Hello everyone
I want to add an item for pick up on my procedural generated levels.
I have a pick up quantity and a table to hold them, and an individual table for each object.
I want items to be placed on tiles with the flag 5 because it's a busy street where my character can't get to a lot of the places.
Seemingly my only option is to use a while loop to iterate through x and y positions until it finds a flag 5 and then discount 1 from the pickup quantity until it's 0.
Unfortunately this results in the game not loading.
while crateamt > 0 do local x = rnd(map_end) local y = rnd(map_endy) if fget(mget(x*8,y*8),5) then add(crates, crate:new({ x=x, y=y })) crateamt -=1 else x = rnd(map_end) y = rnd(map_endy) end end |
What other options do I have?


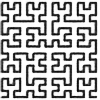
I'm guessing map tiles with flag 5 are quite rare, so your code is taking forever throwing the dice until it has landed crateamt times on a lucky square.
That way of drawing is only viable if the probability of success is high enough.
Plus you can have two or more crates on the same tile this way.
If you don't already have the info somewhere, loop over the entire grid, and add the flag 5 coordinates you encounter into an array.
After that, you can draw one set of flag 5 coordinates at random in the array, add your crate, and remove the picked set from the array.
Do that crateamt times and you're done. Just be sure that crateamt is at most equal to the number of flag 5 of your grid.
Also, mget(x,y) is in tiles, so the times 8 seems wrong to me.
flag5s={} for x=0,map_end do for y=0,map_endy do if fget(mget(x*8,y*8),5) then add(flag5s,{x=x,y=y}) end end end for cpt=1,crateamt do idx=1+flr(rnd(#flag5s)) add(crates, crate:new({ x=flag5s[idx].x, y=flag5s[idx].y })) deli(flag5s,idx) end |


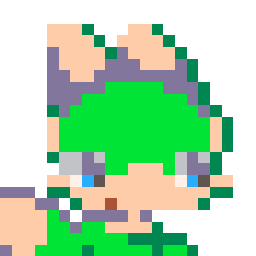
If map_end and map_endy are 15, rnd(map_end)
and rnd(map_endy)
will never become 15.
In other words, fget()
will not be performed for mget(15,0~15)
, mget(0~15,15)
.
[Please log in to post a comment]