So i was trying to make an ASCII style procedural runner with a death wall that constantly approaches and enemies to dodge and spike traps to avoid. Im kinda stuck and am getting an error on line 29. Any ideas?
-- ASCII RUN
-- Constants
player = {x=20, y=64, vy=0, on_ground=false, sprite='@'}
ground_y = 96
gravity = 0.3
jump_power = -2.5
death_wall_x = 0
speed = 1.5
score = 0
level = {}
define_tiles = {
[' '] = {solid=false}, -- Empty space
['#'] = {solid=true}, -- Ground
['^'] = {solid=true, deadly=true}, -- Spikes
['E'] = {solid=true, deadly=true} -- Enemy
}
-- Generate procedural level chunks
function generate_chunk()
local chunk = {}
for i=1,16 do
local tile = ' '
if i > 12 then
tile = '#' -- Ground
elseif i == 12 and math.random(1, 5) == 1 then
tile = '^' -- Spikes
elseif i == 11 and math.random(1, 10) == 1 then
tile = 'E' -- Enemy
end
add(chunk, tile)
end
return chunk
end
function init_level()
for i=1,32 do
add(level, generate_chunk())
end
end
init_level()
function update_player()
-- Gravity
player.vy += gravity
player.y += player.vy
-- Collision with ground
if player.y > ground_y then
player.y = ground_y
player.vy = 0
player.on_ground = true
else
player.on_ground = false
end
-- Jumping
if btnp(4) and player.on_ground then
player.vy = jump_power
end
end
function update_level()
-- Scroll the level left
death_wall_x += speed
score += 1
-- Remove old chunks and add new ones
if #level > 0 and death_wall_x % 8 == 0 then
deli(level, 1)
add(level, generate_chunk())
end
end
function check_collision()
local px = 2 -- Player's x position in array (adjusted for indexing)
local py = flr((player.y - 64) / 8) + 1
if level[px] and level[px][py] then
local tile = level[px][py]
if define_tiles[tile] and define_tiles[tile].deadly then
game_over()
end
end
end
function game_over()
cls()
print("Game Over! Score: "..score, 40, 60, 8)
stop()
end
function _update()
update_player()
update_level()
check_collision()
-- Check if player hits death wall
if player.x <= death_wall_x then
game_over()
end
end
function _draw()
cls()
print("Score: "..score, 2, 2, 7)
-- Draw level
for x=1,#level do
for y=1,#level[x] do
local tile = level[x][y]
if tile ~= ' ' then
print(tile, x*8 - death_wall_x, y*8 + 64, 7)
end
end
end
-- Draw player
print(player.sprite, player.x, player.y, 7)
end



Can you tell us which line is 29 so we don't have to count them, or post the cart?


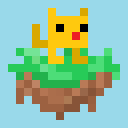
Pico-8 doesn't use the math library that comes with Lua, so it throws a runtime error when it tries to find the math.random
function. Instead it uses its own functions for better performance. Replace math.random with rnd and it should work.
[Please log in to post a comment]