This Lua script is designed to copy files from a directory in /appdata/system/lib to the corresponding destination in /system/lib. The key functionality of this script allows the user to modify the system libraries (e.g., gui_ed.lua in this case) in /appdata/system/lib and have those modified versions persist even after a reboot.
Key Features:
Directory Syncing:
The script checks for files in the /appdata/system/lib directory.
It copies each file to the matching location in /system/lib based on the filenames. For example, if gui_ed.lua is modified in /appdata/system/lib, it will be copied to /system/lib/gui_ed.lua replacing the original.
Persistence of Edits:
Any changes made to files in /appdata/system/lib will persist after reboot, ensuring that the customized libraries remain intact and are used when the system restarts.
Works with Any /system Directory:
The script can be adapted to copy files from any directory within /appdata/system to the equivalent directory in /system, not just /lib. It can be used to update any files in the /system/ directories by making minor changes.
Usage:
Modifying Default Libraries: For example, you can modify the gui_ed.lua file in /appdata/system/lib and, when the script is run, it will copy this modified file to /system/lib/gui_ed.lua. This ensures that your changes are preserved across reboots and will be loaded at startup.
-- Function to check if a file exists in a directory local function exists(path) -- Handle the case where path is just a file name (without a directory) local dir = "" local file_name = path if path:find("/") then for i = #path, 1, -1 do if sub(path, i, i) == "/" then dir = sub(path, 1, i - 1) file_name = sub(path, i + 1) break end end else -- If no directory, treat the whole path as the file name and current directory as default dir = "." end -- List files in the directory local files_in_dir = ls(dir) -- Check if the file exists in the directory for _, file in ipairs(files_in_dir) do if file == file_name then return true end end return false end -- Function to replace a file in the target directory local function replace(source_file, target_dir, source_dir) if source_file == nil or source_file == "" then return -- Return early if the source file is nil or empty end local target_file = target_dir .. source_file -- Only remove the file from the target directory if it already exists in the source directory if exists(source_dir .. "/" .. source_file) then if exists(target_file) then rm(target_file) --print("removing: " .. target_file) end end -- Copy the file from the source to the target directory cp(source_dir .. "/" .. source_file, target_file) --print("copy: " .. source_file) end -- Function to clone a directory local function clone_directory(source_dir, target_dir) local files_in_source = ls(source_dir) -- Replace each file in the target directory for _, source_file in ipairs(files_in_source) do replace(source_file, target_dir, source_dir) end end -- Example usage -- feel free to add other directory here local source_directory = "/appdata/system/lib" local destination_directory = "/system/lib/" clone_directory(source_directory, destination_directory) -- Example adding more directory to the update list: --local source_directory = "/appdata/system/apps" --local destination_directory = "/system/apps/" --clone_directory(source_directory, destination_directory) |
Update Other Directories: The script is easily extendable to work with other directories in /appdata/system/, ensuring that other parts of the system, such as configuration files or additional libraries, can also be edited and preserved.
Put the content of the main.lua inside the cart into /appdata/system/startup.lua for it to work on boot.


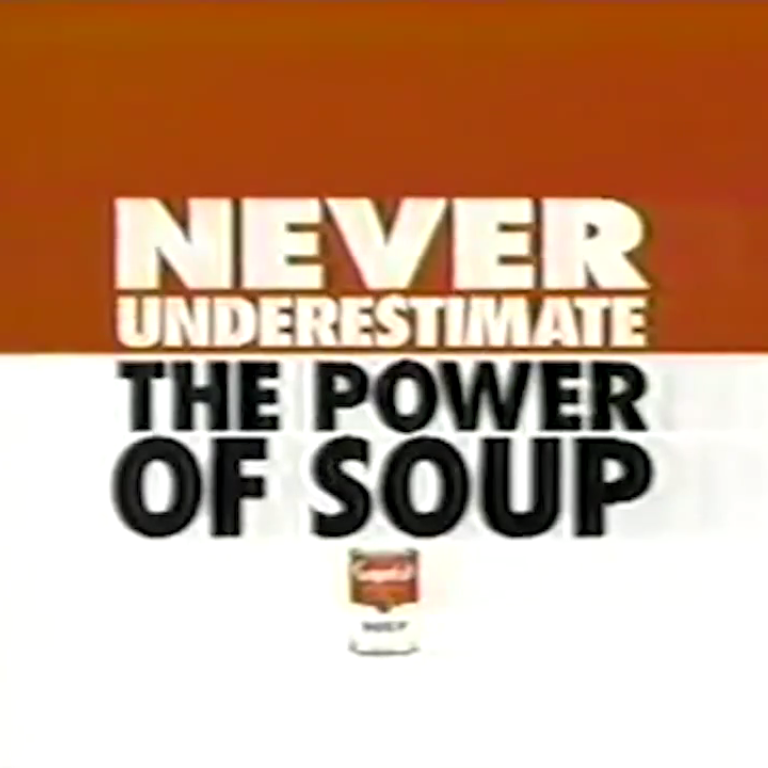
If you don't mind some suggestions to simplify the code:
For exists(), you can do fstat(). It will return "file", "folder", or nil (if a file doesn't exist)
cp() will overwrite a file if it already exists, so you don't actually need to check for or remove the target file.
You can simplify clone_directory down to
local function clone_directory(source_dir, target_dir) local files_in_source = ls(source_dir) -- Replace each file in the target directory for _, source_file in ipairs(files_in_source) do cp(source_dir .. "/" .. source_file, target_dir .. source_file) end end |
[Please log in to post a comment]