When loading a file via #include, pico-8 crashes when running the program from the command line, like "run".
The code loaded is tested and used correctly and does not cause the crash. Also, running via Ctrl+R works and behaves as expected.
Editor:
Result:
#include multi.lua m=multi(0,17) m:draw(10,10) -- this works when run with ctrl+r -- it crashes when running with "run" |
multi.lua
local multi = {} multi.__index = multi setmetatable(multi,{ __call=function(cls,...) return cls.new(...) end } ) local function getxy(index) local y = flr(index / 16) local x = index - y * 16 return x,y end function multi.new(tl,br) local self = setmetatable({}, multi) local tx,ty = getxy(tl) local bx,by = getxy(br) local w = bx - tx + 1 local h = by - ty + 1 self.tx = tx self.ty = ty self.bx = bx self.by = by self.w = w self.h = h return self end local function getindex(x,y) return x + y * 16 end function multi:draw(x,y) for u=0,self.w - 1 do for v=0,self.h - 1 do local index = getindex(self.tx + u, self.ty + v) spr(index, x + u * 8, y + v * 8) end end end |
It also would be great, if the folder for includes was the same folder as the cartridges (AppData).


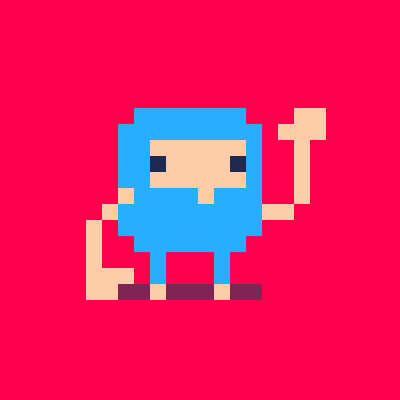
Thanks @greygraphics
I've fixed a related bug that is now live on the downloads page (0.1.12b), and it looks like it might have been a coincidence that it was crashing for you with RUN instead of CTRL-R. Could you try the new version and see if it works for you now?



Hi @zep, I tried version 0.1.12b and I have good and bad news.
Good news: It does not crash anymore.
Bad news: It now throws a syntax error on spr(index, x + u * 8, y + v * 8)
,
namely the first '+'.
When pasting the contents into the editor itself, everything works.
Also, when chaning the line to
spr(index,x-u*8,y+v*8)
(removing all spaces), I get the error
<eof> expected near 'end'
.
I hope this helps.


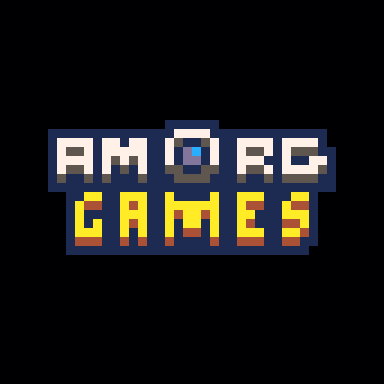
@greygraphics @zep
It is doing the same thing for my friend's windows machine. There is a syntax error for the last line of the file (besides lines that say "end"). I don't have a windows machine to test this more though. But the #include works perfectly in Linux.



It has something to do with how the files are being parsed. Adding 10 or so spaces to the end of the file being included fixes it. I think the parser is terminating early for some reason.



If anyone is still having this problem, it's failing because of the end of line character. if you change it from Carriage Return (CRLF) to Line feed (LF) the problem goes away.
@zep I don't know what the code looks like on the backend, but having the includer convert CRLF's to LF's or whatever would solve this.


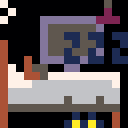
I just ran into this; not the original ctrl-r/run issue, but the other issue mentioned with the #include file being misread whether ctrl-r or run is used. I was testing out #include and it failed, with messages such as:
filetoinclude.p8 line 2 syntax error line 1 (tab 0) function test() print("no") endd syntax error near 'function' |
I was able to "fix" this by including 2 comment hyphens at the end of the file.
The extra "d" was not in my original file, only in what pico8 produced from it.
After a few quick tests my conclusion was that the number of repeated characters equalled the number of Carriage Returns I had.
zerocoolisgod's solution looks ok ... but it's likely there's a bug in the processing of the lines whereby the number of characters read and written doesn't deduct 1 for each "CR", so at the end it looks like there are however-many-"CR"-characters-there-were still to read.
Adding two hyphens to the end of the file seems to make it think the end of the file is reached within a comment so it doesn't look to try to include the extra characters it has counted. (However, I haven't tried this comment trick with long files, only a short test file. Edit: A few further tests indicate that sometimes more space characters at the end are still needed even with the comment characters.)


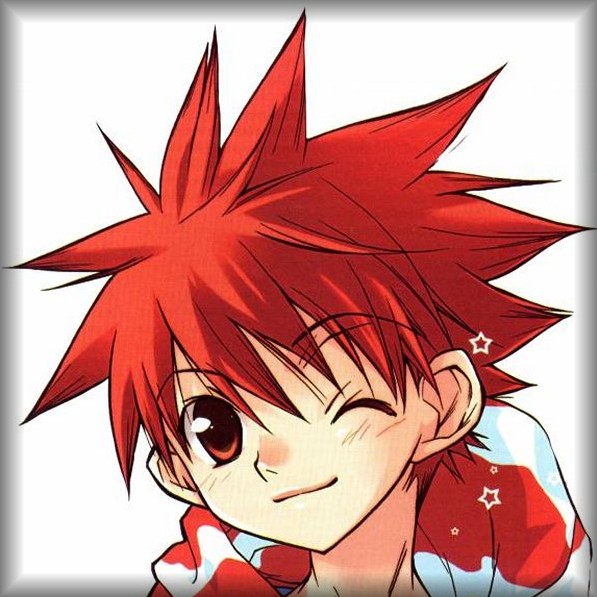
I'm not running into this problem, remcode. I'm using v0.1.12c and I recently wrote a cart that is instrumental for #INCLUDE to work properly.
Tested it with both CTRL+R and typing RUN in immediate mode.
And it's a good practice to get into to always end the line of any include data with a carriage-return.


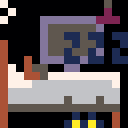
dw817, I'm running the same version as you, 0.1.12c.
I'm using a Windows machine. I omitted to mention this because above, alanxoc3 had already mentioned "It is doing the same thing for my friend's windows machine. ... I don't have a windows machine to test this more though. But the #include works perfectly in Linux."
If you are running Windows and aren't seeing it, well the bug still affects some machines, just not all of them, and that's also not good.
I'm using notepad++ to edit a short p8 program file which is being #included in another p8 program file.
I'm using the enter key to put in carriage returns. (I'm presuming your last comment applies to writing data out to a file, rather than editing a file with a keyboard.)
And the bug manifests when the other p8 program is run either with "run" or "ctrl+r".


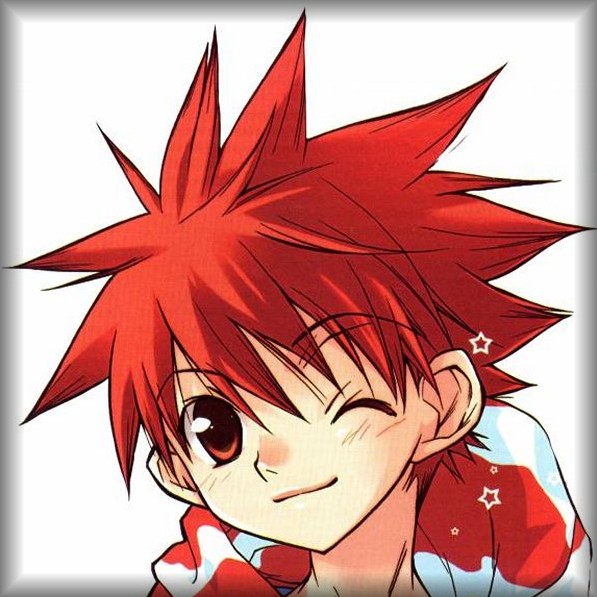
Hmm ... I wonder, remcode, if it has something to do with my own early findings. That you cannot use #INCLUDE online or saved as an EXE unless the data file is present with the EXE.
If you try to run code written with it Online, it crashes, just like mine.
https://www.lexaloffle.com/bbs/?tid=35331
I know it is mentioned this is in immediate mode, just trying to isolate what could cause difficulty with it.
It =IS= a very new command ZEP added. That could be one reason.


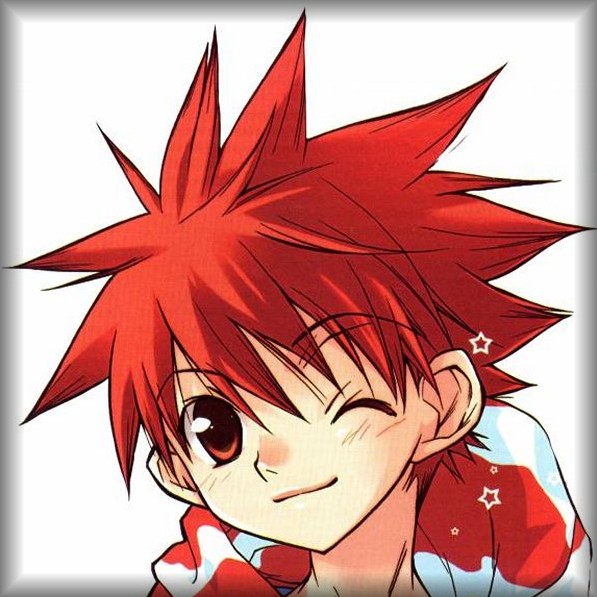
Was doing some more experimentation. Don't know if this means anything. You CANNOT include character 13, only 10 when you use the #INCLUDE command.
If you have character 13 appear it is unknown in all cases.
Try, save this in NOTEPAD:
a=1 b=2 c=3 |
Save it as INC.P8 if you like.
Now try to import it.
#include inc.p8 |
It won't work. That devil #13 character stops it every time.
I would definitely say this is a bug that needs to be addressed.
And this stops me from being able to import real text with CRs apparently.
I can use NOTEPAD++ and work with a text file that only saves #10 through configuration ... apparently that was what I did with that YUKON test by complete accident and didn't even realize it. That's why it worked.
. . .
Okay, here is what to do. Load up the text file you want to be able to import to PICO-8 in Notepad++
Turn on viewing the CRLF mark. You can do so by selecting [V]iew, [S]how symbol, and [S]how end of line.
Now you should see in outline CRLF. To fix this so Pico-8 can read the file do the following.
don't do this, skip to bottom.
Do a[S]earch, [R]eplace.
In FIND WHAT, type: \r\n
In REPLACE WITH, type: \n
Make sure EXTENDED is turned on.
Select REPLACE ALL, and there you have it.
Now as you edit this particular text file it will add the CRLF. When you are ready to save your text file, do this REPLACE WITH method again before you do so you only see LF inverted. Then Pico-8 will read the data.
DO THIS. In Notepad++ click on EDIT and EOL CONVERSION. You should see some choices here.
Select UNIX/OSX format. Done. The added advantage here is you can press ENTER anywheres now and it truly only shows the LF. Phew !
HOPE THIS HELPS !


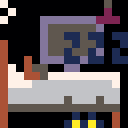
@dw817 Good to see that notepad++ has that built in. Thanks for finding it. And congrats on your progress with your #include experiments.
I didn't know it was a relatively new command - I've not been here a month yet. I'll hope for any little bugs with it to be coded out at some time in the future; meanwhile it's easy enough to not use a CR character. :-)
[Please log in to post a comment]