In ROBLOX I am also learning how to code, but one thing stands out that I feel should be necessary.
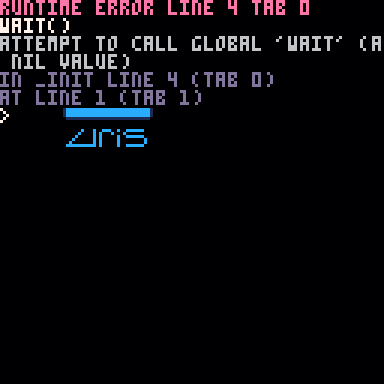
What is supposed to happen is that it runs:
function _init() cls() spr(192,20,20,4,4) wait(3) end |
But it errors with
"Runtime error line 4 tab 0
wait(3)
Attempt to call global 'wait' (a nil value)
In _init line 4 (tab 0)
At line 1 (tab 1)"
Is there a way to make the program wait a few seconds without taking too much space?
Thanks :)



There is no built-in 'wait' command.
Pico basic program pattern is:
- update state & game logic (_update)
- clear + redraw screen (_draw)
every 1/30s
To fit into this pattern, wait is nothing more than a counter. Once the counter reaches a given value, move on to the next game state:
Ex:
local mode="title" local mode_t=0 function _update() mode_t+=1 -- wait 3s (e.g. 90 frames) if mode=="title" and mode_t>3*30 then -- move on to next game state mode="game" mode_t=0 end end function _draw() cls() if mode=="title" then spr(192,20,20,4,4) elseif mode=="game" then -- draw game elseif mode="gameover" then -- draw game over screen end end |
Note: many other variants exist - just find one that suits your design.


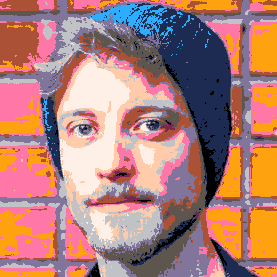
There's also the question: Why do you want to wait? In a "game loop" pattern, like pico-8 uses, waiting the way you seem to want would take away control from the game loop, which would mean that the entire game would simply hang/freeze for a few seconds. That doesn't sound very useful. Try to think about what you want waiting to actually do, and then try to reframe that in a "game loop" model.


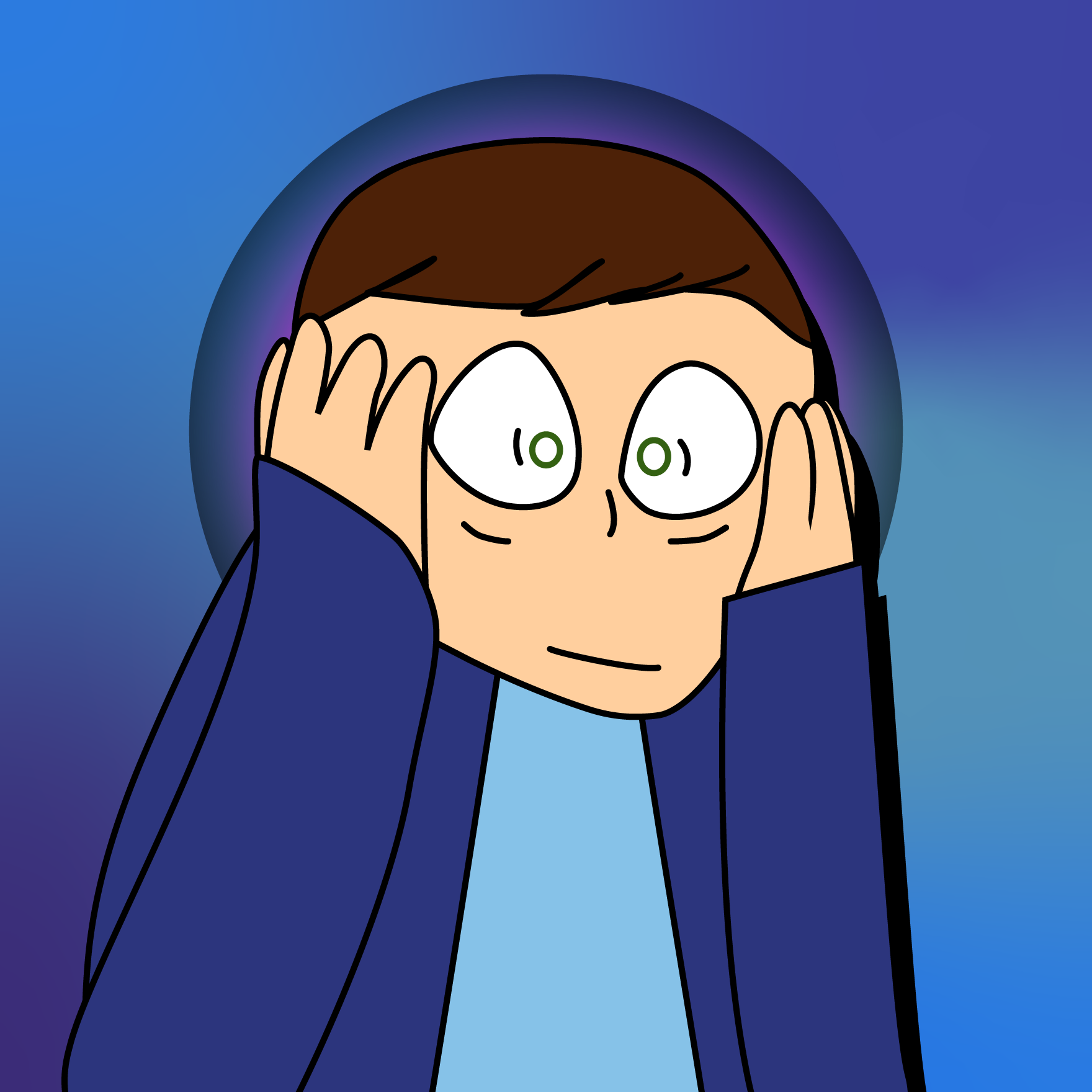



Fade/animation is just using the mode_t counter to switch palette, move things on screen.
(or add other counters as you see fit!)


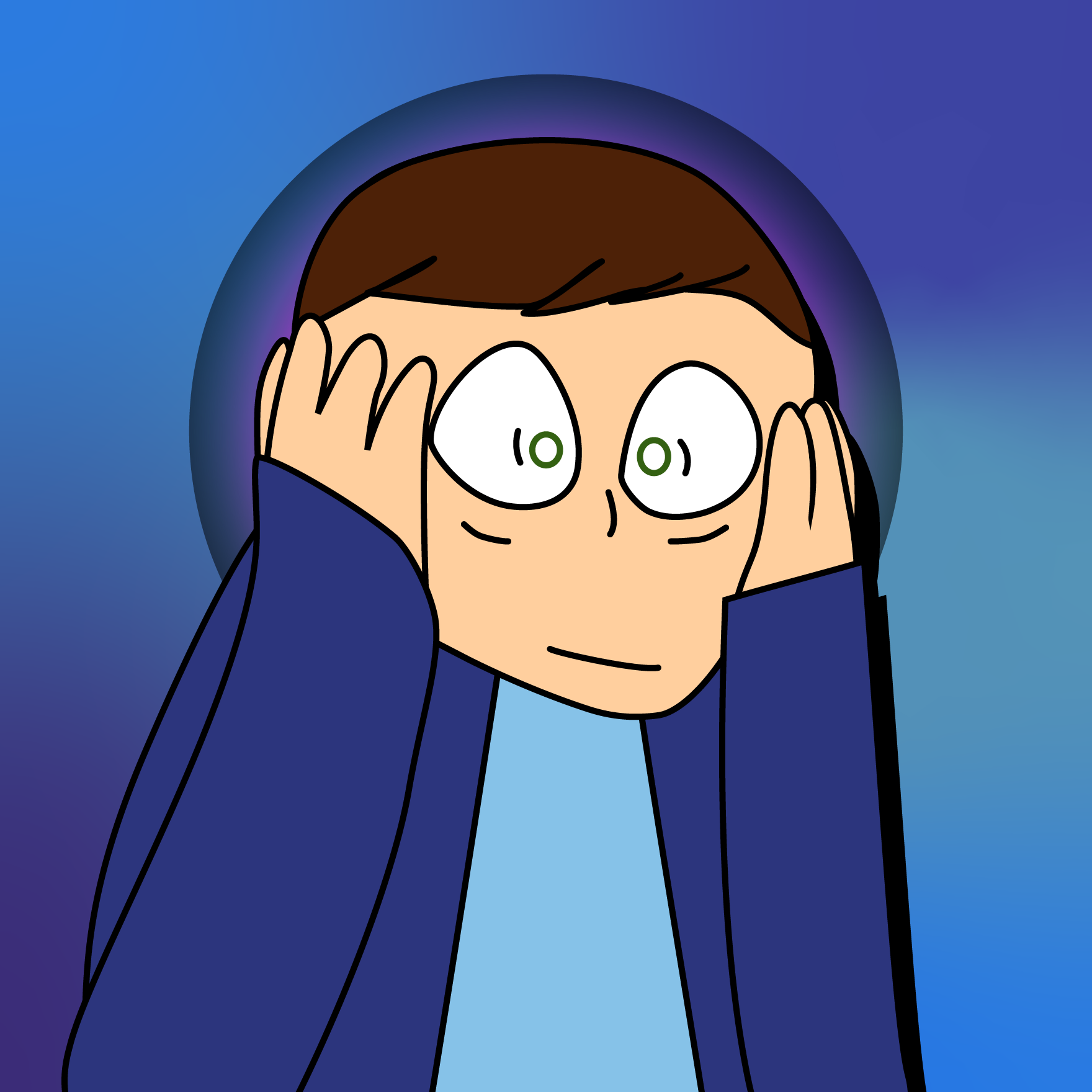
@freds72 Thanks!
offtopic: did you know that _update() can have draw stuff and _draw() can have logic stuff? I didn't know that but heck, I'm new to PICO-8 lol



_update can draw but do not do it
you need code in _draw, but again try to limit game state change as draw calls can be throttled by pico


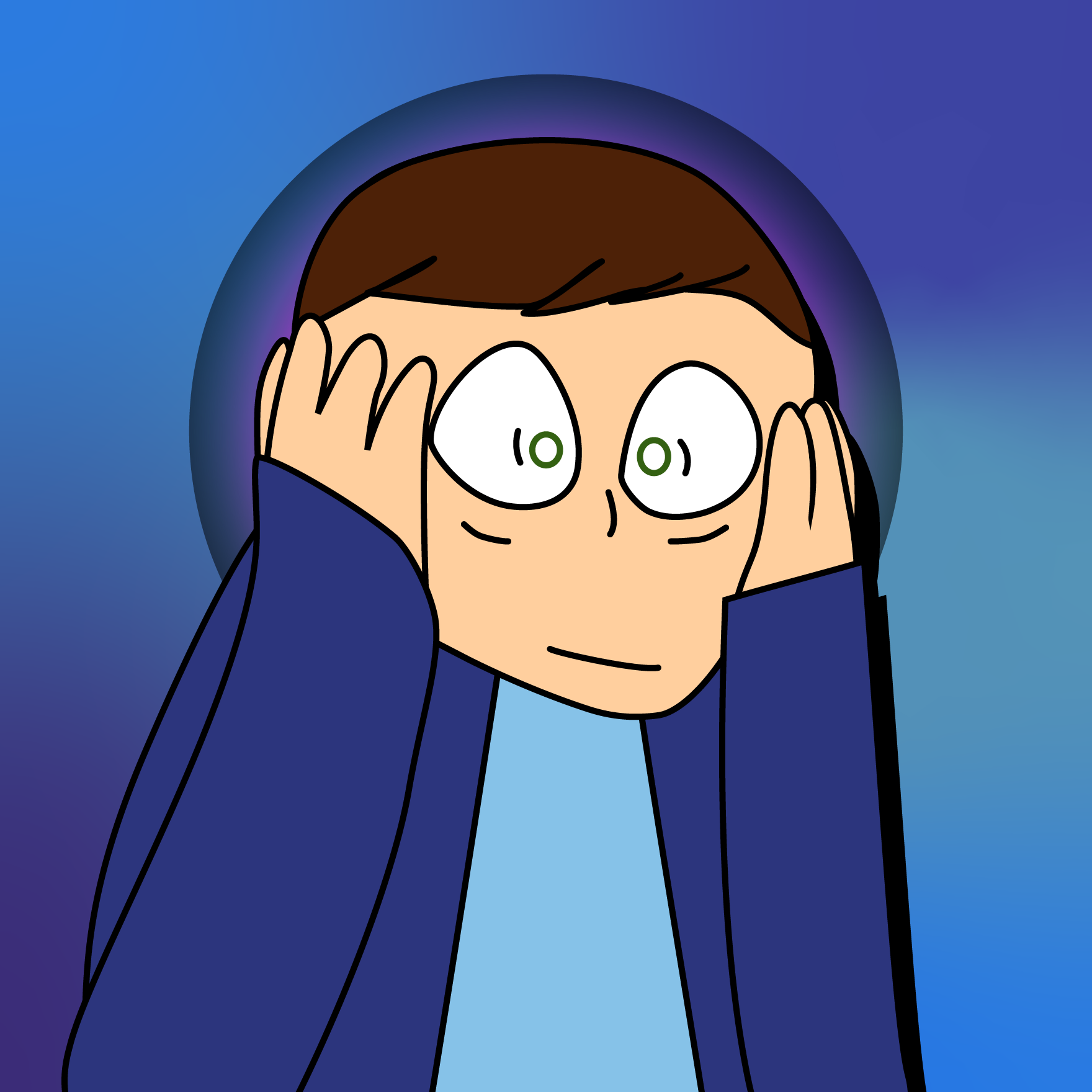
So PICO basically makes calls work less if they aren't in the correct function?


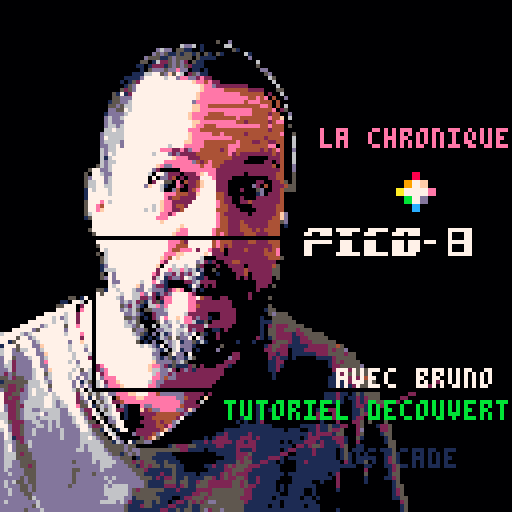
Try this? ;)
function wait(_wait) repeat _wait-=1 flip() until _wait<0 end |


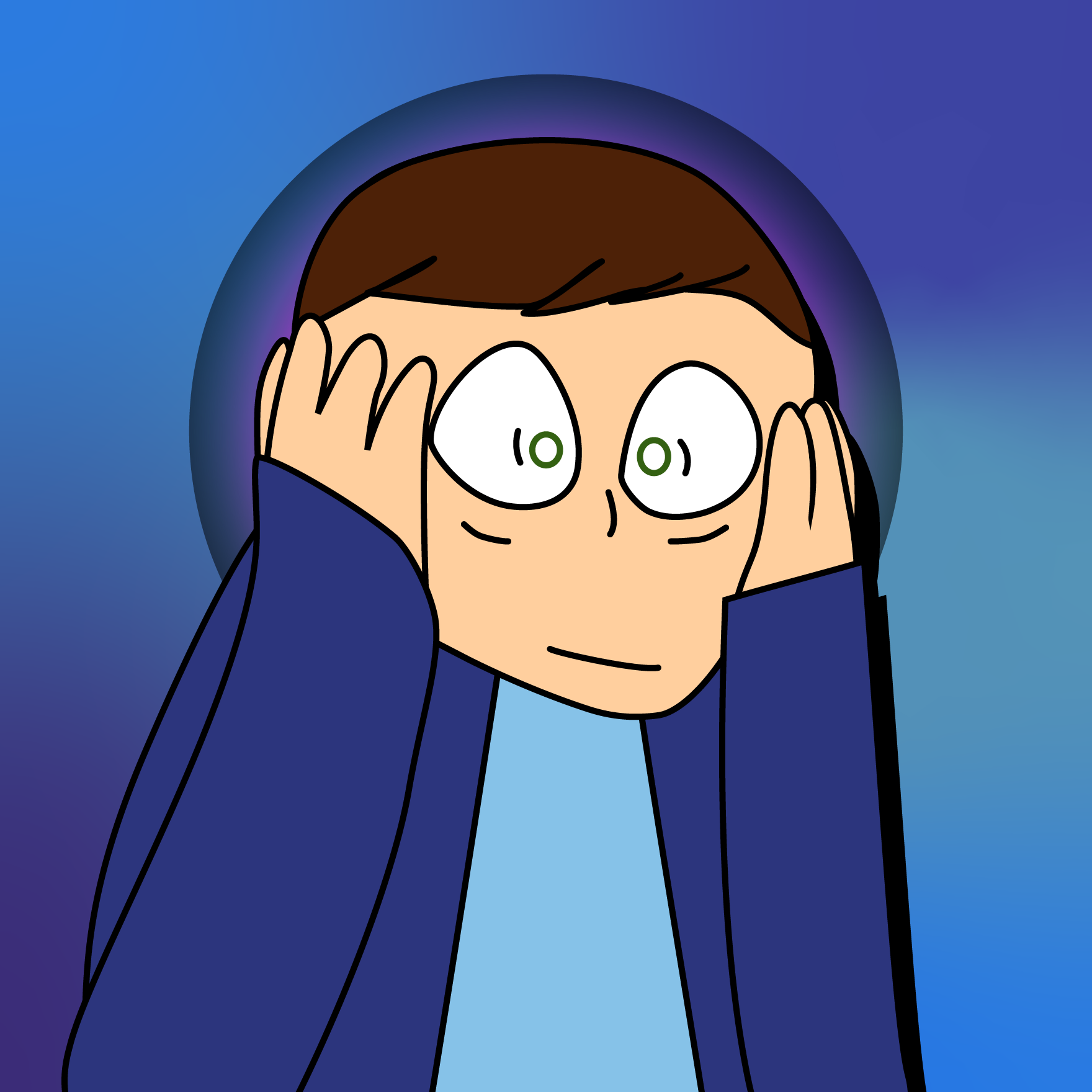
@BGelais Ooh, that's cool too!
We don't need any more responses, I get the gist of how to make a custom wait() thing. Thanks you guys :)



So PICO basically makes calls work less if they aren't in the correct function?
No - this is just to avoid your code being a total mess!


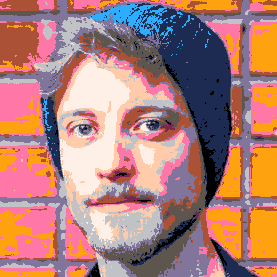
What freds72 meant by "throttling" was just that if you do too much CPU work each frame (ie. each callback of _draw and _update), PICO-8 will "drop frames"; it will skip a _draw() call to catch up. If you're just doing graphics stuff in _draw, then no harm, it will just not be drawn for that frame. (This is what usually happens if a game you're playing "lags" or "stutters" when FPS drops.) However, if you're doing actual game logic in _draw, then your game will behave weirdly and stuff will go out of sync.


If I recall correctly, when Pico-8's CPU is at maximum usage, it will instead call _draw() every other frame to keep up, which can cause problems if you have important stuff in _draw() that needs to happen every frame.
Edit: Whoops! I didn't see Tobias's explanation, so this is kinda redundant.
[Please log in to post a comment]