Pico-8 doesn't provide a built-in way to sort arrays.
However it does provide a way to remove items from an array as well as a way to add them to a random position.
A lot of the time a full array-sorting function isn't needed; sometimes it's just enough to be able to insert one item into an already sorted array.
I did look around in the bbs but I didn't find a function for doing it, so here it is:
do local lt=function(a,b) return a<b end function oadd(arr,obj,cmp) cmp=cmp or lt local l,r,m=1,#arr while l<=r do m=(l+r)\2 if cmp(arr[m],obj) then l=m+1 else r=m-1 end end add(arr,obj,l) return l end end |
The function figures out where to insert the item in an already sorted array (using the same algorithm as quicksort) and inserts the new item there.
For arrays of integers or strings it is a drop-in replacement for the built-in add
function. The numbers will just be inserted in the right place so that they are sorted. The third optional parameter allows for using different comparation functions. Example:
Recently I was working on a project with a friend and I closed Picotron, thinking it would reopen where we left off. It did not.
We searched through all sorts of Picotron folders to try and find a RAM backup but turned up nothing.
I realise that stuff in RAM isn't saved on a real machine and Picotron might be trying to emulate the experience of working with an older machine, but from a usability point of view, it's suboptimal and disappointing when you lose stuff.
I'd like to suggest either:
- Prompt the user to save when the window manager close request is received
-or- - Upon opening, return to the same state the VM was left in
Perhaps a toggle in the settings to switch between both?
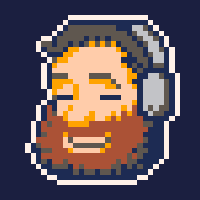

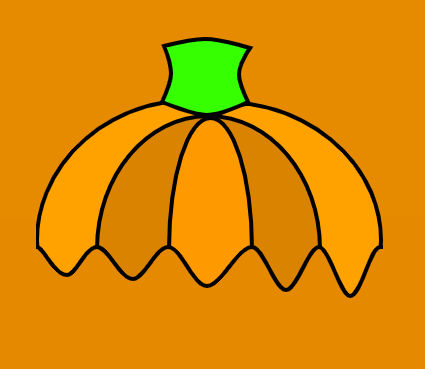
I was using a 2d array with a 'cell' object for each grid and found this weird issue. For some reason i can't change a value in a particular cell on a grid without changing it for the whole grid. Hope i explained it clear enough. Here's an example:
cls() cell={1,1} arr={{cell,cell},{cell,cell}} arr[1][1][1]=2 for x=1,2 do for y=1,2 do ?arr[x][y][1],x*8,y*8,15 end end |
As you can see i'm changing only the first number in the first column and the first row to 2 but the output shows that every first number in the whole grid was changed into 2. Why does that happen??
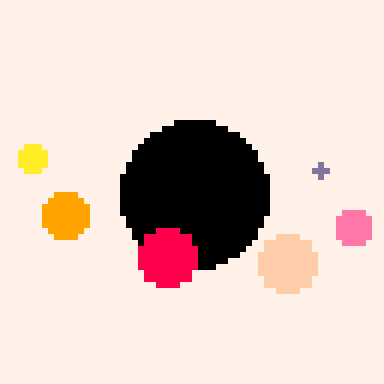
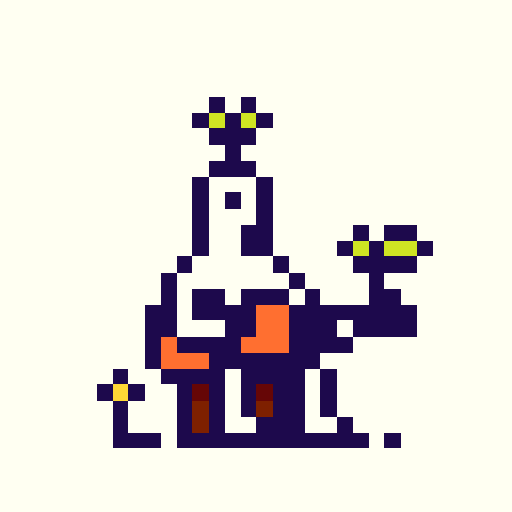
Evil has returned, and You have been called upon to vanquish it! (again) It's all up to You, Hero! (again)
⬆️➡️⬇️⬅️ - Movement
🅾️ - Sword attack
❎ - Arrow attack
🅾️ + ❎ - Staff attack
Enter/Start - Show inventory
Features:
- Hack n slash in an ever-increasingly evil world
- No story to complete, just play until you're sick of it!
- Two characters to switch between
- Iconography for everything
Gotchas:
- Bug: sometimes the inventory doesn't show up in the pause menu
Changelog




.png)
So you know those CMY Cubes? The ones where you rotate them around and the colors mix in the light?
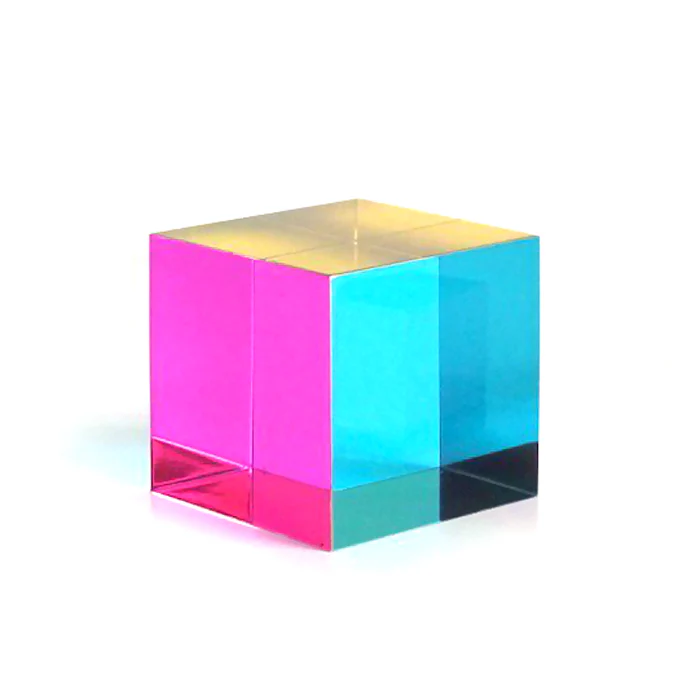
After recently being taught how to mix colors using bitplanes, this idea came to mind, and I just had to make it
The 5 Platonic Solids, in all their CMY glory!
This was actually quite the challenge for me, as I've never messed with 3D rendering before. So I had to learn how to find the vertexes of each polyhedra, and I had to learn how to rotate them using matrix multiplication. But that was all quite fun, and I hope I can use them again in a future project that isn't just basically a gif
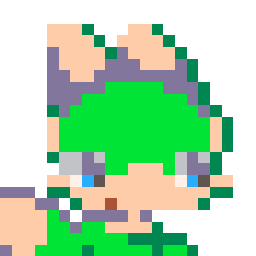

Hello there
I am really new to Pico8, not a dev and English is not my first language but I'll try my best!
When launching a cart from terminal with "pico8 -run cart.p8" and adding it to favorites using pico8 menu, pico8 closes itself and an error pops in the terminal.
Here is it :
./pico8 -run '/$HOME/.lexaloffle/pico-8/carts/cart.p8.png' Segmentation fault (core dumped)
Pico8 is from itch.io and version is : PICO-8 v0.2.6b
OS is : Linux Mint 22.1
Feel free to ask for more infos if needed :)

Seizure warning: Lots of flashing colors as the bogo sorter sorts quickly!
Watch as this bogo sorter (visualized by colored bars) attempts to sort a list. Once it finishes, it will begin trying to sort an even bigger list! Watch as it tries to go as far as it can with an ever-expanding list!
This is my first cart, made in order to mess around with PICO-8. As you may be able to tell, it is a joke.
The hard limit for how many elements that can be visualized is probably 128, but I haven't tested. Not that it will matter, as the chances for it ever reaching that point are astronomical anyway.

A small picross game!
Controls:
Fill block - Left mouse
X block - Left mouse + X
Erase block - Left mouse + Z
Move your mouse to the left/above the board to see more of the row/column clues.
When making the puzzles, I was more focused on the end result than on how easy it is to solve so the difficulty ramps up fairly quickly. That said, all puzzles should have only a single solution and do not require any guessing.
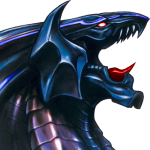


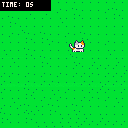
Cat Idle 1
In this really dumb game you just sit and stare at a cat. Sadly I have to say, that this really terrible game is my first game. There is actually no point of playing this game.
Reasons to not play this game:
-
You cant do ANYTHING.
- Sometimes the cat likes to leave the map and never come back.
The 1 fun thing about it:
- You can show your friends how brainrotted you are with the counter!
By Skyme
![]() |
[16x16] |

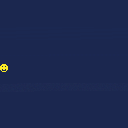
Hi!
Just sharing my first Pico8 game. It's a Tetris inspired by Twintris on Amiga, and it's all about smooth movements. It doesn't follow all the original Tetris rules, since there are already a lot of clones out there doing a good job.
Controls:
- ⬅️,⬇️,➡️ move the piece
- ❎,🅾️ rotate the piece
- ⬆️ hard drops the piece
Versions
1.1
- Added a menu setting for showing/hiding the bg grid
1.0
- Added "ghost piece"
- Added SFX
- Fixed some bugs on levels progression
- Sped up pieces movement
- More time to make the piece slide
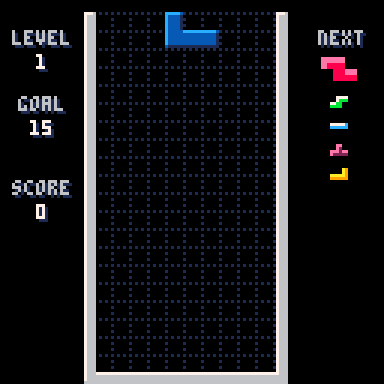
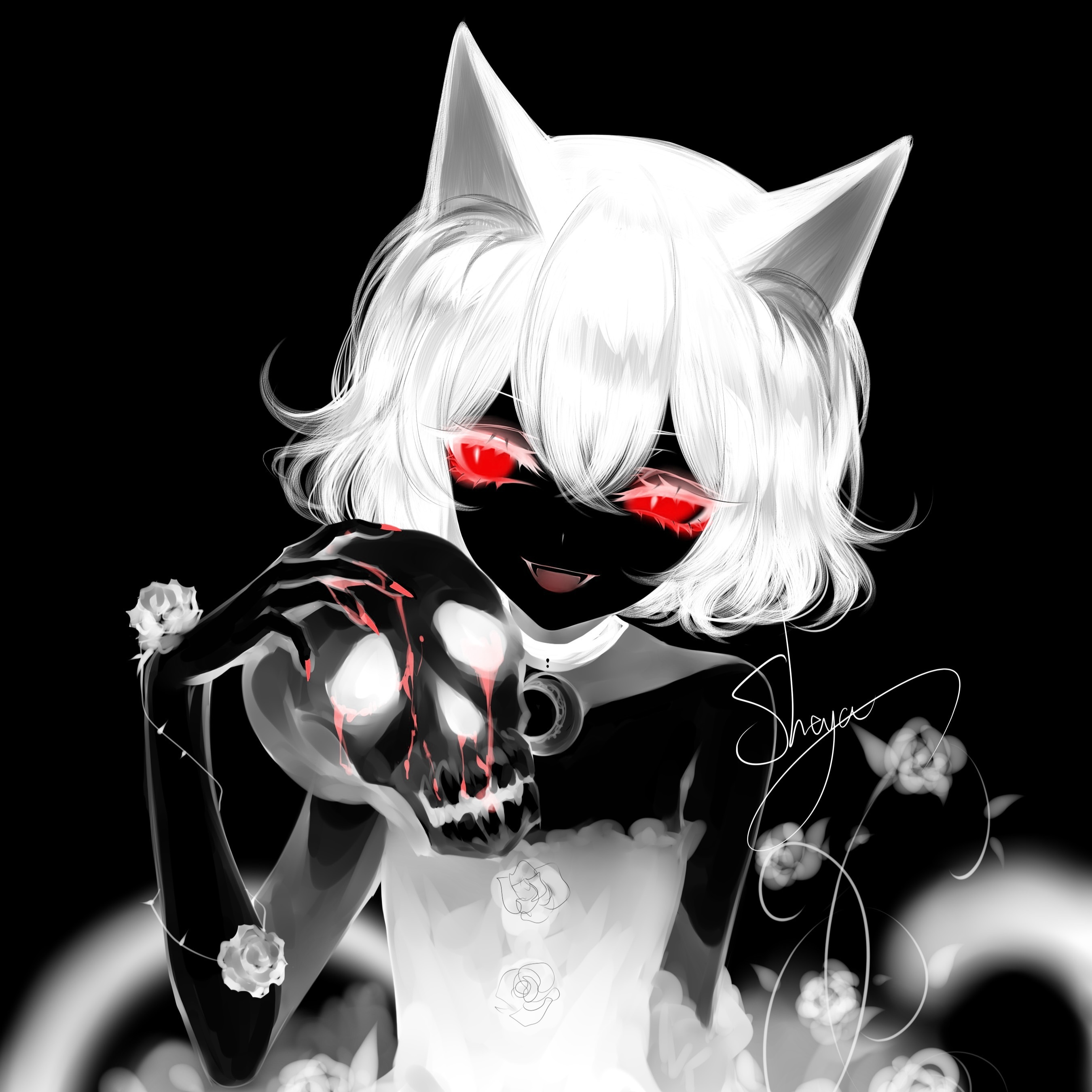


Oh boy it's time to remake my horribly named mods. Back when I was a little bitty boy I made my very first mod, called "funny mountain game", although some of you might call it with a, different word... And now, 7-ish months later, I now made this, a remake (more of a reboot, but you know what I mean.) of the original mod. So uh, have fun I guess.
This Celeste mod is significantly harder than the vanilla game. I'd suggest you play the original first before playing this mod. There are 3 routes, with all of the berries being possible. I'd ask you if you can but lets face it, you're probably gonna try to get all berries anyway.
Controls
- Arrows to walk
- Z to Jump




Hey everyone,
we're finally cleaning up the sources of our demo The Mind from last year's Revision party.
Each effect will be available as a standalone file with a bit of comments added.
If you want to know more, please let me know.
This effect is a classic ray-casting tunnel. It's doing a ray-cylinder intersection test along a rough grid and interpolates the areas in between. By replacing the intersection test, you can render all kinds of continous shapes.
Since the uvs of the cylinder wrap around at some point, a bit of thought went into solving this in a reasonably performant way.
Source code

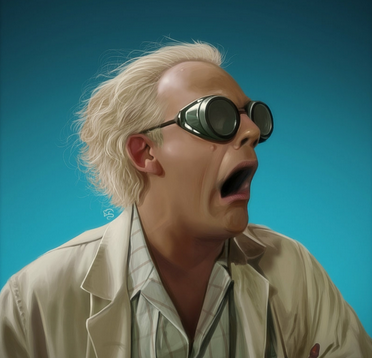
Caveshum
escape the endless dungeon filled with monster and treasure or will you?
How-To-Play:
Kill Monsters to get upgrades and money.
Once you see the 6 rooms, something will happen...
Controls:
- Move: arrow keys
- Jump: X or V
- Whip/????/Gun C or Y
About:
I watched the first Indian Jones recently, that should explain most.
Also you may find alot of similarities with my other game i just published "downmole". Thats because downmole wasdeveloped it for quite long and I kinda made a mini engine. then this game is basically just the same but using other tiles and so on.
This is a update to the original microjam 34 submission, which you can play on itch. But this one is better.
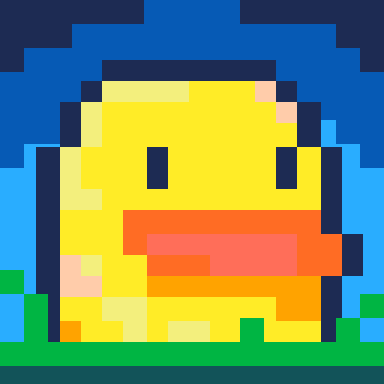
