I’m trying to print text centred around a point.
At the moment my code offsets the x coordinate by half the width
of a string in pixels, calculated as (4* #s)-1
.
This works fine for
alphanumeric text but is wrong when the text contains glyphs.
Is there a built in function to help achieve this?
Or do I need to examine every character when computing the pixel width of the text?


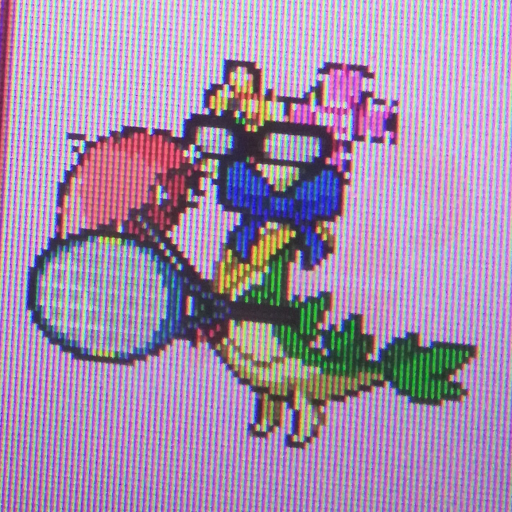
Unfortunately, you pretty much have to watch out for those double-wide glyphs.
I'd suggest either minimizing your use of glyphs or making a function that returns the proper width of a string.
Of course, a more ridiculous hack way could be something like this:
function center_text(string, y, c) local x = 64 - (#string * 2) if(c)color(c) for i = 1, #string do print(sub(string, i, i), x, y) x += 4 end end function _draw() cls(7) color(8) center_text("😐 hello, world! 😐 ", 60) -- Note the extra space after each glyph end |
I put this together in a minute, so it might not be the best.



Cunning!
I ended up taking the width of glyphs into account like this:
-- centered printing -- width of a printed string function printw(s) if #s == 0 then return 0 end w = 0 for i = 1, #s do if sub(s,i,i) >= "\x80" then w += 7 else w += 3 end end return w + #s - 1 end -- print centered function printc(s, x, y) print(s, x - printw(s)/2, y) end |
And that's working well
[Please log in to post a comment]