A basic sokoban codebase. Maybe 25 lines of hopefully very easy to understand code. Most of the games I've been making over the last few months all run off this basic framework, and I wanted to have this out there in case it was helpful to anyone else! The "vibe" is based a lot off of things like Bitsy and Puzzlescript, but the simplicity of the code and fact that I've been able to make really fun and expressive games off this paradigm I think makes it really nice. Some games that use this as their base logic include:
- Sebastian's Quest https://www.lexaloffle.com/bbs/?tid=146554
- Vampire vs Pope Army https://www.lexaloffle.com/bbs/?tid=146644
- Rat Dreams https://www.lexaloffle.com/bbs/?tid=145325
Anyways, please enjoy!
WHAT'S THE BASIC IDEA
All my games like this have a simple approach, which is to check what the player WANTS to do and then see if that's possible or not BEFORE doing it. The guts of my simple sokoban setup is just three steps:
INPUT()
- First, see what direction the player wants to goPUSH()
- Second, check if there's anything to push out of the wayMOVE()
- Third, check if we are clear to move to an open space or not.
This is a pretty powerful approach and can be expanded or extended to use bigger rectangles, mixed shapes like tetris shapes, or even matching chunks of tilemap against other chunks of tilemap (my game DUST SETTLERS does this).
When I'm doing Bitsy or Puzzlescript style games, I tend to store the player position as a tile coordinate, not a real coordinate, since it drastically simplifies steps two and three from above. When I draw the player sprite, I multiply the X and Y values by 8 to put it in the right spot on the screen.
This approach is also very deliberately based on taking as much advantage of the PICO8 tilemap as I can, usually from the start of every prototype. It's such an awesome way to manage game logic and interactions. Plus it's fun to plop little colored squares all over and see what happens...
ABOVE AND BEYOND
Fun things to add or expand on from this base might include interactions like what happens if you push an egg into another egg? what happens if you push an egg into a wall? can the bird lay more eggs? Or you could add animations to the bird by drawing more sprites and changing when they appear. You could extend the map and have the camera follow the bird around on their adventure. You could change the eggs to be waffles. You could add holes to push the eggs into. You could give the bird a "fly" mode where instead of pushing eggs they can eat bugs or something. You could have the eggs hatch every 20 steps and now you control more birds. Every time the bird moves it could leave a new obstacle behind it.
THE TEMPTATION WAS TOO GREAT
I wrote a version of this logic that fits into 256 characters... this is not good as an introductory or exploratory exercise, however, it was fun, and doing fun things is what pico8 is all about !! https://www.lexaloffle.com/bbs/?pid=161737#p


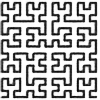
Nice starting framework. Beware that you can cheese moves and pushes by pressing a horizontal and a vertical direction on the same frame.
For example , a diagonal push can unstuck eggs from a two by two solid formation.



good catch! i left that in for this sample as an exercise for the new coder... normally i have a line at the end of input() that forces that function to return only a single axis. usually i would also bail on the actual move and push checks if there's no NX or NY values too! i think these are good for folks to notice and modify on their own tho


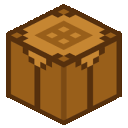
i tried to reduce the code size a bit and i think that the input() function could have a little less if statements
so from this
function input() nx = 0 ny = 0 if (btnp(0)) nx = -1 --left if (btnp(1)) nx = 1 --right if (btnp(2)) ny = -1 --up if (btnp(3)) ny = 1 --down end |
to this
function input() nx=tonum(btnp(1))-tonum(btnp(0)) --horizontal ny=tonum(btnp(3))-tonum(btnp(2)) --vertical end |
not sure if it makes it better nor if it even works but it should



oooh that's cool! i will keep that in mind for future minification attempts or if i ever do a proper little utility library for myself. for this project i specifically wanted to prioritize legibility for folks either first attempting or returning to pico8 - so make sure i'm using mainly only the commands from the introduction parts of the manual, and keeping the code "Small" just so someone can finish reading it without getting too bored hopefully... and make them feel like "oh, that's doable!"
i did do a 256 char version of this codebase for fun too tho, the temptation was too great lol https://www.lexaloffle.com/bbs/?pid=161737#p


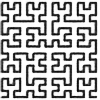
Call me paranoid but the previous poster feels very sketchy : account with broken icon, single post, completely generic comment, witch is even not relevant for a game engine "game"... This feels like a test post in preparation for spam.
Or maybe the broken icon is the consequence of code injected inside the icon file name that got sanitized by the web site ?
I'm flagging it as spam, sorry to the website team and to the user if I'm mistaken.


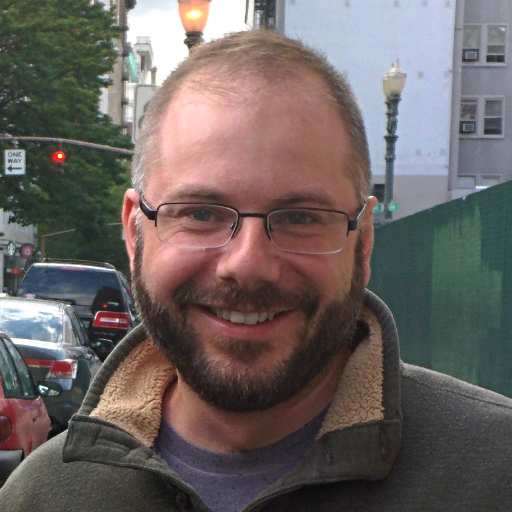
@RealShadowCaster Don't feel bad at all. You'd be shocked (or not, I guess) how much of that kind of spam we get. Normally for obvious spammers, I nuke their whole account. But if it's on the edge of being able to tell and it looks a bit suspicious and it doesn't actually add anything to the conversation, I can also just nuke that one post. Either way, y'all have had pretty good "gut feelings" in this area, so don't feel bad marking something like that. :)
[Please log in to post a comment]