Sorry if this is a completely dumb question. I'm new to Pico 8 and game dev in general. I'm stuck in my first project attempting to randomize map layouts. My game is a simple vertical scrolling game where the player moves from side to side to avoid obstacles. I'd like to begin on one screen, and then end on a final screen with the ones in between being a random selection from the 12 or so other screens I've drawn. I know the easiest way to do this is probably with nested tables, but I'm having trouble understanding how I could accomplish this. Any help would be awesome.



So, you're looking at a few separate tasks here. The first one is the creation of the map layouts, which it sounds like you've already done. The second is having the game decide on a random order. The third is preparing the maps for use in gameplay. The last thing (other than gameplay itself) is drawing the maps. The only notes I have on the first task might be too advanced and would involve unnecessarily creative solutions, so I'll skip that.
deciding a random order
This is a task that needs to be done once per round or game instance or whatever. The point is it doesn't need to be done during gameplay and your game doesn't sound complicated enough to care about tokens. That means the most human way to do it is fine.
Here's one possible way to just make a list of numbers and then randomly add them to a sequence:
function make_sequence(num_maps) local seq = {1} --starting room first local t = {} -- fill t with the maps to randomize for i=2,num_maps-1 do add(t, i) end -- add them to seq at random while #t > 0 do local v = rnd(t) add(seq, v) del(t, v) end -- add the final map add(seq, num_maps) return seq end |
If this code doesn't feel intuitive, I encourage you to make your own version. It's generally a good idea to make sure you feel good about any code you use.
preparing the maps for gameplay
You mentioned nested tables. That's perfectly fine. If the map layouts are actually drawn on the pico-8 map, you can use mget()
to read them into lua memory at the start of the game. From there you can either use the sprite id's directly or you can use fget()
to fetch the flag information. If all you need are the positions of obstacles, then you can just check each map position for if it's an obstacle, add the ones that are to your tables, and then ignore the rest.
drawing the maps
Again, it sounds like you've drawn the map layouts to the pico-8 map. If so, it'd probably be fastest to just draw all the map layouts in order each frame and move the camera as the game progresses. This is because pico-8 will filter out any commands that don't do anything in-engine where it'd be faster to do so. As such, you can just loop through the sequence determined at the start and use map()
on each one. This is what I would recommend for anyone new, since it involves way less complex calculations than manually drawing each tile. You just need to know where each map layout will be on the pico-8 map. This can either be done by keeping them the same size and doing a grid calculation, or by just making a table and putting the {x, y, w, h} rect for each map layout in it. Just make sure the the positions you draw the maps at match up with how you represent the player and obstacle positions.


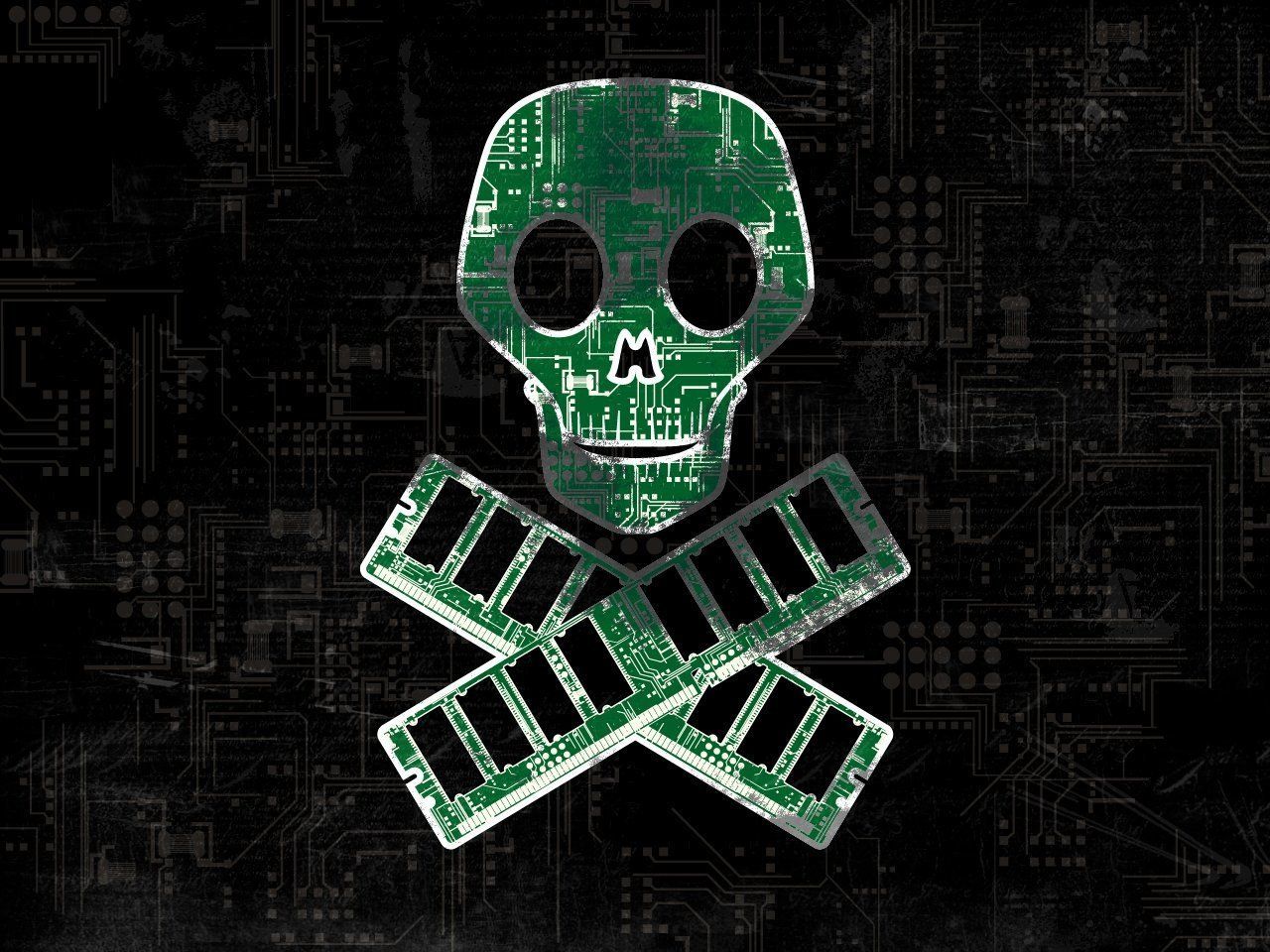
Thanks for your help. That works great to randomize a list of maps. Unfortunately, my attempts to scroll the screens broke the game and my brain lol. I've pivoted and changed some elements so hopefully take two goes better.
[Please log in to post a comment]