Inspired by LRP's lowercase font, I made a little library that lets you print to the screen using a custom 9px variable-width font that is defined entirely in code. In other words, nice and readable text that doesn't use sprites. The cartridge includes the hastily coded example usage you see above.
What's included:
init_print9 initializes font data, must be run before print9() can be used print9 str [x] [y] [col] prints to the screen using a variable-width 9px custom font if x or y are left blank it will continue printing where it last left off col is text color optionally returns the x-coordinate of the cursor, which is useful if you want to continue where you last left off (for example, when typing one character every frame) do note that print9() uses up a reasonable amount of cpu, typing out the entire lowercase alphabet uses up about 10% of the cpu @ 30fps my suggestion for longer text (i.e. dialogue boxes) is to draw to regions of the screen that are not cleared every frame and only clear when necessary is_bit_set var pos checks if the pos-th bit (including fractional part) in var is set or not used internally by print9(), but hey, it's a nice utility |
With all (non-extended) characters defined, this library uses up 847 tokens. If you are desperate for tokens, it works perfectly fine to remove the definitions for characters that you don't need, for example only defining lowercase characters + punctuation can cut it down to ~450 tokens.
Explanation for how the characters are defined:
[hidden] The font data for each character are tucked away in regular Pico-8 numbers treated as bitfields. The bitfield is divided into two parts - an 11-bit header and a variable length body used as a binary bitmap. Here's what the data for the letter "e" looks like on a binary level: |


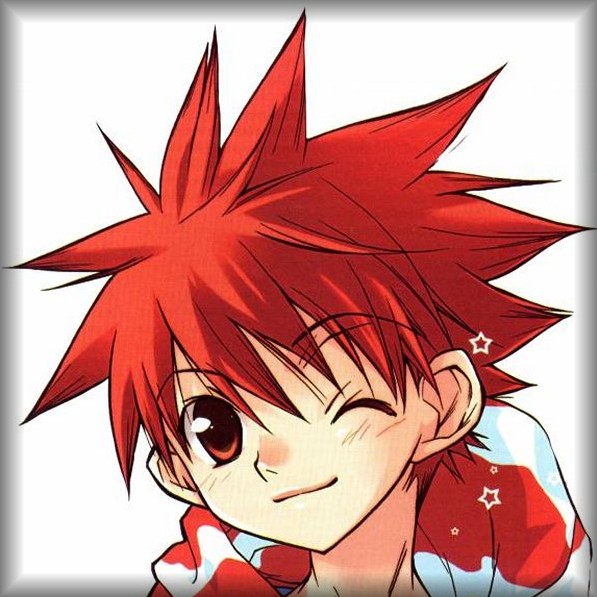
This would be perfect for some great text adventure games.
Nice going, Qbicfeet !


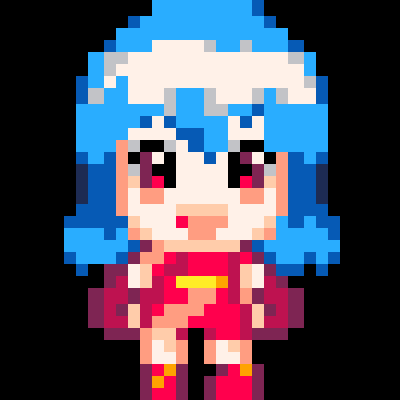
I haven't peeked under the hood, can we design fonts for it? Is it all done with draw commands?



HotSoup: Each character is drawn with pset, one pixel at a time. The data for each character is stored as a number value that is treated as a bitmap. There's a little more explanation at the top of the other thread, although qbicfeet has extended that method to support much larger characters.
The other thread also has a Font Maker cart for converting your own characters from the sprite sheet to numeric bitmaps (although it's limited to the 4x8 size that I originally started with).



@HotSoup: Yes, you can design your own fonts and like LRP said, it's all done using pset. I added an explanation for the font data in the opening post, hope it's descriptive enough.
If there's interest I could make a small tool that generates the character data characters using sprites as input. Is that something anyone would be interested in?


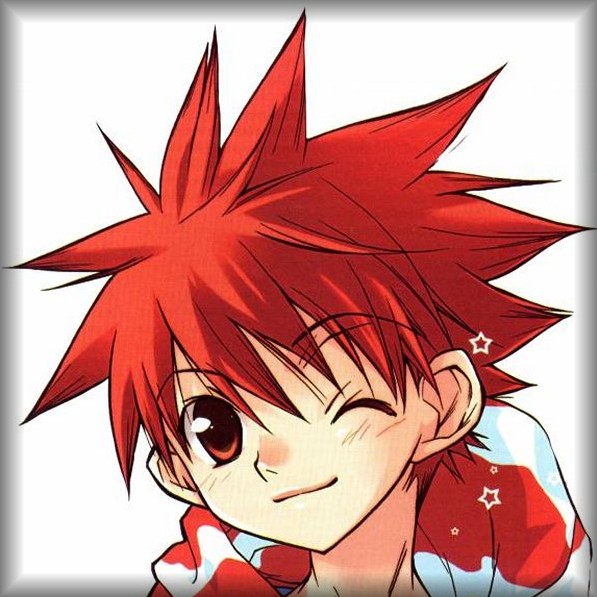
QBicfeet, if you do this, you won't need to save the character length in pixels. If you left-justify your font, all you have to do is scan each 8x8 element from right to left (1-8) and record the length in an array for that character once you hit a non-black pixel.
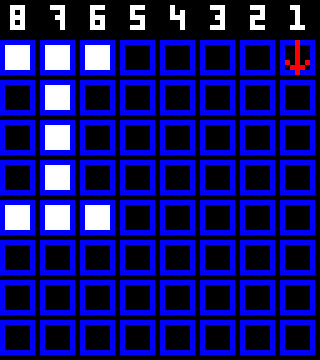
The advantage is, ANYONE can build their own font with your program by changing only the image table and not have to worry at all about manually entering the pixel-length across for each character. :)



I'm not entirely sure what you mean, how would the data be structured code-wise, then? Wouldn't using a static 8x8-size field (if that's what you mean) use up a lot more tokens?


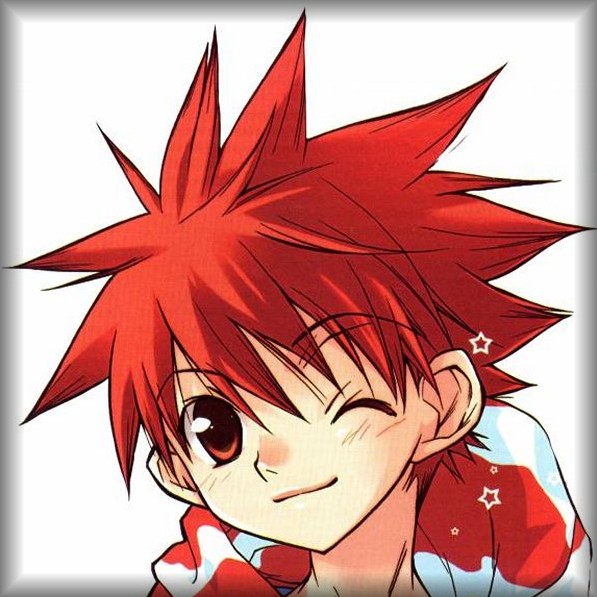
I could write the code, but I don't want to take your fireworks because you've done so much in here already. :)
I'll doodle a little of it.
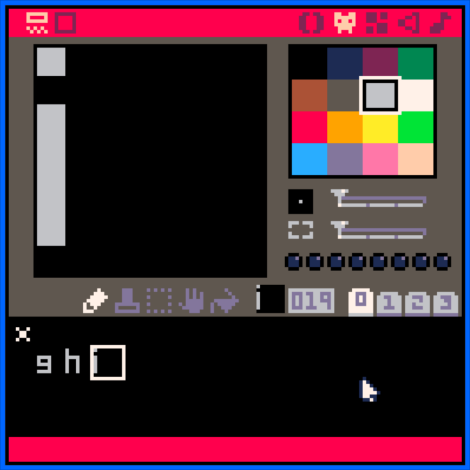
Notice how each of these 3-letters in the tiles are left-justified ? There are 3-letters in this example.
Letter "g" is 4-pixels across. Letter "h" is 4-pixels across, and watch out now ! Letter "i" is 1-pixel across.
What you want to do is scan the 8x8 tile area for each character, from the rightmost pixel to the left and STOP when you hit a pixel that is not black.
That then is the recorded horizontal length for that character - it is right where you found a non-black pixel.
Save that to your character array. So to start, in the tile area, doodle up say 96-characters from SPACE to Exclamation Mark to Question Mark to Uppercase Letters to Square Bracket to Lowercase Letters to Tilde, and you are all set !
Then anyone can easily load up your cart, change the tile table to their liking for a custom set, or use the one you've drawn yourself, and then run your program to display perfectly proportional text, as you have already done so in your code.
BUT they won't need to manually type out in a table as you have earlier done the length for each character as your clever code will already scan each character's size and do this for them. Do you see ?



Ooh, I see what you mean now, I got a bit confused there and didn't get that you were talking about a font builder (thought you meant using it as a new font data structure in the main program).
That's a really great idea! I could definitely do something like that, it would be really intuitive and make it easy to edit and share new fonts. Thanks for the tip!


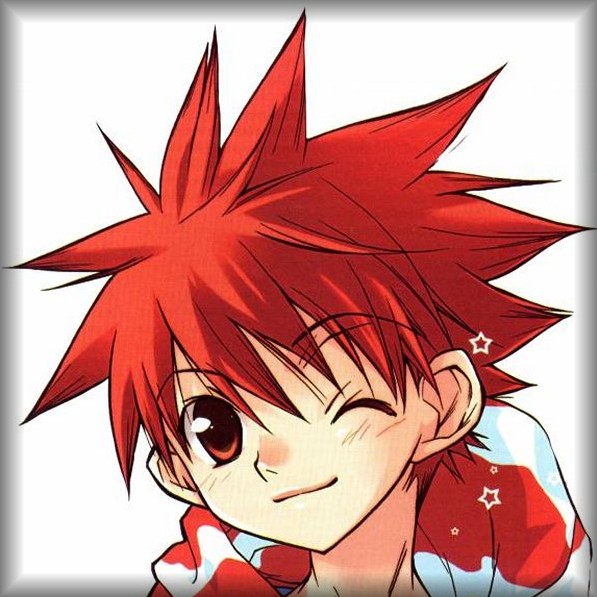
Glad to help and and Good luck ! I guarantee someone will use it. =ME= for instance ! :)
Especially if and when a QWERTY keyboard input is added. Your proportional font will look great for some devilishly puzzling text adventures I've written in my time.
"Professor Twist's Tome"
"Idol Of The Nile"
"Dracula's Castle"
"Orbs Of Ankhar"
"You are at the intersection. A bridge crosses a stream to the North and a twisted path runs to the East."
"You see: Tree Branch, Gold Coin."
"Exits are North, East, and South."
"Lift Grate"
"Climb Tree"
"Open Drawer"
"Get Key"


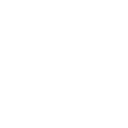
Broken now.
But can be fixed by replacing the "shl" in "is_bit_set" with "rotl"
[Please log in to post a comment]