
Hey all,
A slightly annoying bit of Lua syntax is how verbose the anonymous function syntax is.
For example consider a function filter function that accepts an array and a test function that accepts a single element and returns true or false if the element should be kept. The filter function would then return an array of all elements for which the test function returns true.
function filter(array, test_fn) local ret = {} for elem in all(array) do if (test_fn(elem)) add(ret, elem) end return ret end |
So yeah a pretty straight forward functional programming paradigm that's easy to use and awesome.
It is especially convenient to use with anonymous functions where your test function need not be defined anywhere and can instead be defined inline with the function call. Here's an example that would filter an array to only even numbered elements:
filter({1, 2, 3, 4, 5, 6, 7, 8, 9}, function(v) return v%2==0 end) -- result: {2,4,6,8} |
And this is still good but I find Lua anonymous function syntax to be especially verbose. Consider a similar example in C#:
Filter(someList, (v) => v % 2 == 0) |
Which is obviously much more compact and seems easier to work with especially if you're doing all of your coding in the Pico8 code editor and have limited column width for a big long anonymous function declaration.
Through work, however, I have become familiar with Havok Script which is a modified version of Lua that Havok uses and is available to developers using Havok tools. There are lots of neat features in Havok Script and few of them are of particular importance to Pico8 but consider the Havok Script implementation of anonymous functions with the same Lua example from earlier:
filter({1, 2, 3, 4, 5, 6, 7, 8, 9}, [(v)| v%2==0]) |
Woah, way fewer characters!
All that is going on here is '[' translates to 'function', '|' to 'return', and ']' to 'end'. No fancy lexer/parser magic at all! (well aside from needing to determine that the '[' and ']' aren't trying to index into some table)
One additional feature is that if you have an anonymous function with no parameters the empty parameter list '()' is not necessary as in this incredibly contrived example:
function wait_for_true(fn) while not fn() do end end -- wait until the game timer reaches 5 seconds wait_for_true([| t()>5]) |
Now I'm not sure how viable this is with the pico8 Lua implementation and this specific style isn't necessary but it does seem like it would be in the spirit of pico8 additions to Lua to make things a bit less verbose and this is just one particular feature of Lua that I have found a bit cumbersome.
Anyway, curious if anyone else feels similarly or if this is just my own personal tastes. I know language additions at this stage in Pico8's development might not be viable or prioritized but I think it would be cool!


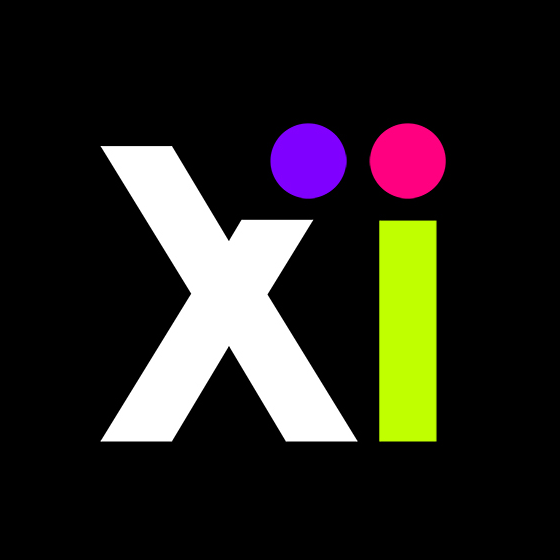
Really? Three whole words? 'function', 'return' and 'end'. I disagree here, PICO-8 and Lua are both about extreme minimalistic simplicity. This is just unnecessary fluff to add.
[Please log in to post a comment]