I see these things in almost every singe program, but i don't know how to use them
snippet from the collisions demo
function make_actor(x, y) a={} a.x = x a.y = y a.dx = 0 a.dy = 0 a.spr = 16 a.frame = 0 a.t = 0 a.inertia = 0.6 a.bounce = 1 a.frames=2 -- half-width and half-height -- slightly less than 0.5 so -- that will fit through 1-wide -- holes. a.w = 0.4 a.h = 0.4 add(actor,a) return a end |
also, what does the "return" do?
Sorry for asking such an obvious question


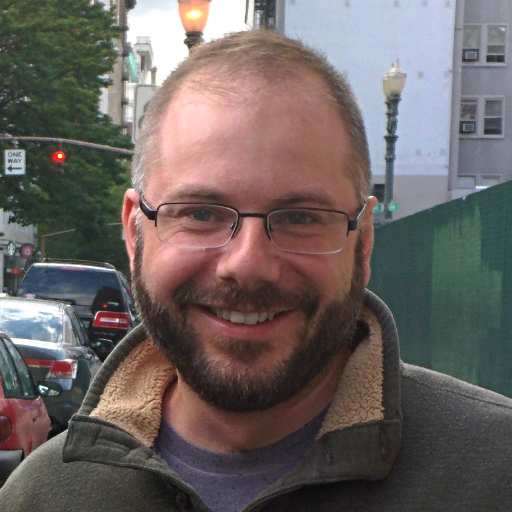
I literally just finished writing the section of my upcoming zine that covers these two topics. I haven't finished the proofreading and editing, but I hope these explanations help. (I'm interested in any feedback on whether these are helpful explanations too!) :)
You can skip the first page on the left (page 16).


The only thing the above pages don't cover that's relevant here is the part where it has add(actor,a). That's just a function that adds a value to another table. In this case, it's adding the newly-created "a" table as a value to the "actors" table. (Tables can store other tables as values.)
So basically what's happening in that function is that the make_actor() function is creating a table and calling it "a", and then adding a bunch of values to that table with specific keys like "x" and "y" and "frame". Then after adding the newly-created "a" table to the "actors" table, it returns "a" as the result of the function and can be used wherever the function make_actor() was run.
I hope that helps some!
EDIT: I should note that the first page (page 16) of what I posted isn't relevant to your question. It seemed like you knew what a function was, but just not what return meant. It's just that the first page was part of the same double page spread.


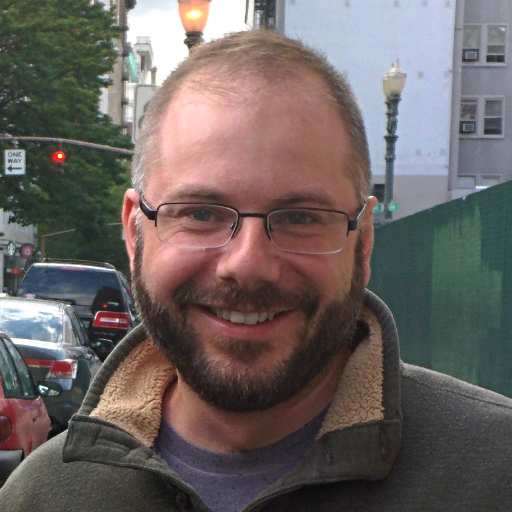
Yup. It allows you to keep a ton of values contained in one variable name.
A lot of game developers refer to the "alive" characters in their game as "actors", hence the name you see used. It's easy to group them together like that because they share a lot of similar characteristics. For example, all actors in a game might have in common the fact that they have an x and y coordinate, a sprite, a direction they're moving, a width and height, etc. Each of those might be different from actor to actor, but they all have them. Tables are a great way to store these kinds of collections of values.
This means you can have a generic function where you just give the function an actor, any actor, and it will be able to do its work without worrying about whether or not that actor has those values attached. For example, maybe you could have a function like this:
function move_actor(a) a.x+=a.dx a.y+=a.dy end |
That function doesn't care which actor you give it, but it can safely assume that the actor ("a") has an x and y coordinate and dx and dy values, because all actors in the game have those.
So the make_actor() function you were looking at up top was just a way to create a generic actor table for the game and give that actor all the normal values an actor should have in the game.


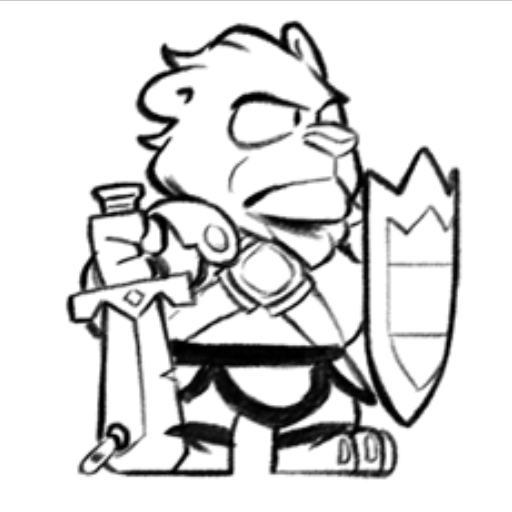
Other languages and scripts I've used in the past did tables differently or not at all, So it took some getting used to but it's the most handy data structure for game programming ever! Position, Lives, Speed, Sprites.. All can be stored within a table!
And unless I'm mistaken, Collections of tables can be stored inside OTHER tables! (At least a friend trying to explain full LUA mentioned something like that.. I might've gotten her wrong tho.)



Lua data is tables, all the way down. Tables hold values, and one of the value types is "table". Global scope is just a hidden table.
Structures and classes are just syntactic sugar on tables, e.g. object.member
is syntactic sugar for object["member"]
, while object:method(...)
is syntactic sugar for object["method"](object,...)
.
It's a cool language. It's not efficient at all, with everything being based on table lookups, but it's incredibly flexible for things that don't need a ton of performance. I've really grown to love it for scripting.


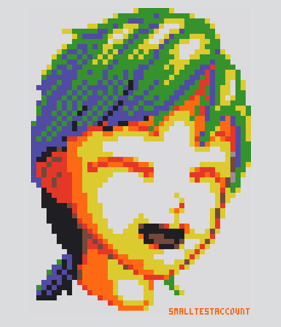
This is way more useful than I thought
thanks to all of the people who answered


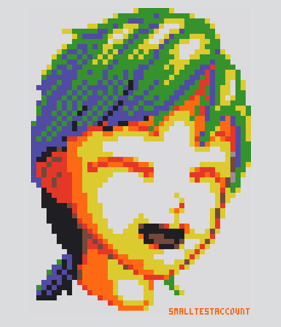
Hey, guys, I'm having an issue changing the values.
I keep getting
runtime error <eof> line 10: attempt to preform arithmetic on glodal 'x' (a nil file) |


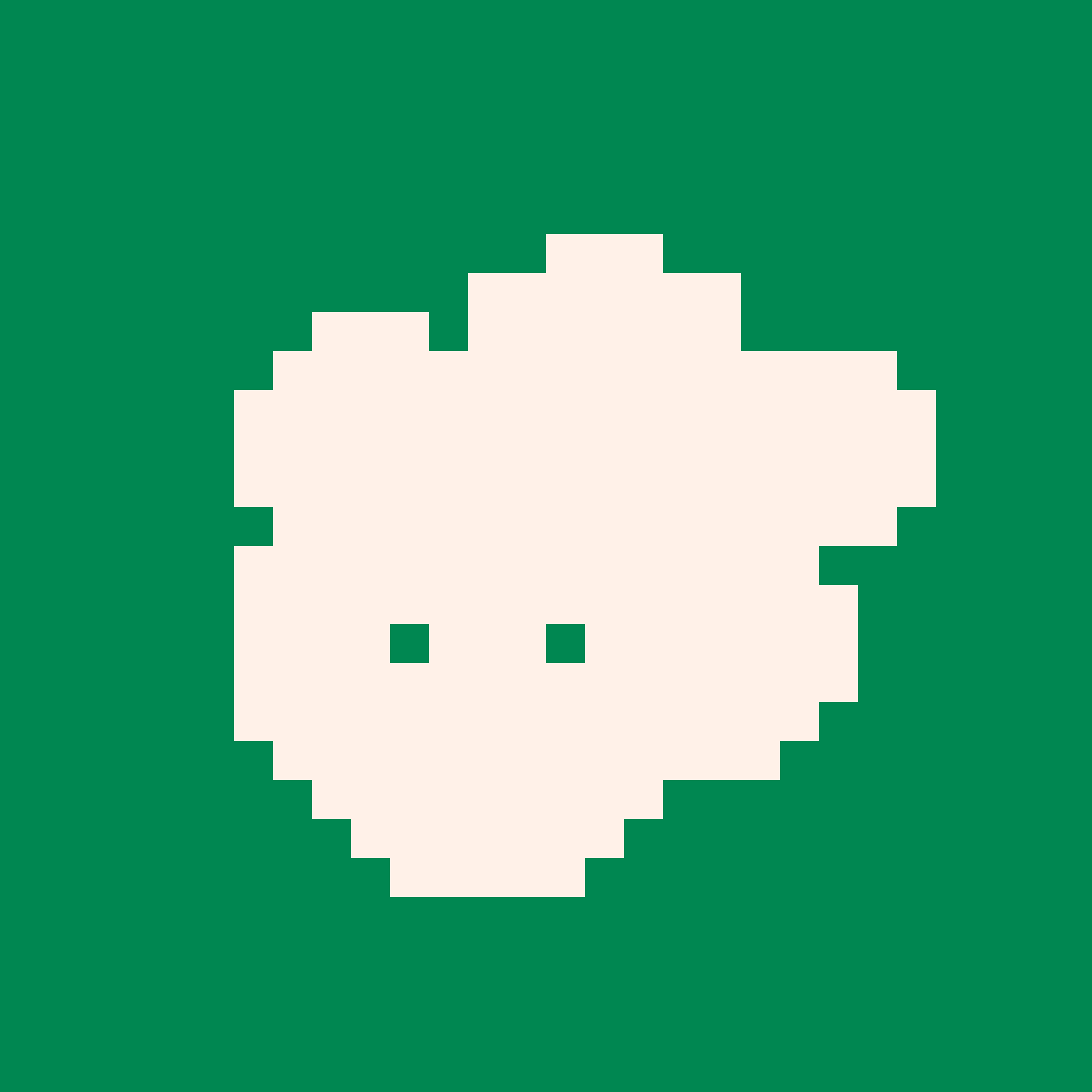
Hi PixelBytes! It's tough to tell without seeing your code, but it looks like your code is running some math on a value that you haven't yet defined (hence the computer being unable to complete the equation). Can you upload your current code, so we can see where your issue is?


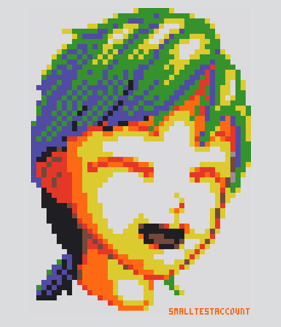
Eggnog
function player() p.x=63 p.y=63 end function _update() if btn(0) then p.x-=1 end end function _draw() cls() print(".",p.x,p.y,7) end |


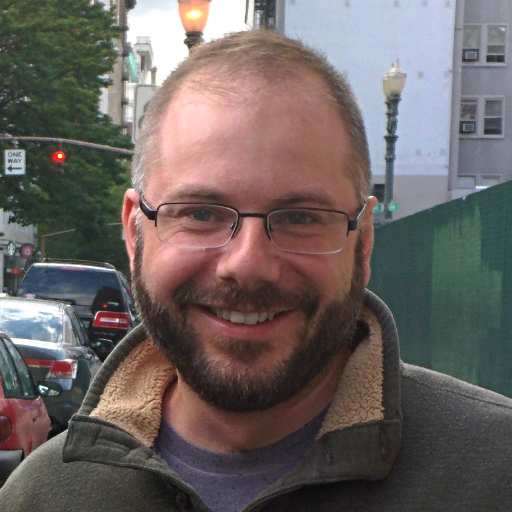
The problem you're running into is that you are defining p.x and p.y, but you haven't defined what p is yet. Add p={} before you set p.x and p.y in the player() function. That will create an empty table called p, which will then allows you to set the x and y keys of that table.
And just as an aside, if you just want to draw a single dot for the player, you could use pset(p.x,p.y,7) instead of print().


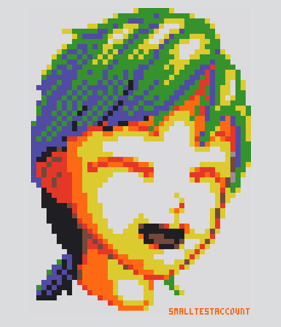
MBoffin
It worked, but I had to get rid of function "Player()" and have the variables out in the open in order to work, but why is that?



@M~Boffin when do you think the zine will be out? And where will it be available? Thanks!



You didn't happen to write the suggested "p={}" as "local p={}", did you? That would explain it not working when the code is inside a function, since local variables only exist while the function is running.



actually you only defined player() but didn't call it at all. either call it "in the open" or in _init()
function _init() player() end |
pico-8 first executes the code "in the open" till the end of the file (that's when functions are defined, but not called), then it calls _init(), then it loops with _update() and _draw().


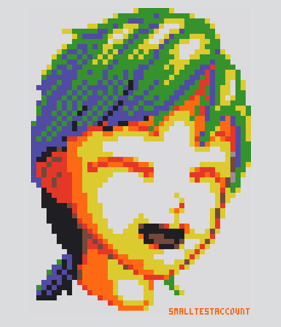
Ultrabrite
Thanks, works now ^-^
I never found a use for "_init()" for my code before, so I never think about it.


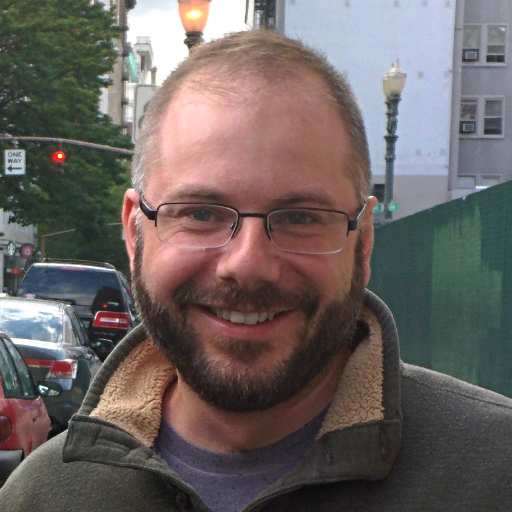
@penthouse_guy I'm giving away printed versions for a workshop I'm giving soon. Once that happens, I'll have it on itch.io for download and I'll likely do a Kickstarter or something to cover costs for those who can't attend the workshop, but would still like a printed version.
[Please log in to post a comment]