For those learning PICO-8, you may have heard that you can write carts that access the hardware mouse. While the information to do this is not readily apparent, I put together this simple demonstration program with remarks to show how you can do it by reading the position and both buttons of the device.
-- simple introduction to -- using stat(32,33,34) -- for reading the mouse -- by dw817 -- (08-01-19) -- -- poke(24365,1) -- enables keyboard + mouse -- -- stat(32) mouse-x position -- stat(33) mouse-y position -- stat(34) =1 if left button -- stat(34) =2 if right button -- stat(34) =3 both buttons -- -- flip() updates the screen cls() poke(24365,1) -- enable mouse c=7 repeat color(7) -- if right-button pressed, -- change plotting color if stat(34)==2 then c=c+1 if (c==16) c=1 -- if both buttons pressed, -- clear the screen elseif stat(34)==3 then cls() end -- read x+y position of mouse x=stat(32) y=stat(33) -- if left button pressed, -- draw on screen if stat(34)==1 then if ox!=nil then line(ox,oy,x,y,c) end ox=x oy=y else ox=nil end p=pget(x,y) pset(x,y,c) flip() pset(x,y,p) -- loop until press [esc] until forever |

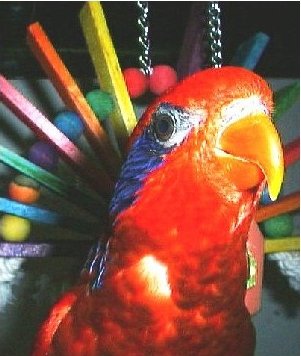
For you new people at PICO-8 and even some of you veterans, you may or may not be aware that you can create a BATCH file to run PICO-8 with very precise settings. Here is the one I use:
CONTENTS OF P8.BAT
@run-pico8.exe -gif_len 120 -windowed 1 -width 692 -height 650 -draw_rect 10,5,672,640 -sound 64 -music 64 -pixel_perfect 0 -software_blit 1 -desktop c:\david\games\pico-8\pico-8_win32\roms\_snaps -home c:\david\games\pico-8\pico-8_win32 |
You can use this as a SHORTCUT too but it is too long, I think a Windows shortcut only allows 64-characters. So if you can, a BATCH file will work just as well.
Modify to your liking and - Hope This Helps !
I built a Raspberry Pi PICO-8 console that uses floppy disks, but SPLORE doesn't work great for browsing a floppy: you have to wait for the preview image of a cart to load before you can scroll past it. For a disk with more than one or two cartridges on it, this is a frustrating experience. Additionally, SPLORE is a little finicky about reloading the floppy contents if you swap out the disk.
To get around this, I boot this cartridge instead of booting into SPLORE, which provides a quicker floppy browsing experience and correctly picks up a new disk if you choose "reset cart" from the menu. It's a pretty rough hack, but I'm sharing it here in case anyone finds any pieces of it useful.
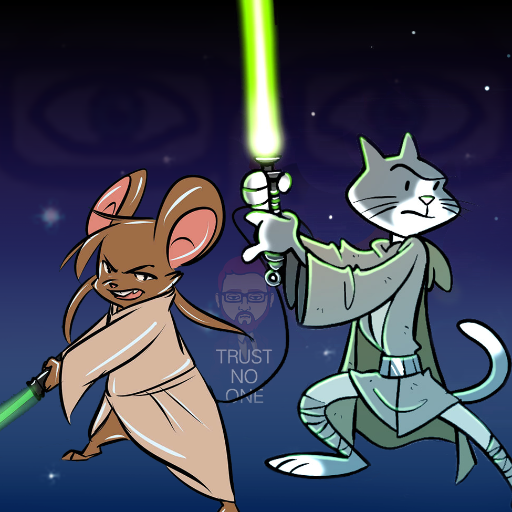
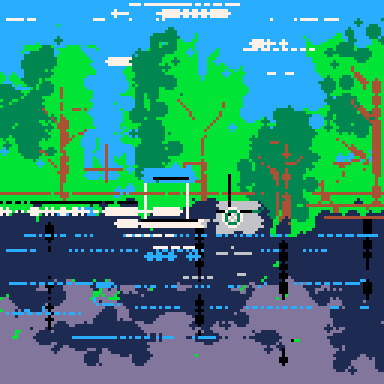
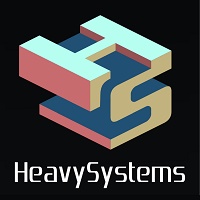
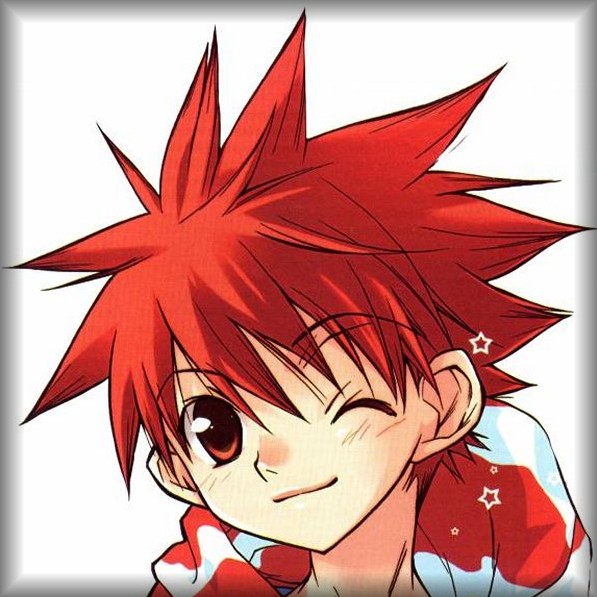
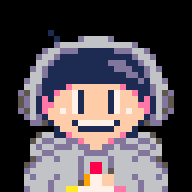

-- 256-colors rehashed -- i think this is as fast as -- i can get the code. -- you will notice that the -- loop only moves a section -- of memory per frame now. mode=0 p=0 cls() function _update60() p=1-p if mode==0 or mode==1 then sfx(0) -- confirm drawing fillp(23130) if(p>0)fillp(-23131) for i=0,15 do for j=0,7 do rectfill(i*8,j*8,i*8+7,j*8+7,i+j*16) end end if mode==0 then memcpy(0,24576,4096) mode=1 elseif mode==1 then memcpy(4096,24576,4096) mode=2 end else if p==0 then memcpy(24576,0,4096) else memcpy(24576,4096,4096) end end end |
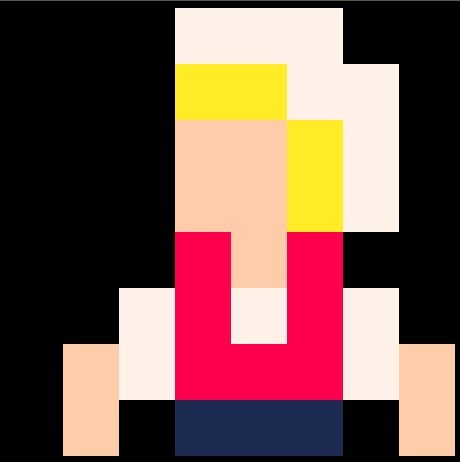
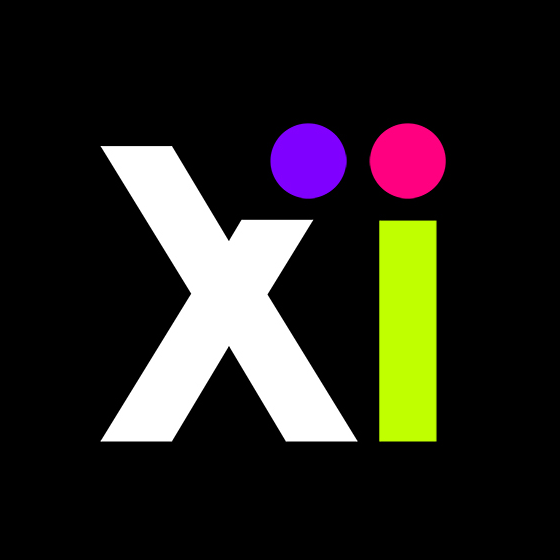
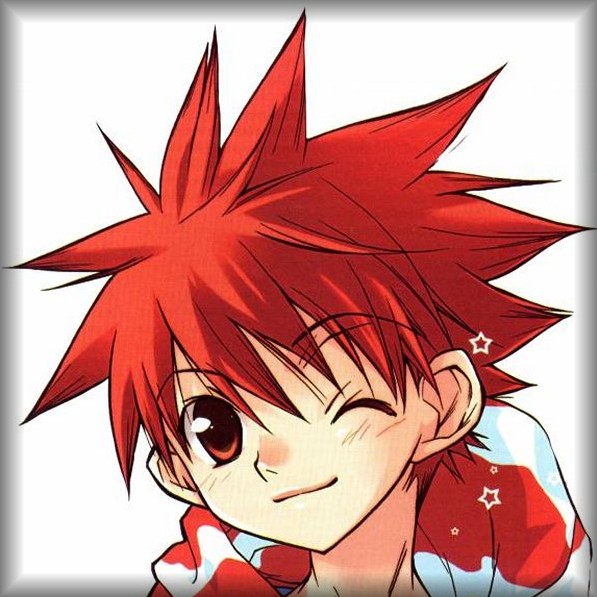


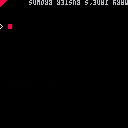
cls() print"mary jane's buster browns" -- 0=using memcpy() -- 1=using standard array kind=1 if kind==0 then memcpy(0,24576,320) color(0) rectfill(0,0,127,5) color(7) sspr(0,0,128,5,0,0,128,5,true,true) reload(0,0,320) sspr(0,0,8,8,0,0,8,8) print("",0,24) stop() end rev={} for i=0,4 do rev[i]={} for j=0,127 do rev[i][j]=pget(j,i) end end for i=0,4 do for j=0,127 do pset(127-j,4-i,rev[i][j]) end end rev=nil spr(0,0) print("",0,24) |
What makes programming interesting is the many different ways in which you can approach a problem.
I'm trying to understand PICO-8's method of NOT seeding a random number, that is, I want to return back to the original seed after I have seeded a number
cls() z=rnd() srand(1) ?"" ?"forced seed" ?rnd() ?rnd() ?"" ?"reset to random seed" srand(z) ?rnd() ?rnd() |
The only way I know how to do it is this. Get a random number to begin with. Seed the number I want, get the values for this forced seed, then return back by seeding with the initial random number. But isn't there a way to reset the SRAND itself ?
In Blitz for instance, you seed (-1) to return it back to normal non-seeded random numbers, but that does not work here.

I'm currently working on a game where you collect items from different areas in a map. I have around 10 spots on the map where I want items to be, but I feel like only 6 or so should actually have items in them when playing as so to create some mediocre replay value. I'm currently detecting the spots using map flags, and sending that data to a function in which I'll create an entity spawner. However I dont know how I can create these exceptions in the code when generating where the items go, does anyone have any hints or ideas for solutions to this problem?
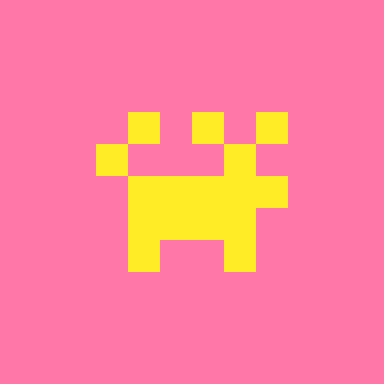
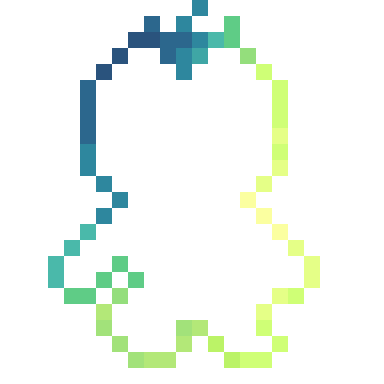
Hello, I am back for a bit, maybe longer, depending on circumstances. Mostly I am programming in BlitzMAX though.
However, I have since come across a program that lets you run LUA in the Pocket Sony Playstation device.
Since PICO-8 is LUA, is it possible to run PICO-8 on PSP then, as an ISO or CSO ?
The emulator PPSSPP which runs PSP flawlessly is for Android, Windows, iOS, macOS, Blackberry, Meego/Harmattan, Symbian, Pandora, and Linux.
https://www.ppsspp.org/downloads.html
One major advantage of running PICO-8 on the PSP is with PPSSPP you can state-save and state-load. That will definitely be helpful to gamers.
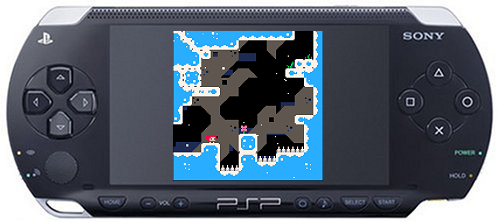
As the resolution of the PSP is 480x272, the screen of 128x128 could be scaled and dithered to 272x272 or 362x272 for a 4x3 proportion.
And it would look great.
So how about it, can it be done ?
Can you do it ?
Controls
Arrow Keys: Move Ozelotl
X: Fire
C: Purge
Fire
Rapid-fire: quick-fireing gun
Power Shell: slow but powerful!
Wide Shot: bullets spread forward
3way Shot: forward and diagonally backward plasma balls
Circular: rotating plasma balls and forward shot
Purge
Detach equipped weapon and detonate for destroy all enemies and bullets.
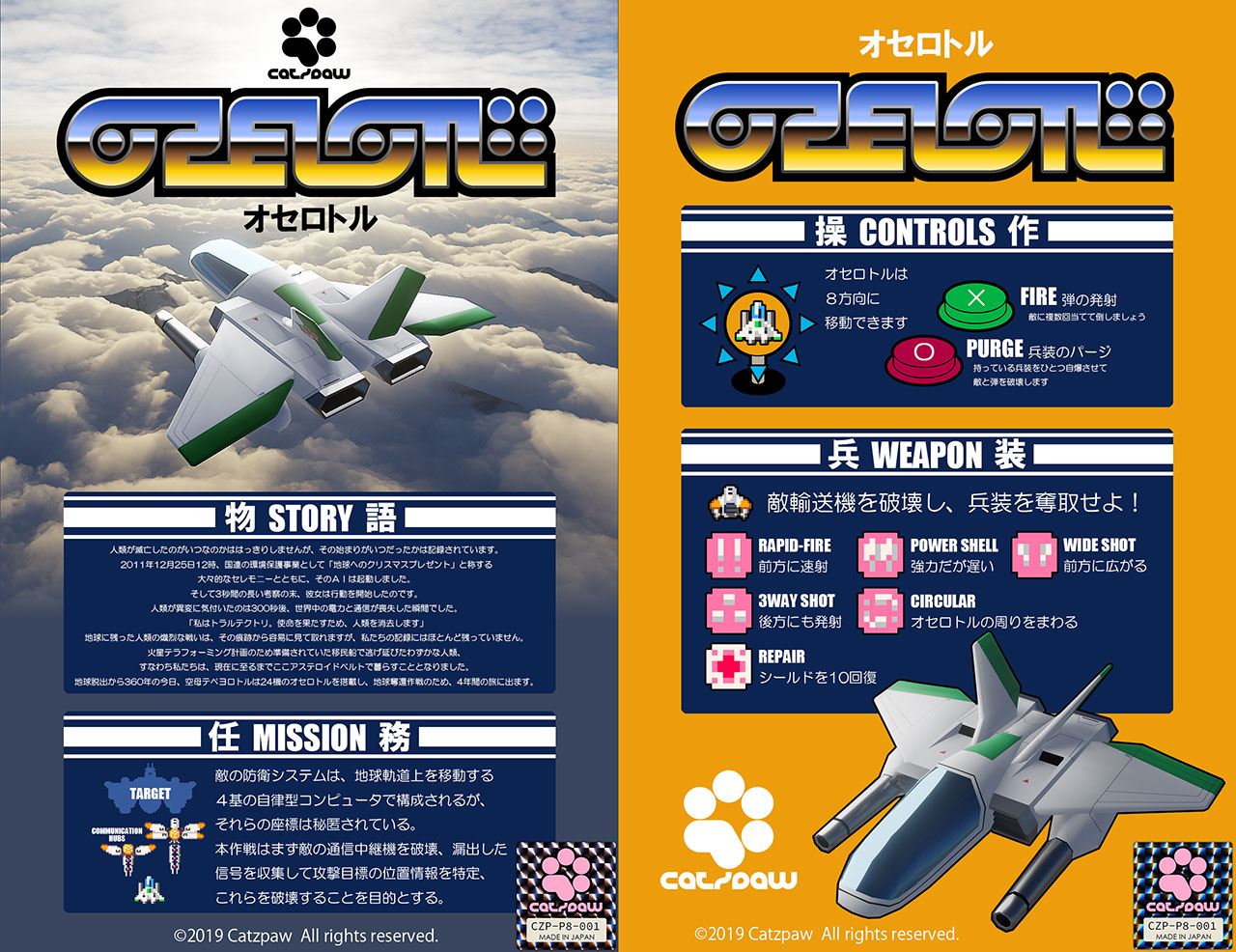

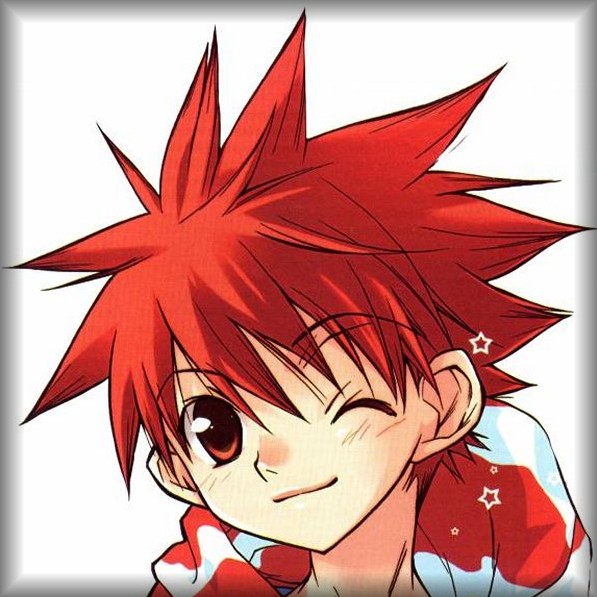

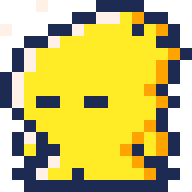


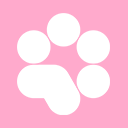
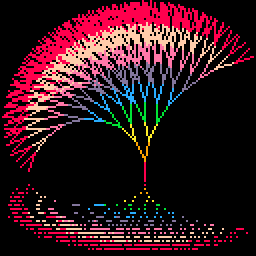

.jpg)
This is a game I used to propose to my wife.
It's a celeste mod (use S and F to cheat the levels and see the bonus Hot Air Balloon level)
I didn't program an option for if she said no...LMAO I figured she just would not of touched the ring.
If you're interested in how this all played out you can see my post on imgur here https://imgur.com/gallery/CuRSO
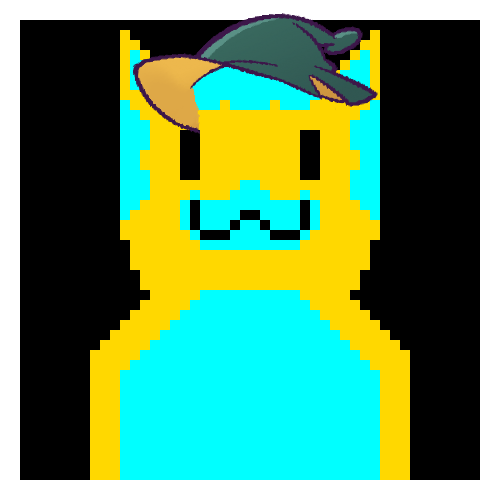
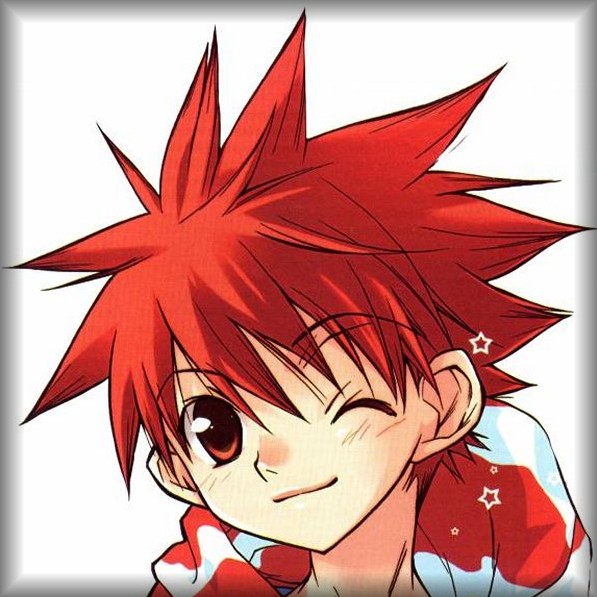


Stix: A Qix demake
Actually more of a remake of Stix for the C64 hence the "varied" colour palette.
My second pico 8 project and, although its a fair bit less ambitious than Boulder Run, I'm pretty happy with the result.
Had my 14 year old Blitz Max code for reference but have enjoyed making everything from scratch. No scanning the web for sound effects and music files this time, just some trig tutorials!
Everything was done in a most enjoyable "sick" week off work!
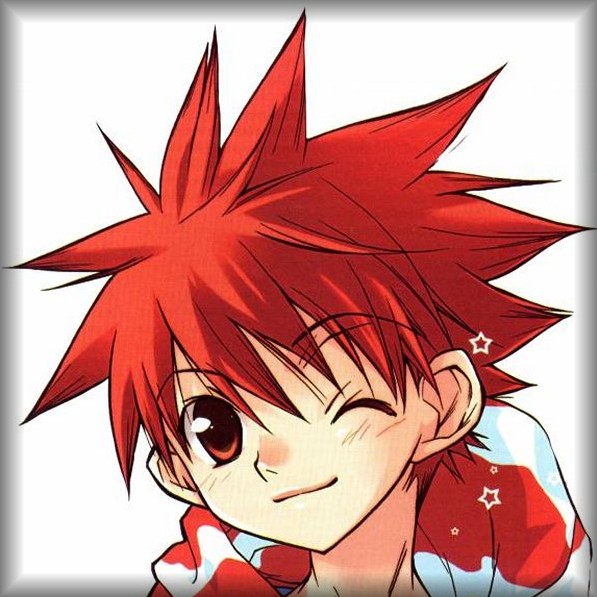
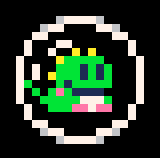

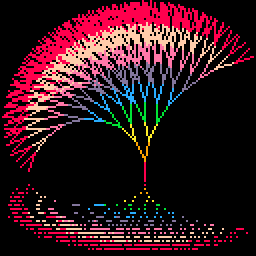
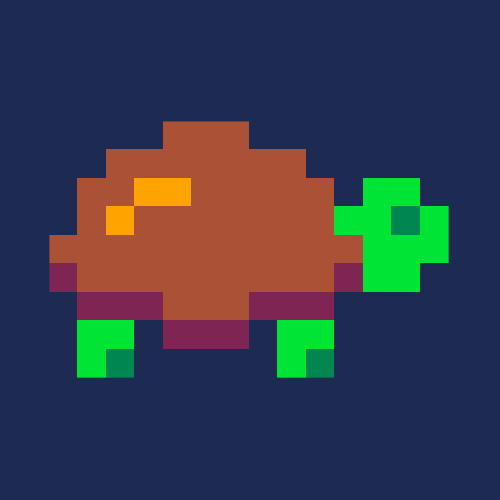

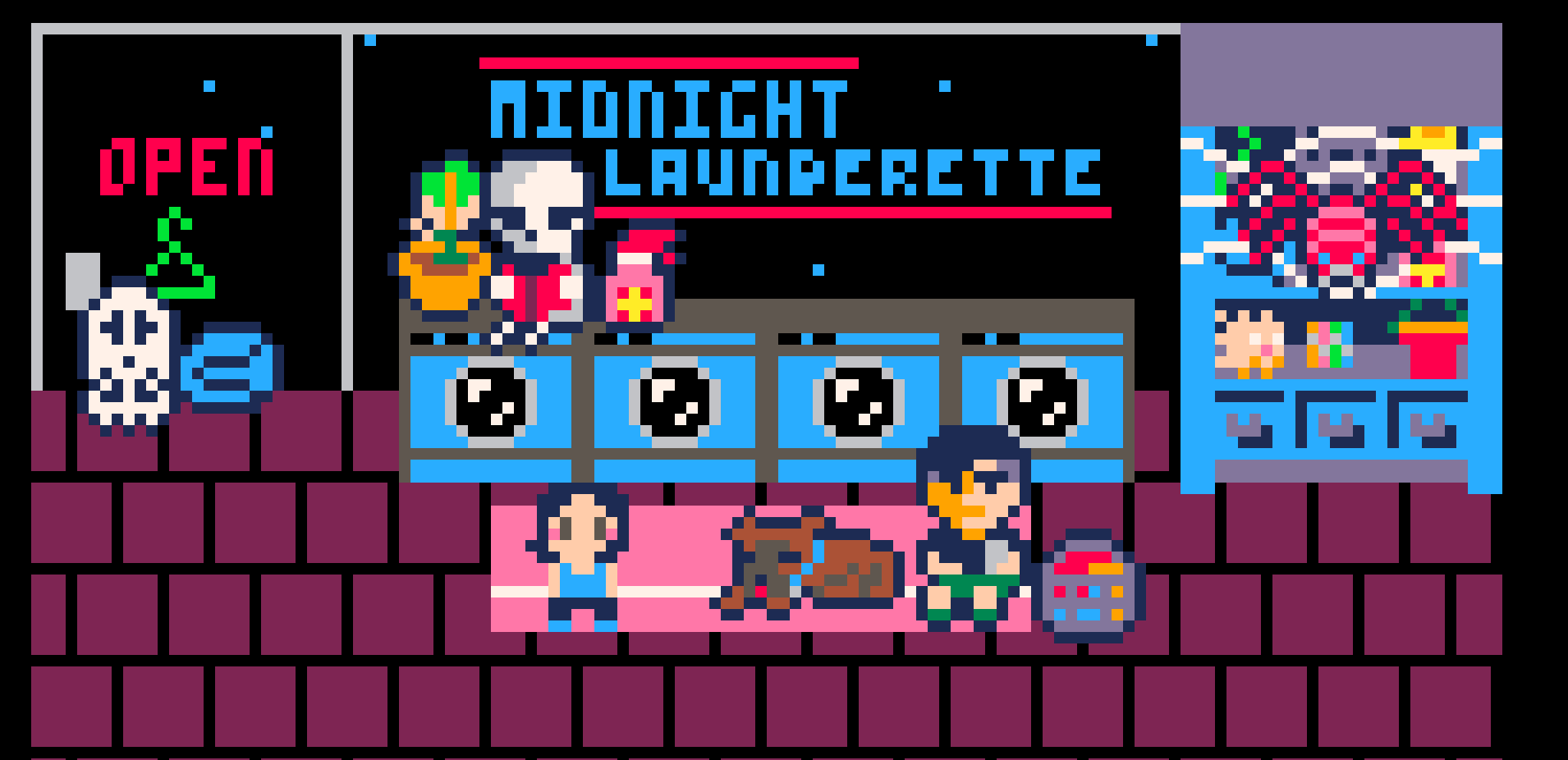
This is my first upload.
I started off with Benjamin_Soule's Chrysopoeia (https://www.lexaloffle.com/bbs/?pid=44647) and just kept editing it until it became this.
@benjamin_soule I'll remove this if you want me to take it down. let me know.
I made it for my wife's birthday. it's based off a "sleepcast" by the app HeadSpace called "Midnight Launderette".
It's one of the free sleepcasts that we use often to help us sleep.
Let me know what you think.

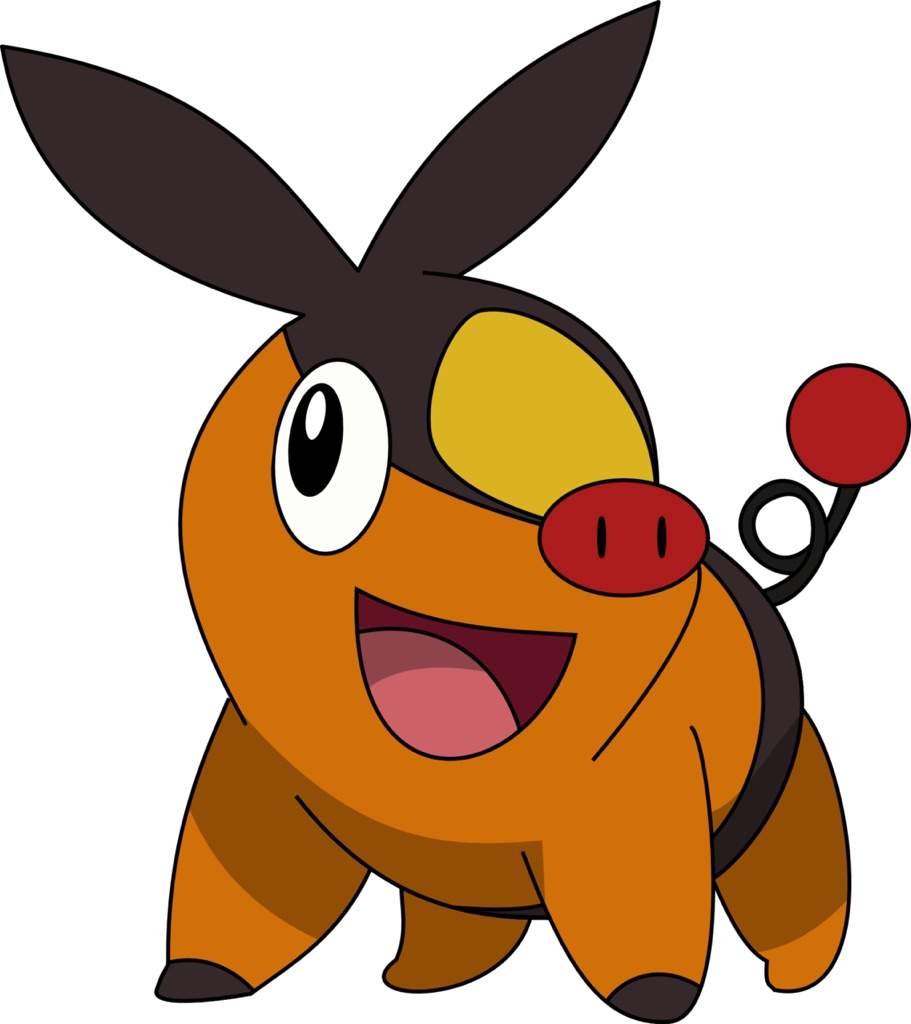
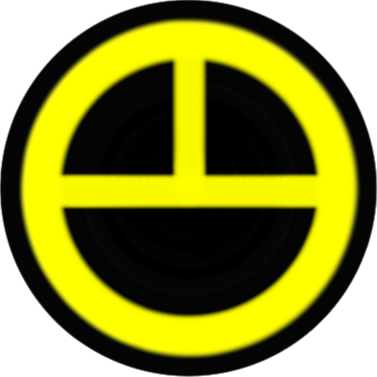
Hello I created a simple wrapper to enable iOS devices to autoplay Pico-8 games.
https://github.com/NinjasCL/pico8-ios-wrapper
The default html export shows a "Play Button". This wrapper can autoplay at full screen.
iOS 9 or newer required.
Cheers!.