Here's a function that will transform whatever you pass it into a table that will hopefully be understandable by a human. It'll take any table or other Lua value, so you can, for example, print it:
print(ins({1,2,3,foo=bar}),1,1) printh(ins({1,2,3,foo=bar})) |
The only parameter besides the string to be transformed is the depth.
local deep={l1={l2={l3={l4="!"}}}}} print(ins(deep)) --prints everything print(ins(deep,2)) --stops at l2 and prints {_} |
As the author of the inspect.lua library, I use it a lot on my day job when I need to debug Lua, and I really miss it when debugging in Pico-8. I found some previous efforts on this direction, but I was missing a lot:
- Marking repeated tables, functions, threads and userdata with numbers
- Handling nested tables / avoiding printing repeated tables
- Sorting keys sensibly
- Printing metatable data
- Presenting arrays as comma-separated single lines, and hashes in multiple-span lines
I made ins' output take less horizontal space than inspect.lua, so it adapts better to PICO-8's output. And I trimmed features(string escaping, preprocessing).
Still, at ~700 tokens, it's ... a chunky boy. You probably shouldn't add it to your project permanently. Think of it as a "debug artillery" function: deploy it to a tab in your game, it should be able to handle whatever you throw at it and return a digestible string. Once you have used it to destroy your problem, remove it.
PS: My biggest surprise was that PICO-8 has no built-in sorting function. So I bundled one. Oh and there is no concat, either. The garbage collector must be having fun with all those strings :)
EDIT: fixed bug in isidentifier function
This is an implementation of the function described on this short paper:
https://arxiv.org/abs/2010.09714 (twitter thread)
It is amenable to tweeing/easing in games. I have programmed easing functions in the past and one of the problems about them is that you need to code "families of easing functions":
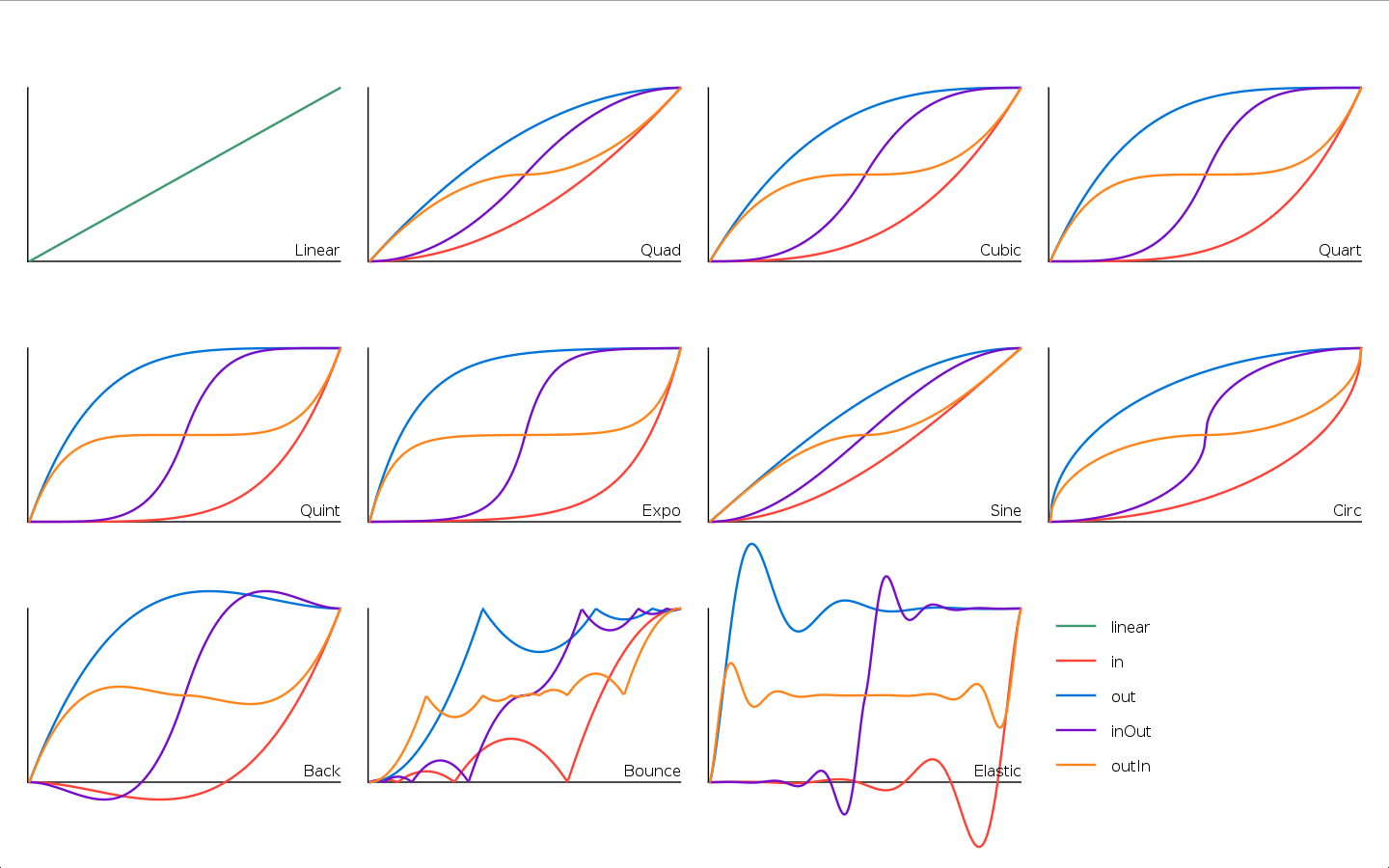
(That's from tween.lua, if you are curious).
I like this function because it can aproximate all of the "smooth families of functions" on that graph (all except the "back", "bounce" and "elastic" families) with a single function, plus it adds an infinitude of variants. Not bad for ~66 tokens.
Usage:
y = easing(x,t,c) |
All params (x, t and c) are restricted to the [0,1] interval. When they are, the result (y) is also in the [0,1] interval.
- x is the input being eased. In games and animations this usually encodes "the percentage of time that has passed since the start of the animation". Notice that x is restricted to [0,1]. If the magnitude you are trying to ease is not on that range, you might have to scale it (like I did in order to display the graphs).
- t is "the threshold point" - that's the location of the "inflection" in the curve (the point at which the curve switches from out to in, or viceversa).
- c is "the curvature". At 0-0.5 the curve will "start in in", gradually approaching linear interpolation at 0.5, and then the curve will "start in out" the more it approaches 1 from there.
- y is the return of the easing. In games and animations this is often the "percentage of space the thing moves, for the time that has passed". Like with x, it will be on the [0,1] range, so it might need scaling.
Usually x changes over time, while t and c are "fixed" during an animation. On the attached Cartridge they are animated, but only because we show the whole curve.
There are a couple differences between my implementation and the one in the paper:
- I had to add 1 to the x>=t branch of the function. I believe this is because Pico-8's y-axis goes "down" instead of up, like in "regular math". Not 100% sure about this.
- The s parameter described in the paper is a "slope". When it goes from 0 to 1 it acts "one way", making the curve more "steep" at the beginning and when it goes from 1 to infinity it acts "the other way", making it more steep at the end. I replaced s with c (c = 1/(s+1)), so that it stays on the [0,1] range, like the other params.
This is a simple game I made with my (now 4-year-old) son. Perhaps influenced by Lexaloffle's BBS icon, its protagonist is a giraffe. It eats falling leaves.
It has no victory or defeat conditions (my son still doesn't demand them, so I keep not including those).
I wanted my son to start getting used to a mouse, so this game has mouse support! It was fun having to activate it via POKEs. (I was reminded of the Sinclair Spectrum 48k of my youth). The game also works with the default "button-based" control scheme.
- Arrows: Move head
- X: Eat
The code, as usual, has been heavily cleaned up before posting.
Notes about the graphical design:
- I only programmed the giraffe head and the leaves with my son. The telescopic neck and body at the bottom was added as a surprise, later on.
- On that note, I am very aware that giraffe's mouths are on their snouts, not on their necks. If you feel the need to comment about this particular anatomical anomaly, let me point out that this particular giraffe has a telescopic neck and its legs don't move while it walks.
- The pinkish falling ... "things" are supposed to be leaves. I think they look more like beets, but my 4yo Chief of Design was very adamant about the colors and shape.
This is the second game my 3-years-old son and I did together.
It started as something simple. A car which moves around. A police car? No, a regular car.
Then we added coins. And made the car pick them! Interactivity! Randomness!
Then we needed a monster. So of course Covid-19 makes an appearance as the bad guy. You "kill it" with the car (which conveniently uses the same code as the coins).
Although to more experienced players the game might feel like it needs some kind of "success screen", my son doesn't seem to miss it at all.
It was also the first time we used the sound editor. Bruno is way too impatient, and I am too inexperienced, to create a whole song for a game just yet.
Fun facts:
- There's exactly 10 instances of the virus because that's the biggest number Bruno is able to count up to so far (reliably).
- There's exactly 51 coins because "51" is the number Bruno uses when he wants "a very big number".
Controls: arrows to move.
I was not yet familiar with pico-8's add, del, and foreach so the first version of this had a lot of duplicated code and it was a bit of a mess (try coding next to a 3-year old child to see what I mean!). I cleaned it up before posting.
Today was the first day I did a videogame with my son.
He's 3 years old. He helped me pick all the colors and with design decision, like what kind of dinosaur to include and what he would be doing.
The game itself is literally 6 if blocks, and I wrote it pretty quickly, starting from api.pb . It still felt like an eternity to my toddler! He could see progress while I was drawing the dino and the flowers, but the programming part was meaningless to him. He was vociferously demanding progress. A true client. I had to cut many features. Bounds checking, for example, only works partially. It became a feature ("ooooh where did the dinosaur go?")
CONTROLS: Move with arrows, water flowers with Z.
Cleaned up before publishing.