Is it possible to fill in vector graphics?
Is there a way to fill in something like this bird shape?
It is currently drawn using line().



Once you've drawn it you can pick a random point inside the shape and do a flood fill.
Here's an explanation of the flood fill algorithm if you're not familiar with it: https://www.youtube.com/watch?v=ldqAmkdthHY


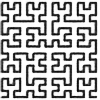
User scgrn wrote a function that does exactly what you want.
https://www.lexaloffle.com/bbs/?tid=28312


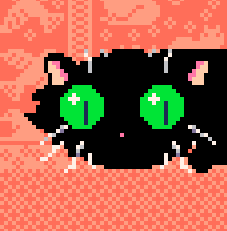
@RealShadowCaster that is exactly what I was looking for. Thanks
@jasondelaat Isn't floodfill too slow? I haven't tried it, so I am not sure, but it sounds like I would need a lot of pset operations each frame.


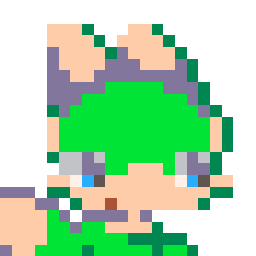
I made VECTOR_FILL for reference.
This code is not intended to be so efficient in logic.
If the drawing coordinates are out of range or the figure is large, it will be out of memory.
function fillvector(x,y,p) local d={1,0,-1,0,1} local i=2 while pget(x,y)~=p do pset(x,y,p) while d[i] do fillvector(x+d[i-1],y+d[i],p) i+=1 end end end |



you are right - floodfill is rarely what you want (terribly slow).
you should:
- split your shape into triangles
- use p01 (or shiftallow) trifill from https://www.lexaloffle.com/bbs/?tid=31478



"Isn't floodfill too slow?"
Oh yeah, for sure. I mean it depends on what you want I guess. If you're just drawing the image statically on screen and nothing else then it doesn't really matter but if you're animating or resizing it or whatever then yeah, floodfill is not your friend. I thought you were just looking for any way to fill it so I went with simple over efficient.


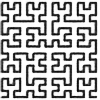
@jasondelaat, I for once like your fillvector, especially how the 4 2D unit vectors are stored in a single array 1D array.
I'm wondering though, why
while pget(x,y)~=p do
instead of
if pget(x,y)~=p then
?



@RealShadowCaster, that's actually @shiftalow's code not mine. I agree though, seems like it should be if
instead of while
since the loop will run at most once anyway.


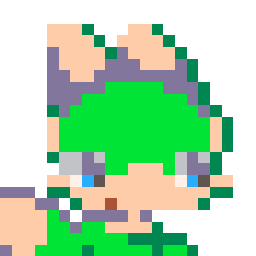
Judging from the content, is this probably a reply to me?
Thank you.
2D reference of 1D array is an interesting method, but it doesn't seem to be very efficient here.
Also, there is no deep meaning behind the pget() check in while. (It's fine to use if
)
And, since FILLVECTOR
is probably commonly called FLOODFILL
, I'll post the correction below.
Fixed version
function floodfill(x,y,p) if pget(x,y)~=p then pset(x,y,p) floodfill(x+1,y,p) floodfill(x,y+1,p) floodfill(x-1,y,p) floodfill(x,y-1,p) end end |
Even faster (more complicated) version
[Please log in to post a comment]