In the following code, everything works apart from table.sort(). How would I know that?
Also, the only keyword highlighted in the code editor is "sub"
s = "hello world" i, j = string.find(s, "hello") print(i..":"..j) print(string.sub(s, i, j)) print(string.find(s, "world")) local t = {} table.insert(t, 1) table.insert(t, 24) for i=1, #t do print(t[i]) end table.sort(t) |


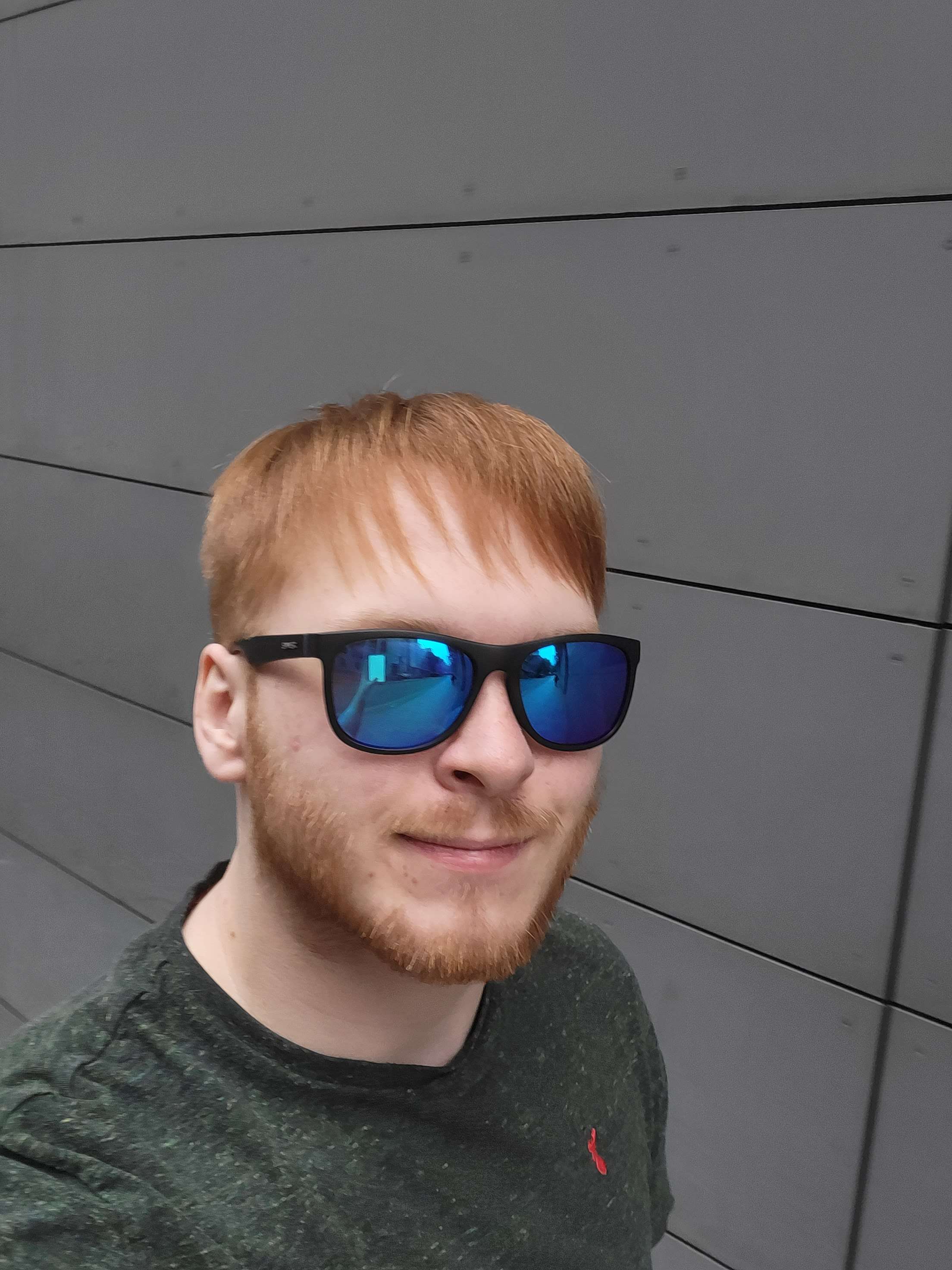
I've found the easiest way to know what functions are available is to check Enview: https://www.lexaloffle.com/bbs/?tid=140816
As an alternative to table.sort, you could try userdata.sort which can work as a replacement in some cases, but it only works with userdata, not tables. You can see an example of how to use it in demos/carpet.lua.
If that's not helpful for your case, abledbody's quick sort implementation is very good: https://www.lexaloffle.com/bbs/?tid=142305
And 'sub' is only highlighted because it's the name of a PICO-8 function.
I hope the highlighting implementation is improved at some point to avoid this kind of thing from happening.



Hmm...this is a bit weird, actually. The spec says that the picotron API is based on Lua 5.4, so it doesn't necessarily have to include all of the standard library. However, that's the only function in the table library that doesn't seem to be included. That makes me wonder if perhaps Zep is making custom implementations and just hasn't gotten around to it yet.
Anyway, in case it's helpful, I went ahead and made an variation on abledbody's quick sort function from the link JoshK provided that matches the specs for table.sort(). Besides the allowance of a custom comparison function, I took out the comments for brevity, adjusted the comparisons to match the Lua 5.4 spec (which uses < rather than <= ), and took out the irrelevant do block (the part in the comments that mentions "discarding" the locals gives me the impression of listening to tutorials that don't understand how garbage collection works). I also took out the pico-8 features so I could test it using the reference interpreter.
Here it is:



Thanks both for your responses. I'm guess what I'm struggling with is how do I know what is supported? Why have a partial table implementation? It's not documented in the manual so is it likely that if I use some of these Lua functions they won't exist in a later Picotron version?



The roadmap page has this at the bottom in the "notes":
"Carts will be mostly backwards compatible starting from 0.1, but not guaranteed until 0.3".
That said, there's not really any reason to take out functions unless they're actually making the user experience worse, so I suspect that the roadmap is referring to the picotron specific functions having the possibility of changing.
As for why to have a partial table implementation, my best guess is that some of the standard library functions are close enough to pico-8 functions to be easily included.
Other than that, I think the quickest way to know if a specific function is currently supported is to try printing it in the console with print(). If the function is nil, then it's not supported. If it is supported, then the console should print "[function]" to let you know it's a function.
[Please log in to post a comment]