there's poke() and poke4(), where's poke2()?
I'm tinkering with sfx mem right now, and the notes are 16 bits...
I know, I'm never happy ;)
--do not use this! the proper version is by Felice below function poke2(a,w) poke(a,w) poke(a+1,w/256) end function peek2(a) return peek(a)+peek(a+1)*256 end |



A couple of notes:
As mentioned in my post earlier, you should multiply by 0x.01, rather than divide by 256, because if your value were 0xffff.ff80 or more, you'd end up poking 0 for the hi byte. Uncommon case, sure, but might as well do it the safe way if it's the same cost, which it is.
Also, you can do peek2() slightly more simply using peek4() shifted left 16.
function poke2(a,w) poke(a,w) poke(a+1,w*0x.01) end function peek2(a) return peek4(a)/0x.0001 end |
Also, less useful, but you can do the fraction instead like this:
function poke2f(a,w) poke(a,w/0x.01) poke(a+1,w/0x.0001) end function peek2f(a) return band(peek4(a),0x.ffff) end |
This is all assuming @zep doesn't cave and give us peek2/poke2. ;) wink, wink, nudge, nudge, @zep?



wow. I had read your other post (I even starred it) and then I'm pasting a snippet featuring the exact same problem! what can I say, I'm a fraud ;)
that's out of laziness btw, the bitwise functions are painful to use and w/256 "looks" more like w>>8 than shr(w,8). can't we have lua 5.3?



I know, right? Ah well, it's not like we paid very much for PICO-8 vs. the fun we get out of it. I have to keep reminding myself of this as I constantly restrain myself from demanding more and more of zep. :)


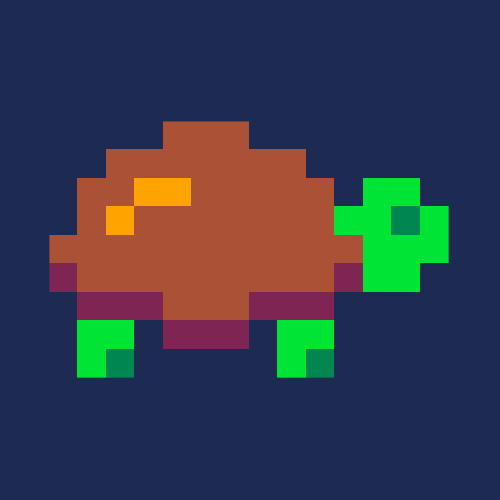
This post is in response to this thread but also several other threads I've seen recently that seem to be asking for "extra" apis such as physics etc. etc.
Maybe it is because I'm oldschool and grew up on BASIC, but I really don't want zep to start adding a bunch of easier apis on top of Pico-8. That'd defeat the fun of it for me. I LIKE that I have to roll my own for tons of things. If I wanted to make a game without writing much code, I'd just use Gamemaker or something like that. :) I hope zep stays true to what he said that Pico-8 will be feature complete and remain an "eternal" product. I LOVE that. So much.
edit Though now I understand the OP a little better, that there's no poke2 between poke and poke4. I didn't even know there was a poke4.


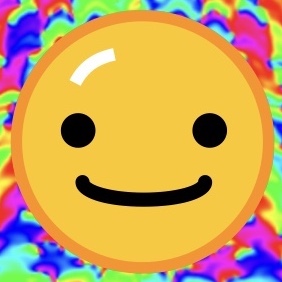
I was thinking that what’s really missing is a library/import system. That way core pico8 is still simple and stripped down but bringing in a more complex library is still possible through maybe some cart swap mechanism. Personally, I’m looking for more math operations like python’s math.py
But poke2 would definitely be useful and might be worth adding to the core.



@notb you can already swap carts and copy and load chunks of memory from one cart or save them to another.
As long as you don't mind your game being required to not run on the bbs.



@Felice would you mind clearing something up for me?
In you post you mention just using peeking 4 and then shifting left 16 bits. Am I wrong in thinking you should be shifting right instead? Maybe my brain is doing something wrong. Also you proceed to instead divide by 0x0001 which i assume is equivalent to the left shift you mention but could you explain how that works for me? I'm pretty hand with bit functions when it comes to shifting, masking, compressing, encoding, etc... But I don't really understand multiplication/division and how that effects binary numbers in terms of bitshifting.



The peek4() function reads values from memory in little-endian mode. The first byte in memory is the bottom 8 bits of the 16.16 fixed-point PICO-8 value. The second is the next 8 bits up. So if you say v=peek4(addr)
, the first two bytes at addr
end up defining the .16 part (the fractional part) of v
. However, I think if people say v=peek2(addr)
, they want v
to be an integer, not a fraction, so I shift left by 16 to move the fraction into the integer part.
The reason why I use 0x.0001 to shift by 16 bits left or right is that you can't multiply or divide by 65536 (2¹⁶) when PICO-8's number system is limited to -32768..32767. So, to effectively multiply by 65536, I instead divide by 1/65536, which is functionally the same, except I can actually represent 1/65536 in the PICO-8 number system, as 0x.0001 in hex.
Note the decimal point, by the way. Dividing by 0x0001 would be pointless. :)



Thanks for the explanation, yeah I typo'd and missed the decimal point. xD



This lib I wrote for passing decimal ints with arbitrary bit counts into GPIO memory, could maybe be extended to support reading/writing any slot in memory, not just GPIO. https://www.lexaloffle.com/bbs/?tid=31098
Granted it only really is intended to work with treating each bit as a binary byte (0 or 255) so might not fit the use case as well.
[Please log in to post a comment]