So I'm trying to use two sprite sheets per frame in my game using the new high memory video mapping. at the start of the game the video memory is moved to 0x8000. there are special sprites that are loaded from a string into 0xa0. What im trying to do is quickly switch to 0xa0, load/draw the compressed sprite, then switch back to the 0x80 sprite sheet. for some reason loading the sprite into 0xa0 overwrites sprites at the same position in 0x80, which is my main issue here. if anyone has advice or help id appreciate, i understand its a new feature so its not as well documented just yet.
copying sprites from video memory to 0x8000 (this is done in _init):
memcpy(0x8000,0x0,0x2000) poke(0x5f54,0x80) poke(0x5f5c,255) |
loading sprite data from string into 0xa000 (this is called sometimes during _update within a decoding function):
poke(0x5f54,0xa0) --load 0xa000 memory --buncha stuff that loads decompressed sprite for i=0,4096do sset(slot*64+i%64,64+i\64,0) end local sx,sy,i=32-w\2+slot*64,128-h,1 for y=0,h-1 do for x=0,w-1 do sset(sx+x,sy+y,img[i]) i+=1 end end poke(0x5f54,0x80) --load back 0x8000 memory |
drawing the sprite loaded into 0xa000 memory (done during _draw as needed during its own function)
poke(0x5f54,0xa0) spr(128+(8*slot),x,y,8,8,flp) poke(0x5f54,0x80) |
EDIT:
i wanted to put it out there that im ok with only being able to draw from 1 of the sheets per frame. as long as i can load gfx from one without corrupting the other.


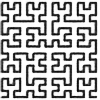
Beware that your pokes are remapping the memory, they are not just parameters used by the display, future pokes are affected.
Keep track of what is currently under what address, and you’ll be able to use sprites from multiple sprite sheets in the same frame.
I’d recommend playing around with memsplore to « view » memory remappings.
Alternatively, you can systematically poke the remap pointers to their original values before poking the memory to reduce headaches.



@RealShadowCaster So if I’m understanding correctly, the location to poke for changing sprite sheets moves when I use it, so I’ll use memsplore to figure out where it goes. I don’t understand the alternative method. Also thank you for your help


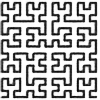
Things move around when you modify the sprite and map pointers.
The currently active sprite sheet is always at address 0, but when you poke 0x80 into the sprite sheet address (0x5f54), the memory segments switch address.
In memsplore, when you poke 0x80 in 0x5f54, the icons disappear, and you also see that the content at address 0 is now 0 all the way.
if you change the content of that section, you can make icons change and appear, and if you poke back the original value into the sprite sheet pointer, your original icons are back intact. Your pokes into the sprite sheet are still there, but visible at address 0x8000.
If 0x5f54 is 0x80 and you poke at 0x8000, you are actually modifying the original sprite sheet that is currently remapped to the 0x8000 address range.
Lastly, the 0x8000-0xFFFF range is not visible in memsplore as it is all zeroes and was not even active by default at the time the tool was written. It's easily patch-able if you want, but the current version is already enough to figure out what's going on.



@tedblue - the whole point of hi-mem is to avoid copying data around.
and if you need, suggest to look at memcpy and/or poke4.
these 2 functions copy whole chunks of bytes much faster than using sset



@RealShadowCaster youre gonna love this, ive been in 0.2.5g this whole time 💀
good news is it works now. Thank you for teaching me so much about how this works!
[Please log in to post a comment]