The Question
Hello everyone,
I am ludicrously close to finishing the engine for my game, but some physics equations have me stuck. Here is what I'm trying to achieve:
- The players are effectively nodes that can switch between being pendulum bobs and fulcrums. One can be anchored to serve as a fulcrum while the other swings. If both are anchored, neither moves. If both try to un-anchor themselves, they fall.
- In order to let the players navigate the playfield, I want the swing of a pendulum bob to increase bit by bit. (This will let players climb the playfield by swinging upward instead of just descending.)
- Once a bob makes a full loop around the fulcrum, I want it to continue in a circle at a more-or-less consistent speed.
With the help of this math writeup, I managed to assemble a simplified set of physics variables and equations that do an okay job of simulating momentum and creating a non-degrading swing (i.e., the swing distance never shrinks due to friction). But I haven't figured out how to make the desired increase in swing distance happen. I'm sure it's a single value or sign that I haven't identified.
I suspect I need to do something to increment the value of p.a
in the function move_player(p)
, but I am not sure how to approach it -- adding a value, multiplying by a value, etc.
Could someone better at physics/math please offer suggestions?
Thank you for any and all pointers!
The annotated code is below. It's 200 lines in total, but the relevant parts are the first ~90.

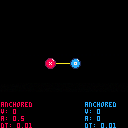
The Code



Try this: don't change dt
, and instead do something like:
p.v+=(k*cos(p.a)+0.01*sgn(p.v))*p.dt |
This adds a small angular acceleration in the direction of the current velocity, and should increase the angle of swing over time.


.png)
This works beautifully! I'd never heard of sgn()
before, either, but I can see it being quite useful.
All I need to do now is figure out how to speed up the swing from here.
Thank you, @luchak. You've helped me solve the last major obstacle in my game. Now for all the stuff I actually know how to do!


.png)
I actually did end up keeping the dt
updates, because they made for a more pleasing swing speed. With a simple acceleration cap included, everything works nicely.
Thanks again, Luchak!

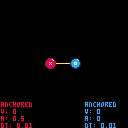
[Please log in to post a comment]