the thing is, this error only happens when I attempt to make a climbable wall.
here's my setup:
my collisions page:
--collisions
function collide_map(obj,aim,flag)
--obj = table needs x,y,w,h
--aim = left,right,up,down
local x=obj.x
local y=obj.y
local w=obj.w
local h=obj.h
local x1=0 local y1=0
local x2=0 local y2=0
if aim=="left" then
x1=x-2 y1=y
x2=x-1 y2=y+h-1
elseif aim=="right" then
x1=x+w+2 y1=y
x2=x+w+1 y2=y+h-1
elseif aim=="up" then
x1=x+3 y1=y-1
x2=x+w-3 y2=y
elseif aim=="down" then
x1=x+2 y1=y+h
x2=x+w-3 y2=y+h
end
--player hitbox test
x1r=x1 y1r=y1
x2r=x2 y2r=y2
--pixels to tiles
x1/=8 y1/=8
x2/=8 y2/=8
if fget(mget(x1,y1), flag)
or fget(mget(x1,y2), flag)
or fget(mget(x2,y1), flag)
or fget(mget(x2,y2), flag) then
return true
else
return false
end
end
my player page:
--player
function player_update()
--physics
player.dy+=gravity
player.dx*=friction
--controls
if btn(⬅️) then
player.dx-=player.acc
player.running=true
player.flp=true
end
if btn(➡️) then
player.dx+=player.acc
player.running=true
player.flp=false
end
--slide
if player.running
and not btn(⬅️)
and not btn(➡️)
and not player.falling
and not player.jumping then
player.running=false
player.sliding=true
end
--jump
if btnp(❎)
and player.landed then
player.dy-=player.boost
player.landed=false
end
--check collision up and down
if player.dy>0 then
player.falling=true
player.landed=false
player.jumping=false
player.dy=limit_speed(player.dy,player.max_dy)
if collide_map(player,"down",0) then
player.landed=true
player.falling=false
player.dy=0
player.y-=((player.y+player.h+1)%8)-1
end
elseif player.dy<0 then
player.jumping=true
if collide_map(player,"up",1) then
player.dy=0
end
end
--check collision left and right
if player.dx<0 then
player.dx=limit_speed(player.dx,player.max_dx)
if collide_map(player,"left",1) then
player.dx=0
end
elseif player.dx>0 then
player.dx=limit_speed(player.dx,player.max_dx)
if collide_map(player,"right",1) then
player.dx=0
end
end
--stop sliding
if player.sliding then
if abs(player.dx)<.2
or player.running then
player.dx=0
player.sliding=false
end
end
player.x+=player.dx
player.y+=player.dy
--limit player to map
if player.x<map_start then
player.x=map_start
end
if player.x>map_end-player.w then
player.x=map_end-player.w
end
end
function player_animate()
if player.jumping then
player.sp=7
elseif player.falling then
player.sp=8
elseif player.sliding then
player.sp=9
elseif player.running then
if time()-player.anim>.07 then
player.anim=time()
player.sp+=1
if player.sp>6 then
player.sp=3
end
end
else --player idle
if time()-player.anim>.3 then
player.anim=time()
player.sp+=1
if player.sp>2 then
player.sp=1
end
end
end
end
function limit_speed(num,maximum)
return mid(-maximum,num,maximum)
end
--attempting to climb
function player_climbing()
if collide_map(player,"right",2)
and btn(❎) and btn(⬆️)
then player.y+=500
end
end
flag 2 is meant to be climbable walls.
should this not increase my vertical velocity by 5?
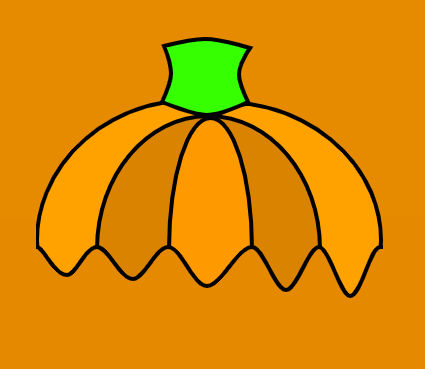
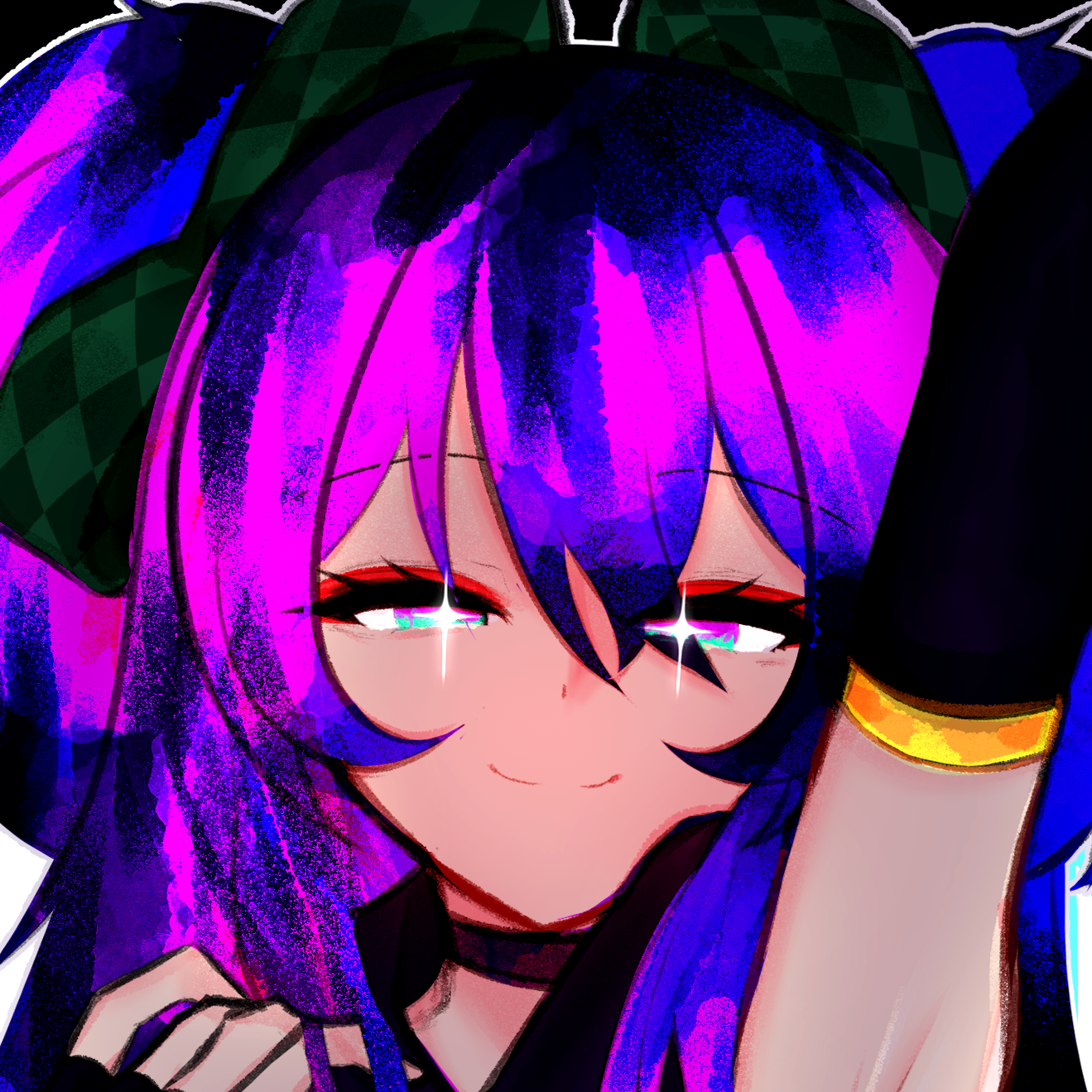
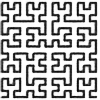
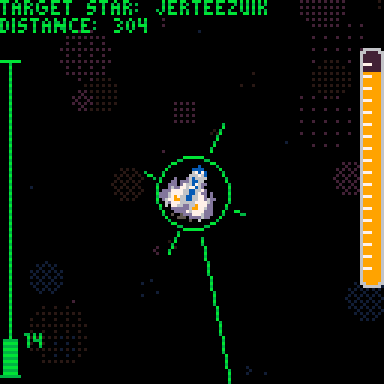
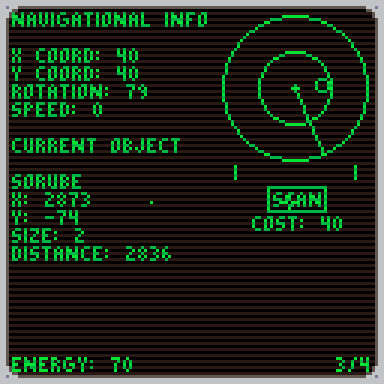
Controls:
Left and Right Arrow keys to rotate the ship and navigate through menus
X to open menus
C to absorb stars
Mouse and Left Click to select things in menus
Arrow key Up to accelerate
Enter to open the PICO-8 pause menu
Game:
In Star Devourer you are the captain of The Aurora, one of the last ships supporting the remnants of humanity. However, as the universe is slowly dying, fewer and fewer stars remain, making energy more and more difficult to come by. Your task as captain is to Locate remaining stars, Approach them and Absorb them, to support the energy needs of The Aurora. Energy is sparse so being mindful of your energy usage is of utmost importance! With whatever energy margins you have, you can upgrade The Aurora, making future energy gathering easier!
Info and Credits:
This game was made in about 1 week for the JumJam#2 game jam and is my second finished game I have released. I have never been so stressed making a game before haha. The code is really sloppy and several things had to be rushed and/or scrapped. However, I managed to create a final game that, although a bit basic, I am very proud of! I poured my everything into finishing this and I sincerely hope you'll enjoy it!
Everything is written, coded, drawn and developed by me!
Except for!
Easing functions by ValerADHD
Hello! This is my first post here, to present my also first game in Pico-8 :D As the title read, yes, it is another Tic-Tac-Toe, but I picked this one because I consider it an easy playground to learn the very basics of the platform without having to worry about complex gameplay or content. This is not the first time I develop a game... it is the second! My first one was 14 years ago for iOS, a small match-3 that was super fun to make.
My version of Tic-Tac-Toe is the vanilla one, and the simplest possible in terms of development: just 2 players, no CPU, sharing P1 controls. No algorithm to check board, no fancy modes, nothing, just pure old TTT. I've introduced some sound and particle effects to learn how to do it and that's all.
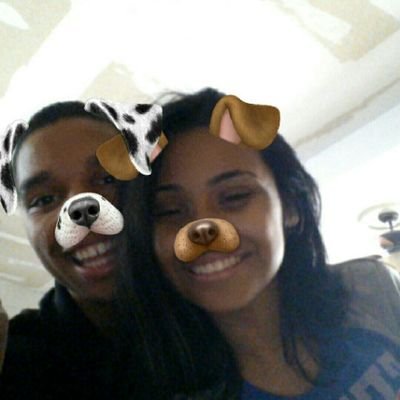
Movement Keys:
Arrow Keys to move and look
X to dash
C to jump
There are 9 levels in total, if you are stuck at a level press 'F' to skip forward. If you've accidentally pressed F, press 'S' to go back
pls play my level then fill in the form...
https://forms.gle/xYzABGA9tiXM6u2T9
Im really sorry if this topic has been there already, didn't find anything about it so i thought i might post it. So its not news that some regions dont have access to PayPal and/or Swift systems. I was wondering if there is a way to get around? Steam maybe? That also accounts for pico-8. Educational edition is cool and all but license is far better.
If no, well, bummers. If yes please tell me.

So I was playing around with making a dynamically generated scrolling background, something simple, and I thought about layers of water, with different hues, and some dithering, by slapping strips of tiles to the screen. And that got me thinking about foolish billionaires and implosions and an innocent young man pressured by his father.
So, anyway, I made an extremely simple little "simulation" of a trip in a suspiciously familiar-looking submersible down to the Titanic, except things go wrong.
Cursor keys make you go up or down. That's it. There's nothing else you can do, if even that after some point.
There's not much to this. It's not so much a game as dark commentary. If this sort of thing bothers you, please don't play it.
It's as finished as it's gonna get.


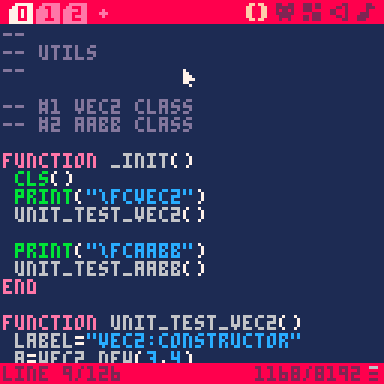
A simple idea I wanted to share with you.
I use a p8 file with a few utility classes. I place the unit tests on the first tab.
When I run my library code, it tests it.
If I want to include my vector class, for example, in another program, I include it “#include utils.p8:1” (only tab 1). This way, the weight of my tests doesn't count on the other program.
I find this an elegant way of managing tested reusable code.
Disclaimer: While this demake does contain an introduction level for each new element, I would highly recommend you play the original game before this! Because the demake is a lot shorter than the original, the difficulty ramps up faster than it probably should.
Controls
Arrows - Move
X/V - Undo
C/Z - Idle
R - Restart level
T - Title screen
Levels
There are 4 "worlds" containing 8 levels each, adding up to 32 levels in total.
Save Data
Your progress will be saved automatically. It will keep track of which levels you've unlocked, which one you were currently on, and your time. You can clear your save data from the pause menu.
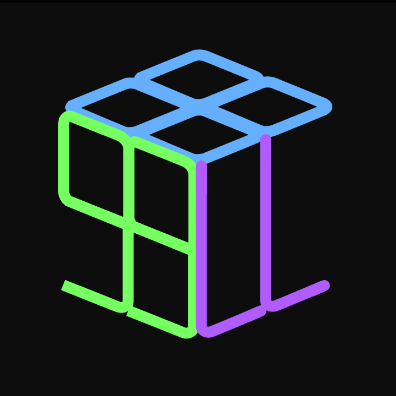


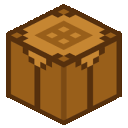
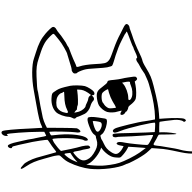
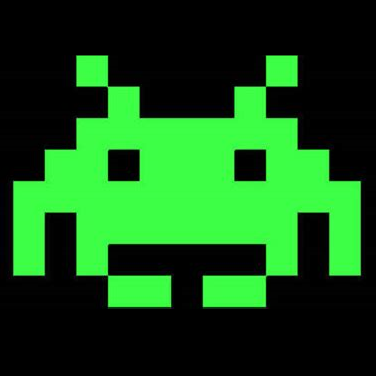
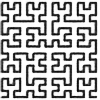
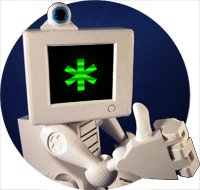
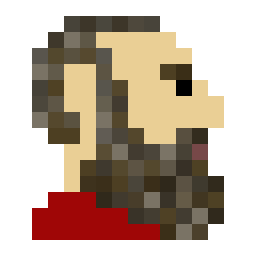
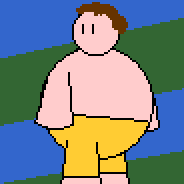
My first game in Picotron !!
Hello there :3
I recently got this workstation and wanted to make something with it.
So why not make a little simple game to start with !!
Controls
For now, you can play only with the mouse. But I think I will add some other control in the future.
Feature
It's a little bit basic for now. But if you install it with load #picotoe
you will be able to personalize the color of the X
and O
symbol. (You can find it in the sprite tab)
However, I want to try some bot
feature. So don't hesitate to follow and keep in touch with future progress.
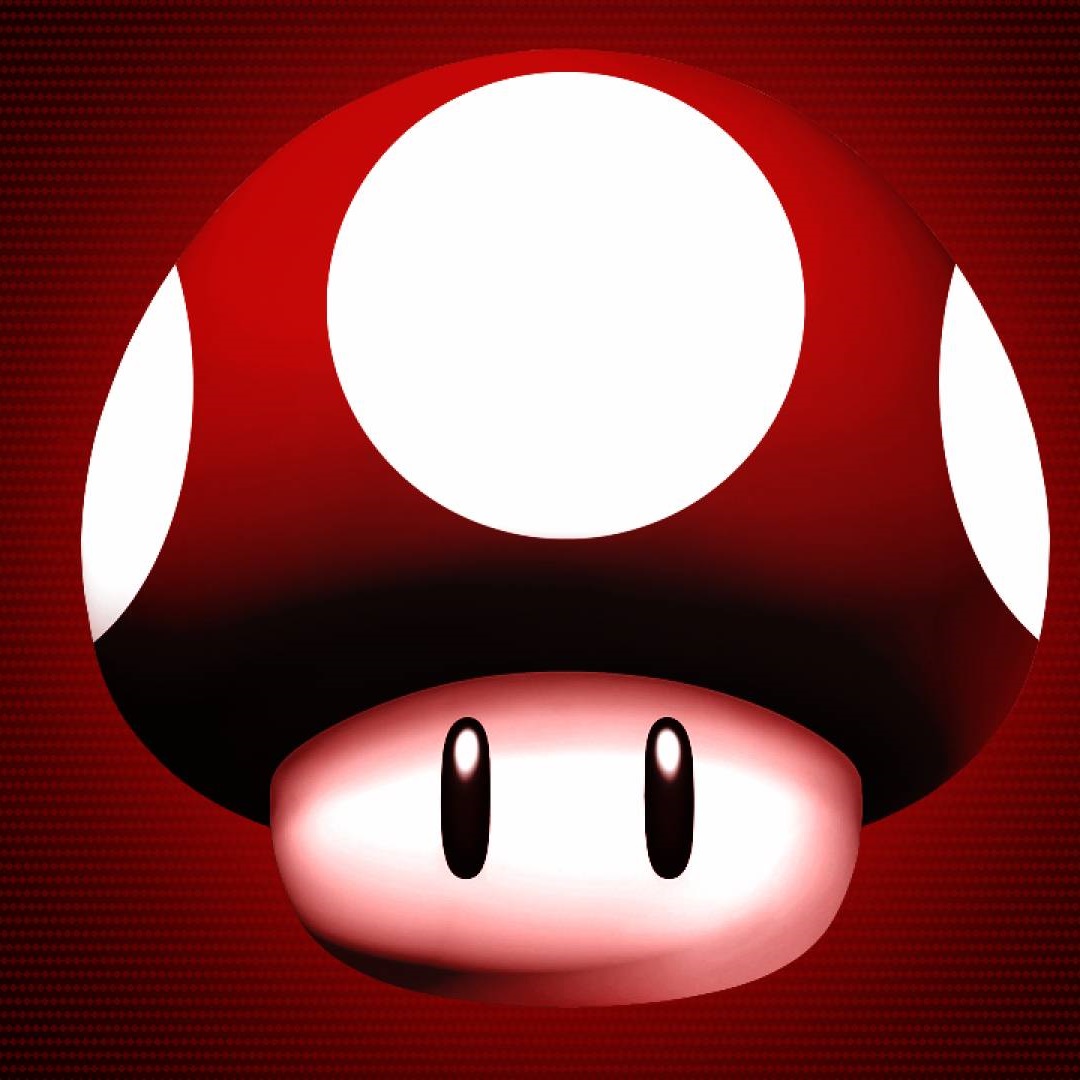
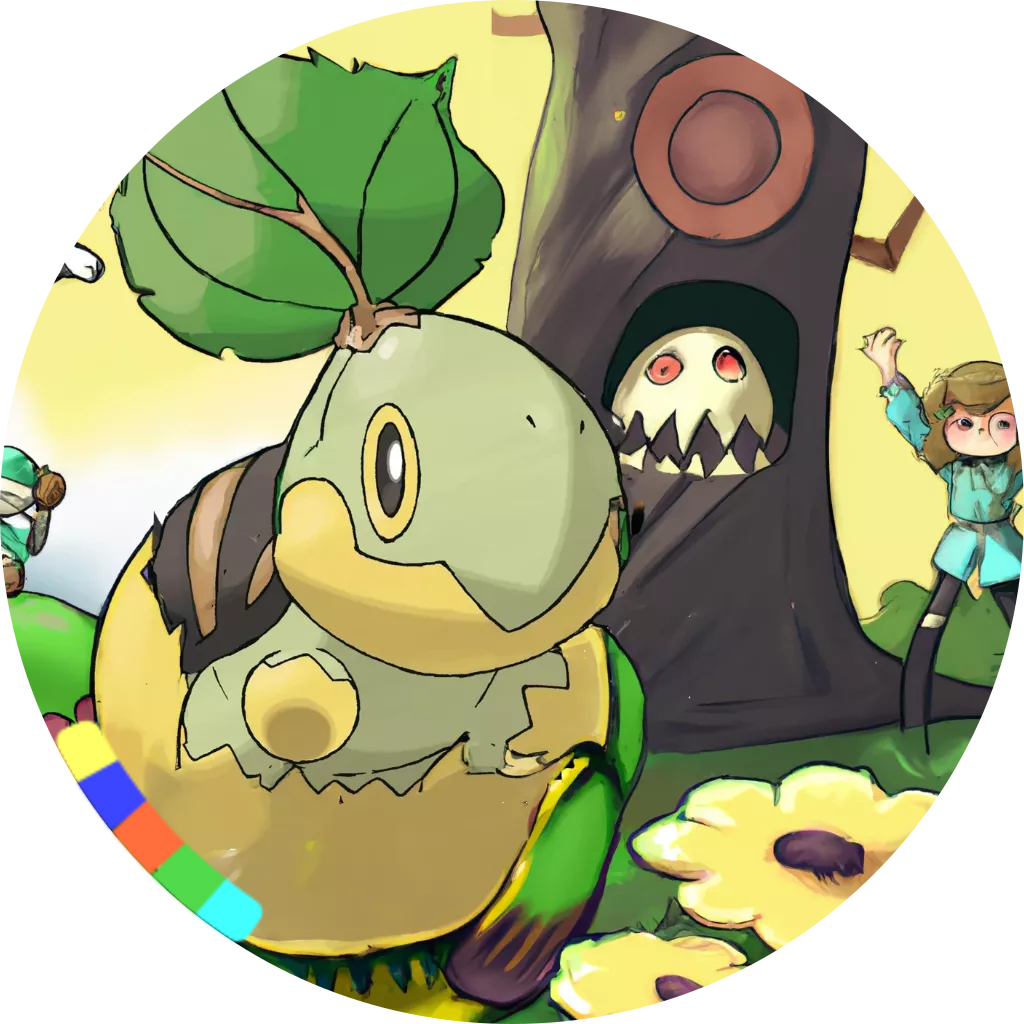
This is a simple transition, that should be fairly easy to use. All the code is in rgb_transition.lua. Simply call rgb_transition_setup()
when starting the transition, and then on each frame, after the game is drawn, call rgb_transition()
. It takes one argument: Whether the transition should be fading in or out. True fades out. In this test cart, press X to reverse the transition.
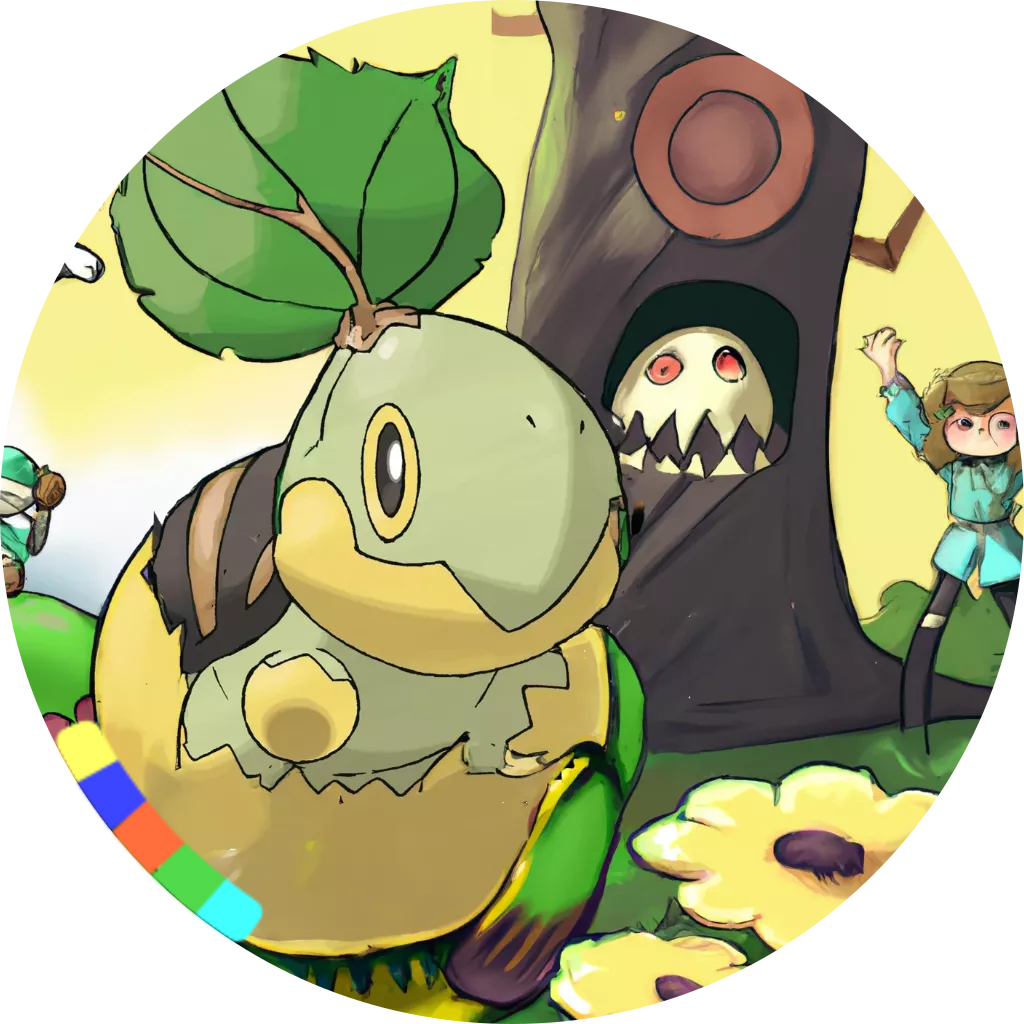