hi, i'm loving picotron so far! i just wanted to report some issues using coroutines.
when using coroutine.resume, it can sometimes return early when the coroutine runs for a while due to how picotron does timeslicing. from reading head.lua, i learned that using coresume instead of coroutine.resume fixes this, which makes me think maybe coroutine.resume should be replaced with it. another more minor issue is that coresume only returns the first value of a multiple-value return/yield from the coroutine, and not any more.
here's a simple test case:
local function long_running_coroutine () for i = 1, 100 do cls() end return 1, 2 end function working () local c = coroutine.create (long_running_coroutine) coresume (c) assert (coroutine.status(c) == 'dead', 'this works') end function not_working () local c = coroutine.create (long_running_coroutine) coroutine.resume (c) assert (coroutine.status(c) == 'dead', 'this doesn\'t work') end function also_not_working () local c = coroutine.create (long_running_coroutine) local a, b, c = coresume (c) assert (a) assert (b) assert (c, 'only one coroutine return value returned') end function _draw () working () not_working () also_not_working () end |


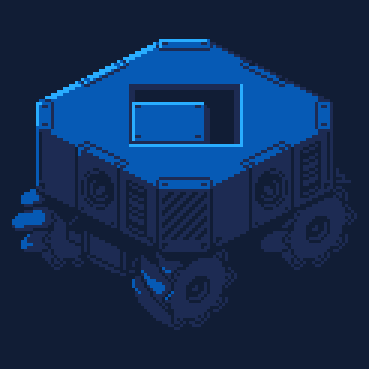
The code for coresume
is inside /system/lib/head.lua
at around line 1078. By the looks of it, coroutine.resume
requires some extra care and the current function only returns 2 values. (assuming the first returned value is related to the status.) It would require the use of pack
and unpack
in order to return more values.


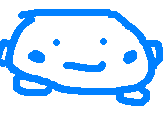
oh this makes sense! in pico-8, coresume returns true, data
if everything was fine (data = what is yielded) and false, error
otherwise
[Please log in to post a comment]