Just a small snippet from a token-saving discussion on the Discord last night.
If you need to iterate over neighboring tiles (for example when writing a path finding algorithm for 7DRL), this natural approach is pretty token heavy:
-- four directions, 29 tokens for direction in all{{-1,0},{0,-1},{1,0},{0,1}} do local x,y=direction[1],direction[2] end -- eight directions, 45 tokens for direction in all{{-1,0},{0,-1},{1,0},{0,1},{1,1},{-1,-1},{1,-1},{-1,1}} do local x,y=direction[1],direction[2] end -- eight directions, 43 tokens directions={0,-1,-1,0,1,0,0,1,1,-1,-1,1,1,1,-1,-1} for i=1,16,2 do local x,y=directions[i],directions[i+1] end -- eight directions, 30 tokens directions={-1,0,1} for x in all(directions) do for y in all(directions) do if x!=0 or y!=0 then -- end end end |
Why not use trigonometry?
-- four directions, 16 tokens for i=0,1,0.25 do local x,y=cos(i),sin(i) end -- eight directions, 24 tokens for i=0.125,1,0.125 do local x,y=flr(cos(i)+.5),flr(sin(i)+.5) end |

2

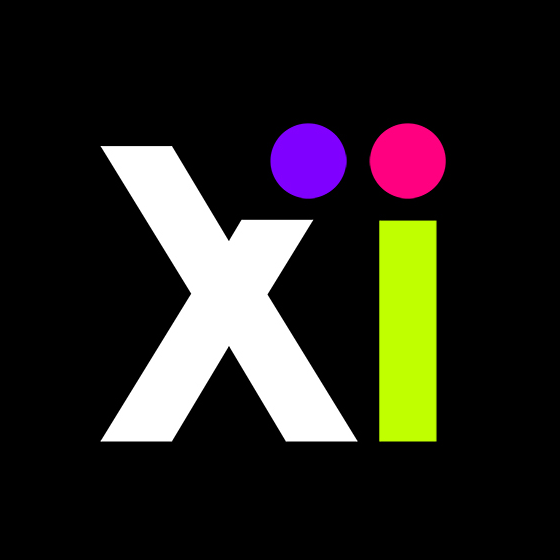
Clever!
Got you beat tho ^_^
-- eight directions, 23 tokens directions=explodeval("0,-1,-1,0,1,0,0,1,1,-1,-1,1,1,1,-1,-1") for i=1,16,2 do local x,y=directions[i],directions[i+1] end |
Provided, of course, you have this defined (which you absolutely should, because it's universally useful):
function explode(s) local retval,lastpos={},1 for i=1,#s do if sub(s,i,i)=="," then add(retval,sub(s, lastpos, i-1)) i+=1 lastpos=i end end add(retval,sub(s,lastpos,#s)) return retval end function explodeval(_arr) return toval(explode(_arr)) end |



Have you benchmarked the different variations? I expect the trig functions to be slower.

1


I'll see your 23-45 tokens with trig or tables or a helper function and raise you [EDIT]19 tokens of just loops and logic...
-- eight directions, 19 tokens for y = 0xffff, 1 do for x = 0xffff, 1, y==0 and 2 or 1 do -- end end |
And a much less competitive bonus...
-- four directions, 24 tokens for d = -2,1 do local x, y = d % 2 * d, d < 1 and d + 1 or 0 -- end |
[Please log in to post a comment]