Right now if you want to draw a line loop with the cool line(x,y) continuation method, you still have to do at least one line(x0,y0,x1,y1) first to make it work:
-- draw a simple triangle line(32,96,96,96) -- base line(64,32) -- right side line(32,96) -- left side |
This isn't so bad if you're drawing the figure manually as I just did. But if you're, say, drawing an algorithmic figure's loop, you have to handle that first line conditionally within the loop:
-- draw a simple segmented circle local cx,cy,r=64,64,32 for a=0,1,0x.01 do local x,y=cx+cos(a)*r,cy+sin(a)*r if a==0 then line(x,y,x,y) -- sets the continuation point else line(x,y) -- continued from the last point end end |
Or (not shown) you can remove the condition from the loop, but it requires doing math to get the first point outside of the loop and then calling line(x,y,x,y) as above before looping over the subsequent points.
All in all, neither is really better in terms of code than just drawing a series of distinct line(xi,yi,xj,yj) segments.
However, let's say line() with no args invalidates the last position. Let's also say that trying to continue via line(x,y) from an invalid position draws either nothing or just the one pixel, but it does update the last position. Suddenly everything gets a heck of a lot more concise and elegant:
-- draw a simple segmented circle local cx,cy,r=64,64,32 line() for a=0,1,0x.01 do line(cx+cos(a)*r,cy+sin(a)*r) end |
This should be safe in terms of backcompat, because line() with no args doesn't currently do anything useful, and in fact I'd consider it an undocumented usage that would have been ill-advised to use anyway. Similarly, since there was no such thing as an invalid last point in the past, no one would have written line-continuation code that didn't first update it explicitly in a way that would still work with this change in place.


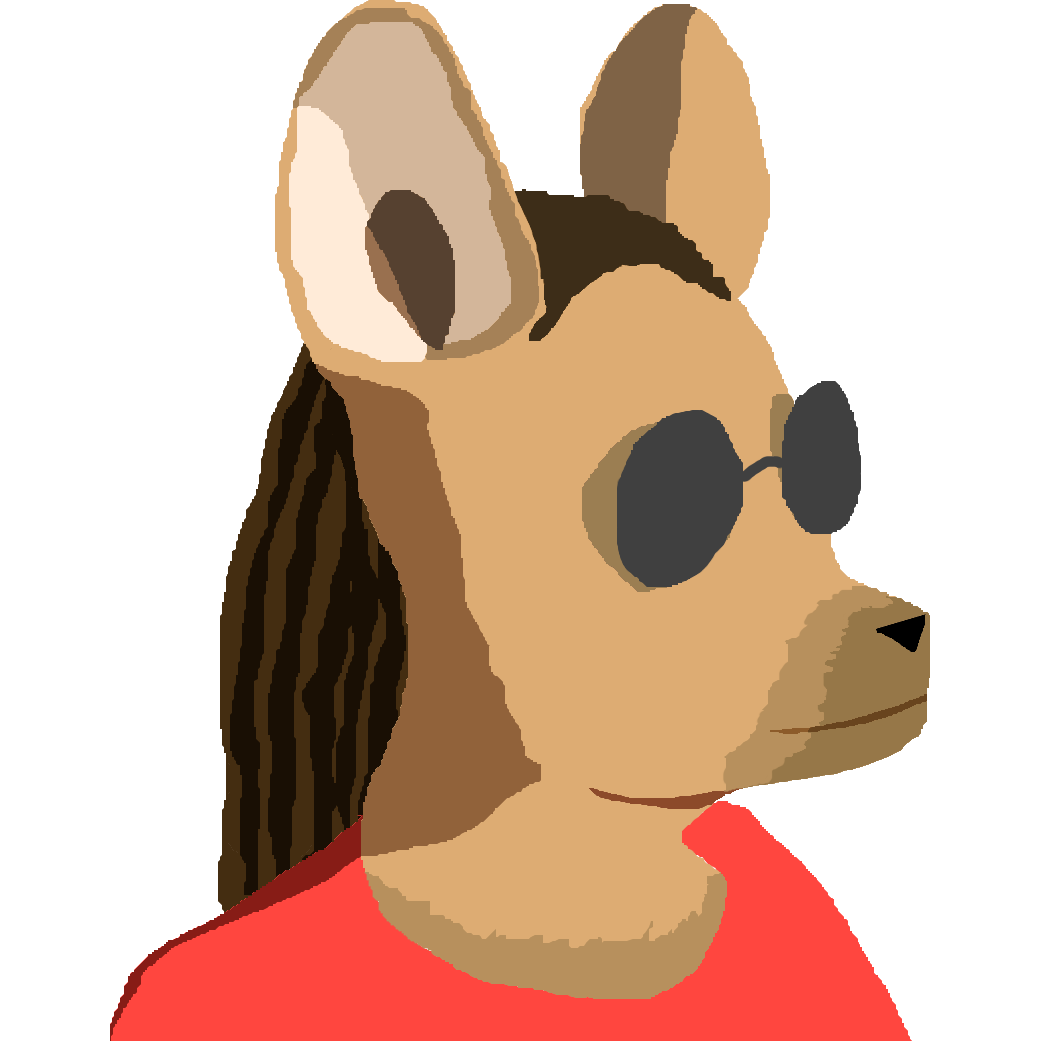
-nods-
We've been referencing the wiki page on the draw state part of memory and using poke2(0x5f3c,[x coord]) poke2(0x5f3e,[y coord]) to set starting coords; I'm not sure how your proposal would be implemented in the fiction of the hardware, but it'd be a lot more elegant and a lot less magic-numbers than that.


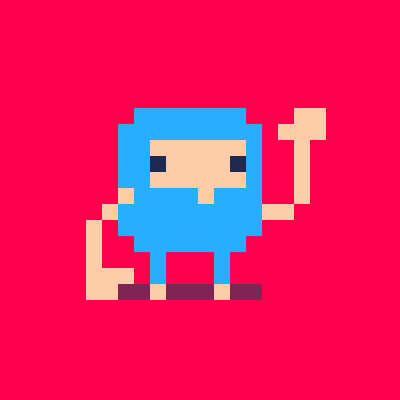
Heeey, this is nice. I've implemented for 0.1.12d and exposed the set-position flag (0x1) at 0x5f3f 0x5f3b.
Only downside I can see is that it creates slightly more statefulness / cryptic code, but I think this is justified by the cleaner code it allows (like the circle example). For the same reason, I've also added setting the draw color by adding a single or 3rd parameter. The result is that anywhere between 0 and 5 parameters are meaningfully accepted \m/


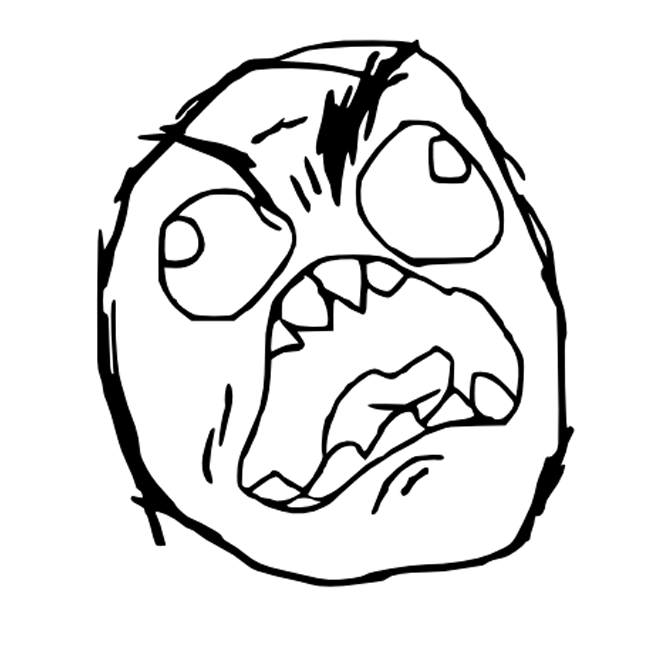
@zep What will happen to the higher bits of the stored Y coordinate if 0x5f3f is now used for that flag?
[Please log in to post a comment]