This has been solved. you may still look at the non-working code and working code.
So for my Merge Chickens game, I have a limit of 64 chickens because there are only 64 save spaces, but when I try to make it so I can save more chickens all I get is:
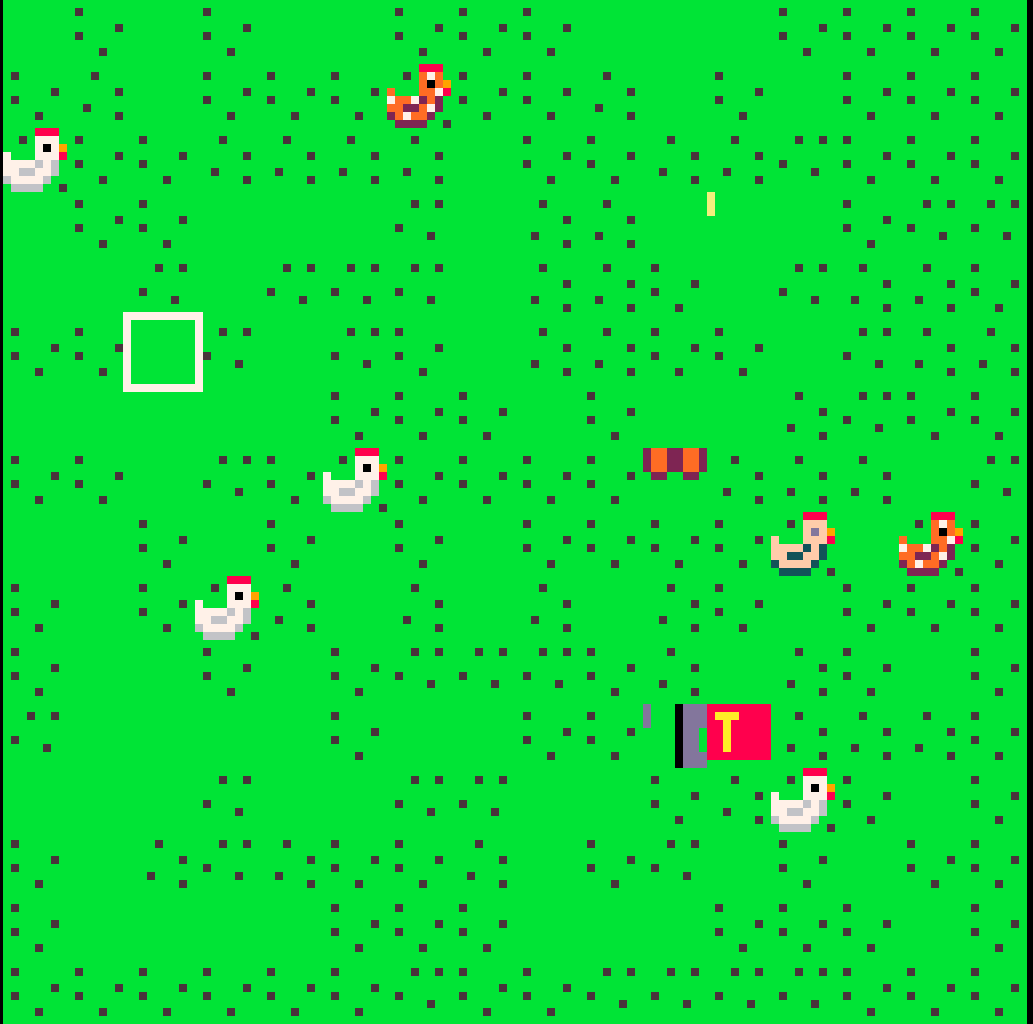
here is my code:
--savebuk() and loadbuk() function savebuk() local d=0 for x=0,15 do--go thrugh all of for y=0,15 do--the map tiles local i=0 local s=0 if mget(x,y)>63 then i=mget(x,y) --if this tile is a chicken --set i to the sprite number end if x+1<16 then if mget(x+1,y)>63 then s=mget(x+1,y)*1000 --if the tile next to the --tile at x is not off the --map and if the tile is a --chicken set s to the tile --times 1000 end else if mget(0,y)>63 then s=mget(0,y)*1000 --if the tile next to the --tile at x is off the map --do the same thing but --with zero end end dset(d,i+s) --save i+s here is an example --i=109 --s=72 --s gets multiplied by 1000 --s=72000 --s+i=72109 --then save that is save spot --d d+=1 --on to the next save spot! end end end function loadbuk() for d=0,63 do --all commented lines is an --example local val=dget(d) --val=72109 local s=flr(val/1000) --s=72.109 rounded down local s2=flr(val/1000)*1000 local i=val-s2 --i=72109-72000 --i=109 local x=flr(rnd(15)) local y=flr(rnd(15)) --find a random position if mget(x,y)<64 then mset(x,y,s) --and if there is not a bird --already there place s --there end x=flr(rnd(15)) y=flr(rnd(15)) if mget(x,y)<64 then mset(x,y,i) end --do the same thing with i end end |
This is the WORKING code
--savebuk() and loadbuk() function savebuk() for sp=64,127 do local num=0 for x=0,15 do for y=0,15 do if mget(x,y)==sp then num+=1 end end end dset(sp-64,num) end end function loadbuk() for sp=64,128 do local num=dget(sp-64) for i=1,num do x=flr(rnd(15)) y=flr(rnd(15)) if mget(x,y)<64 then mset(x,y,sp) end end end end |



So your save function loops over every row and column on the map, generating some i and s numbers, and saving that to persistent memory. d is an index saying which memory slot you're saving to.
One issue is that no matter what your i and s are, you're always incrementing d, and setting memory. Even if nothing is there. So you're saving a bunch of empty tiles, then running out of room with dset.
So probably only increment d and save when you find something. I guess the location of the chickens doesn't matter, but when you load, your random x,y coordinates could overlap with another bird, so you could lose some that way.
Hopefully this helps. It's a bit hard to follow, because the variable names mean nothing, and there are no comments, explaining what's going on.


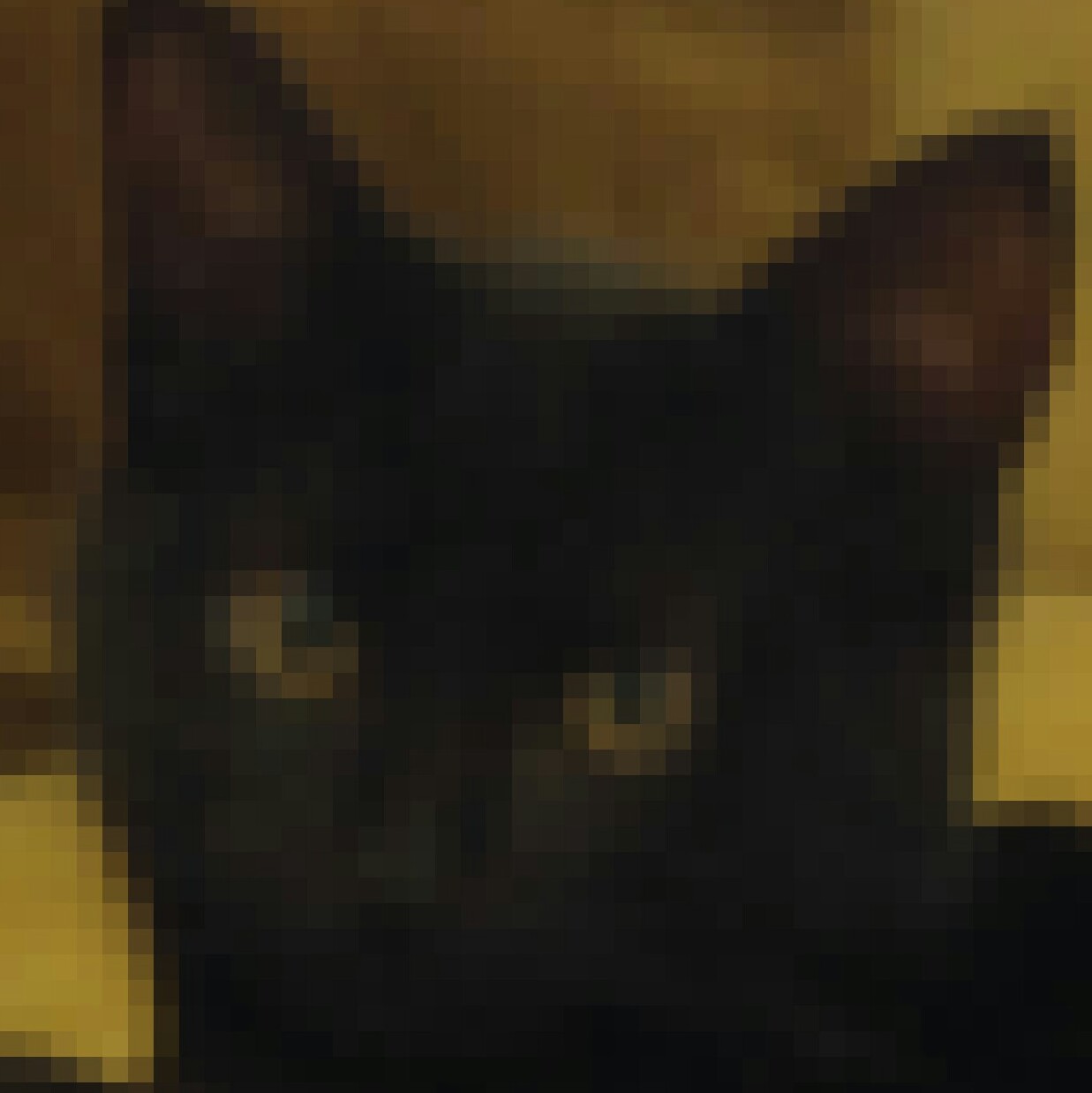
[Please log in to post a comment]