Hello everyone, I'm currently working on a platformer that is almost complete. The problem I have now is that I'm all out of flags. All the flags I set on different sprites are connected to certain mechanics. Now I need another one, and I'm out of ideas on how to do this. I love flags, I have overused them I feel. Any suggestions would be awesome! Thanks


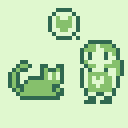
are the features mutually exclusive in any way?
edit: i guess i should elaborate: if some of the features are never used together, it's possible you can use the flags for a more efficient binary encoding, if you aren't already doing so.


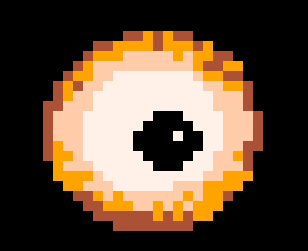
No, that's also the problem, I've tried setting a new sprite with 2 flags enabled, but then the logic of writing
if (fget(val, flag1) and fget(val,flag2)) then
enables both the effects of the flags.


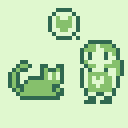
do you need the lookups to be fast (done in a tight loop)? if not, you can have a lookup table indexed by the sprite number, like so:
sprite_features = { [0] = {'stationary', 'boss', 'deadly'}, [1] = {'stationary', 'turret'}, -- etc. } |
edit: this might even be fine in a tight loop if there are few sprites to check
edit 2: probably this is a better idea:
sprite_features = { [0] = {stationary=true, boss=true, deadly=true}, [1] = {stationary=true, turret=true}, -- etc. } |
later…
if sprite_features[the_sprite].boss then -- etc. end |
(also put previous code in code block)


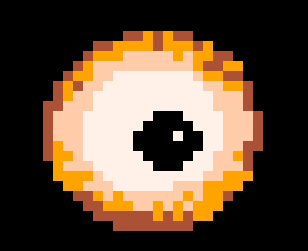
Thank you so much! I'll give this a go tomorrow. I've been sitting way to long today!



enables both the effects of the flags.
That's how it should work. The correct way to then check for flags in the manner you want would be:
if fget(val, flag1) and not fget(val,flag2) then ---example: jump elseif fget(val, flag2) and not fget(val,flag1) then ---example: swim elseif (fget(val, flag1) and fget(val,flag2)) then ---example: run end |



You could also treat is as binary.
instead of:
0:10000000 .. fget(x,0) 1:01000000 .. fget(x,1) 2:00100000 .. fget(x,2) 3:00010000 .. fget(x,3) 4:00001000 .. fget(x,4) 5:00000100 .. fget(x,5) 6:00000010 .. fget(x,6) 7:00000001 .. fget(x,7) |
use a binary representation:
0:0000[0000] .. fget(x,0)==false and fget(x,1)==false and fget(x,2)==false and fget(x,3)==false and ... 1:1000[0000] .. fget(x,0)==true and fget(x,1)==false and fget(x,2)==false and fget(x,3)==false and ... 2:0100[0000] .. fget(x,0)==false and fget(x,1)==true and fget(x,2)==false and fget(x,3)==false and ... 3:1100[0000] .. fget(x,0)==true and fget(x,1)==true and fget(x,2)==false and fget(x,3)==false and ... 4:0010[0000] .. fget(x,0)==false and fget(x,1)==false and fget(x,2)==true and fget(x,3)==false and ... 5:1010[0000] .. fget(x,0)==true and fget(x,1)==false and fget(x,2)==true and fget(x,3)==false and ... 6:0110[0000] .. fget(x,0)==false and fget(x,1)==true and fget(x,2)==true and fget(x,3)==false and ... 7:1110[0000] .. fget(x,0)==true and fget(x,1)==true and fget(x,2)==true and fget(x,3)==false and ... 8:0001[0000] .. fget(x,0)==false and fget(x,1)==false and fget(x,2)==false and fget(x,3)==true and ... ... etc |
So instead of seven layers, you have 256 layers


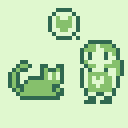
@josefnpat: that would give you 256 distinct values, not 256 on/off flags. this was the basic idea behind my suggestion: if some values never occur together, then they become an enumeration that you might be able to binary encode. if they all can occur together, you're out of luck and eight on/off flags is already as good as you can get.



@josefnpat: in that case you're better off using the full flag when you do the comparison:
if fget(x)==0 then elseif fget(x)==1 then ... elseif fget(x)==255 then |
You could also store a second set of flags for all your sprites:
moreflags = {} moreflags[1] = 1 moreflags[2] = 2 moreflags[4] = 3 |
Where the index is your sprite number and the value is your flag.
This is a function that allows you to read flags similar to fget:
function bitget(value, bitindex) return band(value, shl(1, bitindex)) != 0 end |
you can also read flags in a variable like this:
band(g, 2) == 2
Where g is your variable, 2 is the total of the flags you want to check. If the flags that total 2 are set, then band returns the total of the flags, and the equation is true.
---8421 --flags 01 0001 02 0010 03 0011 04 0100 05 0101 06 0110 07 0111 08 1000 09 1001 10 1010 11 1011 12 1100 13 1101 14 1110 15 1111 |
Looking at this chart, if you wanted spot 4 and 2 to be true, then you total them together, 6, and you check band(variable, 6) == 6. If that is true, then flags 4 and 2 are set. I'm only showing 4 flags here, but you can work with a total of 16, but if you use all 16 then you have to contend with the positive and negative sign, which is what the last digit is for pico variables.


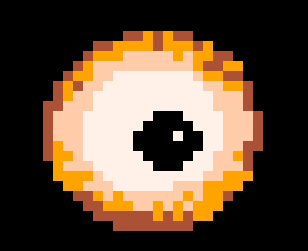
Thanks for all the answers! I managed to work this out in another way. I will be sure to use some of your ideas the next time I run into this problem.
[Please log in to post a comment]