Like the title says, I have rudimentary collision detection on the top and sides of my sprite but not on the bottom.
I'm very new to game dev, but I have some experience in python. I can't see if I have a typo, or I've made a mistake in following the tutorial I watched.
--player--
function create_player()
player={
state="normal",
sprite=1,
health=4,
x=64,
y=82,
h=8,
w=8,
gravity=0.30,
friction=0.15,
inertia=0,
thrust=0.80
}
end
function collide(o)
local x1=o.x/8
local y1=o.y/8
local x2=(o.x+7)/8
local y2=(o.y+7)/8
local a=fget(mget(x1,y1),0)
local b=fget(mget(x1,y2),0)
local c=fget(mget(x2,y2),0)
local d=fget(mget(x2,y1),0)
if a or b or c or d then
return true
else
return false
end
end
function move_player(o)
o.y+=o.gravity --applies player gravity
local lx=o.x --last x pos
local ly=o.y --last y pos
if (btn(❎)) o.y-=o.thrust --player move
if (btn(⬅️)) o.x-=0.5
if (btn(➡️)) o.x+=0.5
--if the player collides, moves back
if collide(o) then
o.x=lx
o.y=ly
end
end
function ani_player(o)
if btn(⬅️) then --player animation
o.sprite=2
elseif btn(➡️) then
o.sprite=3
else
o.sprite=1
end
end
function draw_sprite(o)
spr(o.sprite,o.x,o.y)
end
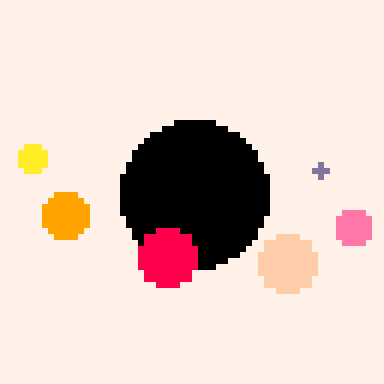
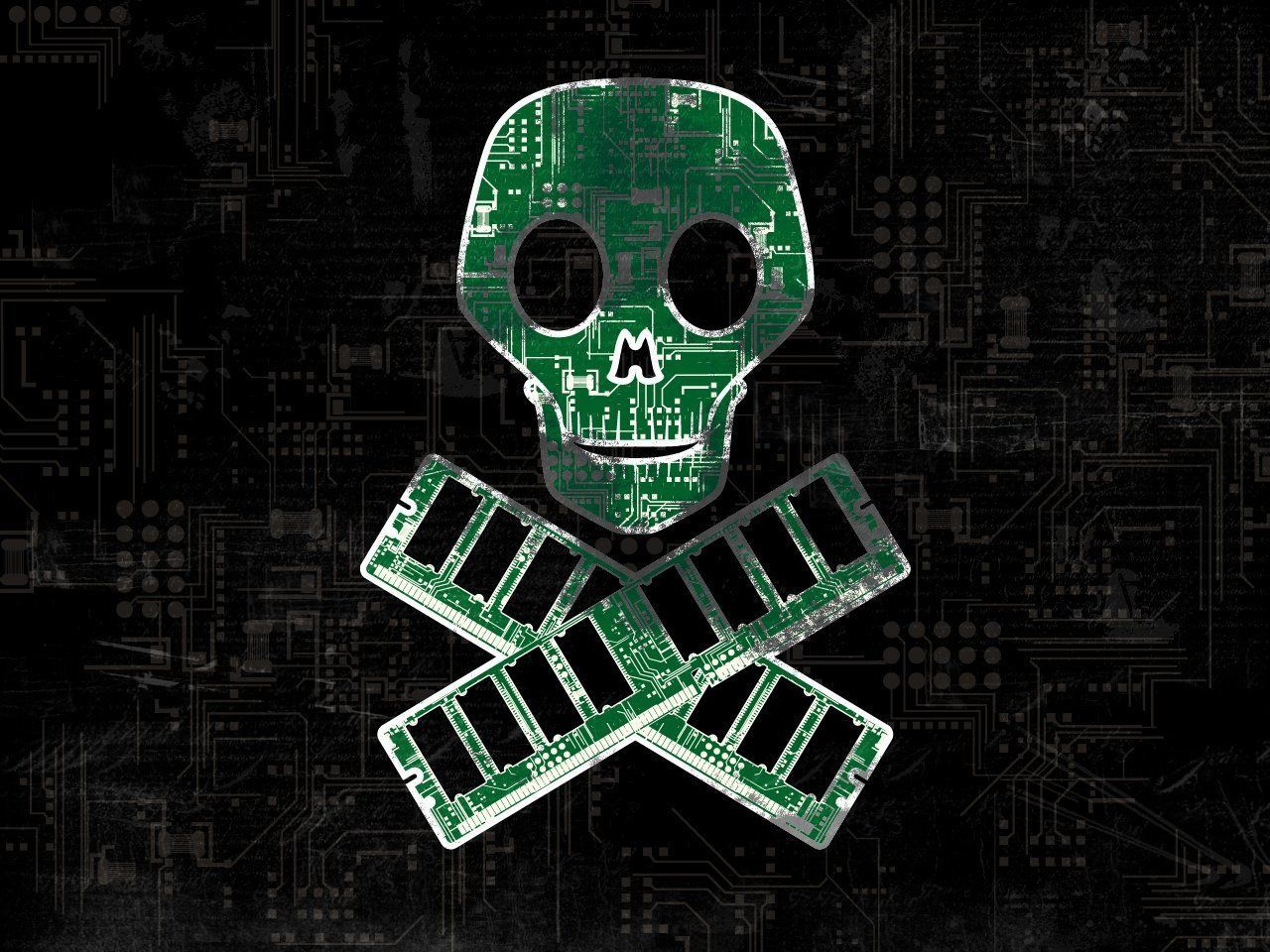
Feature Overview
QSORTZ()
Quick sort by z.
- sorts by reference to z from the argument table.
- See the code in the cart for the single value type
QSORT()
where table t is sorted by v. - This function consumes 76 Token.
tb={{id='a', z=1}, {id='b', z=2}, {id='c', z=1.5}, {id='d', z=-0.5}} ?'before sort',6 for i,v in pairs(tb) do ?v.id..':'..v.z end qsortz(tb,1,#tb) ?'\nafter sort' for i,v in pairs(tb) do ?v.id..':'..v.z end --[[ before sort a:1 b:2 c:1.5 d:-0.5 after sort d:-0.5 a:1 c:1.5 b:2 ]] |
nothing to see here; this cart is a bug repro for @zep
- download this cart:
load #teredubafu
- turn down your volume!!
- play sfx 30,31,64,72 in the sfx editor -- the a00/b00
effects cause a high-pitched artifact - note: sfx 65 and 73 are minimally altered versions of 64 and 72
and have no artifact
my system: picotron 0.1.0h / linux
Hi Pico-8 community! a few months ago I built a pico8 themed VS code extension, it follows the colour scheme of the pico 8 text editor, and I have also added instructions in the read me on how to get the font and the cursor!
You can get it here: https://marketplace.visualstudio.com/items?itemName=mai314.pico-8-theme&ssr=false#overview
or
-
Open the Extensions sidebar panel in VS Code. View → Extensions
-
Search for Pico-8 theme by mai314
- Click Install
Enjoy!
Screenshot examples
Is experiment on pal and rnd im add in function _init and function _update60
Photo of caracal im use Depict and im open file PICO-8 cartridge and paste in gfx

I'm working on something with lots of overlapping sprites, that need to be drawn in the correct order. After my failed attempts at implementing quicksort, I found a thread suggesting Z values could be used as keys in a table. My table of sprites will be rebuilt every frame, so the plan is to insert sprites at specific positions in the table once they're created. This way, I should hopefully be able to iterate over the table with a for loop without sorting it at all. Unfortunately, I wasn't able to figure the last part out.
table={} add(table, 10, 1) add(table, 20, 2) add(table, 15, 1.5) add(table, 27, 2.7) for i in all(table) do print(i) --[[ 15 27 10 20 ]] end print(table[1.5]) --[nil] print(table[1]) --15 |
As a test, I tried something like this, only to realize add()
floors your input keys, and ignores the keys of previously added items. What I really need for this to work, is a way to add a key and value to a table directly, and a way to stop PICO-8 treating my numerical keys like a sequence. I've tried adding items the way you would with string keys, but it didn't work.
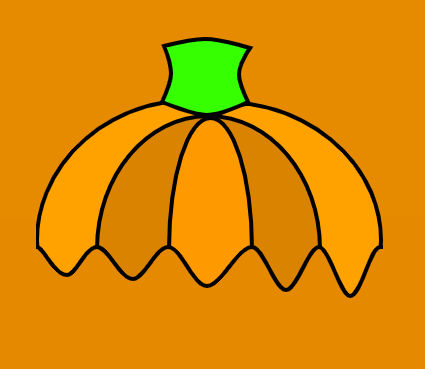
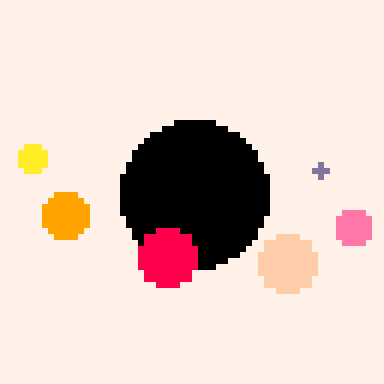

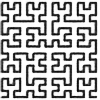
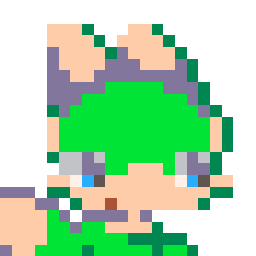
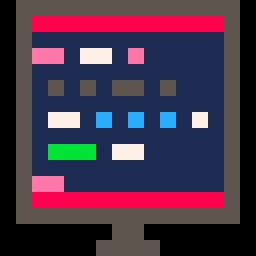
Big Header
Medium Header
Small Header
italics bold
Embed:
[**could not embed: 1234] // cartridge id
[tweet]
This is a code snippet within a line
while (true) do color(rnd(8)+8) print("multi-line code listing") end |
it contains the main steps of creation for a warhammer fantasy rp character of the fourth edition which is translated to spanish by Devir (i still don't put the step of the talents because i'm fighting with myself whether to put them in this cartridge or put them in a different one). i wanted to translate it to english and make it equal to the spanish version but i realized that i need the cubicle7 version xd because that one has the data of the roll tables different from the spanish version and i don't want anyone to be confused.
I plan to include the gnome and then try to translate it to english but I want it to be very faithful to the english version.
Tower Defense Game for Pico-8.
This game is inspired by the old flash games:
Bubble Tanks Tower Defence.
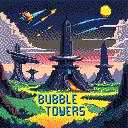
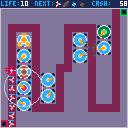
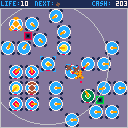
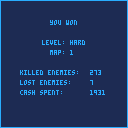
Description
This is not the usual tower defence game you are used to. Sure, you have to build towers to defend against enemy waves and also earn cash by destroying them to update your towers.
What is special here is that we not only can build defence towers to destroy enemies but also to define the path the enemies can take. Where the enemies have different skills like self-healing or flying over towers. In addition, we have various different maps where some may have blockades, are limited in space or have already predefined fixed paths.
Game Play
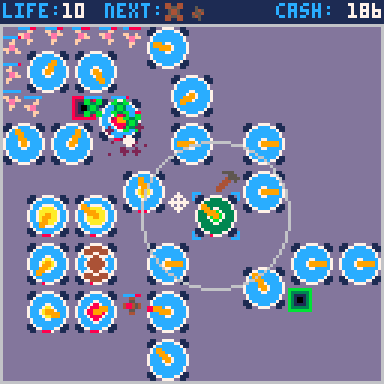
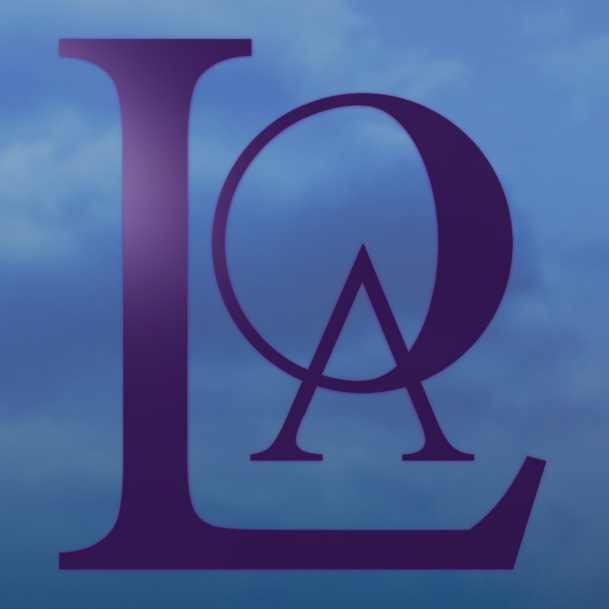
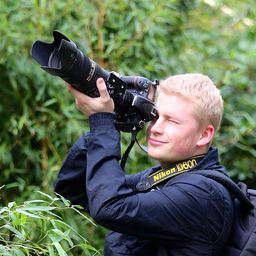
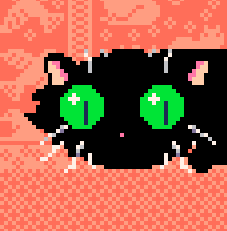
I'm working on a farming simulator game. In the _init() function it should randomly generate the pattern on the grass tile and then randomly place the grass tile on the map (replacing any empty tiles.) However, a strange bug is occurring where some map tiles are corrupt. Can somebody explain what's going on? Use arrow keys to move around.
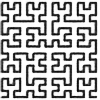

I have been attempting to make a Picotron game. When there are too many objects in a table that are being updated, some objects just do not update. I really don't know if this is a problem on my end or a Picotron bug.
I have falling rocks from the sky (as it is a sequel to Rockfall 3), and when there are maybe 10-20 of them at once, they will collectively pause in the air before continuing. The player movement does not turn choppy, and the CPU usage stays quite low (15% with about 100 rocks), so I don't think it's an optimization problem.
Here's the code in my rocks.lua
:
rocks = {} function add_rock(x,y,xv,yv) add(rocks, { x=x, y=y, xv=xv, yv=yv }) end function add_rock_master() add_rock(rnd(464),-rnd(32)-8,0,0) end function update_rocks() for i,r in pairs(rocks) do r.x += r.xv r.y += r.yv r.yv += grav r.yv /= wind_res if r.y > 270 then [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=151628#p) |

When turning knobs in the SFX or instruments editors, it can be difficult to adjust by dragging.
This happens when the window size is x2 or more and you use Alt + Tab to switch application windows.
As a temporary fix to this problem, you can restore adjustments by returning the window to normal size.
This has been the case since the version before v0.1.0h when the mouse lock function was added.
In "> Pico Hack_" you are a freelancer Hacker who take jobs for corporations.
You can be a Black Hat or White Hat. It is your choice.
Any comments, criticisms or ideas are welcome :)
release 1.0.1 (2024-08-03)
- fix "disconnect by server" outcome
- small fixes in account registration
- fix mission debriefing
- fix news



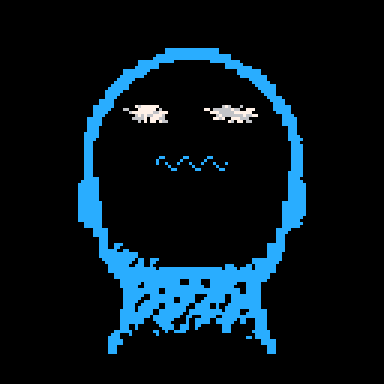
Sorry if this is a completely dumb question. I'm new to Pico 8 and game dev in general. I'm stuck in my first project attempting to randomize map layouts. My game is a simple vertical scrolling game where the player moves from side to side to avoid obstacles. I'd like to begin on one screen, and then end on a final screen with the ones in between being a random selection from the 12 or so other screens I've drawn. I know the easiest way to do this is probably with nested tables, but I'm having trouble understanding how I could accomplish this. Any help would be awesome.

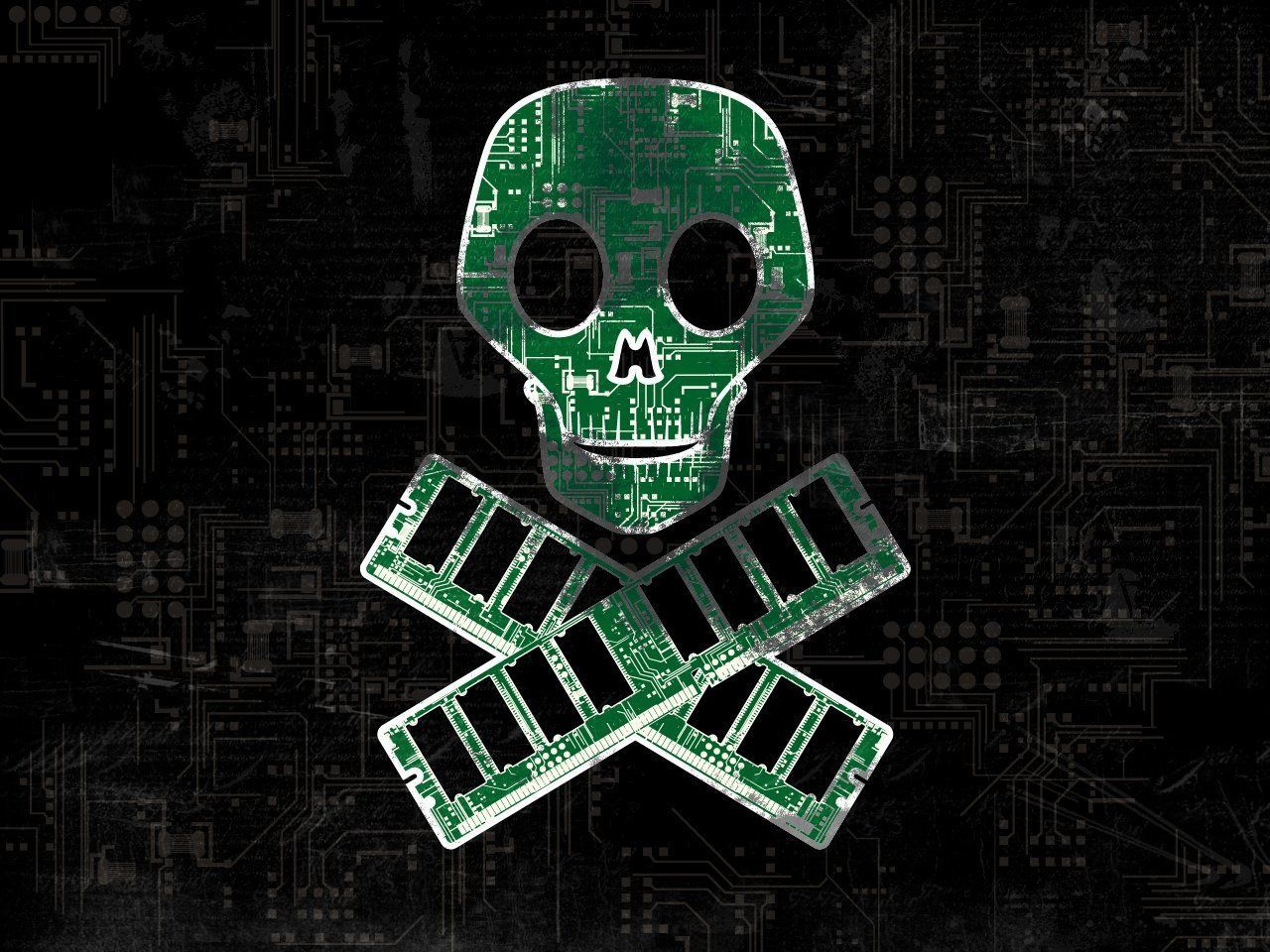