So I have this planet generator that creates a texture and a set of points as userdata, drawing the planet with a single pset(planet.points) line. But then I want to animate it so it looks like rotating. In each frame I get the UVs for each point, sample the texture with an offset and I get what you see in that image, but rotating. Looks nice.
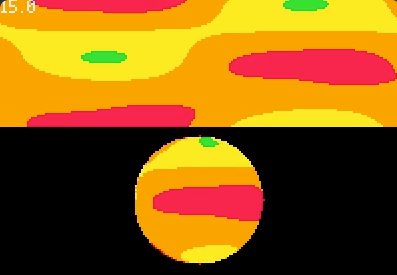
So far so good except because it's painfully slow - a planet with radius 32 is drawn at 15fps :(
So I thought I could speed this up by precomputing UVs and got to something like this to update the animation:
for i=1,planet.discArea do
local tx,ty = planet.uvs:get(0,i,2)
local xx,yy = planet.points:get(0,i,2)
tx = (tx + planet.rotation) % texture_width
local index = flr(ty) * texture_width + flr(tx)
local a,b,c = planet.texture:get(0,index,3)
planet.points:set(0,i,xx,yy,c)
end
Which got me exactly to the same outcome, at exactly the same fps :'(
Given that I do have to iterate all points to get the new color from the texture based on the planet rotation, I fear I can't do much more to speed this up. Or could I? Would love to read ideas! :D


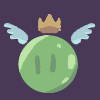
I did something like that to have an “planet pet” in my desktop2 a while back. I just saw your post on discord, if you what to share your cartridge we can compare approaches but at first glance I think reading and writing into a userdata for every pixel might be quite costly. Or somewhere else you might be doing calculations for parts that would not appear on screen in that frame, from experience, that costs a lot.
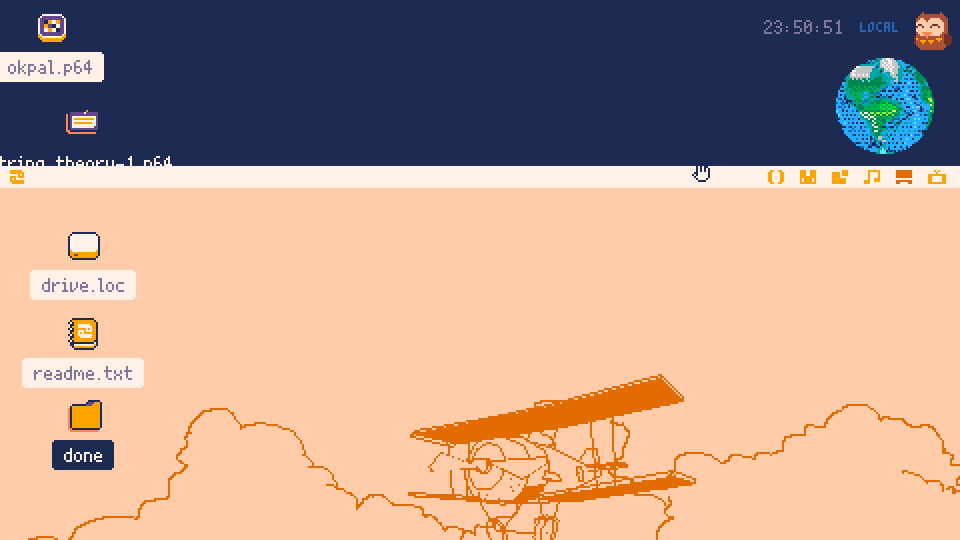


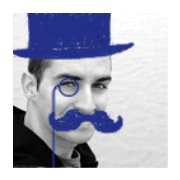
I'm not doing much more than this
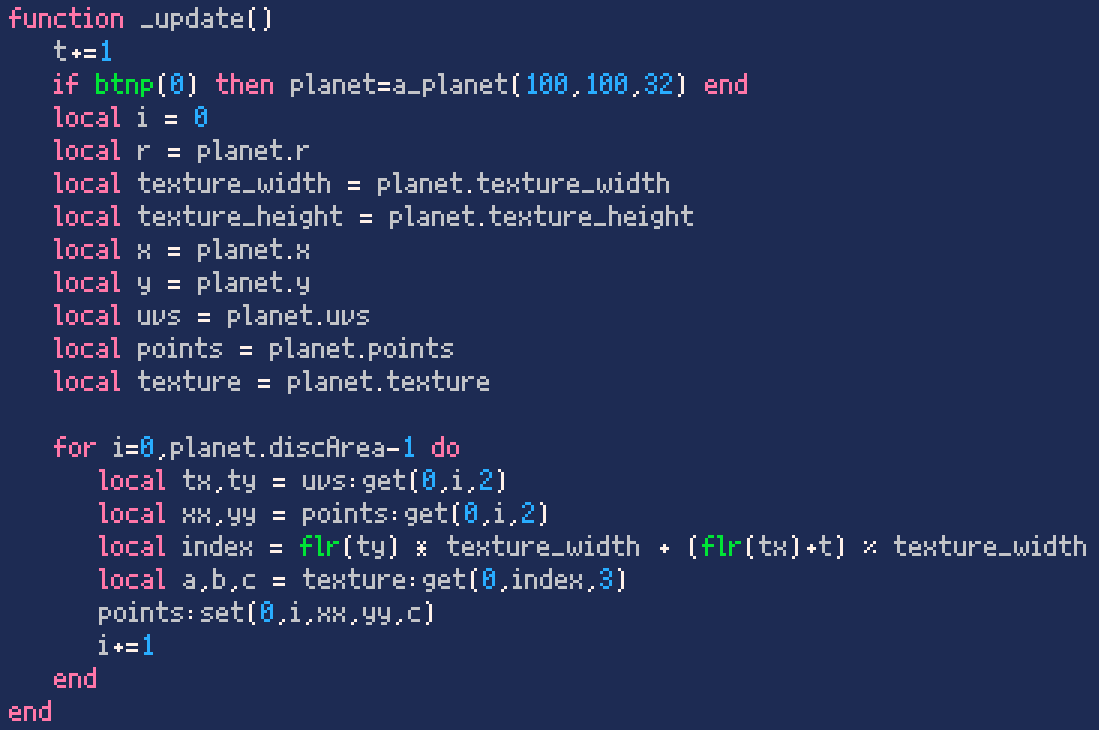
The only thing I see I could speed up would be doing those operations on tx (adding one, modulus with texture width) and maybe on indexes (this one I'm not sure, as it involves a multiplication with one component, and adding the other one)
But I still have to loop planet.discArea to set point colors :(


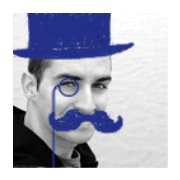
Something that also puzzles me is that all those userdata are f64. They could be integers, as I don't need floats, but if I use i32 points are drawn all black... That's a different issue, but I might be doing something wrong at some point.


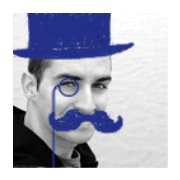
Just an update, and thanks to very nice people in the discord channel, I got up to the point where the drawing loop looks like this:
for i=0,planet.discArea-1 do
local tx,ty = uvs:get(0,i,2)
local xx,yy = points:get(0,i,2)
local c = albedo:get(tx,ty,1)
points:set(0,i,xx,yy,c)
i+=1
end
which only uses get and set! So maybe I can get rid of the loop altogether? That's the next step.


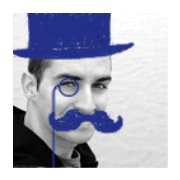
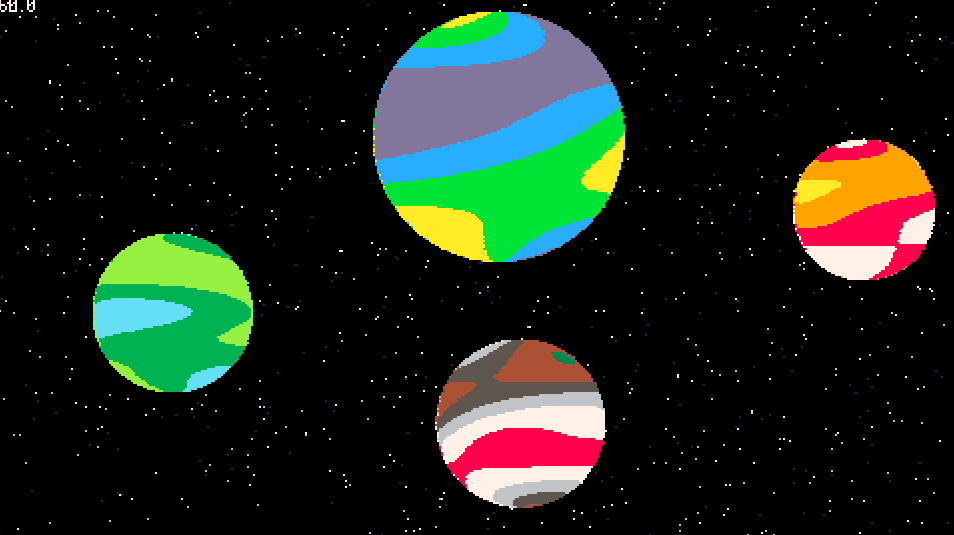
Well, thanks to @abledbody and @fred72 (oh, no mentions here?) I got to draw not one but four rotating planets at 60fps. Which is great, because I don't need them so big, which means I can add more content. I finally managed to do it without looping with userdata's take, like this:
function update_planet(planet) -- animates uvs to make the planet go round planet.us=planet.us:add(planet.rotation_speed,false,0,0,1,1,1,#planet.us) planet.us=planet.us:mod(planet.texture_width,false,0,0,1,1,1,#planet.us) -- get new flat indexes local flat_indexes=planet.vs:mul(planet.texture_width,false,0,0,1,1,1,planet.discArea) flat_indexes:add(planet.us,true,0,0,1,1,1,planet.discArea) planet.texture_albedo_flat:take(flat_indexes,planet.points,0,2,1,1,3,planet.discArea) end |
and think I can get rid of the planet.vs:mul operation precomputing indexes because that's a constant if only Us change! Still would need a take operation to copy base values though.
Yay!
[Please log in to post a comment]