I based this post on an article by nerdyteachers
NB: to make the code easier to read, the examples shown here contain spaces, which is not recommended in Pico 8 to save space.
How to write a class~ish
We will start with a simple class:
function monster(x, y, sp) m={x=x,y=y,sp=sp or 0} function m.update(s) s.x+=1 end function m.draw(s) spr(s.s,s.x,s.y) end return m end |
The word class is a bit of a misnomer, because in reality monster
is just a function.
This function constructs the class instance, using a table
.
Attributs
Inside the table
You will write the attributes of your class:
function monster(x,y) p={x=x,y=x} ... |
Note that the function's parameters act as constructors, allowing arguments to be passed to the class.
Methods
There are two ways to write a method with a :
or with a .
.
The first way is the more usual in Lua, because in this way you can omit the parameter reserved for the class instance.
The parameter self
automatically exists in your function without declaration.
The other way ask you to declare, in first place, a parameter reserved for the class instance.
In short, function m:draw()
can be written like that function m.draw(self)
.
We will prefer the second way because it's make economize tokens using this solution.
For instance, the method draw will look like that
function m.draw(s) spr(s.sp,s.x,s.y) end |
Using the class
To create an instance of the class, you just need to use the function mo=monster(64,64,0)
And to call a method is also simple mo:update
Note that using :
as a link between the instance and the method, is a way of avoiding re-passing the instance to the method. Simply put, writing mo:draw()
is a way to avoid writing mo.draw(mo)
Here is an example of code using the class monster
function _init() mo=monster(64, 64, 0) end function _update() mo:update() end function _draw() cls() mo:draw() end |
Inheritance~ish
Here is the spider class that inherits from monster
function spider(x, y) spi=monster(x,y,0) return spi end |
It's yet really basic, but it works.
New attribute
If you want to add a new attribute you can proceed like it was a table
, (because it's a table
)
function spider(x, y) spi=monster(x,y,0) spi.hp=50 return spi end |
New method
To add another method, you just do as you did before.
function spider(x, y) spi=monster(x,y,0) spi.hp=50 function spi.damage(s,d) s.hp-=d end return spi end |
Overwrite a method
The most "complex" task is the overwriting.
Before, you need to understand that a function is store in memory, in the same way that a variable.
For instance, if I want, I can store print
in a variable a and use a
as print
.
a = print function _draw() a("This is a test.", 5, 10) end |
It works because when you write a=print
, a
contains a reference to the print
function.
Now that we are aware of that, we can proceed to overwriting.
If I want to add a behavior to my spider
on update
, in addition to the existing code already inside the update
method from monster
, I will proceed like this:
... spi.supspiupdate=spi.update function spi.update(s) spi.supspiupdate(s) s.y +=1 end ... |
First you store the update method from the ancestor inside a variable here spi.supspiupdate
This variable need to be unique inside your class (including parent and child).
Then you can overwrite your function.
You can use the variable with the reference of the ancestor method, inside the new method.
Note that we pass directly the instance to the function (s
passed as argument to s.supspiupdate
).
Like this, you can have almost every feature expected by object-oriented programming.
function monster(x, y, sp) m={x=x,y=y,sp=sp or 0} function m.update(s) s.x+=1 end function m.draw(s) spr(s.s,s.x,s.y) end return m end function spider(x, y) spi=monster(x,y,0) spi.hp=50 function spi.damage(s,d) spi.hp-=d end spi.supspiupdate=spi.update function spi.update(s) spi.supspiupdate(s) s.y +=1 end return spi end |
function _init() sp=spider(64, 64) end function _update() sp:update() end function _draw() cls() sp:draw() end |
So i was trying to make an ASCII style procedural runner with a death wall that constantly approaches and enemies to dodge and spike traps to avoid. Im kinda stuck and am getting an error on line 29. Any ideas?
-- ASCII RUN
-- Constants
player = {x=20, y=64, vy=0, on_ground=false, sprite='@'}
ground_y = 96
gravity = 0.3
jump_power = -2.5
death_wall_x = 0
speed = 1.5
score = 0
level = {}
define_tiles = {
[' '] = {solid=false}, -- Empty space
['#'] = {solid=true}, -- Ground
['^'] = {solid=true, deadly=true}, -- Spikes
['E'] = {solid=true, deadly=true} -- Enemy
}
-- Generate procedural level chunks
function generate_chunk()
local chunk = {}
for i=1,16 do
local tile = ' '
if i > 12 then
tile = '#' -- Ground
elseif i == 12 and math.random(1, 5) == 1 then
tile = '^' -- Spikes
elseif i == 11 and math.random(1, 10) == 1 then
tile = 'E' -- Enemy
end
add(chunk, tile)
end
return chunk
end
function init_level()
for i=1,32 do
add(level, generate_chunk())
end
end
init_level()
function update_player()
-- Gravity
player.vy += gravity
player.y += player.vy
-- Collision with ground
if player.y > ground_y then
player.y = ground_y
player.vy = 0
player.on_ground = true
else
player.on_ground = false
end
-- Jumping
if btnp(4) and player.on_ground then
player.vy = jump_power
end
end
function update_level()
-- Scroll the level left
death_wall_x += speed
score += 1
-- Remove old chunks and add new ones
if #level > 0 and death_wall_x % 8 == 0 then
deli(level, 1)
add(level, generate_chunk())
end
end
function check_collision()
local px = 2 -- Player's x position in array (adjusted for indexing)
local py = flr((player.y - 64) / 8) + 1
if level

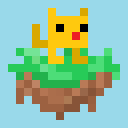
Sweep life's troubles away!
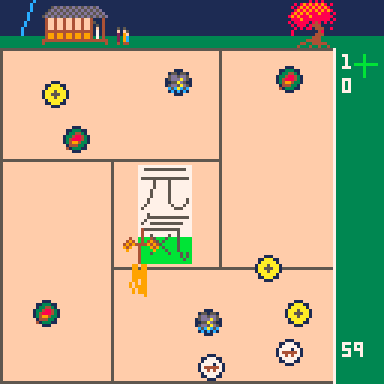
Controls
Move with the directions/arrows
Sweep left and right with the buttons (sweep directions can be flipped in the pause menu)
Hold both buttons to auto sweep quickly
How to play
Sweep the various troubles into groups of 3-5 before sweeping them off the right side to score points.
Game over when the time runs out, a group of 6 forms, or 42 troubles are on the board.
Made with help from
ThaCuber's vector.p8
Gruber's Pico-8 Tunes Vol. 2
Updates
Mar 23, 2025

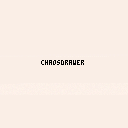
an interactive drawing tool to create and explore unpredictably chaotic black-and-white graphics

This cart has already had its ID written to the bottom of the MAP (x:0-127, y:32-64).
When you run it, the following processes are performed.
- Change the drawing target to the sprite sheet
- Call
rectfill()
with color 0 on the bottom of the sprite sheet (shared with the bottom of the MAP) - Change the drawing target to the screen
- Draw the bottom of the MAP
The screen should turn completely black as expected, but the MAP continues to draw what it was before it was updated.
Finally, it calls cstore()
to exit, and when you run it again, it will update to a completely black screen.
https://goblindriver.itch.io/murdercrab <- gotta download the carts here if you wanna play.
https://www.youtube.com/watch?v=9iiId70Op0o <-- gameplay
Murdercrab! A 5 stage caravan thing that I'm currently tweaking. There is a bug or two but should be able to make it all the way through to the TLB. There are lots of knobs under the hood to mess with level scaling and balance, let me know if you come up with a good balance. I'm still dinking around with the bullet patterns as I'm not really happy with them atm.
Ripped all the music from pico tunes, so all credit goes to gruber for music
Update 1
I found the fun
Most of the balancing and patterns are in a better state, although the game is too easy.
The core gameplay loop is locked for the most part. I'm still chasing a couple of errors and bugs, especially with the hi score.
Next step is to split it in to two carts and polish
Update 2
did the split and a little polishing. High scores still broken.
Hmm, ill have to figure this out later, it's not loading right in the BBS. If you download both carts you should be able to play it fine. Start with the menu cart
Well, this is no pong.
This game was made for the NOKIA 3310 JAM 6, in like 2 days or so.
The entire game was made in only like 823 compressed bytes! which is like a 1/20 of the original donkey kong game :O
Check out the original jam post Nokia Jam / Nopong
READ THE CONTROLS!
- Left and right to move
- DOWN TO GAIN MOMENTUM DOWNWARTS
- you have to keep your height to break the blocks
Gain points by breaking blocks,
when you break all blocks you have to die ONCE and then go on the platform again,
I recommend playing it with sound, have fun :)