
I have a tile object which contains the sprite number and other properties. I want to update different properties at different times, instead of passing all properties all the time.
First I tried this approach:
function update_tile(values) tile = values end |
but of course that unsets anything that you don't pass in values
Then I settled on the following approach:
function update_tile(values) for k,v in pairs(values) do tile[k] = v end end |
Am I correct that this is the right way to do it, or is there a better (code readability or less tokens) way to do this?


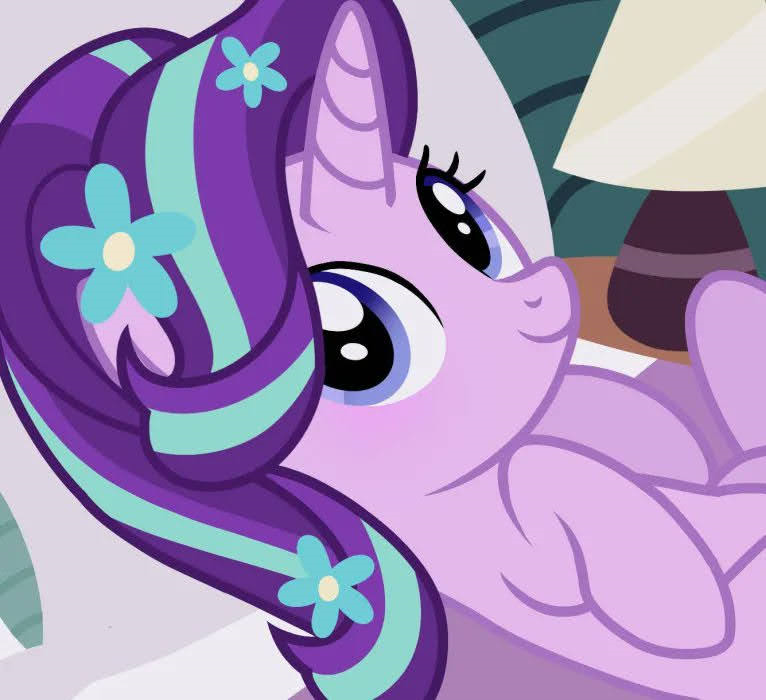
Hi,
I'm iterating over all map cells multiple times, to do various actions:
for t_x = 0, 127 do for t_y = 0, 63 do -- scan tiles end end for t_x = 0, 127 do for t_y = 0, 63 do -- do something end end for t_x = 0, 127 do for t_y = 0, 63 do -- place tiles end end |
This works, but uses a lot of tokens (do something is actually 4-5 different actions).
As there are a lot of interdependencies between cells, I can not do this:
for t_x = 0, 127 do for t_y = 0, 63 do -- scan tiles -- do something -- place tiles end end |
Instead, I wanted to do this:
functions = { scan_tiles(t_x, t_y), do_something(t_x, t_y), place_tiles(t_x, t_y), } for f in all (functions) do for t_x = 0, 127 do for t_y = 0, 63 do f(t_x, t_y) end end end |
But I never get the arguments passed to the functions I call.
How to go about this?
All help very much appreciated!


Hi,
I'm using a metatable for particles in my game. For example, when the player dies the sprite explodes in a burst of pixels. I use rnd() to off-set the player position with a random number, and as I generate a lot of different particles (based on the colors the character is composed of) I want to move the for-loop into the constructor.
Unfortunately, for some reason the randomness doesn't work when I build the for-loop inside the particle constructor. Compare these two examples:
For loop in caller function
particles={} particle = { x_offset=0, y_offset=0, } function particle:new(o) self.__index = self local pi = setmetatable(o or {}, self) pi.x=pi.x-rnd(pi.x_offset) pi.y=pi.y-rnd(pi.y_offset) add(particles,pi) end function game_over() for i=1,6 do particle:new({x=p.x,y=p.y,x_offset=12,y_offset=8}) end end |
Result: I end up with 6 particles, each with a different x and y value due to the rnd() function being passed the x_offset and y_offset values.
For loop in constructor
particles={} particle = { x_offset=0, y_offset=0, } function particle:new(count,o) self.__index = self for i=1,count do local pi = setmetatable(o or {}, self) pi.x=pi.x-rnd(pi.x_offset) pi.y=pi.y-rnd(pi.y_offset) add(particles,pi) end end function game_over() particle:new(6,{x=p.x,y=p.y,x_offset=12,y_offset=8}) end |
Result: I end up with 6 particles, but they all have the same x and y values. As if the rnd() is called only once and then applied to the other 5 iterations of the for-loop.
Questions
- Why does the code behave this way?
- What's the right pattern to apply here?

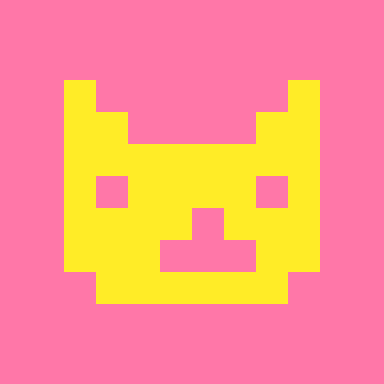
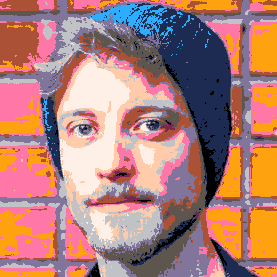
Introduction
I'm trying to implement my "map to table" conversion as proposed in my previous forum post. To this end I created two functions: one for iterating over the map and storing it in a multi-dimensional table (generate_world()) and one for iterating over that table and placing the sprites (build_world()).
Problem
I get it to nicely iterate through the map, print out the tiles it finds and (presumably) store it in a table. However, when iterating over the table things get awry. I do get it to iterate over the first dimension (when printing it returns [table]), but when iterating over the second dimension it's empty (doesn't print anything).
All help would be greatly appreciated!
Code
function generate_world() world={} t_x=0 t_y=0 max_x=48 max_y=16 print "generating world ..." for x=0,max_x do world[x]={} for y=0,max_y do [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=60896#p) |

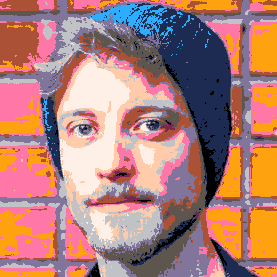
Hey all,
Introduction
I haven't done any development for many years and in general hardly did any game development. Now I'm getting back into it with the awesome Pico-8 and to get familiar with the engine and game dev I'm working on a platformer.
Current progress
The current status is that I have a player character, physics, a (very) basic map and some sound effects. It all works nicely and I'm currently working on items that the player can pick up (power-ups and such). Before moving forward I was hoping to learn some best practices from you all and get some feedback on my current approach.
Approach or implementing items
As I want to have rather large maps with many items I want to place items using the map editor, not by defining them manually in the code (as tweaking during play-testing would be a pita). At the same time I want the items to have some animation before they're picked up and of course an animation when they're picked up as well.
My current idea is to scan the map during _init() using mget() for these items and then add them to an array which would be scanned and updated during the game loop. The idea is to iterate over the elements in the array and use mset() to change the tiles to do the animation before they're picked up.
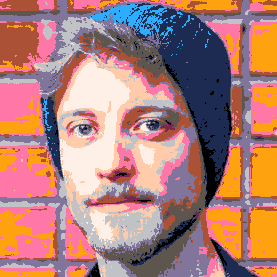

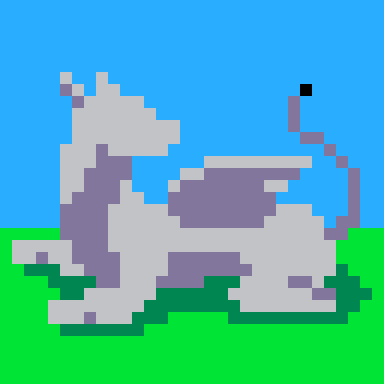