

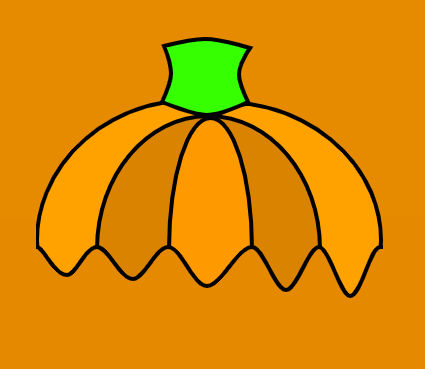
Decimals are stored in floating point format, so it doesn't make sense to use bitwise operators on them. Perhaps treating them as fixed point just for the bitwise operators could be a solution.


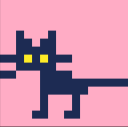
-
First, we have
0x0.1 << 4
. The<<
operator means left shift, which effectively multiplies the number by 2 raised to the power of the right operand. In this case,4
. So:
0x0.1
in decimal is0.0625
.
Left-shifting by4
gives usceil(0.0625 * 16) = 1
. - Next, you mentioned using
ceil(0x0.1 * (2^4))
. Let’s calculate:
2^4
is16
.
0x0.1 * 16
is1
.
The ceiling of1
is still1
.
If, instead of expecting the value to be 1, we have 0x10
(which is 16
in decimal), it’s clear that there’s a misunderstanding about bit shifting. However, the approach in this case is similar.
The desired value, which is the result of the calculation, is 0x10
. To achieve this, consider the following:
Calculate 16 / 0.0625
, which gives us 256
.
Therefore, 0x0.1 * 256 = 16.0
, and expressing 16
in hexadecimal gives us 0x10
.
As further evidence that the issue is not due to a bug, let’s consider the same scenario in Python. First of all, the value 0x0.1
cannot be handled.
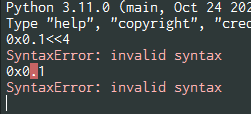


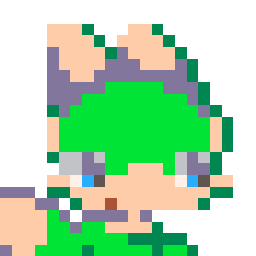
Thank you.
I misunderstood, and it seems that bitwise cannot be handled in floating point in general.
I think I need to be more specific about why I tried to perform this operation.
bunpack()
This is a function that slices a number by bit and returns it.
Bitwise, which supports pico-8's 32-bit fixed point, is used.
I was trying to introduce this function to convert data read from a spritesheet to picotron. (It divides it into multiple bit lengths.)
My personal feeling is that I hope for a future where assets accumulated from the pico-8 era can be smoothly migrated to picotron, not just to the generally considered correct method.
However, at present, poke4-poke8 and peek4-peek8 do not return numbers after the decimal point, so I am aware that my thoughts are troublesome.


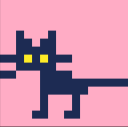
I see, I think it's fine with normal bit operations, but I'm sure there are some convenient ways to use them that I don't know about.
I was also surprised to learn that there are such ways to use them in Pico-8.
As usual, I can't seem to find a way to use them, but I wonder if they would be useful in demo scenes or something. It was a learning experience. Thank you.


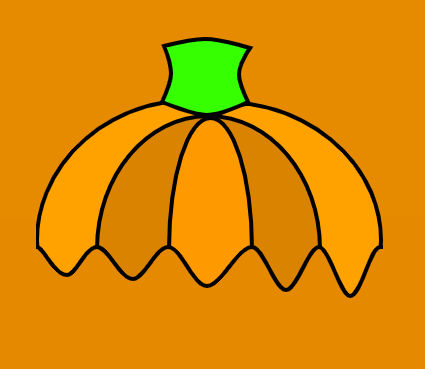
Hmmm.. on second thought, floating-point bitshifts are just a matter of incrementing or decrementing the exponent, are they not?



0xabcd.1235 in pico8 was actually a 32bits integer (16:16 fixed point).
On picotron this is a floating point and there is no implicit conversion when doing bit maths.


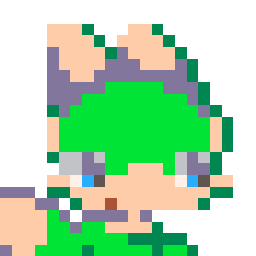
For now, I have created replacement code for the part that led me to write this post.
I can now accept this handling of decimal points as a picotron specification.
- bunpack for picotron


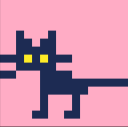
To be honest, I didn’t take this matter too seriously, but it may be more serious than I think.
a = userdata("f32",4) a[0] = 0.01 a[1] = 0.2 a[2] = 11.0 a[3] = 12.1 for i=0,#a-1 do print(string.format("%d: %f", i, a[i])) end |
The execution result is as follows, surprising!
0: 0.000000 1: 0.000000 2: 11.000000 3: 0.000000 /> |
As a precaution, using a table will yield the expected values.
b = {0.01, 0.2, 11.0, 12.1} for i, v in pairs(b) do print(string.format("%d: %f", i, v)) end |
> Ah, I see! When using userdata
with the type f64
, it seems to work as expected. Interesting!
> However, when using memmap
to map userdata
to memory, it seems that it doesn’t work with f64
. Well, it’s still in alpha, so let’s be patient and wait.


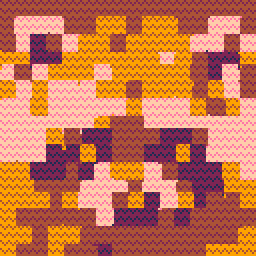
> I hope for a future where assets accumulated from the pico-8 era can be smoothly migrated to picotron
have you seen p8x8? you can convert a p8 cart to p64, load the p64 cart, and then copy out the generated .gfx files. (I also made an earlier version for spritesheets only, but I recommend using p8x8)


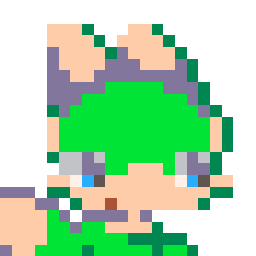
With the handling of decimal points, the output may not be as expected, and we may end up questioning whether it is really correct, so there seem to be unexpected risks in developing it at this point.
But even so, I want to be honest with my urge to create!


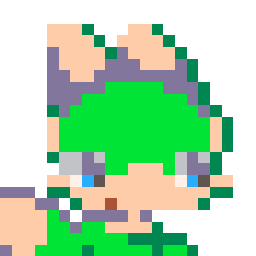
That's a great tool! I think your attempt is great.
However, most of what I make is bitwise-heavy, and when I try using p8x8, I have to manually correct it. (There's also the decimal point problem mentioned above.)
If it also supported old pico8 functions like bor()
, lsh()
, and rotr()
, I think it would be possible to do "p8 to p64" more widely.
Once all the bit operators are available for picotron, I'll have more opportunities to use p8x8.
[Please log in to post a comment]