changelog says that time() has been removed
but I can still use it?
though it does seem to behave a bit oddly by eventually returning negative time?
any ideas?


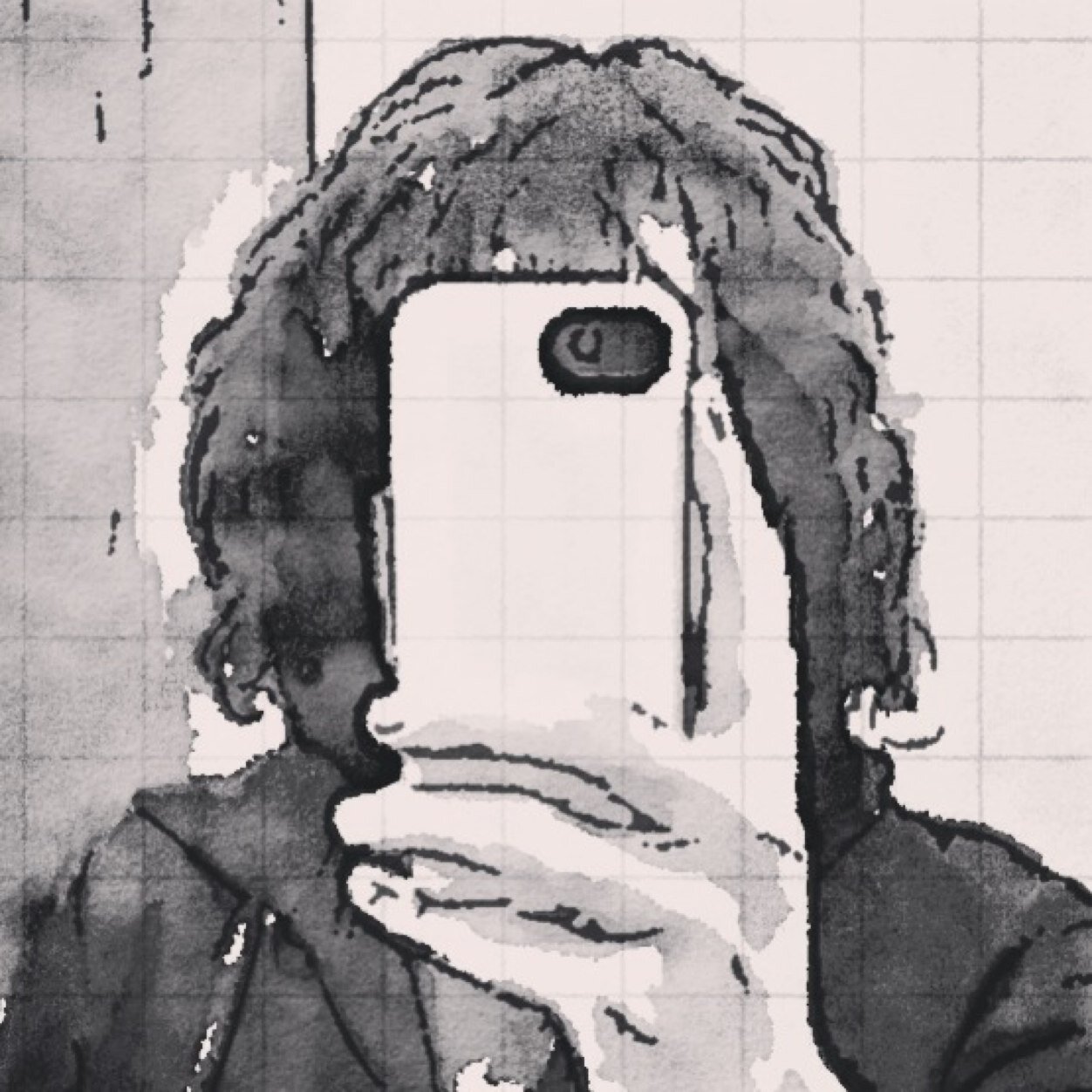
It still works as of 0.1.6. But since Pico-8 numbers only go up to 32767, it's going to roll around to a negative number after the console has been running for a little over 9 hours, I think. You could write a wrapper around time() to handle this.



thanks for the info.
i see negative numbers much sooner than that.
anyway, i ended up rolling my own tick counter


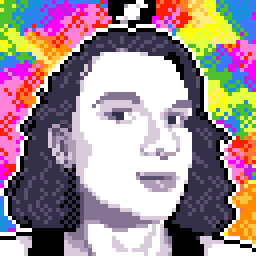
Yes it's actually a tick counter itself I think. So it should roll around to a negative after 32767 miliseconds. Which are almost 33 seconds. Which isn't that great! But handling it yourself is definitely a good way to go!!


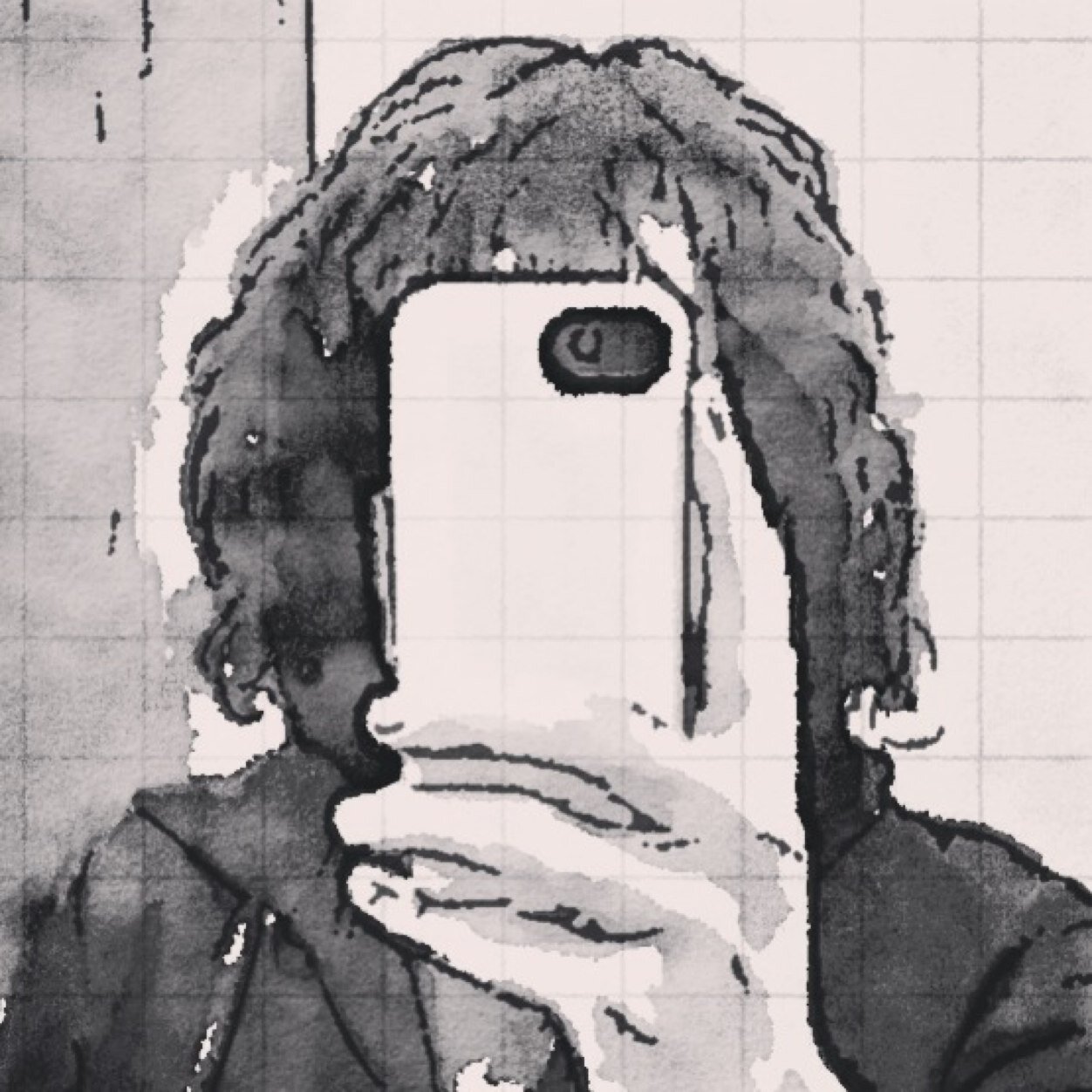
time() isn't a tick counter -- do print(time()) in the console and you can see it prints seconds from console start. (On my system, at least.)


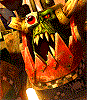
From what I understand, time() isn't going to be your best bet for a lot of things because of that stupid negative number issue. Instead, you can do a number of make-shift things to effectively create your own estimation that's more suited to your game's particular needs.
For instance, there is a game type where the player gains incremental income based on purchased point-earning items. The player receives point income per second based on how many they own and the point value associated with them. My included cart shows how one can use the _update function as a counter and correctly count up to a second. Since _update gets called 30 times per second, incrementing a global value to 30, performing the point increment, and returning the global variable back down to zero then allows for the 'tick' effect without needing to know the time.
-- time fudging test -- by vlek points = 0 time_tracker = 0 function _update() if time_tracker < 29 then time_tracker += 1 else time_tracker = 0 points += 1 end end function _draw() cls() print(points) end |



Hey guys, if the number limitation is 32767... how do your tick counter compensate it?
I mean, if you're at tick #32765 and have something to do in 5 ticks, how do you manage the fact that your counter will either go to -32767 in 2 ticks or 0 if you decided to reset it?
Cheers,



All variables wrap at 32767. If you want to fire off an event in a few ticks, just set its tick to what the tick number will actually be in a few ticks, wrapping included.
Like, in your case, if cur_tick is 32765 and you do "event_tick=cur_tick+5", you'll find that event_tick wraps and ends up being -32766, which is what cur_tick will be in 5 ticks.
You do have to make sure you compare for equality and not less-than/greater-than. That means you have to process your event queue every tick so you never miss the tick where cur_tick==event_tick.
Also...
If you really want more numbers before your game's tick count wraps, you can count with the smallest fractional increment ( 0x.0001):
cur_tick = 0 function _update60() if btnp(0) and not event then event = { tick = cur_tick + 0x.0005, damage = 100 } end if event and event.tick == cur_tick then explode(event.damage) event = nil end cur_tick += 0x.0001 -- smallest possible increment end function _draw() -- switch between blue and black about once per second if band(cur_tick, 0x.0040) == 0 then cls(0) else cls(1) end end |



You could also do event_tick-cur_tick <= 0 and get an inexact comparison, yes? As long as the difference you're looking for is smaller than 32765 ticks. The wraparound preserves the difference.



True, dddaaannn, you can always look at the delta for short-term delays, since it'll handle wraps correctly. I just don't like to use 16-bit frame counters that way since I've been burned by them in the past on games that had very long delays on things like spell cooldowns.



You can also have an infinite ticker by using a sprite as a metronome.
function tictac()
tic = false
metronomo.x += 4
if metronomo.x > 128 then
metronomo.x = 0
tic = true;
end
return tic
end
If you add tictac() to the _update you'll have a working metronome. You can decide wether you want to show it on screen or not.
[Please log in to post a comment]