I'm trying to figure out the alternate colors a bit so I can use them in a game. I'm not trying to get all 32 colors available at once. I'm happy with having 16 but just want to adjust which 16 I have. From what I've read, it seems like this is possible.
I put the display pal() swaps at the top of the cart which does switch out colors. And it works when drawing and with sprites. Cool.
pal(3,129,1) -- blue129 pal(5,140,1) -- blue140 pal(6,135,1) -- yellow135 pal(11,137,1) -- orange137 pal(12,132,1) -- brown132 pal(14,128,1) -- brown128 |
So I have a sprite that is drawn with original blue12 and then on the screen it displays as brown132 because of the swap. I get that and it's working.
But I want to be able to display that same sprite again in a different color.
How do you do a swap on a swap?
-- this sprite is draw in blue12 and displays as brown132 = expected spr(1,12,96) -- how do i make the same sprite display as orange137 -- pal(12,137) -- this doesn't work, it swaps both -- pal(132,137) -- this doesn't work, both still brown spr(1,62,96) |
I'm still looking for the flexibility of easy pal() swaps even after I've swapped for colors in the alt palette. Maybe it's not possible in the way I'm wanting. It's certainly not the end of the world if it won't work like I think. I've found a ton of posts on the alt colors but none of them seem to address swapping within a swap.


I did try passing the 1 as the third param but it didn't work like I had hoped. It changes the color in both sprites like it does without the param.
Thanks for chiming in...this is one of things that seems like it should be easily handled with pal() stuff but clearly isn't. It's a bummer.


@morningtoast
koam0naut's response is correct. pal(12,11,0) or pal(12,11) for short is what you wanted
Here's why :
You have palette 0, the draw palette, and palette 1, the display palette.
(technically, you also have palette 2, the alternate palette, that is not used in standard display mode)
To render the screen, each pixel is encoded as a 4 bit value (0-15) in memory.
That pixel value is an index into the display palette. That's why you only get 16 different colors maximum onscreen in the default display mode.
Each value in the display palette is a byte, but the 256 possible values have cyclic representation (7 is white, but so is 32+7, 64+7...), there is only 32 different colors in the end.
The 16 draw palette entries on the other hand, have values in the 0-15 range. These are not colors, but indexes that will be written in the video memory when you use drawing instructions like spr() or line().
when you do
pal(A,B) which is equivalent to pal(A,B,0)
it's not really a color swap : you are telling that entry A in the draw palette will now write index B into the video memory when used in drawing instructions (if entry A is not currently set to transparent).
when you do pal(12,137) you are using a color number where an index is expected, but in pure lua spirit, everything is fine, we'll just silently ignore the extra bits and confuse you instead of crashing the game.
What you wanted was pal(12,11) : your sprite that shows in color 12 in the editor and writes index 12 in memory will now write index 11 (currently orange137 in the display palette)


Thanks for the insight, that makes sense...and I think that helped me solve it???
I think what I wasn't doing was the pal() swap BACK to the original.
I'm swapping 12 for 131 at the init. That covers the first sprite but then when I pal() swap for the second to be a different color, that loops back and affects the first sprite so they both end up the same color when displayed. At least I think that's what's happening. Because when I add the pal(12,12,0)
before that first sprite, I do get each one being a different color like I want.
This code gets me what I want...
pal(11,137,1) -- green11 to orange137 always pal(12,131,1) -- blue12 to green131 always function _draw() cls() pal(12,12,0) --green131; needed so the next pal() doesn't effect this one when looping(reset it) spr(1,10,10) pal(12,11,0) --orange137 spr(1,20,20) end |
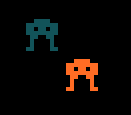
Cool. But I guess the true downside here is that if I need to "reset" the pal()
each time, that could be a ton of extra pal()
calls which add up the tokens.
So the task now seems to be how to efficiently plan for the color swapping so it doesn't suck up too much token space otherwise. Or the decision to be is it worth having the secondary colors if I have to jump through a ton of pal() hoops? Hmmmm...
But thanks for the tip and info. It helped me think about what was happening and missing.


And it looks like I don't need that third 0 param in this case. It works without it too.
function _draw() cls() pal(12,12) spr(1,10,10) pal(12,11) spr(1,20,20) end |


To save tokens if you have lots of spr calls with palette change :
If 12 is your special "draw" color across all sprites, you could do
function spr_color(s,x,y,c) pal(12,c) spr(s,x,y) end |
Or if you want to keep entry 12 of the draw palette intact after use :
function spr_color(s,x,y,c) local draw_12 draw_12=@0x5F0C -- C is 12 in hexa 0x5F00 is the address of the draw palette pal(12,c) -- same as poke(0x5F0C,c) spr(s,x,y) pal(12,draw_12) -- same as poke(0x5F0C,draw_12) restore old value end |


@koz - Thanks! That could save the day. I could toss the pal(0)
at the end of the loop.
My idea might be practical yet! Thanks all!
[Please log in to post a comment]