Hi there!
I've been using a relatively simple system for sprite animations that I find works pretty well without much overhead. It relies on a simple function to retrieve the current frame of an animation frame list (ani
) based on time
(t()
) and a given speed (spd
) in frames per second:
--get animation frame function anifrm(ani,spd) return ani[1+flr(spd*t()%#ani)] end |
That's it!
Now all we need is to define an animation as a list of sprite IDs:
--define player walk animation pwalkani={2,3,4,5} |
And we can assign this animation to our player just like assigning a single sprite:
--in _update(), during walk logic (e.g. btn left or right) player.sprite=anifrm(pwalkani,10) --in _draw(), using the normal spr() call spr(player.sprite,player.x,player.y) |
Here's an example cart to demonstrate fully, also taking sprite flipping into account:
Full code:
Be aware that because this function is tied to global time (t()
) the current frame shown isn't dependent on when the action began/the animation was set.
Good luck and feel free to let me know if there are any questions or comments about this function or its usage!


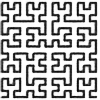
Nice. For those who need the timed animation to be relative to the start of movement, I added a 3rd optional parameter that is the time stamp of the start of the animation.
Full code
[Please log in to post a comment]