I needed a BigInteger implementation in Pico-8, so today I made one with the help of Sonnet 3.5.
The cart includes tests and test utility functions. Copy only the relevant code for you project.
It's not minified/compressed whatsoever, and the big integer functions come in at just under 700 tokens.
The cart is not very interesting in the browser, as it just printh
s the results of an array of test cases.
The cart:
- Download using
load #bigint-0
- Save using
export bigint.p8
- Run using
pico8 -x bigint.p8
The cart code:
The lua library:
The cart outputs:
If you've got suggestions, improvements, bugs, or whatever else, please let me know.
This is also my first post on BBS, so there's probably also something I could do better in regards to that.
Thanks for reading, have fun!


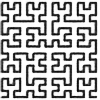
Implementing big integers is how I familiarized myself with metatables and metamethods.
You could start with __add and __mul and __div, but nothing stops you from going overboard as practice :
__unm , __mod , __pow , __band , __bor , __bxor , __bor , __shl, __shr, __eq, __lt and __le
all make sense for big integers.
__idiv is redundant with __div since we are dealing with integers.
Speaking about integer division, you could have used it in your code :
flr(n/10) can be written as n \ 10
For pico-8 games, my preference goes to using string numbers for big numbers, and have a small function for addition and multiplication. Haven't needed big divisions yet, but I now know where to look, should the need arise.
[Please log in to post a comment]