Hi guys:
This is kinda embarrassing but I'm not 100% sure how ELSE works.
If an if condition is originally TRUE, does the ELSE say "Well I'll do this is it's FALSE"?
c="ORANGE"
IF c="ORANGE" then print "YES" (This is definitely true)
ELSE print "NO" (Somewhere in the code, c changes to APPLE)
But what about OR comparisons?
c="ORANGE"
IF c="PLUM" OR c="GRAPE" then then print "YES"
ELSE print "NO" (It does this line because c is NEITHER PLUM or GRAPE)
I think that's correct so far. But I get a bit stuck on ELSEIF
c="ORANGE" b="APPLE"
IF c="PLUM" OR c="GRAPE" then then print "YES"
ELSEIF b="APPLE" print "THIS LINE!"
Does the psudo code above confirm the fact that c is NOT PLUM or GRAPE but sees that b is APPLE so runs that and prints "THIS LINE!"
It's hard to explain why I get stuck. I hope someone gets what I'm trying to ask.
Cheers


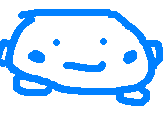
First, be careful in your comparisons: always 'if c=="orange"', never '=' which assigns a value and does not compare.
For your question: yes, else runs if the expression evaluated by if is not true. It doesn't matter if you have 'if something' (goes to 'else' only if something is false or nil), 'if something < 20' (goes to 'else' if something is 20 or higher), 'if not something.dead and something.blip and something.blip == "bloop"' (goes to else if something.dead is true / if dead is false but something.blip is false or nil or not defined / if dead is false and blip is not equal to "bloop"). To put it differently, 'if' and 'else' don’t know what’s in the expression; the format is really 'if anything-here-that-is-checked-to-be-not-false-or-nil then do stuff else do other stuff'.
'elseif' is really the same as having another 'if' inside an 'else' block, but one line more compact.



c="ORANGE" b="APPLE" IF c="PLUM" OR c="GRAPE" then then print "YES" ELSEIF b="APPLE" print "THIS LINE!" |
lets write it a little bit diffrent:
c="orange" b="apple" if c=="plum" or c=="grape" then print("yes") elseif b=="apple" then print ("this line!") end |
is the same as
c="orange" b="apple" if c=="plum" or c=="grape" then print("yes") else if b=="apple" then print ("this line!") end end |
elseif is only a shortcut - and always indent lines, it makes so many things easier.
so your code first check if c is "plum" or "grape". When yes it print "yes" and jump over the end of this snippet.
When c is not "plum" and not "grape" the code in the else-part is executed. In this case you have a nested second if statement. And when then b is "apple", it output "this line!".
but what happend when you:
c="plum" b="apple" if c=="plum" or c=="grape" then print("yes") else if b=="apple" then print ("this line!") end end |
in this case c is "plum", so the first if-statment ist true and it will print "yes".
the nested if in the else part will never be executed here!
You can short this to:
c="plum" b="apple" if c=="plum" or c=="grape" then print("yes") elseif b=="apple" then print ("this line!") end |
Same as above - it prints only "yes"!
And remember "or" means here "at least one of the conditions must be true". for example
c="circle" b="box" if c=="circle" or b=="box" then print("yes") else print ("no!") end |
will output "yes"



Many thanks for the help here merwok, GPI & MrGoober. Much appreciated.
Cheers



@merwok
ups. I didn't test the code :) - my problem is, that I primär program in Basic and there are no ==
funny thing is, that lua doesn't allow an assign of a value in the If, while ... statments und could do the same.


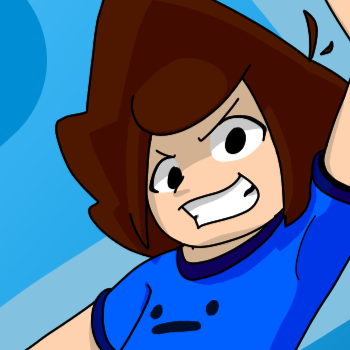
Years later this forum still proves useful for those of which who are trying to learn Lua through Pico-8! Thanks, guys!
[Please log in to post a comment]