to flip the sign of this modulation? Am I thinking about this the wrong way?
