Hi,
I'm using a metatable for particles in my game. For example, when the player dies the sprite explodes in a burst of pixels. I use rnd() to off-set the player position with a random number, and as I generate a lot of different particles (based on the colors the character is composed of) I want to move the for-loop into the constructor.
Unfortunately, for some reason the randomness doesn't work when I build the for-loop inside the particle constructor. Compare these two examples:
For loop in caller function
particles={} particle = { x_offset=0, y_offset=0, } function particle:new(o) self.__index = self local pi = setmetatable(o or {}, self) pi.x=pi.x-rnd(pi.x_offset) pi.y=pi.y-rnd(pi.y_offset) add(particles,pi) end function game_over() for i=1,6 do particle:new({x=p.x,y=p.y,x_offset=12,y_offset=8}) end end |
Result: I end up with 6 particles, each with a different x and y value due to the rnd() function being passed the x_offset and y_offset values.
For loop in constructor
particles={} particle = { x_offset=0, y_offset=0, } function particle:new(count,o) self.__index = self for i=1,count do local pi = setmetatable(o or {}, self) pi.x=pi.x-rnd(pi.x_offset) pi.y=pi.y-rnd(pi.y_offset) add(particles,pi) end end function game_over() particle:new(6,{x=p.x,y=p.y,x_offset=12,y_offset=8}) end |
Result: I end up with 6 particles, but they all have the same x and y values. As if the rnd() is called only once and then applied to the other 5 iterations of the for-loop.
Questions
- Why does the code behave this way?
- What's the right pattern to apply here?

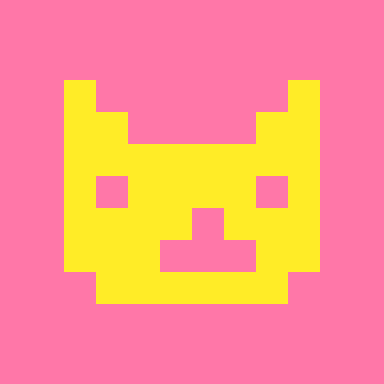
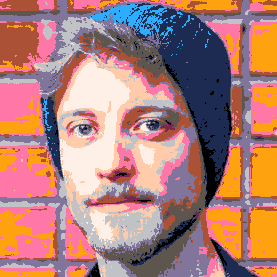
I can't seem to find any tutorials on how to make monsters move or patrol or have movements routines.
Nothing fancy, something like:
Go from A to B, wait a few frames, go to C, wait again, go back to A and repeat.
The monster doesn't even have to notice the player, just walk its path.
I've tried with loops but I can't make it work.
Thank you very much!!! :D
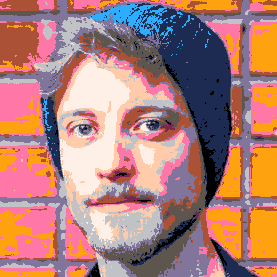
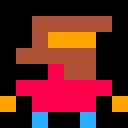
Just a small snippet from a token-saving discussion on the Discord last night.
If you need to iterate over neighboring tiles (for example when writing a path finding algorithm for 7DRL), this natural approach is pretty token heavy:
-- four directions, 29 tokens for direction in all{{-1,0},{0,-1},{1,0},{0,1}} do local x,y=direction[1],direction[2] end -- eight directions, 45 tokens for direction in all{{-1,0},{0,-1},{1,0},{0,1},{1,1},{-1,-1},{1,-1},{-1,1}} do local x,y=direction[1],direction[2] end -- eight directions, 43 tokens directions={0,-1,-1,0,1,0,0,1,1,-1,-1,1,1,1,-1,-1} for i=1,16,2 do local x,y=directions[i],directions[i+1] end -- eight directions, 30 tokens directions={-1,0,1} for x in all(directions) do for y in all(directions) do if x!=0 or y!=0 then -- end end end |
Why not use trigonometry?
-- four directions, 16 tokens for i=0,1,0.25 do [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=62065#p) |
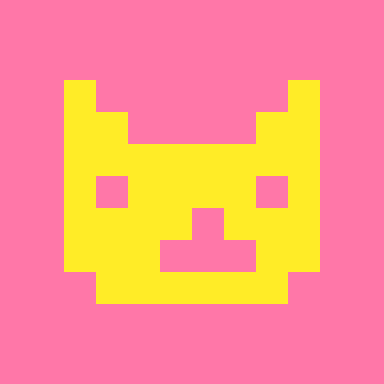
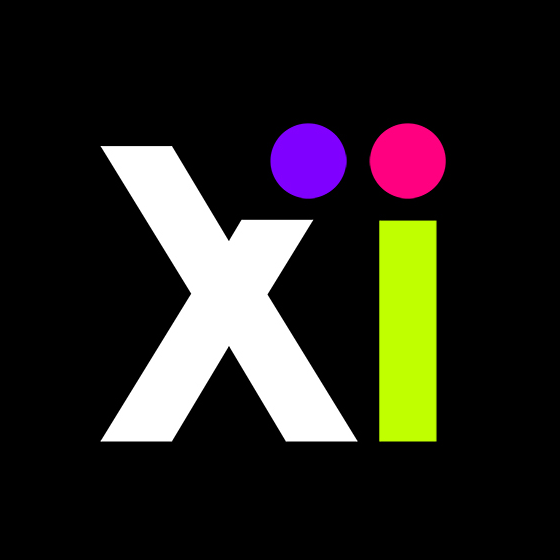

I decided to start a library of scripts to help those transitioning from visual scripting to lua.
You should be able to copy and paste code into your new script object put object in the room and run it with "Crt+R"
If something doesn't work please let me know.
Feel free to ask questions.
Have fun
/Digital Monkey
Lua scrip to print.
place scrip object in the room.
function draw() boxfill(0,0,63,128,128,63,3) set_draw_slice(120, true) print("printing", 48, 11, 7) print("in voxatron 3.5b", 40, 20, 7) end |
This is basic player controllers
control the box with arrows, z and x
x = 64 y = 64 updown = 30 function _update() if (btn(0)) then x=x-1 end if (btn(1)) then x=x+1 end if (btn(2)) then y=y-1 end if (btn(3)) then y=y+1 end if (btn(4)) then updown=updown-1 end if (btn(5)) then updown=updown+1 end end function _draw() clv() boxfill(x,y,updown,x+10,y+10,updown+10,14) boxfill(0,0,63,128,128,63,3) set_draw_slice(50) print("control the box", 33, 10, 7) print("using arrows, z and x keys", 10, 100, updown-1) end |
Scrip to change rooms made with "function draw()"
on J and K button press
(It's a flip book to go back and forth between rooms)
I made this by looking at script from GARDENING by: PROGRAM_IX



Remember SAM? (https://simulationcorner.net/index.php?page=sam).
Would it be possible in some way to use the white-noise for samples and/or digi-speech?
I'm not talking about a full-blown conversion of SAM (https://github.com/s-macke/SAM), but maybe a smaller version. Also - shouldn't it be possible to use at least 3-bit samples on the PICO-8? (https://gist.github.com/munshkr/30f35e39905e63876ff7)
I'm not the person to dive into this, but maybe someone else might be able to do some sort of conversion of the above. Personally, I would really enjoy being able to have short/small samples and make the Pico speak!
/ Pingo

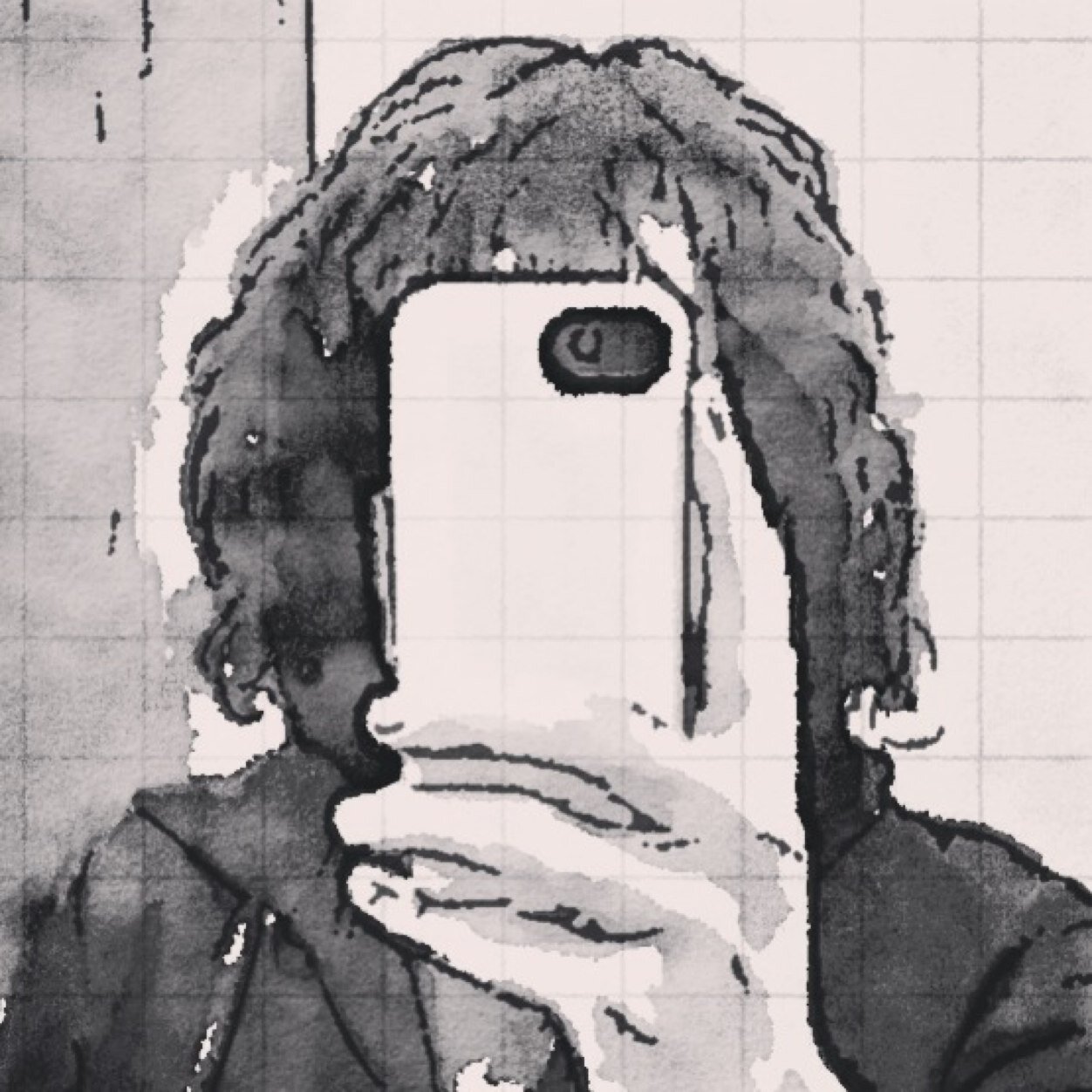



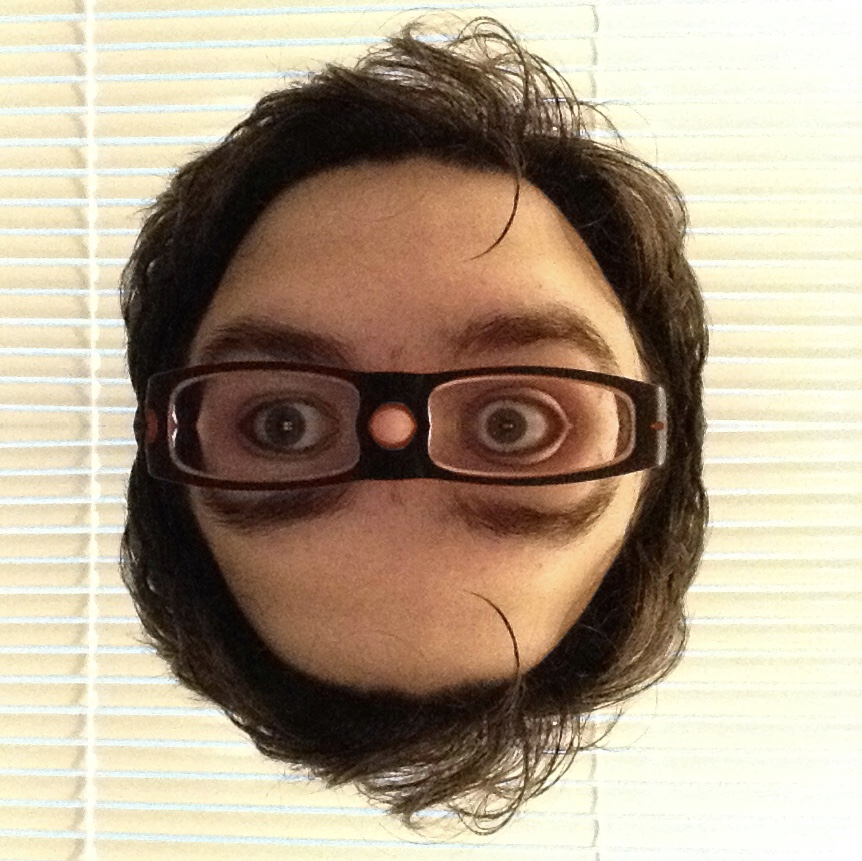



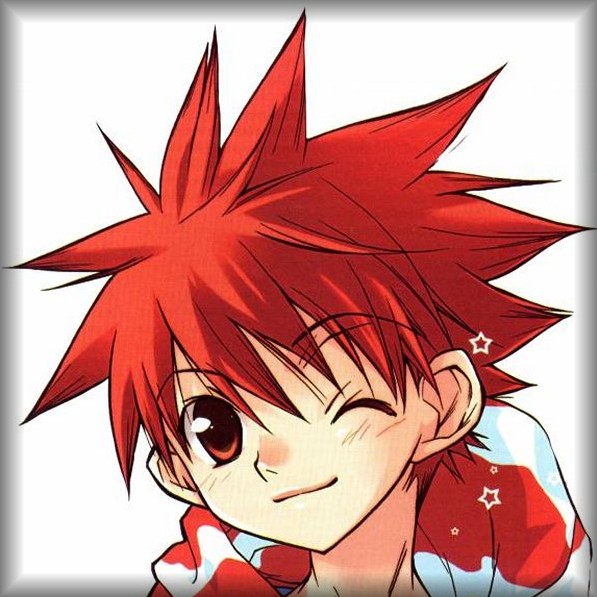
Previous version
About
Wal-Rush! is a game of walrii, fish, and flight. Based on a game I made for a programming competition at codewalr.us, this game lets you play as the mascot of the website, Walrii, as he flies through the sky. Collect fish, rack up points, and watch out for the spike mines!

Hello everyone, I'm Dead_Pixel.
I recently discovered Pico-8 and I'm really intrigued by the whole concept of it and look forward to getting to grips with it.
I'm really impressed by the quality of the cartridges and look forward to contributing some of my own work in the future too.
Before signing off I have one question - why was Lua chosen as the programming language?
Thanks.
A while ago I made a function for this
https://www.lexaloffle.com/bbs/?tid=30033
This was very clunky so I remade it. It used to have 124 tokens but I lowered it to 43.
You can use this function as much as you want without credit.
How to use:
printl(text,x,y,inner color,outer color,height)
text,
x,
y,
inner color --the color inside the border
outer color --the outline,
height --how far down you want your outline to reach. 0 means the outline has the same width in all directions.
--the x and y are the coordinates of the top right corner of the inside color.
Here it is! The game you weren't anticipating because it's my first post!
Cave Bat
Game Description
This is Cave Bat. You are a bat and you fly through a cave.
Controls:
- X -- Start or continue
- UP -- Fly
Objective
You gain points by flying. Coins aren't worth points, but you will get an extra life for every 10 coins you collect.
A game about rockets, for little and big kids. This is a one-button game with no possibility of failure.
Press X to skip the SPACE WALK.
See if you can activate the hidden autopilot for landing!
Change log:
0.2
- Sound, stars, random birds, sky colours, tracking camera
0.3
- Added a countdown! Player can tap UP or hold it.
0.4
- Used fill patterns to dither the gradations of atmosphere.
0.5
- Attract screen plays out an animation of astronaut getting on board.
- Added states to complete the game loop, you can get to orbit and back.
- Added SPACE WALK.
- Added rocket heating up depending on reentry velocity.
- Adjusted volume for less pew-pew and more engines.
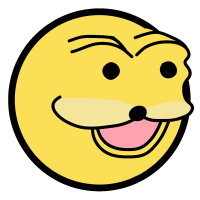
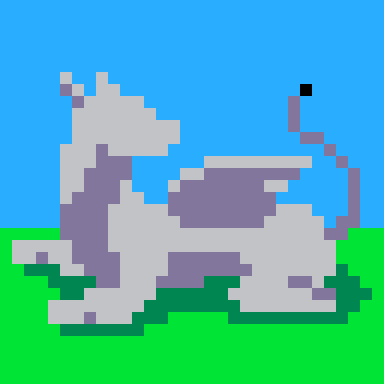
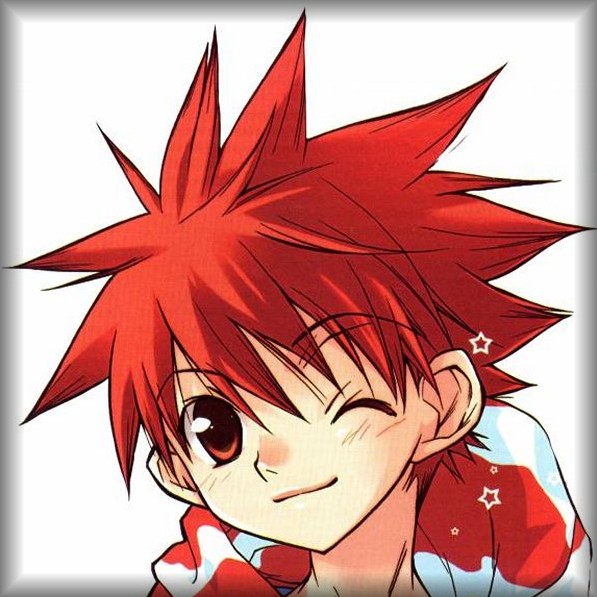

I see in the manual that Qubicle file format is supported for importing models, but I use MagicaVoxel on my computer and Particubes on my iPad, which use the same .vox format. (Spec: https://github.com/ephtracy/voxel-model/blob/master/MagicaVoxel-file-format-vox.txt)


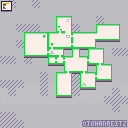
Inspired by an idea from JW of Vlambeer, I built this dungeon generator.
In short it adds a bunch of rooms on top of each other and then moves them randomly around until they don't fit any longer. Usually this makes for some very nice and organic looking scenes. This version also has a bunch of other parameters like wider door ways, interconnectedness, and decoration among others. The example in this thread randomises these parameters but in the code it is fairly easy to set them up to whatever.
I've tried to document it to a degree, so hopefully it can help out if someone wants to dive into my implementation. It should be said though that I primarily wanted to visualise the process and to look nice, not necessarily be the most efficient data structure. ;)



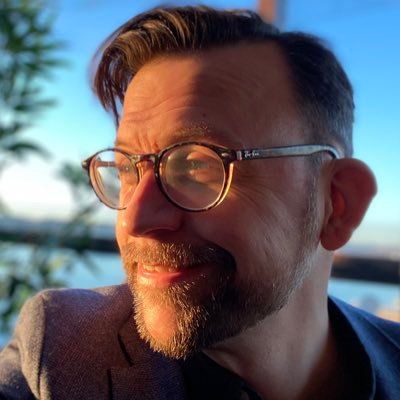
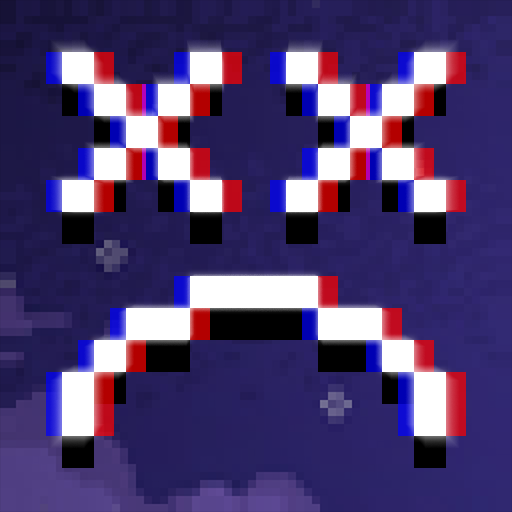
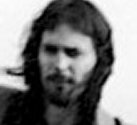

A starfield effect, inspired by the old Windows screensaver, though the style is more in line with Star Trek. Use up and down to increase and decrease speed, and press X to show stats.
I used nucleartide's Pico-8 snippets, specifically vec3 and pline(), to do the 3D projection. I was encouraged by reinvdwoerd's Perspective Lines and used his cartridge to figure out how to use pline().
To really get immersed, shout "increase speed to [warp number]!" as you hold the up key or "all stop!" as you slow down to zero.


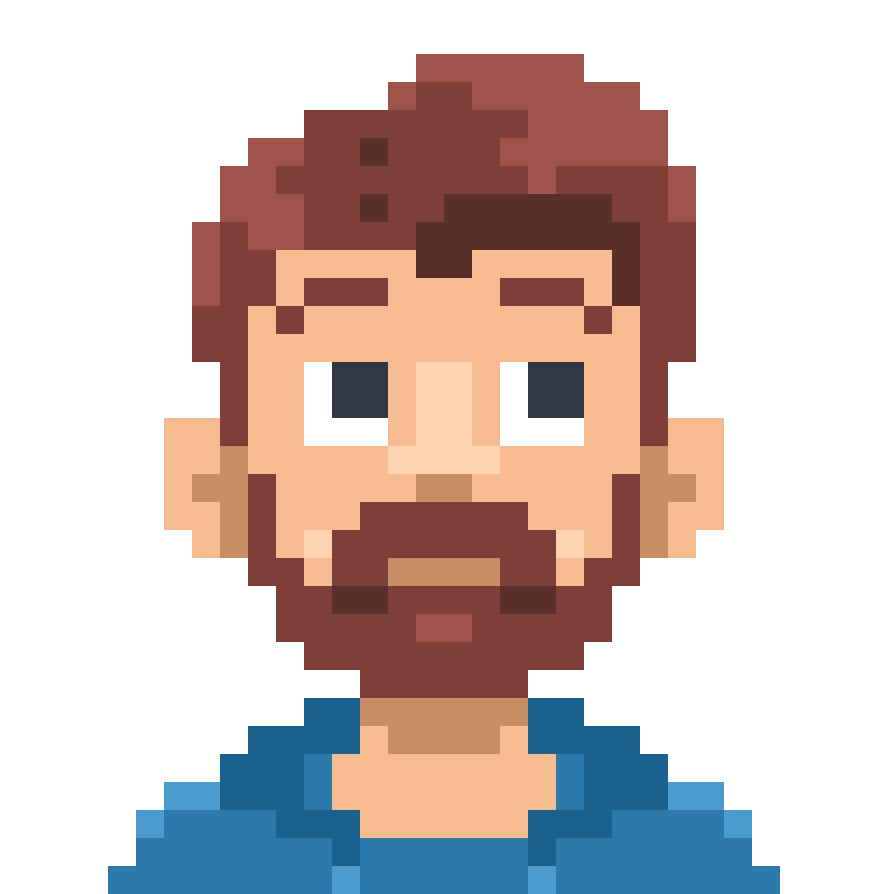
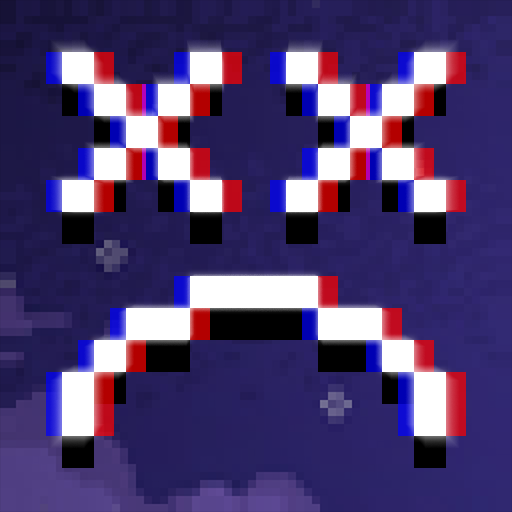
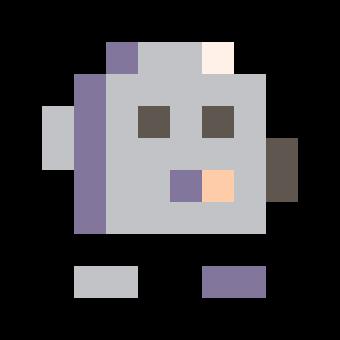
Dodge is a fast paced reflex game. There is no end, you just have to survive the longest possible. Enemies move towards you and it's game over if they touch you, but they explode and give you a point if they touch each other.
There is a powerup (the ball with fire particles) that you can touch to destroy all enemies that are currently on the screen.
This game is still WIP and will be updated regularly. There will be music, better sound effects, updated graphics, and maybe even some more gameplay elements depending how far we want to go with it.

This is a short puzzle game based off of another puzzle game I saw, SPAB. The player's inputs affect the world as well as the character. I came up with some messy ways to make it work and would redo a lot of it if I made a more complete version.
If I do expand on this idea I see two ways to with it. Either a sort of action-puzzle game with fighting enemies and smoother movement, or a more complex puzzle game with much more responding environmental parts and maybe more rigid movement.


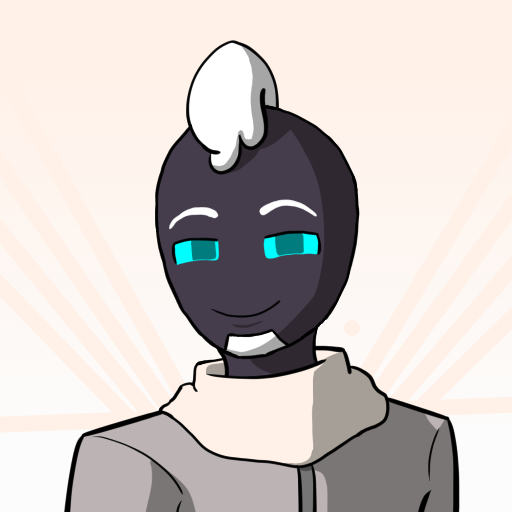