I'm working on a game that notes how high players climb. Right now I'm trying to put together a "ruler" that stays on the left side of the screen and scrolls with the player, letting them know at a glance how far they've traveled. Every 50 pixels, the ruler has a slightly longer line, and a printed numerical value indicating the corresponding height. Here's what I have so far.

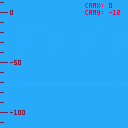
Right now, the ruler is powered by a for
loop in the function draw_elevation()
, which means that it has a definite start and end. Could anyone recommend a method for making the ruler extend in both directions indefinitely (without crashing PICO-8)? My efforts to try while
and repeat
loops have failed. (Bonus points for making the 0
line start at the bottom or center of the screen!)
Here is the code as it stands, since the site's cart dropdowns aren't working right now:
--height measure function _init() camx=0 camy=0 screen_height=128 end function _update() if btn(⬆️) then camy-=1 end if btn(⬇️) then camy+=1 end end function _draw() cls(12) camera(camx,camy) draw_elevation() --debug: cam values print("camx: "..camx,85,camy+3,8) print("camy: "..camy,85,camy+10,8) end function draw_elevation() --to do: have 0 line at screen bottom (or at center with negative heights below) --to do: make the ruler extend indefinitely so that any height is recorded/printed --these values are kind of arbitrary at the moment for i=150,-screen_height,-10 do --draw measuring lines line(0,i,3,i,2) --longer line for every multiple of 50 if i%50==0 then line(4,i,7,i,2) --label for longer line print(-i,10,i-2,2) end end end |


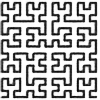
You're almost there, but instead of displaying your ruler in the fixed 150 to -128 range, you have to adapt the starting and ending point to only display the part of the infinite ruler that is onscreen. Depending on your game, remember that valid integer coordinates can't go above 32767 or below -32768. If you need more, things get a bit more complicated.
for i=150,-screen_height,-10 do
becomes
top_tick=(camy\10)*10
for i=top_tick,top_tick+screen_height+10,10 do
top_tick is the height where to place the tick that is on or above the 1st line of the screen. By integer dividing by 10 and remultiplying by 10, you discard the unit digit, and because the Y axis goes down, your tick goes up onscreen.
For original placement of the 0 tick to the bottom of the screen, I just set camy to -127, but you usually get that kind of placement for free once the camera is tied to the player.
[Please log in to post a comment]