Hello, I'm trying to use a function that uses a power that is less than 1, and for some reason pico will always evaluate it to 0. Is this just a limitation of pico? and any ideas on alternatives to achieve a similar result?
sin( x ^ ( 1 / 3 )) |




I concur that Pico-8 doesn't do the right thing for fractional powers. I get 1 for every power between 0 and 1, and 0 for every power less than 0.
?9^2 -- 81 ?9^1 -- 9 ?9^(0.5) -- 1, should be 3 ?9^0 -- 1 ?9^(-0.5) -- 0, should be 0.3333 ?9^(-1) -- 0, should be 0.1111 |
These operations work in Lua 5.3, so this seems specific to Pico-8's fixed point implementation.
If you're desperate you could use a lookup table, but I would agree that this should get fixed.


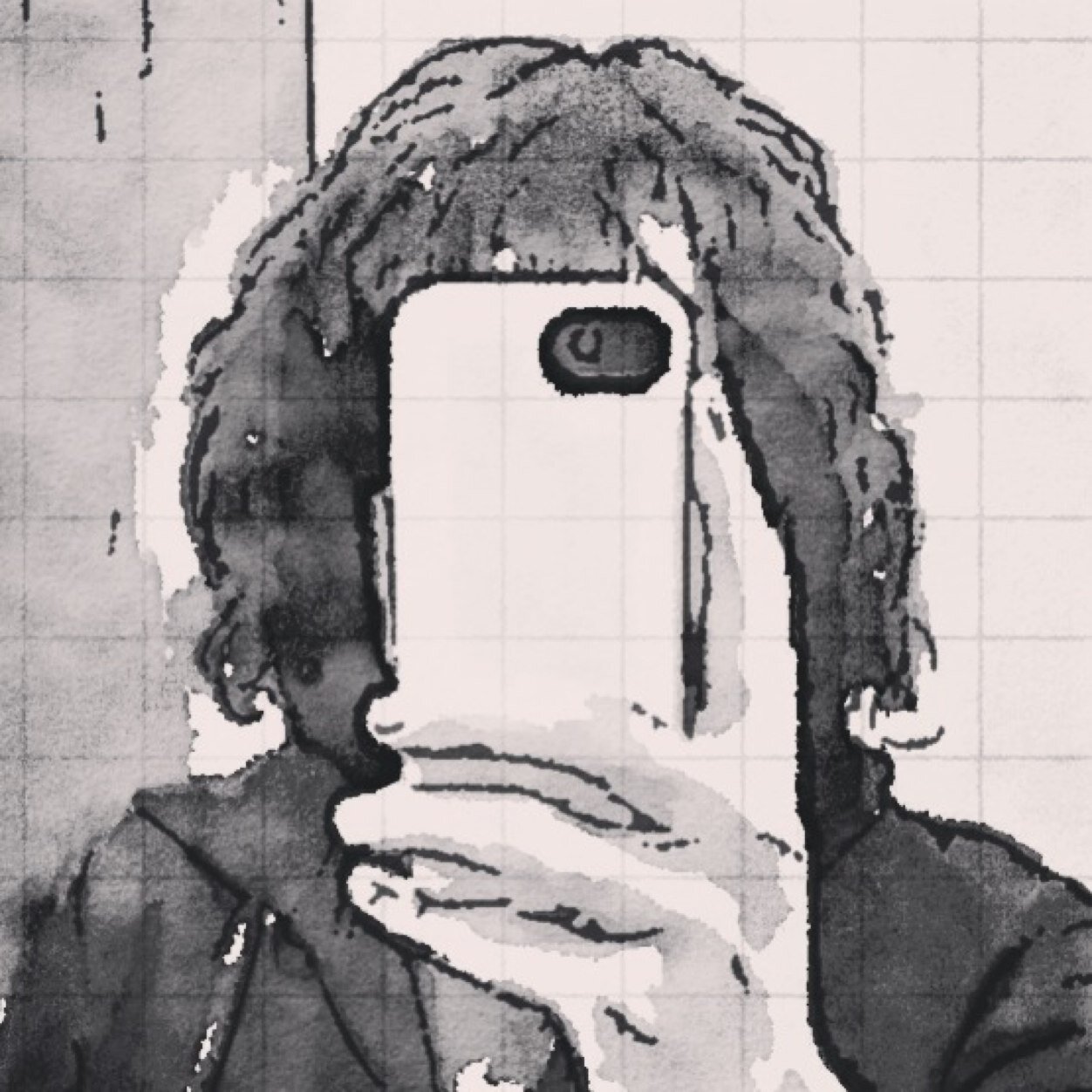
I'm sure there's a more efficient way to write this, but here's a function based on the Newton method:
function nroot(k,n) if(k<0 and n%2==0) return 0 --nan if n==1 then return k elseif n==2 then return sqrt(k) end local num=abs(k) local lowbnd,highbnd if num<1 then lowbnd=sqrt(num) highbnd=1 else lowbnd=1 highbnd=sqrt(num) end local guess=lowbnd+(highbnd-lowbnd)/2 local precis=0.001 local oneoverroot=1/n local deltaguess=32767 while abs(deltaguess)>precis do deltaguess=oneoverroot*(num/guess^(n-1)-guess) guess=guess+deltaguess end return guess*sgn(k) end print("cuberoot of 4: "..nroot(4,3)) print("7-root of -17: "..nroot(-17,7)) print("5-root of 0.4: "..nroot(0.4,5)) |
(since x^(1/3) == nroot(x,3))


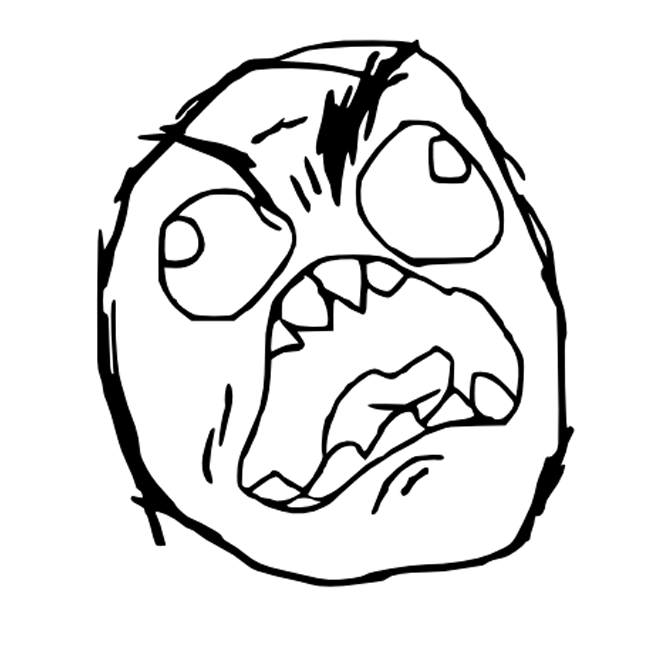
I don’t believe the ^ operator is supposed to be supported (at least it’s not in the documentation). In this thread I provided a pow() function that uses some kind of exponentiation by squaring, it has a pretty good speed vs. precision trade-off.
[Please log in to post a comment]