-- converts anything to string, even nested tables function tostring(any) if type(any)=="function" then return "function" end if any==nil then return "nil" end if type(any)=="string" then return any end if type(any)=="boolean" then if any then return "true" end return "false" end if type(any)=="table" then local str = "{ " for k,v in pairs(any) do str=str..tostring(k).."->"..tostring(v).." " end return str.."}" end if type(any)=="number" then return ""..any end return "unkown" -- should never show end |
Example usage:
> print(""..tostring({true,x=7,{"nest"}})) { 1->true 2->{ 1->nest } x->7 } |



nice! would be a great addition to the pico8 api. tables are a pain to debug.



I imagine a lot of us have made something similar. I have a ton of pretty-printing stuff. Lua's string-ifying isn't the greatest in the first place, and even then, Pico-8 doesn't provide its full facilities. Pretty inconvenient when you have to debug with print(). It'd be nice if there was better native support.


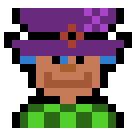
@Felice,ultrabrite I totally agree, debugging in pico-8 is hard and not being able to print whatever, makes it even harder. Would be cool if pico-8 did this natively.



It would be much simpler if the tostr() function just converted as table to a string of the table's values rather than just "[table]". I always have to make for loops in order to see what in a table is causing me issues.



Thank you for your code. While debugging I was looking for a function that would do the exact same thing.
I took your code and improved upon it.
-- converts anything to string, even nested tables function tostring(any) if (type(any)~="table") return tostr(any) local str = "{" for k,v in pairs(any) do if (str~="{") str=str.."," str=str..tostring(k).."="..tostring(v) end return str.."}" end |
This will print like this
> t = {5, {c="b"}, 'a', true} > print(tostring(t)) {1=5,2={c=b},3=a,4=true} |
[Please log in to post a comment]