I wanted a tool to help me import a large folder of asset .pngs (such as one you might get from https://kenney.nl/) into .gfx files. I know there are tools such as @pancelor 's helpful importpng.p64 (or just click-n-dragging onto the gfx editor), but I didn't want to do that for hundreds of files.
Using importpng.p64 as a foundation, I create a command-line utility for Picotron that will import entire folders of assets into .gfx files! Below is the snippet that you'll put in /appdata/system/utils/simport.lua
-- a tool to import all pngs in a folder into a single .gfx file -- tool by @fletch_pico -- version 1.1 -- -- makes use of: -- importpng.p64 code by @pancelor -- https://www.lexaloffle.com/bbs/?tid=141149 cd(env().path) local argv = env().argv if (argv[1] == "--help") then print("Usage: simport [FOLDER]") print("Populate the .gfx file using a folder of PNGs.") [ [size=16][color=#ffaabb] [ Continue Reading.. ] [/color][/size] ](/bbs/?pid=151555#p) |
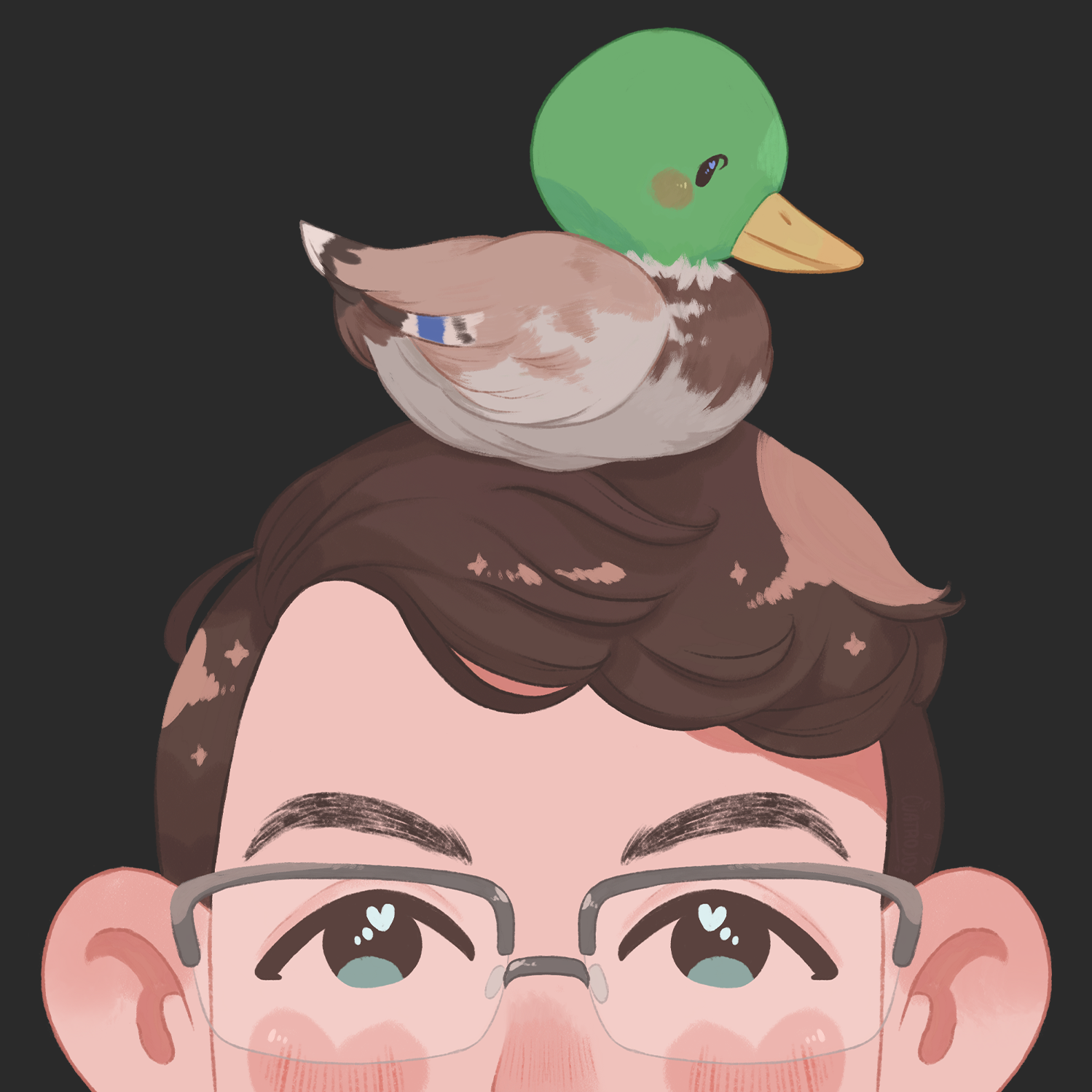
Hauler
Welcome to the Zeta Sector in a remote part of the Milky Way. You are a Space Trucker for the Eastwood Mifune Cooperative. Your job is to transport cargo and people from one space station to another. Or deliver a delicious Moloko Shake from a diner to a customer.
Explore a compact sector of the galaxy with planets to scan, jobs to complete, and Easter eggs to discover. Only one thing seems to be missing: Good coffee. Can you solve the mystery of where to get it?
What you can do
- Docking at stations to accept fetch quests.
- Fuel scoop at stars.
- Collect salvage or mine resources to sell.
- Scan planets to document the sector.
- Use your friendship drive to jump to other systems.
This game was made for the Pursuing Pixels James Jam Game Gam #3 with theme parallel. Some art (mainly my trees and grass) were taken from the first version: Druid Dash: Magic Mushroom Mania
Shorter than what I wanted, but that is the life of weeklong jams that you develop under 10 hours.
There is a bug that sometimes comes up with spirits not moving, you will have to restart the stage with C x)
Gameplay:
Move spirits around the map and guide them to their shrines. Once they move, they will continue to do so until they hit a wall.
⬅️⬇️⬆️➡️ - Move around
❎: X - Next Stage
🅾️: C or Y/Z - Restart Level
Devlogs:
You can read all about the development if you are into things like that:
This game was made for the Minigame A Month May 2024 for theme WIZARDS!
Gameplay:
Move around on the field to avoid the relentless attacks of the mages.
⬅️⬇️⬆️➡️ - Move around on the board.
❎: X - Activates Currently held Item.
🅾️: C or Y/Z - Switch your current color.
Stand on appearing attack spots with the right color to hurt the mage of that color.
Items:
Sands of Time: Freezes time for the world. You are allowed to move, but nothing else.
Chromatic Star: Allowes you to become invulnerable, and hurt any mage while stepping on attack spots.
Wizards:
Each wizards has a unique logic behind their patterns as three distinc phases with them. Figure out who does what an plan ahead!
I've struggled with collision for a long time, but I've discovered/learned two collision types that can be implemented into just about any game genre.
This is my first time writing a tutorial so please any advice or mistakes a must.
Some background:
PROBLEMS AND SOLUTIONS OF THESE COLLISIONS WILL BE LOCATED AT THE BOTTOM
OBJECT-TO-OBJECT COLLISION
This collision type involves four to eight parameters.
- A first X value (
x1
)
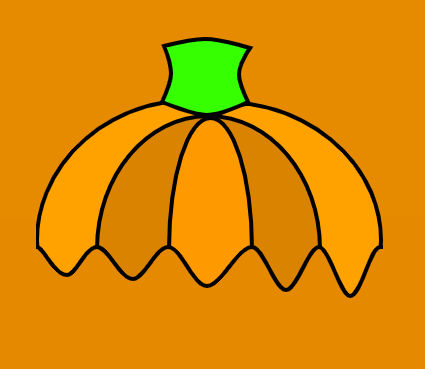
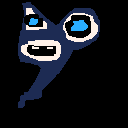

Knucklebones
Controls
⬅️⬆️➡️⬇️ Move cursor ❎/X or 🅾️/Z Select
Rules
Each player has a 3x3 grid, organized into three columns. They take turns rolling a die and placing it into an open spot in their grid. If there is a matching die in their opponent's matching column, the opponent must remove all matching dice in that column. When one player's grid is full, the game is over and the player with the highest score is the winner.
Scoring
Each column is calculated separately, then added to the player's total score. If there are matching dice in the column, their values are added together and multiplied by the amount of matching dice.

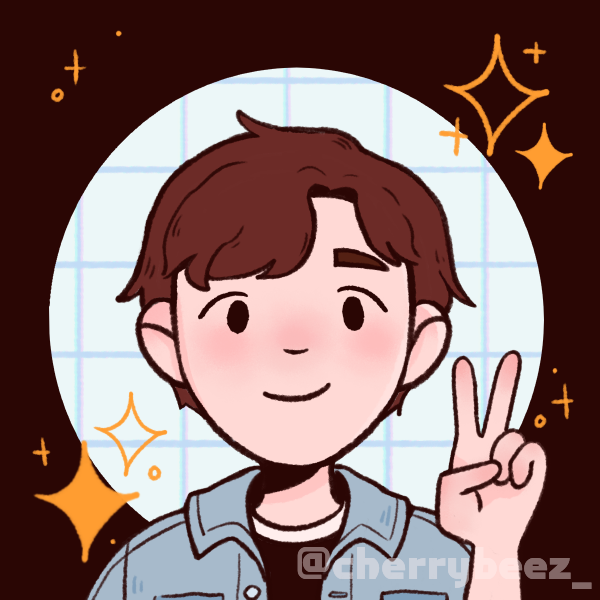



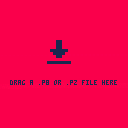
I am working on compressing .p8 files.
That's all you need to know.
Currently it is only saving characters by only advancing tokens once.
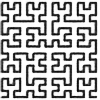

As far as I know, every variable is returning as nil. That doesn't mean that it is. I cant get far enough into my game to figure out more
I am unsure what i did. I have gone through my code multiple times and i am just done currently. Any help or advice would be greatly appreciated.
[hidden]
-- game loop function _init() gamestart() _upd=update_game() _drw=draw_game() end function _update() frm+=1 _upd() end function _draw() _drw() end function gamestart() frm=1 col=false ---------------- --menus ---------------- men_main={ items={"start game","controls"}, menx=10, meny=10, curx=2, cury=10, menoff=7 } ---------------- --player ---------------- p={ sp=1, x=48, xo=0, y=40, yo=0, w=8, h=8, ymt=0, spd=1, flp=false, dx=0, dy=0, --gravity/collision stuff hbx1=1, hbx2=6, hby1=7, hby2=1, grv=0.2, acc=0.5, landed=false, jumping=false, running=false, falling=false, dashing=false, rolling=false, attacking=false, itemgrab=false, --animation (in sprite #) frame=0, anim_idle=1, anim_walk={1,2,3,4}, anim_jf=5, anim_atk={1,4,6}, anim_dashing=8, anim_rolling={9,10,11,9}, anim_attacking=6, anim_itemgrab=7, --game stats mhp=10, chp=10, atk=1, def=1, agi=1.1,--1.1 beacuse fucking math } inv={} abilities={} ---------------- --tiles ---------------- --collision t={--x1=l x2=r y1=t y2=b wall={x1=4,x2=6,y1=0,y2=7}, floor={x1=0,x2=7,y1=4,y2=7} } end -->8 -- updates function update_game() --player--------------------- ----------------------------- p.frame+=1 if p.frame==5 then p.frame=1 end if (btn(⬅️)) then p.dx=-1 p.flp=true p.running=true elseif (btn(➡️)) then p.dx=1 p.flp=false p.running=true else p.dx=0 p.running=false end --collisions & gravity local ly=p.y p.dy+=p.grv --check collision left and right if p.dx<0 then if collide_map(p,"left",0) then p.dx=0 end elseif p.dx>0 then if collide_map(p,"right",0) then p.dx=0 end end --jump if btnp(⬆️) and p.landed then p.dy-=2 p.landed=false end --check collision up and down if p.dy>0 then p.falling=true p.landed=false p.jumping=false if collide_map(p,"down",0) then p.landed=true p.falling=false p.dy=0 p.y-=((p.y+p.h+1)%8)-1 end elseif p.dy<0 then p.jumping=true if collide_map(p,"up",0) then p.dy=0 end end p.x+=p.dx p.y+=p.dy --animation if p.jumping or p.falling then p.sp=p.anim_jf elseif p.running then if frm%5<=4 then p.sp=p.anim_walk[p.frame] end elseif p.attacking then p.sp=p.anim_attack else --player idle p.sp=1 end end function update_mm() upd_menu(men_main) end -->8 -- draws function draw_game() cls() map() spr(p.sp,p.x,p.y,1,1,p.flp) camera(p.x-60,p.y-60) print(p.dx,1+p.x-59,1+p.y-59,8) print(p.dy,9+p.x-59,1+p.y-59,8) end function draw_mm() cls() menutext(men_main.items,men_main.menx,men_main.meny,8) drw_menucur(men_main) end -->8 --functions function does_tile(flag,x,y) -- bool tile=mget(x,y) has_flag=fget(tile,flag) return has_flag end function collide_map(obj,aim,flag) --obj = table needs x,y,w,h --aim = left,right,up,down local x=obj.x local y=obj.y local w=obj.w local h=obj.h local x1=0 local y1=0 local x2=0 local y2=0 if aim=="left" then x1=x-1 y1=y x2=x y2=y+h-1 elseif aim=="right" then x1=x+w-1 y1=y x2=x+w y2=y+h-1 elseif aim=="up" then x1=x+2 y1=y-1 x2=x+w-3 y2=y elseif aim=="down" then x1=x+2 y1=y+h x2=x+w-3 y2=y+h end --pixels to tiles x1/=8 y1/=8 x2/=8 y2/=8 if fget(mget(x1,y1), flag) or fget(mget(x1,y2), flag) or fget(mget(x2,y1), flag) or fget(mget(x2,y2), flag) then return true else return false end end -->8 --menu function startmenu(menutable) end function menutext(menutable,x,y,off) for i=1, #menutable do print(menutable[i],x,y+menutable.off*i) end end function drw_menucur(menutable) local x1 = menutable.curx-8 local y1 = menutable.cury-10 local x2 = x1+12 local y2 = y1+6 rectfill(x1,y1,x2,y2,7) end function upd_menu(menutable) if btnp(⬆️) then menutable.cury-=7 end if btnp(⬇️) then menutable.cury+=7 end -- if btnp(❎) then menuacc(menutable) -- if btnp(🅾️) then menuden(menutable) end |

I've been trying for a day to figure this out and I can't. There's barely any information online about this. Although it feels like everyone knows it but me.
My idea is on initialize to run a nested for loop and add any enemies to a list. And in the update move them.
I did this and it worked! But it incremented in grids. There's no actual code of this since I deleted it, but I could easily reprogram it, if someone asks what the block was.
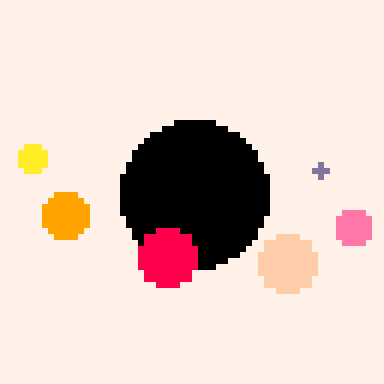
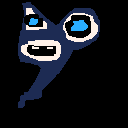

This game is a WIP prototype with a few known (but rarely game-breaking) bugs.
Progression is not persisting between sessions here on Lexaloffle, so I updated the game to unlock level select. I recommend trying to beat each level before progressing, but not everybody has time for that!
Concept
Spoiler alert: these billiards are not regular (and they certainly aren't perfect). I wanted to take the game of billiards, which I've always enjoyed both in real life and in video game form, and turn it on it's head a little. As you play through the 25 levels in this prototype, you will encounter more and more... irregularities.
Story
Bill Iards is a perfectly regular person. He is perfectly happy with his perfectly regular life. One day, a man in a suit knocks on his door, bringing news of the passing of his estranged Uncle Whiz. Bill had no recollection of such a relative, but in any case, Uncle Whiz had willed to him one item: a billiards table.

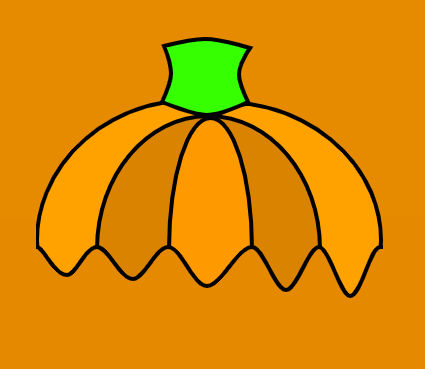
This is my second 3D game demo. But I am not finished it yet.
control:
common mode:
press ⬅️ to turn left
press ➡️ to turn right
press ⬆️ to move forward
press ⬇️ to move backward
press 🅾️ to shift to left
press ❎ to shift to mouse mode
mouse mode:(only available on splore)
use your mouse to turn around
press Key_S to shift to left
press Key_F to shift to right
press Key_E to move forward
press Key_D to move backward
press ❎ to shift to common mode
Done:
--basic raycasting
--textured wall
--static sprite
plan:
--animated sptite
--moving enemies
--shoot game
--dialogue
Bite-sized Celeste Classic mod.
Requires no tech.
Intermediate-Advanced difficulty. Designed with non-linearity in mind.
The character featured is Cash, designed by Lash sleeper. I do not own them.
This card was designed under 3 days for the artfight 2024 event.
v1.2 : Changed menu music (again)
v1.1 : Changed menu music
v1.0 : Initial release

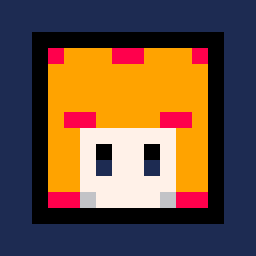
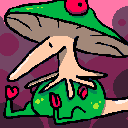

