I want to do something like this:
function make_object_from_flag(x, y, flag) local flag_obj = { [1] = make_impassable_object(x, y), [2] = make_coin(x,y), [4] = make_screen_transition_object(x, y), } flag_obj[flag] end |
But for some reason it keeps saying flag_obj[flag] is a syntax error: syntax error line 119 (tab 0) flag_obj[flag] syntax error near 'end' attempt to call a string value
Oddly enough, if I comment out flag_obj[flag] but leave the flag_obj table uncommented, it says I run out of memory despite only having a few hundred lines of code
here's the entire code snippet if you need more context:


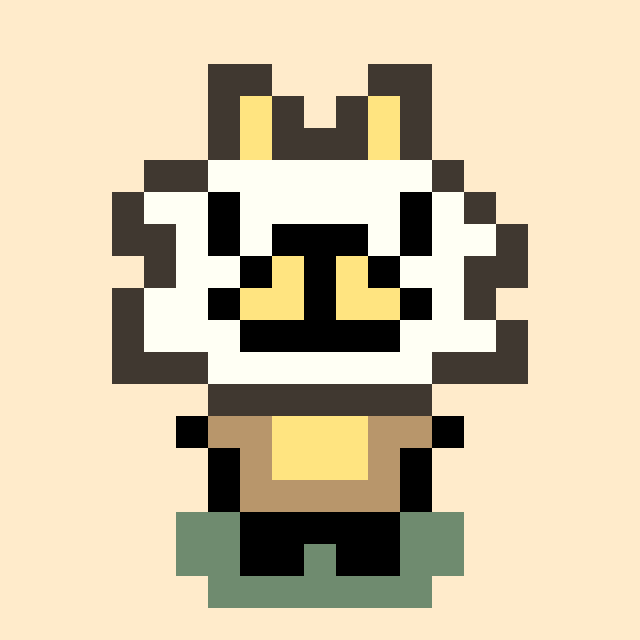
when you do table[key]
it looks for that key and the table doesn't have it.. Like:
t={ apple="poops", jar="nowhere!" } print(t[apple]) -- poops print(t[cup]) -- nil? where is cup? |
Also checking it on it's ownsome; the compiler won't like that.
So ya not sure what your trying to do, if your running out of memory it probably means it's calling it's self.



@picodrake your factory function is a good start but you are mixing function reference and function call.
when writing:
... [2]=make_coin(x,y), .. |
the code is actually invoking make_coin function and stores a coin at table index 2.
this is why you end up with a out of memory error.
the code created maph x mapw x 3 ‘objects’!
the fix is to reference the functions in the table and delay the call, eg:
function make_thing(x,y,flag) local factory={ ... — register a function reference [2]=make_coin, ... } — call function with current parameters factory[flag](x,y) end |



@freds72 thank you so much! That was a great explanation and it makes a lot of sense. For some reason its still throwing up errors, but when I replicated it in a blank program it worked just fine, so I'm assuming it's just some syntax issue I can weasel out.
[Please log in to post a comment]