increment_second = function(this) this.second = (this.second + 1) % 60 if this.second == 0 then this.minute = (this.minute + 1) % 60 if this.minute == 0 then this.hour = (this.hour + 1) % 24 if this.hour == 0 then this.day = this.day + 1 if this.day > days_in_a_month[this.month] or this.day > 29 and this.month == 2 and this.year % 4 == 0 then this.day = 1 this.month = (this.month % 12) + 1 if this.month == 1 then this.year += 1 end end end end end end |
I'm creating a tamagotchi kit, and since os.clock is no longer part of the API, I figured it'd be okay if the user would have to type his/her current time at every launch.
Currently at 1500 tokens, with a datetime input screen, an "are you sure this is correct?" screen, a class for stats/resources (ie. health, hunger, random_event_countdown, etc...), a class for date calculations, and plenty of overflow control for both classes.
Nearly done, but before I submit it, I'd like to inquire if there's a possible improvement to this eye sore of a function?


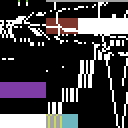
Seems fine to me! If you want to reduce the amount of indenting you can use early returns:
increment_second = function(this) this.second = (this.second + 1) % 60 if (this.second != 0) return this.minute = (this.minute + 1) % 60 if (this.minute != 0) return this.hour = (this.hour + 1) % 24 if (this.hour != 0) return this.day = this.day + 1 if this.day > days_in_a_month[this.month] or this.day > 29 and this.month == 2 and this.year % 4 == 0 then this.day = 1 this.month = (this.month % 12) + 1 if this.month == 1 then this.year += 1 end end end |



Ahhh! Thank you.
I'm a big fan of early returns, so it's a bit strange that this never occurred to me. It will in the future, tho! So that's good... Thanks, haha.


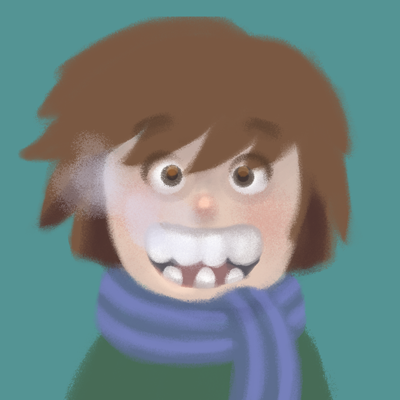
Here is an imbrication-free way and, thanks to inline tests, a shorter one:
function increment_second(this) this.second+=1 if(this.second>=60)this.second=this.second%60 this.minute+=1 if(this.minute>=60)this.minute=this.minute%60 this.hour+=1 if(this.hour>=24)this.hour=this.hour%24 this.day+=1 if(this.day>getdaysinmonth(this))this.day=this.day%getdaysinmonth(this) this.month+=1 if(this.month>12)this.month=this.month%12 this.year+=1 end |
Note that it needs a getdaysinmonth function, which returns how many days are in this.month (based on this.year obviously). Functions are better for this than locally managing this, as you may need it elsewhere.
And just for nitpicking, a leap year is not just a multiple of 4, it also must not be a multiple of 100 unless it is a multiple of 400 (wikipedia). That's right, 2100 is not a leap year. What would your players then think of that bug? :D
Hopefully you'll have and call isleapyear(this.year) function ;).



Yep, don't think it can get any better without changing the architecture. Thanks ElGregos!


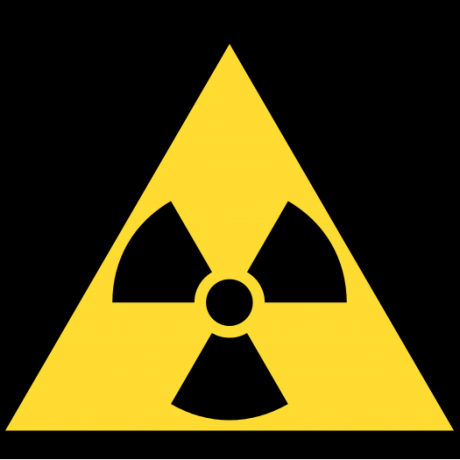
What if you didn't need any conditionals...
function tick() t.second += 1 t.minute += flr(t.second/60) t.second %= 60 t.hour += flr(t.minute/60) t.minute %= 60 -- etc end |
[Please log in to post a comment]