How would I go about doing something like
finisher=4 --or finisher="4"
attack..finisher()
and having the second line trigger a function called attack4 as though "attack4()" were written there?
I'm currently doing it like
IF FINISHER==4 THEN ATTACK4() END
but I'm pretty sure there's a much better way to do it if I only knew the formatting: what is it?
I wonder if I just didn't test guesses enough.
I think this belongs in "Workshop" but I personally don't think most people here really adhere to that...


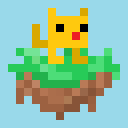
You could do something like this with tables:
attacks = { attack1, attack2, attack3, attack4 } finisher = 4 attacks[finisher]() -- calls attack4 |
If you don't do any math with the finisher variable, you could also just do this:
finisher = attack4 finisher() -- calls attack4 |


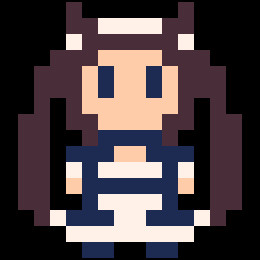
Oh! I see! So what I wanted isn't most accurately possible but there is indeed an easy setup that allows something similar! Thanks a bunch! This new knowledge should be easy for me to integrate into something I'm working on and will save me very many lines of code!
Thank you! 🥰🥰🥰🥰🥰💛💛💛♥️
EDIT: I can't believe that works... that seems so weird to me. Thank you!


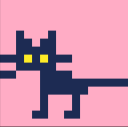
Your problem may have been solved already, but I'll write about the following as one of the ways to solve things flexibly.
(I was just checking something equivalent to eval or exec to see if it could be used for something)
sc = [[ print("hello") return "my script response" ]] f = load(sc) if not f then print("script error") else res = f() print("finish. response: " .. res) end |
It can also include error handling, so it may be useful.


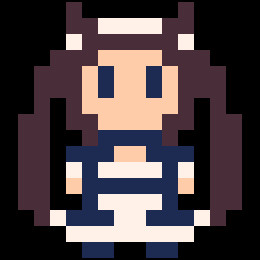
I'm sorry but I think you are too smart for me... I can't really understand what you're showcasing. / .w .\


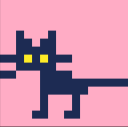
This is an example of executing a lua script written in a variable called sc.
If you are sure that there are no errors in the variable contents, you can write it as follows. The return value is also omitted.
sc = "print('hello world')" -- Insert a lua script into variable fn = load(sc) -- Use the load function to make it executable fn() -- Execute |
I presented this as one way to execute the contents of a variable as a script. I hope it will be useful someday.


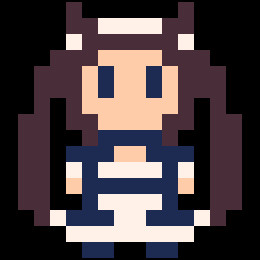
I think I understand now!
So, with the ideas in my original post, it would be like this:
finisher = attack4() --Function trigger from variable
currentattack = load(finisher) --Allows currentattack() to execute the script from finisher ("attack()" in this case)
currentattack() --Executes attack4()
The bottom two lines enable me to set finisher anywhere in the program and have whatever its value is (a function in this case) to be executed whenever my program goes to those two lines without having to change either of said lines.
Am I understanding correctly?
Thank you for wanting to help me! 🥰🥰🥰🥰🥰💛💛♥️
I am not as experienced as you are so it was difficult for me to understand.



@sugarflower this is working but really an overkill solution.
A simpler alternative is to use env:
function attack4() print("attack 4!!") end local atk=4 -- look up the global function dictionary and call _ENV["attack"..atk]() |


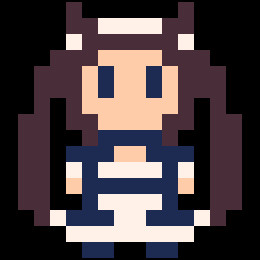
The first solution perfectly suited my needs just fine but I think your solution might even be more efficient.
Could I have some more information about ENV? I couldn't make sense of its entry in the manual.
It's not limited to local variables, right?


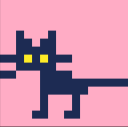
@freds72
It's certainly an exaggeration of the situation.
This is just one example of a method like this.
I intended to write it that way from the beginning. I'm sorry if it was confusing.


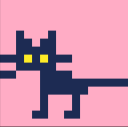
_ENV
or _G
allows you to refer to a list of callable functions, variables, etc.
This is not limited to picotron; it works the same way when using lua.
I was creating a Cart that outputs the contents of _G
and _ENV
to the clipboard to check if there were any hidden functions.
https://www.lexaloffle.com/bbs/?tid=142256
These manage where functions and variables are located in memory, so if you know their name you can find their reference, and if it's a function you can actually call it by adding ().
For example, print can also be called like this:
_ENV["print"]("hello world")


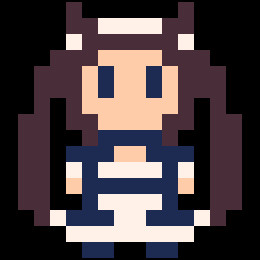
Thank you so much!
I was wary of ENV kinda prior because it's listed in the manual under System and the first command in System is for debugging so I was wondering "is this a temporary feature that shouldn't actually be used in programs?" But you saying it's in normal Lua, not just the still-in-development Picotron, reassures me.
So, after looking up ENV a bit to try to find more information and reading the information you provided, I've reached the following understanding: ENV processes various types of data (functions, strings, variables, etc.) from both global and local contexts and can even manipulate (such as concatenate) them or even perform them as a function.
Is that accurate?
Thank you both for helping me learn about this useful tool for data interaction across multiple types.


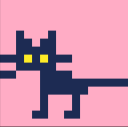
_ENV refers to a table of variables, functions, etc. that are visible in the current scope.
The difference is that _G is a global reference.
You can manipulate variables and execute functions.
If you need to know the actual type of the referenced item, you can find out with:
target = "print" print(type(_ENV[target])) |
In this case, function is printed.
That is, it is an executable function.


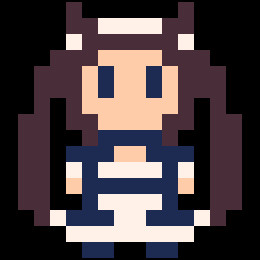
OH! I see! So I was basically correct but ENV doesn't check global things like I thought -- only local things... right? G , however, DOES check everything.
Thank you!


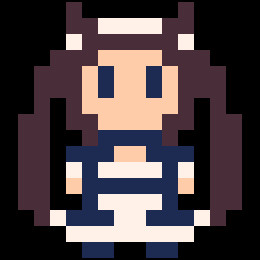
Freds72!
Thank you so much! The principles of that solution you taught me not only desirably suited my syntax and context perfectly but I was able to apply them in some very dirty situations (I'm talking multiple lines of code that just do hardcoded things based on the value of a certain variable) to make those sections perfectly beautifully clean! Thanks a bunch. ^w^ <3
[Please log in to post a comment]